First look at the results to be achieved:
Link Address
This is the effect of my actual development of a project. Here I just extract the sliding control of the lock screen page. In fact, the other effects of the sliding control are easy. The sliding control is the key point.
Dead work
Android There are still a lot of knowledge points about the position parameters of View, and they are easy to confuse, so I think it is necessary to understand the basic knowledge before customizing View.
View has four attributes: top, left, right, bottom
top refers to the ordinates of the upper left corner.
Left refers to the abscissa of the upper left corner.
Right refers to the abscissa of the lower right corner.
bottom refers to the ordinates of the lower right corner.
There are also many coordinate methods for an event:
getX() refers to the leftmost distance between the event and the control itself.
getY() refers to the distance between the event and the top of the control itself.
getRawX() refers to the distance from the event to the left of the screen.
getRawY() refers to the distance between time and the top of the screen.
TouchSlop: Android judges whether a touch is a sliding event or a clicking event.
With the basic concepts clear, it's much easier to customize the view.
Do something
Firstly, the layout file is written. The layout file is simple and can be coded directly.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="60dp"> <ImageView android:id="@+id/image_lock_view_drag" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:src="@mipmap/home" /> <ImageView android:id="@+id/image_lock_view_left" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_centerInParent="true" android:layout_marginLeft="50dp" android:src="@mipmap/xiazai" /> <ImageView android:id="@+id/image_lock_view_right" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentRight="true" android:layout_centerInParent="true" android:layout_marginRight="50dp" android:src="@mipmap/yaoshi" /> <ImageView android:id="@+id/image_lock_view_zuo" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerVertical="true" android:layout_marginRight="20dp" android:layout_toLeftOf="@+id/image_lock_view_drag" android:src="@mipmap/zuo" android:visibility="gone" /> <ImageView android:id="@+id/image_lock_view_you" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerVertical="true" android:layout_marginLeft="20dp" android:layout_toRightOf="@+id/image_lock_view_drag" android:src="@mipmap/you" android:visibility="gone" /> </RelativeLayout>
Then the next step is to customize the View. Here I adopt the way of inheriting Relative Layout, to put it simply: the difficulty of this control is that the home key in the middle slides with your finger when you slide, and to determine whether it slides into the icon range on the left and right, and slides to the icon. Callback to Activity to handle the corresponding event.
In fact, it's a good solution to judge in a move event that if the distance between the event and the abscissa on the left side of the screen (getRawX() method) is less than half the width of the screen, that means that the user wants to slide left, and vice versa, to slide right.
When sliding to the left, getRawX() gets a distance less than the distance from the right of the left icon to the left of the screen, it shows that it slides to the left, records the tag, and calls back the interface if the tag holds up when the up event occurs. Vice versa.
ok, all the ideas are said, so just go to the code directly. The ideas are clear and the code will look much simpler later.
public class LockView extends RelativeLayout { private static final String TAG = "MainActivity"; private ImageView homeImageView; //home private ImageView zuoImageView; //The left arrow on the left of home private ImageView youImageView; //The right arrow on the right of home private ImageView leftImageView; //Image View to Make Money on the Left private ImageView rightImageView; //Image View unlocked on the right private int width; //Screen width private int mx, my; private int top; private int bottom; private int left; private int right; private int leftImageView_left; private int rightImageView_right; private boolean left_flag = false; private boolean right_flag = false; private int leftImageView_right; private int rightImageView_left; private Context mContext; private LockViewClickListener lockViewClickListener; //Coordinates pressed by home key private float homeDownX, homeDownY; //home key lifting coordinates private float homeUpX, homeUpY; public interface