First of all, blog writing should be done at one go. Don't trust two or three days. The problems of the day should be solved and absorbed on the same day. Then be patient. You have to be patient in your study.
Refer to this link for some content in this article: c + + memory management (super long, detailed examples, good layout)_ Caogenwangboqiang's blog - CSDN blog_ c + + memory management
(1) : C + + memory includes kernel space, stack, memory mapping segment, heap, data segment and code segment.
1. First, the memory here is a virtual mapped space. The physical memory depends on the performance of the hardware device. Programs running on the operating system will also be affected by memory. The size of memory is related to the system on which the program is executed. In 32-bit programs, the vast majority of memory exists on the heap, and the stack is generally a few mb, so we often mention the error of stack overflow due to too deep recursion.
2. The functions of these five main areas: kernel space (related to the operating system, not understood temporarily), code segment: used to put executable code and read-only constants. This is generally a non modifiable part.
Stack and heap involve dynamic memory allocation. The stack grows downward. When the function is executed, the storage units of local variables in the function can be created on the stack, and these storage units are automatically released at the end of function execution. Stack memory allocation is built into the instruction set of the processor, which is very efficient, but the allocated memory capacity is limited.
The pile grows upward. Store the memory blocks allocated by new, and their release is controlled by our application. Generally, there is only one new and one delete. If the programmer does not release the program, the operating system will automatically recycle it after the program ends.
Data segment global variables and static variables are allocated to the same block of memory. In the previous C language, global variables are divided into initialized and uninitialized. There is no distinction in C + +. They share the same block of memory.
There are also some memory mapping segments, which are used to store dynamic and static libraries.
(2) Distinction
1. Heap and stack.
void f() { int *p=new int[5]; //Meaning: a pointer on the stack points to the memory of the heap, and the allocation will be determined first The size of memory on the heap, and then call operate new Apply for memory on the heap Then it returns the first address pointing to this memory
Essential difference: because stack memory is managed by compiler, heap memory is maintained by programmer. In contrast, the stack is more structured. It is reflected in that the stack is first in and last out, and the stack is pressed and destroyed according to the execution steps. Therefore, the orderly mandatory distribution and release ensure that the distribution of the stack is orderly. But there are also static memory allocation (compiled) and dynamic memory allocation. Dynamic memory allocation is allocated by malloc function. The allocation and release of the heap are discontinuous, resulting in many fragments in the heap.
2.delete and new:
(3) Memory management in C + +
For malloc -- and free in C language, we have improved new / / and delete in C + +.
A. Comparison between new ~ delete and malloc~free [first group of experiments]
B.new~delete and malloc~free failed to open memory [second group of experiments]
C. Locate new expression
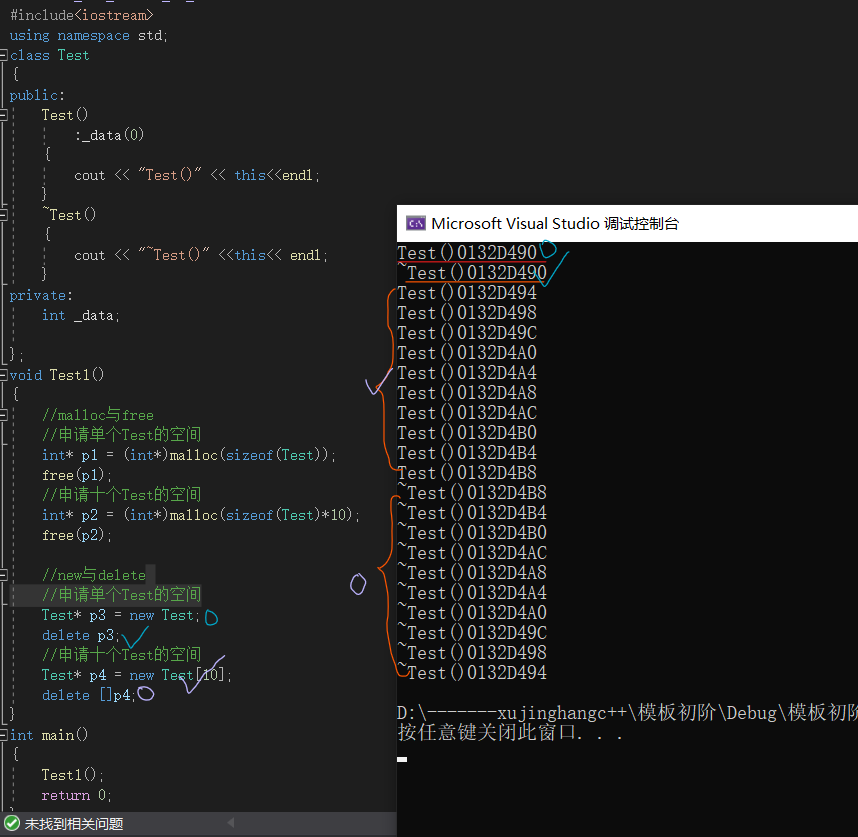
[A] From the analysis: 1 New and delete call the constructor and destructor respectively. malloc and free didn't. And it has nothing to do with whether it is a built-in type. 2. Inference principle. The function of the pointer. Why open up and free up space by deleting and instantiating pointers?
