- Manually implement a vue cli
- 1. Thinking and preparation
- 2. The source code of our organization will be placed in the directory named src. An entry file is required for webpack packaging, which we take as main.js
- 3. First anticipate which of the following basic dependencies will be used. The first easy to think of is Vue, and then we assume that the project needs to use elementUI and axios
- 4. Our goal is to compile and package the in src from main.js to dist folder, so we need a configuration file for configuration. So create the configuration file of webpack, webpack.config.js
- 5. Write configuration:
- 6. Write the entry file main.js
- 7. Prepare the running project and create a shortcut script to call the non global webpack command
- 8. Start the project
- 9. Make some enhancements and introduce routing
- 10. Further enhance and introduce webpack development server
- 11. Optimize the configuration and automatically open the browser
- 12. More
Manually implement a vue cli
1. Thinking and preparation
First: to create a web project, you need an html file and a Vue project, so you need a div and an id
The file packaged by webpack needs to be introduced into bundle.js, which should be placed in dist (distribution) folder.
---->html file indicating div of id
---->Dist folder
The current structure is:
. └── dist └── index.html
The content of index.html is:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8" /> <title>VueStarter</title> </head> <body> <div id="app"></div> <script src="bundle.js"></script> </body> </html>
2. The source code of our organization will be placed in the directory named src. An entry file is required for webpack packaging, which we take as main.js
. ├── dist │ └── index.html └── src └── main.js
3. First anticipate which of the following basic dependencies will be used. The first easy to think of is Vue, and then we assume that the project needs to use elementUI and axios
npm i vue element-ui axios
Finally, the core file we need is the packaged bundle.js. The next core file we need is webpack, so we need to install webpack
It is also thought that the vue loader code that needs to be introduced into vue and the parsing of. vue files depends on vue template compiler
Style sass loader and sass Ontology
The processed css file needs to be loaded through css loader, and then parsed by style tag. Style loader is needed
babel is also required to handle the new syntax of es6, so it needs to be installed
Preset configuration of Babel Babel loader @ Babel / core Babel @ Babel / preset env (Env stands for compatibility with the latest syntax)
# -D refers to the development environment npm i -D webpack webpack-cli vue-loader vue-template-compiler sass-loader sass css-loader style-loader babel-loader @babel/core @babel/preset-env
4. Our goal is to compile and package the in src from main.js to dist folder, so we need a configuration file for configuration. So create the configuration file of webpack, webpack.config.js
It is found that the package.json file is not generated automatically. The command npm init generates it manually and specifies the entry file as main.js
. ├── README.md# Manually generated ├── dist ├── node_modules ├── package-lock.json ├── package.json ├── src └── webpack.config.js
5. Write configuration:
Familiar with basic structure:
module.exports = { entry:'',//Entry file output:{//Export documents, path:'', filename:'', }, module:{//Everything in webpack is a module rules:[ { test:/\.vue$/,use:'vue-loader'}, .... ] }, plugins:[//Plug ins are used to enhance webpack new PluginsA(),//Each plug-in is an instance new PluginsB(), ... ] }
Start filling content:
const path = require("path"); const { VueLoaderPlugin } = require("vue-loader"); module.exports = { entry: "./src/main.js", //Absolute / relative path of entry file output: { //Export documents, path: path.resolve(__dirname, "dist") + "", //Absolute path__ dirname is the "current path pwd" of node. Since the directory separator of window is' \ 'and the class nix is' /', in order to maintain flexibility, the built-in path object method of node is used to automatically splice the current system directory separator and directory name filename: "bundle.js", }, module: { //Everything in webpack is a module rules: [ { test: /\.vue$/, use: "vue-loader" }, /** * Handle the loading of. vue files. The writing method can be specified * Single dependency - String, * Single dependency with configuration - {} * Multiple dependencies without configuration - [] String, * Multiple dependent [] objects with configuration * { test: /\.vue$/, use: [{loader:'vue-loader',options:{}},{}...] }, */ { test: /\.s[ca]ss$/, use: ["style-loader", "css-loader", "scss-loader"], }, //Pay attention to the order and parse from back to front { test: /\.m?js$/, //. msj is a modular js file for es6 use: { loader: "babel-loader", options: { presets: ["@babel/preset-env"], }, }, }, { //Image processing // Test: / \ (png|jpe? G|gif|svg|webp) $/, use: {laoder: "file loader", options: {esmodule: false}}, / / old syntax test: /\.(png|jpe?g|gif|svg|webp)$/, type: "asset/resource", }, ], }, plugins: [ //Plug ins are used to enhance modules // The function of vue template compiler is to process js blocks and css blocks when parsing. vue files and reuse the rules defined above new VueLoaderPlugin(), ], }
6. Write the entry file main.js
import Vue from "vue"; import App from "./App.vue"; new Vue({ el: "#app", render: (h) => h(App), //Render the app dependency into a DOM node through the rendering function and mount it under the #app node });
<!--App.vue--> <template> <div>hello vue starter</div> </template> <script> export default {}; </script> <style lang="scss" scoped></style>
7. Prepare the running project and create a shortcut script to call the non global webpack command
#package.json "scripts": { "serve": "webpack --mode=development --watch", "build": "webpack --mode=production" },
The script specified in the package.json file will automatically execute node_ For the script under modules / bin, the mode option specifies the startup mode:
- production: generate environment, compress code, no error prompt
- Development: a development environment that does not compress code
watch: detect file changes and automatically recompile and package
8. Start the project
cmd execute command npm run serve
The bundle.js file is generated in the dist directory,
Open the index.html file in the dist directory. You will see the content written in App.vue:
This shows initial success.
