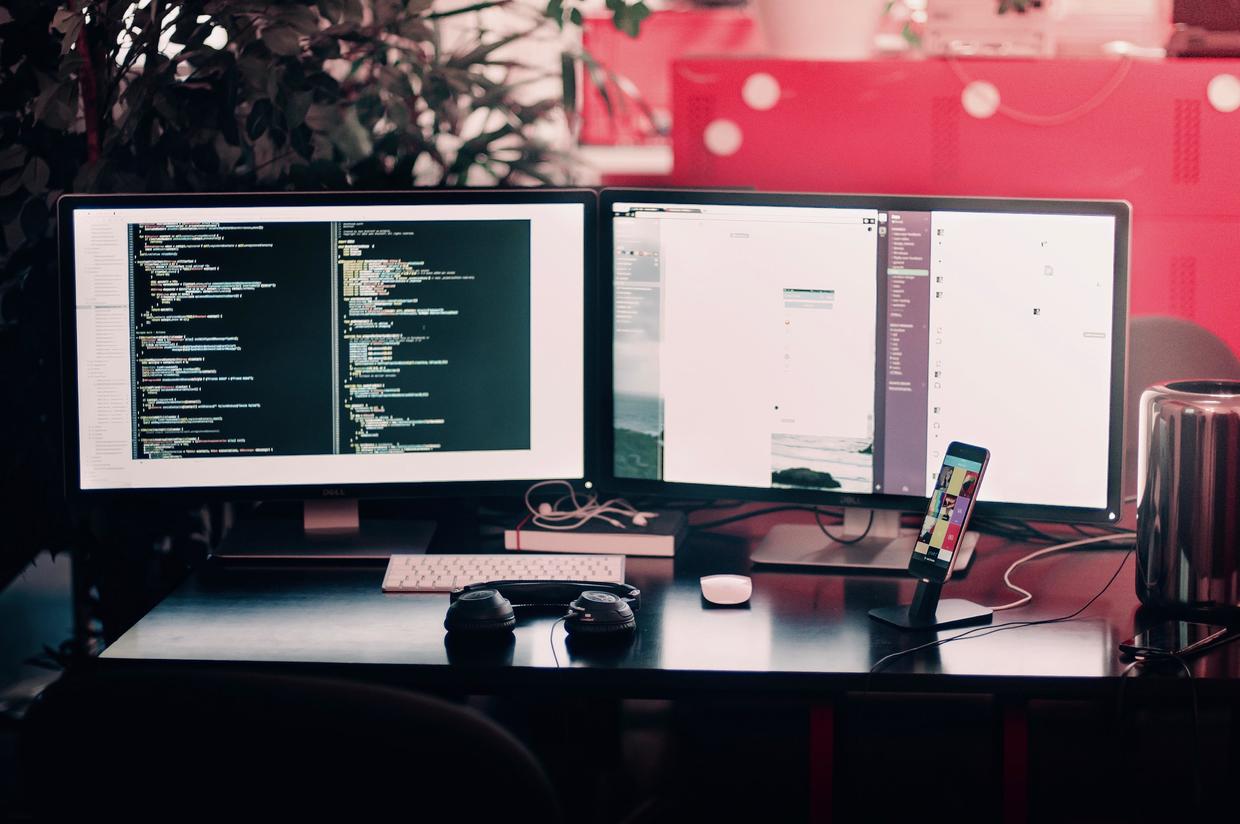
Technical dry goods: Linux Shell programming foundation, read this article is enough!
Copyright Statement: This article is an original article of cdeveloper, which can be reproduced at will, but the source must be indicated in a clear location!
Summary of this article
This paper mainly introduces the basic knowledge of Linux Shell programming, including the following eight aspects:
- Overview of Shell Programming
- Shell keyword
- Shell variable
- Shell operation
- Shell statement
- Shell function
- Shell debugging
- Shell is prone to errors
The following are introduced one by one.
Overview of Shell Programming
There is a scripting language under Linux called Shell script, which can help us simplify a lot of work, such as writing custom commands, so it is necessary to learn its basic usage. A simple hello.sh script, like the following, identifies which shell interprets the shell script in the first line!/bin/bash:
#!/bin/bash echo "Hello World!"
Only by granting authority can it be executed:
# Give executable authority chmod a+x hello.sh # implement ./hello.sh # Result Hello World!
Shell's writing process:
- Writing Shell scripts
- Give executable authority
- Execution, debugging
Let's introduce the specific grammar.
