This document is an example of g + + compilation developed in C/C + + under Linux. If you continue to read this article and hope to gain something, you should have the following skills
- Fundamentals of C + + programming language
- Fundamentals of Linux system operation
Article catalog
1. Prepare source code 2. Compilation practice 2.1. Import Directory for compilation 2.2. use-Wall,-std Parameter to compile 2.1.3. Generate library file Link static libraries to generate executables Link dynamic library to generate executable file The difference between static library and dynamic library in generating executable files
This is the second article in this series. You can read the first article through the following link
1. g + + tools for compiling C + + code in Linux and common operation instructions of g + +
1. Prepare source code
Create the following code files in a working directory. The directory structure is shown in the following figure
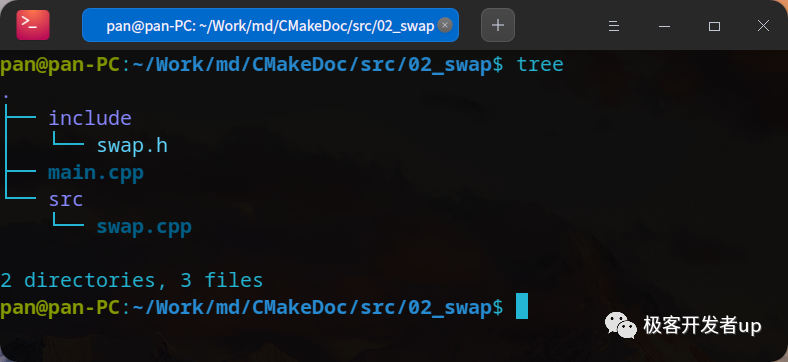
The code is as follows:
swap.h file, which defines the C + + header information
#include <iostream> using namespace std;
swap.cpp file, which defines the C++ code we will call in the main function.
#include "swap.h" void swap(int &a, int &b) { int temp; temp = a; a = b; b = temp; }
main.cpp file, which is the main function file and the entry of function call
#include <iostream> #include "swap.h" using namespace std; int main(int argc, char const *argv[]) { int val1 = 10; int val2 = 20; cout << " Before swap:" << endl; cout << " val1:" << val1 << endl; cout << " val2:" << val2 << endl; swap(val1, val2); cout << " After swap:" << endl; cout << " val1:" << val1 << endl; cout << " val2:" << val2 << endl; return 0; }
2. Compilation practice
2.1. Import Directory for compilation
Direct compilation
g++ main.cpp src/swap.cpp
You will see the error shown in the figure below
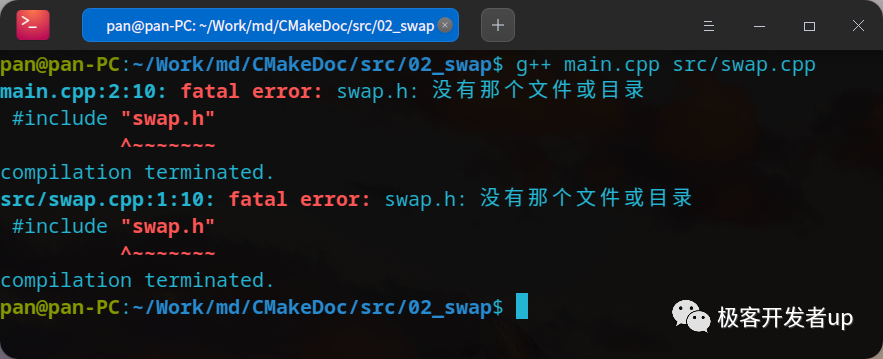
This is because g + + can't find Swap under the include directory H file, so we need to use the - I parameter to include the include directory, as follows
g++ main.cpp src/swap.cpp -Iinclude
At this time, the code can be compiled by the way.
