1, Preface
The use of Lombok is controversial. The author strongly recommends Lombok, and even believes that some common functions should be directly integrated in the form of JDK.
It is meaningless to write a bunch of Set/Get methods in the process of building entity classes; The toString method that inherits the Object class by default is officially recommended to be rewritten. Each entity class rewrites the toString method to express concrete entity information, which is cumbersome.
2, Common annotation analysis
(1) Entity class annotation
The annotations discussed below are added to entity classes.
public class XUser { private Integer userId; private String userName; }
1,@Data
After adding @ Data annotation, the compiled entity class will automatically add the following information: the Set/Get method of the attribute will automatically increase or decrease after change; The default constructor, in which the equals method, hashCode method and toString method are overridden by Lombok. The rewriting logic will be discussed in detail later.
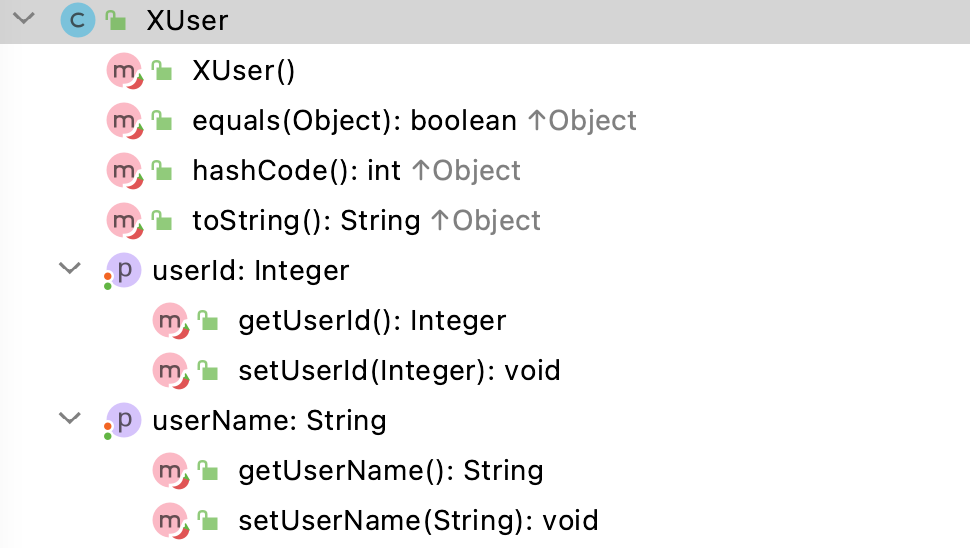
2,@AllArgsConstructor
After adding @ AllArgsConstructor annotation, the compiled entity will automatically add the following information: all parameter constructor. After adding @ AllArgsConstructor annotation, the annotation @ NoArgsConstructor without parameter constructor is generally required.

After adding @ AllArgsConstructor annotation, the entity class changes as follows after adding @ NoArgsConstructor:

3,@ToString
After adding @ toString annotation, the compiled entity will automatically add the following information: rewritten toString method.