Shell keyword
The commonly used keywords are as follows:
- echo: Print text to screen
- exec: Execute another Shell script
- read: read standard input
- expr: Arithmetic operations on integer variables
- Test: Used to test whether variables are equal, empty, file type, etc.
- Exit: exit
Let's take an example:
#!/bin/bash echo "Hello Shell" # Read in variables read VAR echo "VAR is $VAR" # Computing variables expr $VAR - 5 # Test string test "Hello"="HelloWorld" # Test integer test $VAR -eq 10 # Test catalogue test -d ./Android # Execute other Shell scripts exec ./othershell.sh # Sign out exit
Before running, you need to create a new othershell.sh file to output I'm othershell, and need an input in the middle. Here I enter 10:
Hello Shell 10 VAR is 10 5 I'm othershell
Learning any language requires understanding its variable definition method, Shell is no exception.
Shell variable
Shell variables are divided into three types:
- User-defined variables
- Predefined variables
- environment variable
Defining variables requires attention to the following two points:
- No spaces around the equal sign: NUM=10
- General variable names are capitalized: M=1
Call variables with $VAR:
echo $VAR
1. User-defined variables
This variable only supports string type, does not support other characters, floating point and other types. There are three common prefixes:
- unset: Delete variables
- readonly: Marks read-only variables
- export: Specifies global variables
An example:
#!/bin/bash # Define common variables CITY=SHENZHEN # Define global variables export NAME=cdeveloper # Define read-only variables readonly AGE=21 # Print the value of a variable echo $CITY echo $NAME echo $AGE # Delete CITY variable unset CITY # No SHENZHEN output echo $CITY
Operation results:
SHENZHEN cdeveloper 21
2. Predefined variables
Predefined variables are often used to get input from the command line. There are the following:
- $0: script file name
- $1-9: Name of command line parameters 1-9
- $#: Number of command line parameters
- $@: All command line parameters
- $*: All command line parameters
- The exit status of the previous command, which can be used to obtain the return value of the function
- $: Process ID of execution
An example:
#!/bin/bash echo "print $" echo "\$0 = $0" echo "\$1 = $1" echo "\$2 = $2" echo "\$# = $#" echo "\$@ = $@" echo "\$* = $*" echo "\$$ = $$" echo "\$? = $?"
Results of execution. / hello.sh 1 2 3 4 5:
print $ # Program name $0 = ./hello.sh # First parameter $1 = 1 # Second parameter $2 = 2 # There are five parameters altogether. $# = 5 # Print out all parameters $@ = 1 2 3 4 5 # Print out all parameters $* = 1 2 3 4 5 # Process ID $$ = 9450 # No other commands or functions have been executed before. $? = 0
3. Environmental variables
Environmental variables exist by default. The following are commonly used:
- HOME: User Home Directory
- PATH: System environment variable PATH
- TERM: Current Terminal
- UID: Current user ID
- PWD: Current working directory, absolute path
Or look at examples:
#!/bin/bash echo "print env" echo $HOME echo $PATH echo $TERM echo $PWD echo $UID
Operation results:
print env # Current home directory /home/orange # PATH environment variable /home/orange/anaconda2/bin:There are many more behind. # Current terminal xterm-256color # current directory /home/orange # User ID 1000
This is where Shell variables are introduced. Here we introduce Shell's variable operations.
Shell operation
We often need to calculate in the Shell script. It is necessary to master the basic calculation methods. Here are four common calculation methods. The functions are m + 1:
- m=$[ m + 1 ]
- m=expr $m + 1 # wrapped in `characters
- let m=m+1
- m=$(( m + 1 ))
Let's look at a practical example:
#!/bin/bash m=1 m=$[ m + 1 ] echo $m m=`expr $m + 1` echo $m # Note: No spaces should be added around the + sign. let m=m+1 echo $m m=$(( m + 1 )) echo $m
Operation results:
2 3 4 5
Understanding the basic operation method, the next step to learn Shell's statement.
Shell statement
Shell statements are similar to high-level languages, including branches, jumps, loops. Here's a breakthrough for everyone.
1. if statement
This is similar to if - else - if in high-level languages, but in a different format. Let's take an example.