2.2. Compile using the - Wall, - std parameters
-Wall stands for the output of warning information during program compilation, - std stands for compiling using specific c + + standards, as follows
# Compile using the c++11 standard and use the - Wall parameter g++ main.cpp src/swap.cpp -Iinclude -Wall -std=c++11 -o a.out
At this point, we can see that we can compile normally without outputting warnings, and we can also execute a.out normally. Because our code complies with the c++11 standard, in order to demonstrate a warning, we are in main Add a line of code to CPP. as follows
#include <iostream> #include "swap.h" using namespace std; int main(int argc, char const *argv[]) { int val1 = 10; int val2 = 20; // Define a variable, but do not use double d = 0.0; cout << " Before swap:" << endl; cout << " val1:" << val1 << endl; cout << " val2:" << val2 << endl; swap(val1, val2); cout << " After swap:" << endl; cout << " val1:" << val1 << endl; cout << " val2:" << val2 << endl; return 0; }
Change the output file to b.out, and execute the compilation again, as follows
g++ main.cpp src/swap.cpp -Iinclude -Wall -std=c++11 -o b.out
The following warning appears
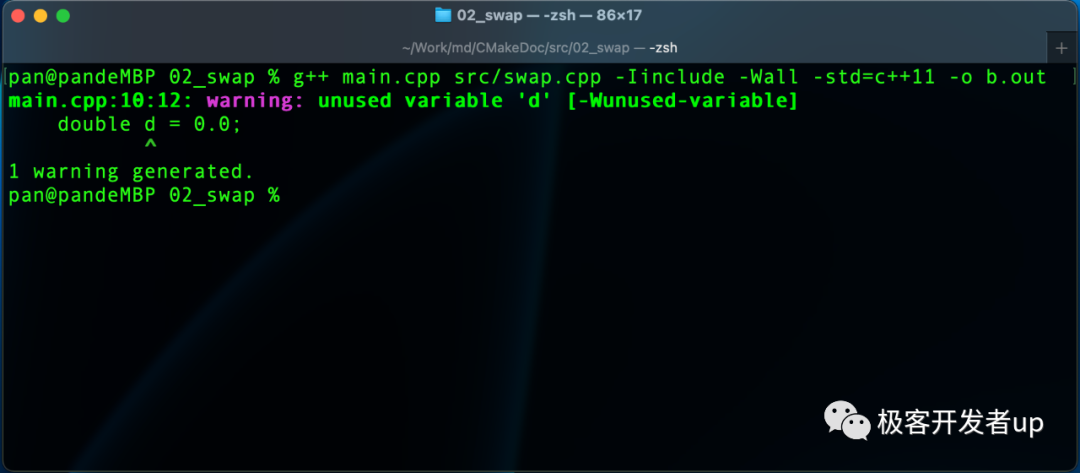
Because defining a variable is not used, it does not comply with the standard of c++11, but it is not an error, and b.out can execute normally.
2.1.3. Generate library file
Let's delete the code that will warn in 2 first
Link static libraries to generate executables
# Enter src directory first cd src # Assemble to generate swap O documentation g++ swap.cpp -c -I../include # Generate static library libswap o ar rs libswap.a swap.o # Go back to the code root directory and call the static link library to generate the executable file g++ main.cpp -lswap -Lsrc -Iinclude -o static_main
Link dynamic library to generate executable file
# Enter src directory cd src # Generate the dynamic link library file libswap so g++ swap.cpp -I../include -fPIC -shared -o libswap.so # The above instruction is equivalent to the following two instructions # gcc swap -I../include -c -fPIC # gcc -shared -o libswap.so swap.o # Go back to the parent directory cd .. # Link to generate executable file dyna_main g++ main.cpp -Iinclude -lswap -Lsrc -o dyna_main
The difference between static library and dynamic library in generating executable files
- When the static library is packaged to generate binary files, it directly includes the static library
- The dynamic library does not package the library file when it is packaged, but introduces the dynamic library file at run time
Therefore, when we run, the executable packaged by the static library file can run directly. The executable file compiled with the dynamic library will report an error when running directly, because the dynamic link file is in our code directory, not in the system library search directory. If we want to run the executable generated by dynamic link library packaging, we need to manually specify the library directory on which the program runs.
The command to run the executable file is as follows:
Run statically packaged executables
# Run statically packaged executables ./static_main
Run the executable generated by dynamic link library packaging
# To run dynamically packaged executable files, LD needs to be set_ LIBRARY_ Path variable. The variable value is the directory where the dynamic link file is located LD_LIBRARY_PATH=src ./dyna_main