JavaScript tips
- 1, Will cause a variable to be false
- 2, String sorting in JS
- 3, js by deleting the specified elements in the array
- 4, Two ways to get object values
- 5, Extend type function to basic data type
- 6, Recursive solution of Hanoi Tower problem
- 7, Closure
- 8, Implementation delay function
- 9, The front end is compatible with the string type returned by the back end
Author: Gorit Date: 2021/8/25 Blog post published in 2021: 21 / 30
Reference:
- Daily work summary
- ***
1, Will cause a variable to be false
- false
- null
- undefined
- ’’Empty string
- Number 0
- Digital NAN
The rest is true
2, String sorting in JS
2.1 scenario restoration
Now there is a list that is sorted according to a field a, but now the requirements have changed and field b needs to be used to sort. After investigation, it is found that field a and field b are passed from the server in JSON. At first I didn't know
2.2 basic usage:
JavaScript API
localeCompare
We used StringObject.localeCompare(target) to sort strings
If the StringObject is smaller than the target, a number less than 0 will be returned, and vice versa
1.3 example:
let str = ['2','1','3'] str.sort((a,b) => { return a.localeCompare.b; }) obtain ['1','2','3']
3, js by deleting the specified elements in the array
This is generally used to delete elements in the background management system, which is implemented through ajax deletion
deleteLink (id) { let arr = this.page.list // Gets the resulting array arr.forEach((item, index, res) => { if (item.linkId == id) { res.splice(index,1) // Modify the returned new array, and res will be assigned to this.page.list } }) }
4, Two ways to get object values
let user = { name: "Gorit", age: 18 } user["name"] = Gorit user.age =18
5, Extend type function to basic data type
key word
- Prototype chain
- regular expression
The following tests are performed in the Node.js environment
- Based on the dynamic nature of JavaScript prototype chain inheritance, new methods will be given to all object instances
- Extend the function of types to add additional functions to the basic types of JavaScript. We only need to add methods through Object.prototype to apply to functions, arrays, strings, numbers, regular expressions and Boolean expressions
// A little trick. By adding a method method to Function.prototype, you don't have to enter prototype next time you add a method to an object Function.prototype.method = function(name, func) { // In order to prevent mixing with other public class libraries, it is added only when the conditions are met if (!this.prototype[name]) { this.prototype[name] = func; } return this; }
Use example:
/** * JavaScript Instead of providing a function to handle integers, we add an integer method to Number.prototype. Judge Math.ceiling or Math.floor according to the positive and negative numbers * Less than 0 is rounded up and greater than 0 is rounded down * Cannot use arrow function */ Number.method('integer',function() { return Math[this < 0 ? 'ceil' : 'floor'](this); }) console.log((-10 / 3).integer()); // -3 /** * JavaScript The lack of a method to remove whitespace from the beginning and end of a string is easy to make up for * Regular expression \ S refers to whitespace, including whitespace, line feed, tab indentation, etc. all whitespace \ S is the opposite * / ... / Regular expression wrap * \s+ Represents any number of * | Indicates that one is selected at random on both sides * ^ Match from scratch * $ Match to end * g Indicates adaptation to all possible situations */ // Algorithm problem: the implementation of string trim() method String.method('trim', function() { return this.replace(/^\s+|\s+$/g, ''); }) console.log(" dwdwd ".trim()); // Node environment usage // Document. Write ("DWD". Trim()) is used in HTML
6, Recursive solution of Hanoi Tower problem
Students who don't know the Hanoi Tower problem can go to 4455 Miniclip games to experience it. The implementation code is as follows:
// count indicates the number of plates let hanio = (count, src, aux, dst) => { if (count <= 0) return; hanio ( count - 1, src , dst , aux); console.log ('Move disc ' + count + ' from ' + src + ' to ' + dst); hanio( count - 1, aux, src , dst); }; hanio(2, 'A', 'B', 'C');
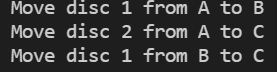
7, Closure
/** * closure * js Global variables can be read inside the function, but local variables inside the function cannot be read outside */ let n = 1000; function f1 () { console.log(n); } function f2 () { let y = 999; } f1() f2() // y undefined // How to read local variables from outside? Define another function inside the function // js, the parent element is visible to the subset, and vice versa function f3 () { let t = 1001; function f4 () { console.log(t); } // Taking f4 as the return value, you can read the internal variable outside f3 return f4; } let res = f3(); res(); // The above f4 function is a closure. Closures can connect inside and outside functions /** * effect: * 1. Read function internal variables * 2. The values of these variables are always stored in memory * * Disadvantages: large memory consumption */
8, Implementation delay function
function sleep (ms) { return new Promise(resolve => setTimeout(resolve,ms)); }
9, The front end is compatible with the string type returned by the back end
let res = await getXX("/xxx"); if (!res || res.length === 0) { // Abnormal data processing } else { // Normal data processing }