9. Make some enhancements and introduce routing
Installation dependency
npm i vue-router # -S is the default parameter
Create / router/index.js and edit:
import VueRouter from "vue-router"; import Home from "../pages/Home.vue"; import Vue from "vue"; Vue.use(VueRouter); const router = new VueRouter({ routes: [ { path: "", component: Home, }, { path: "/me", component: () => import("../pages/About.vue"), }, ], }); export default router;
Introduced in main.js:
import Vue from "vue"; import App from "./App.vue"; import router from "./router"; new Vue({ el: "#app", router, render: (h) => h(App), //Render the app dependency into a DOM node through the rendering function and mount it under the #app node });
App.vue registration < router View > tag:
<template> <div> <span>hello vue starter</span> <div><button @click="$router.push('/')">HomePage</button></div> <div><button @click="$router.push('/me')">About Me</button></div> <router-view></router-view> </div> </template> <script> export default {}; </script> <style lang="scss" scoped></style>
Start program npm run serve
10. Further enhance and introduce webpack development server
```bash npm i -D webpack-dev-server ``` Modify startup script: pacakge.json ```json "serve": "webpack --mode=development --watch", ``` Amend to read: ```json "serve": "webpack serve --mode=development", ``` > webpack -dev-server Subcommands are provided serve And will automatically watch to configure webpack.config.js Start project: ```bash npm run serve ``` 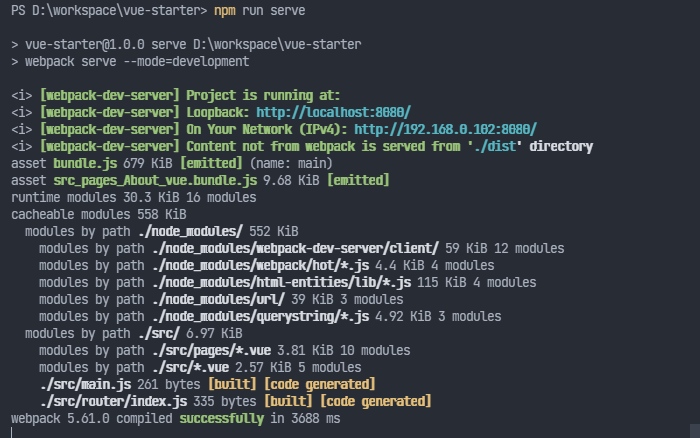 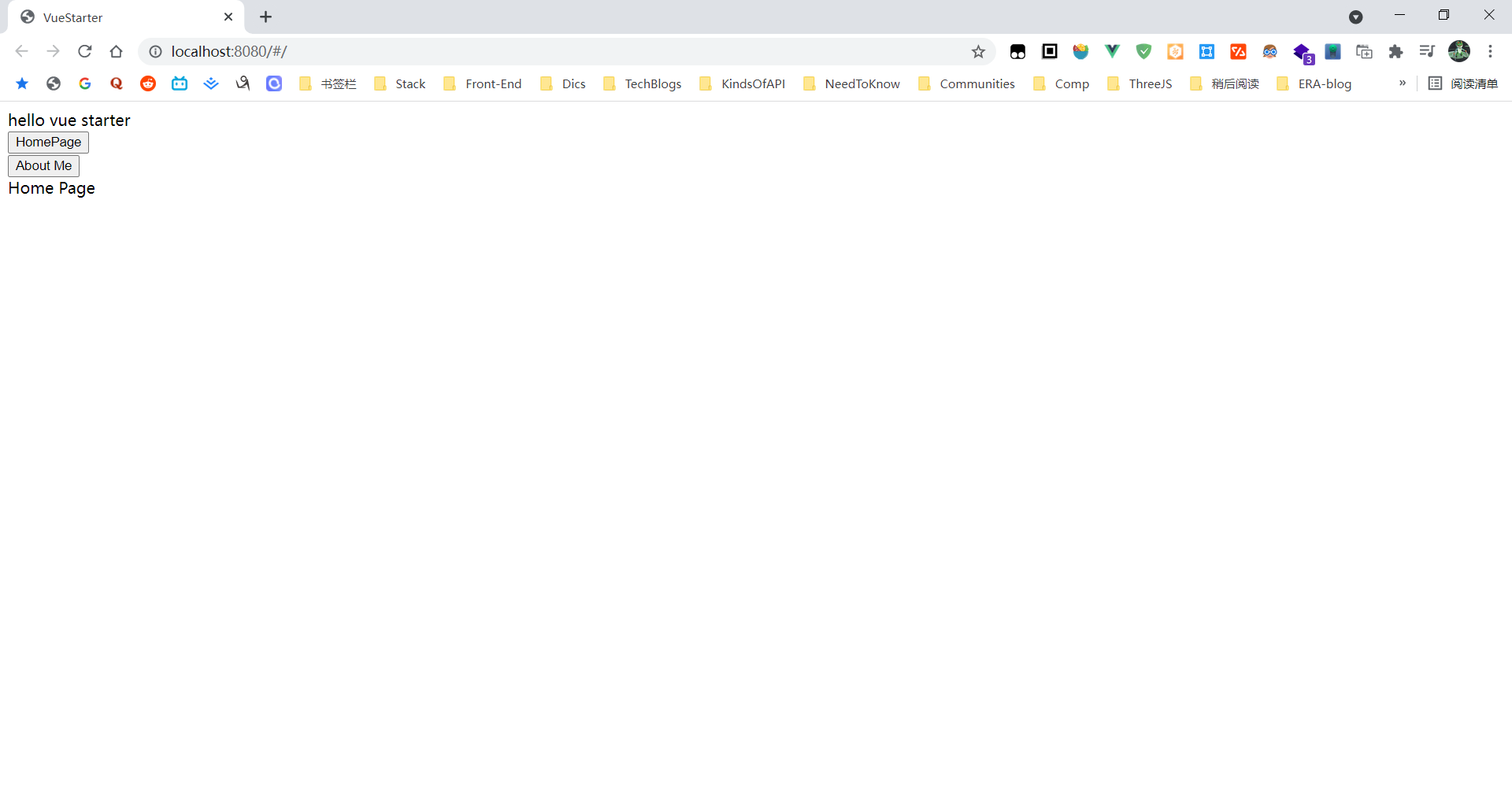
11. Optimize the configuration and automatically open the browser
webpack.config.js: ```json devServer: { static: "./dist", open:true }, ```
12. More