#!/bin/bash read VAR # Next, both of these methods can be used. Use [] Pay attention to the left and right spaces. #if test $VAR -eq 10 if [ $VART -eq 10 ] then echo "true" else echo "false" fi
2. case statement
The case statement is somewhat complicated, so we should pay attention to the format:
#!/bin/bash read NAME # The format is a bit complicated, we must pay attention to it. case $NAME in "Linux") echo "Linux" ;; "cdeveloper") echo "cdeveloper" ;; *) echo "other" ;; esac
Operation results:
# Enter Linux Linux Linux # Enter cdeveloper cdeveloper cdeveloper # Enter other strings hello other
3. for cycle
This is a basic example of for loops, quite simple, a bit like Python:
#!/bin/bash # Ordinary for cycle for ((i = 1; i <= 3; i++)) do echo $i done # VAR represents each element in turn for VAR in 1 2 3 do echo $VAR done
Operation results:
1 2 3
4. while loop
Note the difference between for loop and for loop:
#!/bin/bash VAR=1 # If VAR is less than 10, print it out. while [ $VAR -lt 10 ] do echo $VAR # VAR increases by 1 VAR=$[ $VAR + 1 ] done
Operation results:
1 2 3 4 5 6 7 8 9
5. until cycle
The difference between the until statement and the above loop is that its end condition is 1:
#!/bin/bash i=0 # When i is greater than 5, the loop ends until [[ "$i" -gt 5 ]] do echo $i i=$[ $i + 1 ] done
6. break
The break usage in Shell is the same as that in high-level languages. It's all about jumping out of the loop. Let's take an example:
#!/bin/bash for VAR in 1 2 3 do # How to jump out of the loop when VAR equals 2 if [ $VAR -eq 2 ] then break fi echo $VAR done
Operation results:
1
7. continue
continue is used to skip this cycle and move on to the next one. Let's look at the example above.
#!/bin/bash for VAR in 1 2 3 do # If VAR equals 2, skip and go straight to the next VAR = 3 loop if [ $VAR -eq 2 ] then continue fi echo $VAR done
Operation results:
1 3
Here's a look at some of the more important functions in Shell programming, as if functions in each programming language were important.
Shell function
Function can be explained in one sentence: with input and output of a certain function of the black box, I believe that students with programming experience will not be unfamiliar. So let's first look at the format of function definitions in Shell.
1. Defining Functions
There are two common formats:
function fun_name() { } fun_name() { }
For example:
#!/bin/bash function hello_world() { echo "hello world fun" echo $1 $2 return 1 } hello() { echo "hello fun" }
2. Call function
How to call the above two functions?
# 1. Call hello function directly with function name hello # 2. Use "Function Name Function Parameters" to Pass Parameters hello_world 1 2 # 3. Call indirectly using "FUN= `Function Name Function Parameter'" FUN=`hello_world 1 2` echo $FUN
3. Get the return value
How do I get the return value of the hello_world function? Do you remember $?
hello_world 1 2 # Can be used to get the return value of the previous function, where the result is 1 echo $?
4. Define local variables
Local is used to define local variables in functions:
fun() { local x=1 echo $x }
As the saying goes, program 3 points by writing, 7 points by tuning, let's see how to debug the Shell program.
Shell debugging
Use the following command to check for grammatical errors:
sh -n script_name.sh
Use the following commands to execute and debug the Shell script:
sh -x script_name.sh
For a practical example, let's debug the following test.sh program:
#!/bin/bash for VAR in 1 2 3 do if [ $VAR -eq 2 ] then continue fi echo $VAR done
First check for grammatical errors:
sh -n test.sh
No output, no error, start actual debugging:
sh -x test.sh
The debugging results are as follows:
+ [ 1 -eq 2 ] + echo 1 1 + [ 2 -eq 2 ] + continue + [ 3 -eq 2 ] + echo 3 3
The output of the Shell debugger is represented by + and the output of our program is not represented by +.
Shell is prone to errors
Here I summarize some mistakes that are easy to make in Shell programming for beginners. Most of them are grammatical errors.
- [] Can not nest (), can nest []
- $[val + 1] is a common way to add variables to 1
- [] It's best to add blanks to the contents of a test or calculation.
- Single quotation marks are similar to double quotation marks. Single quotation marks are more stringent. Double quotation marks can be nested with single quotation marks.
- Be sure to pay attention to the format of statements, such as indentation
summary
This blog mainly introduces the basic knowledge of Shell programming, a blog can not cover all aspects, you do not understand and hope that more Google, try to develop a good habit of self-study, thank you for reading, must practice oh:)