Implement pwd and ls commands by yourself
In mybash version 1.0 and mybash version 2.0, the command operation in / usr/bin / directory is realized by executing the program fork and then replacing the sub process. For details, see Write your own version of bash1.0, Write your own version of bash2.0 , execvp will automatically find the command under the path. Let's check the path through echo
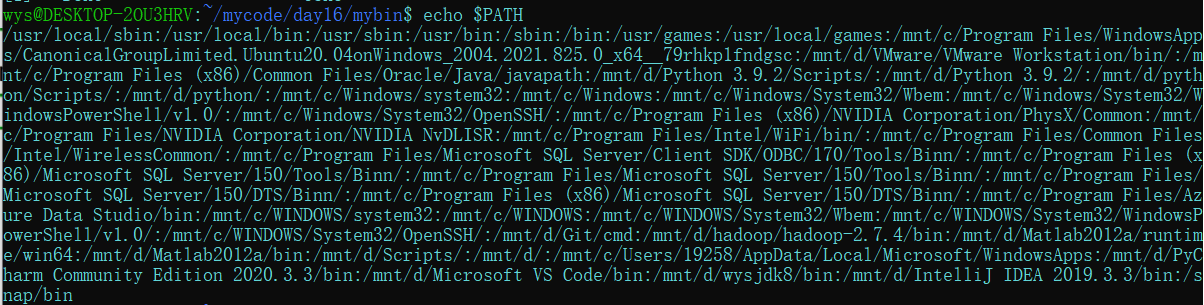
So the function is not implemented by ourselves. Now we try to write pwd and ls commands ourselves
1.pwd command
The program can determine its current working directory by calling the getcwd function
**#include <unistd.h>
char getcwd(char buff,size_t size);
The getcwd function writes the name of the current directory to the given buffer buff. If the length of the directory name exceeds the buffer length of the parameter size (an ERANGE error), for example, if your directory is very deep, it returns NULL. If it succeeds, it returns the pointer buf. (in) Write your own version of bash2.0 Mentioned).
1.1 write pwd.c
The code is as follows:
1 #include<stdio.h> 2 #include<unistd.h> 3 #include<string.h> 4 #include<stdlib.h> 5 6 int main() 7 { 8 char buff[128] = { 0 }; 9 if(getcwd(buff,128) != NULL ) 10 { 11 printf("%s\n",buff); 12 } 13 exit(0); 14 15 }
1.2 compilation and operation
The current directory has been printed out!
2.ls command
A common problem on Linux system is to scan the directory, that is, to determine the files stored in a specific directory.
Functions related to directory operations are declared in the dirent.h header FILE. They use a structure called dir as the basis for directory operations. The pointer to this structure (DIR *) called directory stream is used to complete various directory operations, which is very similar to the FILE stream (FILE *) used to operate ordinary files.
2.1 opendir function
The opendir function opens a directory and creates a directory stream. If successful, it returns a pointer to DIR, which is used to read directory data items.
#include <sys/types.h>
#include <dirent.h>
DIR opendir(const charname);
opendir returns a null pointer on failure.
2.2readdir
The readdir function returns a pointer. The structure pointed to by the pointer holds the relevant data of the next directory item in the directory stream dirp. Subsequent readdir calls will return subsequent directory entries. Readdir returns NULL if an error occurs or the end of the directory is reached.
**#include <sys/types.h>
#include <dirent.h>
struct dirent readdir(DIR dirp)
The contents of directory entries contained in the dirent structure include the following parts.
ino_t d_ino: the inode node number of the file.
char d_name []: the name of the file.
2.3 closedir function
The closedir function closes a directory stream and frees the resources associated with it. It returns 0 on successful execution and - 1 on error.
*#include <sys/types.h>
#include <dirent.h>
int closedir(DIR dirp);
2.4 writing ls.c
The code is as follows:
1 #include<stdio.h> 2 #include<unistd.h> 3 #include<string.h> 4 #include<stdlib.h> 5 #include<dirent.h> 6 7 int main() 8 { //Get current path 9 char buff[128] = { 0 }; 10 if(getcwd(buff,128) == NULL ) 11 { 12 return 0;//If you fail to get the current path, exit the program directly 13 } 14 15 DIR * p = opendir(buff);//Get directory stream 16 if( p == NULL ) 17 { 18 return 0; 19 } 20 21 struct dirent * s = NULL; 22 while ( (s = readdir ( p ))!= NULL ) 23 { 24 printf("%s ", s->d_name); 25 } 26 printf("\n"); 27 closedir(p); 28 exit(0); 29 }
2.5 compilation and operation
The result shows that there are one more. And one... Two files in the current folder. This is because the LS executable program we wrote ourselves also shows the hidden files in the current folder. However, the LS we usually use does not display hidden files. To display hidden files, we need to add a parameter - A, that is, ls -a. so we improve our ls. When executing LS executable files, we do not display hidden files.
2.6 improvement ls.c
1 #include<stdio.h> 2 #include<unistd.h> 3 #include<string.h> 4 #include<stdlib.h> 5 #include<dirent.h> 6 7 int main() 8 { //Get current path 9 char buff[128] = { 0 }; 10 if(getcwd(buff,128) == NULL ) 11 { 12 return 0;//If you fail to get the current path, exit the program directly 13 } 14 15 DIR * p = opendir(buff);//Get directory stream 16 if( p == NULL ) 17 { 18 return 0; 19 } 20 21 struct dirent * s = NULL; 22 while ( (s = readdir ( p ))!= NULL ) 23 { 24 if (strncmp(s->d_name,".",1) == 0 ) 25 { 26 continue;//When the first character of the file name is. Exit this cycle and do not print 27 } 28 printf("%s ", s->d_name); 29 } 30 printf("\n"); 31 closedir(p); 32 exit(0); 33 34 }
2.7 recompile and run
Hidden files are no longer displayed. ls command is implemented successfully!