```json const path = require("path"); const { VueLoaderPlugin } = require("vue-loader"); module.exports = { entry: "./src/main.js", //Absolute / relative path of entry file output: { //Export documents, path: path.resolve(__dirname, "dist") + "", //Absolute path__ dirname is the "current path pwd" of node. Since the directory separator of window is' \ 'and the class nix is' /', in order to maintain flexibility, the built-in path object method of node is used to automatically splice the current system directory separator and directory name filename: "bundle.js", }, module: { //Everything in webpack is a module rules: [ { test: /\.vue$/, use: "vue-loader" }, /** * Handle the loading of. vue files. The writing method can be specified * Single dependency - String, * Single dependency with configuration - {} * Multiple dependencies without configuration - [] String, * Multiple dependent [] objects with configuration * { test: /\.vue$/, use: [{loader:'vue-loader',options:{}},{}...] }, */ { test: /\.s[ca]ss$/, use: ["style-loader", "css-loader", "scss-loader"], }, //Pay attention to the order and parse from back to front { test: /\.m?js$/, //. msj is a modular js file for es6 use: { loader: "babel-loader", options: { presets: ["@babel/preset-env"], }, }, }, { //Image processing // Test: / \ (png|jpe? G|gif|svg|webp) $/, use: {laoder: "file loader", options: {esmodule: false}}, / / old syntax test: /\.(png|jpe?g|gif|svg|webp)$/, type: "asset/resource", }, ], }, plugins: [ //Plug ins are used to enhance modules // The function of vue template compiler is to process js blocks and css blocks when parsing. vue files and reuse the rules defined above new VueLoaderPlugin(), ], devServer: { static: "./dist", open: true, host: "local-ip", //The boot address is the LAN ipv4 address port: 3333, //Manually specify the address, onListening(devServer) { //Command line custom output console.log( "Listening :http://", devServer.server.address().address, ":", devServer.server.address().port, ); }, }, }; ```