Litepal is an open-source Android database framework. It uses the object relational mapping (ORM) mode to encapsulate some database functions that we usually use.
Add dependent file
Object relational mapping (ORM): the programming language we use is the object-oriented language, and the database we use is the relational database.compile 'org.litepal.android:core:1.6.1'
1. Create a new assets directory under main, and a new literal.xml under assets
Here, we use the < mapping > tag to declare the mapping class model we want to configure. Be sure to use the full class name. No matter how many model classes need to be mapped, use the same method in the < list > tag.<?xml version="1.0" encoding="utf-8" ?> <litepal> <dbname values="BookStore" /> <version value="1" /> <list> <mapping class="com.example.app2.fileStorage.Book" /> </list> </litepal>
1. Create databasepublic class Book { private int id; private String author; private double price; private int page; private String name; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } public double getPrice() { return price; } public void setPrice(double price) { this.price = price; } public int getPage() { return page; } public void setPage(int page) { this.page = page; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
2. Upgrade databasepublic void onLitePalClick(View view) { Connector.getDatabase(); }
Add press column to Book
private String press;
New Category classpublic String getPress() { return press; } public void setPress(String press) { this.press = press; }
Modify literal.xmlpublic class Category { private int id; private String categoryName; private int categoryCode; public void setId(int id) { this.id = id; } public void setCategoryName(String categoryName) { this.categoryName = categoryName; } public void setCategoryCode(int categoryCode) { this.categoryCode = categoryCode; } }
3. Add data<?xml version="1.0" encoding="utf-8" ?> <litepal> <dbname values="BookStore" /> <version value="2" /> <list> <mapping class="com.example.app2.fileStorage.Book" /> <mapping class="com.example.app2.fileStorage.Category" /> </list> </litepal>
Let Book inherit the DataSupport class
public class Book extends DataSupport { }
Add the data through the book.save() method.public void onLitePalInsertClick(View view) { Book book = new Book(); book.setName("four"); book.setAuthor("dave"); book.setPage(300); book.setPrice(34.26); book.setPress("UnKnow"); book.save(); }
3. Update data
For literal, whether an object has been stored is determined according to the model.isSaved() method. A return of true indicates that it has been stored and a return of false indicates that it has not been stored.
perhapspublic void onLitePalUpdateClick(View view) { Book book = new Book(); book.setName("five"); book.setAuthor("cheery"); book.setPage(367); book.setPrice(85.36); book.setPress("UnKnow"); book.save(); book.setPrice(573); book.save(); }
public void onLitePalUpdateClick(View view) { Book book = new Book(); book.setPrice(85.36); book.setPress("UnKnow"); book.updateAll("name = ? and author = ?", "five", "cheery"); }
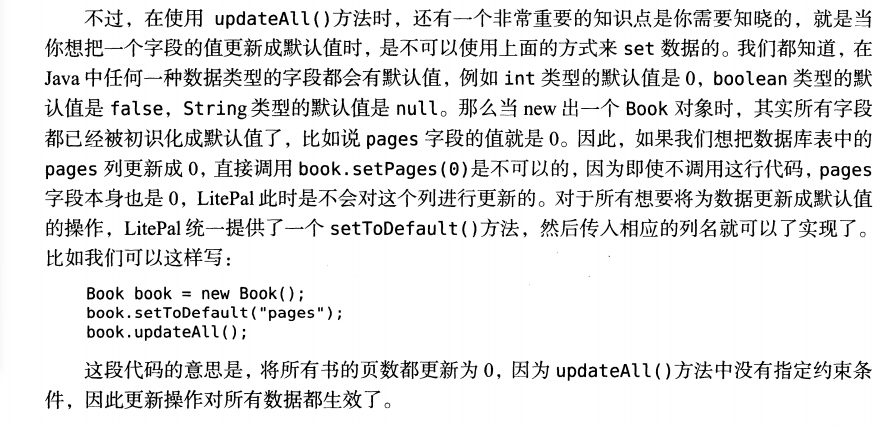
4. Delete data
5. Query datapublic void onLitePalDeleteClick(View view) { DataSupport.deleteAll(Book.class, "price < 55"); }
public void onLitePalQueryClick(View view) { List<Book> list = DataSupport.findAll(Book.class); for (Book book : list) { Log.d("TAG", "book name is" + book.getName()); Log.d("TAG", "book author is" + book.getAuthor()); Log.d("TAG", "book pages is" + book.getPage()); Log.d("TAG", "book price is" + book.getPrice()); Log.d("TAG", "book price is" + book.getPress()); } }
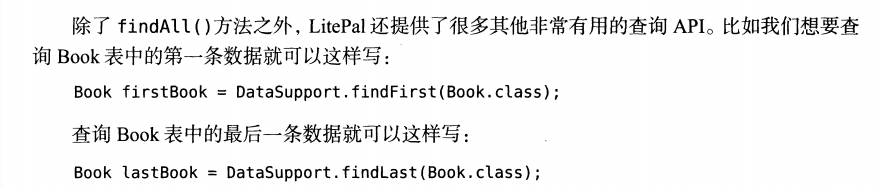
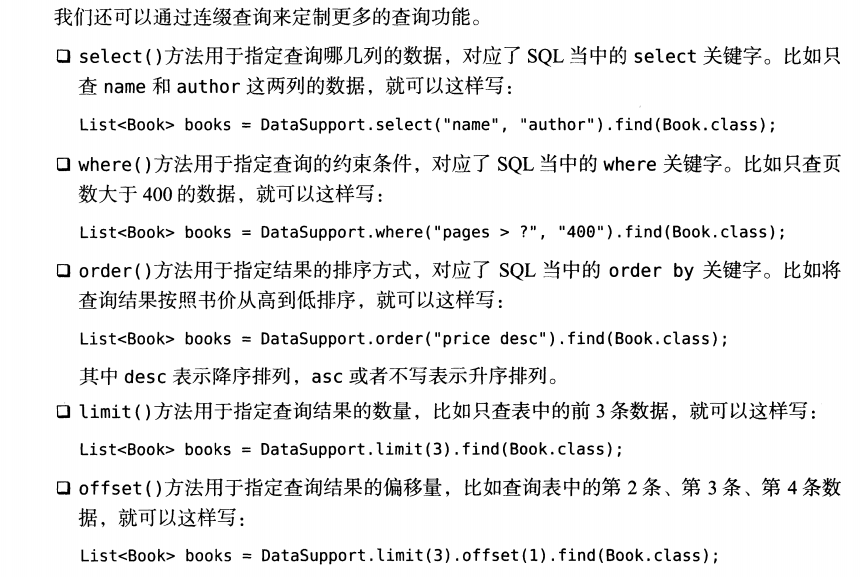