(1) Principle: if a pointer on the stack points to the memory of the heap, it will first determine the size of the memory allocated on the heap, then call operate new to apply for memory on the heap, and then return the first address pointing to this memory.
(2) new and delete call constructors and destructors
/* operator new: This function actually applies for space through malloc. When malloc successfully applies for space, it returns directly; Failed to apply for space. Try to implement the response measures for insufficient space. If the response measures are set by the user, continue to apply, otherwise throw an exception. */ void *__CRTDECL operator new(size_t size) _THROW1(_STD bad_alloc) { // try to allocate size bytes void *p; while ((p = malloc(size)) == 0) if (_callnewh(size) == 0) { // report no memory // If the memory application fails, bad will be thrown here_ Alloc type exception static const std::bad_alloc nomem; _RAISE(nomem); } return (p); } /* operator delete: This function finally frees up space through free */ void operator delete(void *pUserData) { _CrtMemBlockHeader * pHead; RTCCALLBACK(_RTC_Free_hook, (pUserData, 0)); if (pUserData == NULL) return; _mlock(_HEAP_LOCK); /* block other threads */ __TRY /* get a pointer to memory block header */ pHead = pHdr(pUserData); /* verify block type */ _ASSERTE(_BLOCK_TYPE_IS_VALID(pHead->nBlockUse)); _free_dbg( pUserData, pHead->nBlockUse ); __FINALLY _munlock(_HEAP_LOCK); /* release other threads */ __END_TRY_FINALLY return; } //Implementation of free #define free(p) _free_dbg(p, _NORMAL_BLOCK)
[B]operator new actually applies for space through malloc. If malloc successfully applies for space, it will return directly. Otherwise, it will take measures to deal with insufficient space provided by the user. If the user provides this measure, it will continue to apply, otherwise it will throw an exception. operator delete finally frees up space through free
Operator new and operator delete functions: new and delete are operators for dynamic memory application and release by users. Operator new and operator delete are global functions provided by the system. New calls operator new global function at the bottom to apply for space, and delete releases space through operator delete global function at the bottom.
#include<iostream> using namespace std; int main() { try { char* p = new char[0x8fffffff]; //char* p = new char[08ffffffff]; printf("%p\n", p); } catch (const exception& e) { cout << "Memory request failed" << endl; } return 0; }
For Non internal data type objects, maloc/free alone can not meet the requirements of dynamic objects. The constructor should be executed automatically when the object is created, and the destructor should be executed automatically before the object dies. Since malloc/free is a library function rather than an operator, it is not within the control permission of the compiler, and the task of executing constructors and destructors cannot be imposed on malloc/free. Therefore, C + + language needs an operator new that can complete dynamic memory allocation and initialization, and an operator delete that can clean and free memory.
class Test { public: Test() : _data(0) { cout<<"Test():"<<this<<endl; } ~Test() { cout<<"~Test():"<<this<<endl; } private: int _data; }; void Test() { // pt now points to only a space of the same size as the Test object, which can not be regarded as an object because the constructor does not execute that 's ok Test* pt = (Test*)malloc(sizeof(Test)); new(pt) Test; // Note: if the constructor of Test class has parameters, you need to pass parameters here }