LockViewClickListener{ public void clickRight(); public void clickLeft(); public void clickHome(); } public void setOnLockViewClickListener(LockViewClickListener lockViewClickListener){ this.lockViewClickListener = lockViewClickListener; } public LockView(Context context) { super(context); mContext = context; width = MeasureUtil.getWidth(context); LayoutInflater.from(context).inflate(R.layout.layout_lock_view, this); initViews(); addListener(); } public LockView(Context context, AttributeSet attributeSet){ super(context, attributeSet); mContext = context; width = MeasureUtil.getWidth(context); LayoutInflater.from(context).inflate(R.layout.layout_lock_view, this); initViews(); addListener(); } private void addListener() { homeImageView.setOnTouchListener(new OnTouchListener() { private int height; public boolean onTouch(View v, MotionEvent event) { switch (event.getAction()) { case MotionEvent.ACTION_DOWN: homeDownX = event.getRawX(); homeDownY = event.getRawY(); homeImageView.setImageResource(R.mipmap.tongqian_pressed); leftImageView.setImageResource(R.mipmap.xiazaizhong); rightImageView.setImageResource(R.mipmap.yaoshizhong); // leftImageView_left:32 rightImageView_right:688 zuoImageView.setVisibility(View.VISIBLE); youImageView.setVisibility(View.VISIBLE); leftImageView_left = leftImageView.getLeft(); leftImageView_right = leftImageView.getRight(); rightImageView_right = rightImageView.getRight(); rightImageView_left = rightImageView.getLeft(); Log.i(TAG, "leftImageView_left:" + leftImageView_left + "rightImageView_right:" + rightImageView_right); Log.i(TAG, "event.getRawX()" + event.getRawX() + "event.getRawY()" + event.getRawY()); Log.i(TAG, "event.getX()" + event.getX() + "event.getY()" + event.getY()); height = (int) (event.getRawY() - 50); System.out.println("v.getTop()" + v.getTop() + "v.getBottom()" + v.getBottom() + "v.getLeft()" + v.getLeft() + "v.getRight" + v.getRight()); left = v.getLeft(); right = v.getRight(); top = v.getTop(); bottom = v.getBottom(); break; case MotionEvent.ACTION_MOVE: System.out.println("----------event.getRawX()" + event.getRawX() + "event.getRawY()" + event.getRawY()); System.out.println("----------event.getX()" + event.getX() + "event.getY()" + event.getY()); mx = (int) (event.getRawX()); my = (int) (event.getRawY() - 50); Log.i(TAG, " mx " + mx + " my" + my + " img.getWidth()/2" + homeImageView.getWidth() / 2 + " img.getHeight()/2" + zuoImageView.getHeight() / 2); if (mx < width / 2) { if (mx < leftImageView_right) { v.layout(leftImageView_left, top, leftImageView_right, bottom); left_flag = true; } else { v.layout(mx - homeImageView.getWidth() / 2, top, mx + homeImageView.getWidth() / 2, bottom); left_flag = false; } } else if (mx > width / 2) { if ((mx + homeImageView.getWidth() / 2) < rightImageView_right) { v.layout(mx - homeImageView.getWidth() / 2, top, mx + homeImageView.getWidth() / 2, bottom); Log.i(TAG, " int l " + (mx - homeImageView.getWidth() / 2) + " int top" + (my - homeImageView.getHeight() / 2) + " " + "int right" + (mx + zuoImageView.getWidth() / 2) + " int bottom" + (my + homeImageView.getHeight() / 2)); } if (mx > rightImageView_left) { v.layout(rightImageView_left, top, rightImageView_right, bottom); right_flag = true; } else { v.layout(mx - homeImageView.getWidth() / 2, top, mx + homeImageView.getWidth() / 2, bottom); right_flag = false; } } break; case MotionEvent.ACTION_UP: homeImageView.setImageResource(R.mipmap.home); homeUpX = event.getRawX(); homeUpY = event.getRawY(); zuoImageView.setVisibility(View.GONE); youImageView.setVisibility(View.GONE); if (Math.abs(homeUpX - homeDownX) > 3){ //Drag and drop leftImageView.setImageResource(R.mipmap.xiazai); rightImageView.setImageResource(R.mipmap.yaoshi); if (right_flag) { lockViewClickListener.clickRight(); rightImageView.setImageResource(R.mipmap.yaoshiok); } else if (left_flag) { lockViewClickListener.clickLeft(); leftImageView.setImageResource(R.mipmap.xiazaiok); } right_flag = false; left_flag = false; v.layout(left, top, right, bottom); }else { //click lockViewClickListener.clickHome(); } break; } return true; } }); } private void initViews() { leftImageView = (ImageView) findViewById(R.id.image_lock_view_left); rightImageView = (ImageView) findViewById(R.id.image_lock_view_right); homeImageView = (ImageView) findViewById(R.id.image_lock_view_drag); zuoImageView = (ImageView) findViewById(R.id.image_lock_view_zuo); youImageView = (ImageView) findViewById(R.id.image_lock_view_you); } }
Finally, go to github address: github
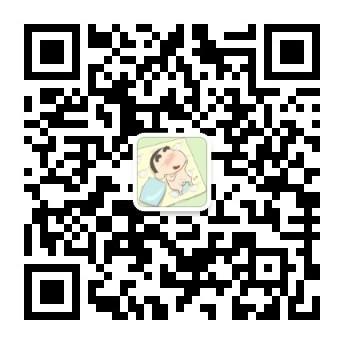