@ToString annotation does not need to be added when @ Data annotation exists, unless its attribute configuration is required. The common attribute configuration is callSuper = true, which means that the attribute values of the parent class are added to the overridden toString method.
4,@Builder
After adding @ Builder annotation, the compiled entity will automatically add the following information: chained Set method.
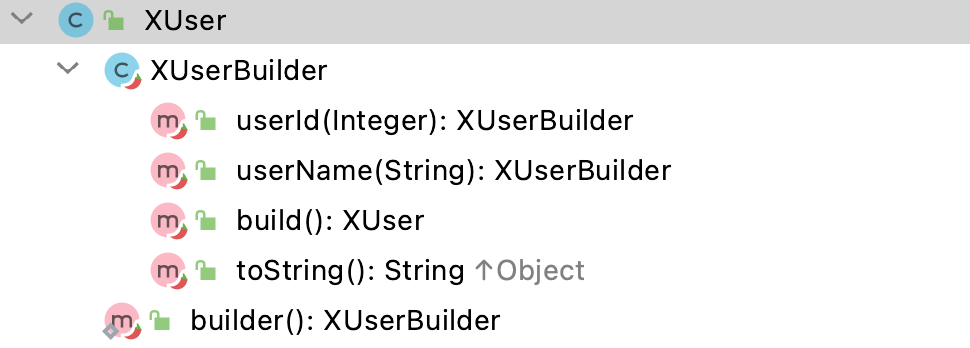
The advantages of the chain Set method are as follows:
// Instantiate the entity class in a concise way and complete the assignment operation XUser xUser = XUser.builder().userId(1).userName("AAAAA").build();
5,@Accessors
After adding the @ accessories (chain = true) annotation, the compiled entity class changes as follows: the setXxx method has a return value and is an example of the current object. There are three points to note when using this annotation:
- This annotation is experimental and may be deleted later;
- This annotation sets the attribute chain to true, which is one of the necessary conditions for the effect to take effect;
- Use with @ Data annotation or @ Setter annotation is a necessary condition for the effect to take effect.
@Setter @Accessors(chain = true) public class XUser { private Integer userId; private String userName; }
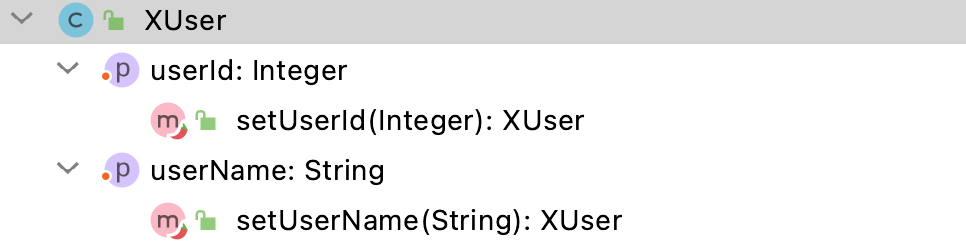
Introduction to main parameters
parameter | Default value | notes |
---|---|---|
fluent | false | Controls whether the generated getter and setter methods are preceded by get/set. If it is true, the chain will start automatically without setting again |
chain | chain | If it is set to true, the setter returns this object, which is convenient for chain calling methods |
The parameter fluent should be used with caution. For Jackson serialization framework, methods without get/set prefix have the probability of being unrecognized.
(2) Service class annotation
The above mentioned annotations are commonly used for entity classes. Here we mainly talk about service class annotations. There is no obvious distinction between the two. Both can be used in theory. This place only distinguishes them according to the occasion of use.
1,@Log4j2
After adding @Log4j2 annotations to the class, the current class instance automatically holds the log member variable, which can be used to add log information.
@Log4j2 public class XUserServiceImpl { public void saveUser(XUser user) { log.info("This method saves user information"); } }
Such information changes as follows:

3, Process inquiry
(1) Rewrite
Some annotations in Lombok will override the following methods: equals method, hashCode method, toString method
1. toString method
(1) Default implementation
Implementation of superclass Object inherited by default
public String toString() { return getClass().getName() + "@" + Integer.toHexString(hashCode()); }
Examples
XUser xUser = new XUser(); System.out.println(xUser.toString()); System.out.println(xUser.toString());
The output results of the same instance are consistent
xin.altitude.lombok.domain.XUser@43d7741f xin.altitude.lombok.domain.XUser@43d7741f
(2) Rewrite implementation
Examples
System.out.println(new XUser(1,"AAAAA").toString()); System.out.println(new XUser(1,"AAAAA").toString());~
The output values of the same member variables are the same. The output results are different only when the member variables are different, which has nothing to do with the execution times and the instance object reference.
XUser(userId=1, userName=AAAAA) XUser(userId=1, userName=AAAAA)
2. equals method
(1) Default implementation
Implementation of superclass Object inherited by default
public boolean equals(Object obj) { return (this == obj); }
Examples
XUser xUser1 = new XUser(1, "AAAAA"); XUser xUser2 = xUser1; // In the same JVM, the same reference object instance is the same (the output is true) boolean bl = xUser1.equals(xUser2);
(2) Rewrite implementation
Examples
// Whether the attribute values of the object are equal (the output is true) boolean bl = new XUser(1, "AAAAA").equals(new XUser(1, "AAAAA"));
The object constructed from the same member variable uses the rewritten equals method, and the result is true no matter how many times it is executed.