★ micro service series
Microservices 1: microservices and its evolution history
Microservice 2: microservice panoramic architecture
Microservice 3: microservice splitting strategy
Microservice 4: service registration and discovery
Microservice 5: service registration and discovery (practice)
1 service registry
Previously, we introduced several common registration centers in the industry: Eureka, Zookeeper, Consul and Etcd.
It also makes a comparison on various indicators: registration method (watch\polling), health check, avalanche protection, security and permission, and the degree of support on Spring Cloud, Dubbo and Kubernets. It is convenient for us to make correct technical selection in different scenarios.
index | Eureka | Zookeeper | Consul | Etcd |
Consistency agreement | AP | CP (Paxos algorithm) | CP (Raft algorithm) | CP (Raft algorithm) |
health examination | TTL(Time To Live) | TCP Keep Alive | TTL\HTTP\TCP\Script | Lease TTL KeepAlive |
watch/long polling | I won't support it | watch | long polling | watch |
Avalanche protection | support | I won't support it | I won't support it | I won't support it |
Security and authority | I won't support it | ACL | ACL | RBAC |
Does it support multiple data centers | yes | no | yes | no |
Is there a management interface | yes | No (third party ZkTools available) | yes | no |
Spring Cloud integration | support | support | support | support |
Dubbo integration | I won't support it | support | support | I won't support it |
K8S integration | I won't support it | I won't support it | support | support |
We can see that the four technology types have high support for Spring Cloud. Spring Cloud is a one-stop solution for microservice architecture. What we need to do in the process of building microservices, such as configuration management, service discovery, load balancing, circuit breaker, intelligent routing, control bus, global lock, decision-making campaign, distributed session and cluster state management. Spring Cloud provides us with a set of simple programming model, which enables us to easily realize the construction of micro service projects based on Spring Boot.
Spring Cloud includes many different open source products to ensure one-stop micro service solutions, such as Spring Cloud Config, Spring Cloud Netflix, Spring Cloud Security, Spring Cloud Commons, Spring Cloud Zookeeper, Spring Cloud CLI and other projects.
2. Implementation under spring cloud framework
Spring Cloud makes a layer of abstraction for service governance, which can support a variety of different service governance frameworks, such as Netflix Eureka and consult. Let's take these two as examples to see how service governance is implemented.
Under the function of Spring Cloud service governance abstraction layer, the implementation of service governance can be switched seamlessly without affecting any other service registration, discovery and invocation logic.
Therefore, let's introduce the implementation of these two kinds of service governance to realize the benefits of Spring Cloud abstraction.
2.1 Spring Cloud Eureka
Spring Cloud Eureka is a service governance module under the Spring Cloud Netflix project. The Spring Cloud Netflix project is one of the subprojects of Spring Cloud. Its main content is the packaging of a series of open source products of Netflix company. It provides self configured Netflix OSS Integration for Spring Boot applications.
Through some simple annotations, developers can quickly configure common modules in the application and build a huge distributed system. Its main modules include: Eureka, Hystrix, Zuul, Ribbon, etc.
Next, let's take a look at how to use Spring Cloud Eureka to realize service governance.
2.1.1 creating a registry
Create a Spring Cloud project, which we name micro service center, and create in POM Introduce the required dependent content into XML:
1 <packaging>pom</packaging>
It indicates that there can be no Java code in this project, and no code is executed. It is just for the purpose of aggregating projects or passing dependencies, so you can delete the src folder. This is a parent project, because we also need to establish several sub projects such as Eureka's registry and client.
Under the micro service center, create a new Module named Eureka service, which is still a Spring Cloud project. After completion, POM XML is changed as follows:
1 <!-- Add parent project name to child project--> 2 <parent> 3 <groupId>com.microservice</groupId> 4 <artifactId>center</artifactId> 5 <version>1.0.0</version> 6 </parent> 7 8 9 <dependencies> 10 <!-- join eureka service --> 11 <dependency> 12 <groupId>org.springframework.cloud</groupId> 13 <artifactId>spring-cloud-netflix-eureka-server</artifactId> 14 </dependency> 15 </dependencies>
After modification, go back to the parent project micro service center and modify the information in pom:
1 <groupId>com.microservice</groupId> 2 <artifactId>center</artifactId> 3 <packaging>pom</packaging> 4 <version>1.0.0</version> 5 <name>center</name> 6 <description>Demo project for Spring Boot</description> 7 8 <!-- Add child project name to parent project--> 9 <modules> 10 <module>eureka-service</module> 11 <module>eureka-client</module> 12 </modules>
clean + install two projects should be successful.
Eureka service is used as a registry, so adding the @ EnableEurekaServer annotation to its main class Application can enable the registry function.
1 @SpringBootApplication 2 @EnableEurekaServer 3 public class ServiceApplication { 4 public static void main(String[] args) { 5 SpringApplication.run(ServiceApplication.class, args); 6 System.out.println("Start Eureka Service"); 7 } 8 }
However, by default, the registration center will also treat itself as a client, which will become a self registration center. This can be eliminated. Let's take a look at the detailed configuration in its YAML. The comments are clear:
1 server: 2 port: 1000 3 spring: 4 application: 5 name: eureka-server 6 eureka: 7 instance: 8 hostname: localhost 9 client: 10 register-with-eureka: false # Do not register as a client 11 fetch-registry: false # Do not get registration list 12 service-url: # Registered address. The client needs to register in this address 13 defaultZone: http://${eureka.instance.hostname}:${server.port}/eureka/
The notes in the article are relatively clear. As you can see here, the port number is 1000, so after the project is started, access http://localhost:1000/ You can see the page of Eureka registration center. No services have been found.
2.1.2 create client
At present, the service center is still empty, so we create a client that can provide services and register it in the registry.
Similarly, we create a Spring Cloud subproject named Eureka client, POM The configuration in XML is as follows:
1 <!-- Add parent project name to child project--> 2 <parent> 3 <groupId>com.microservice</groupId> 4 <artifactId>center</artifactId> 5 <version>1.0.0</version> 6 </parent> 7 8 9 <dependencies> 10 11 <!-- join eureka service --> 12 <dependency> 13 <groupId>org.springframework.cloud</groupId> 14 <artifactId>spring-cloud-netflix-eureka-server</artifactId> 15 </dependency> 16 17 <dependency> 18 <groupId>org.projectlombok</groupId> 19 <artifactId>lombok</artifactId> 20 </dependency> 21 22 </dependencies>
Add the @ EnableDiscoveryClient annotation in the Application main class Application file, which ensures that the current service is discovered by Eureka as a provider.
1 @SpringBootApplication 2 @EnableDiscoveryClient 3 public class ClientApplication { 4 public static void main(String[] args) { 5 SpringApplication.run(ClientApplication.class, args); 6 System.out.println("start client!"); 7 } 8 }
Add the following configuration to YAML file:
1 server: 2 port: 1001 3 spring: 4 application: 5 name: eureka-client 6 eureka: 7 client: 8 service-url: # This ensures that you can register with eureka-service Go to this registration center 9 defaultZone: http://localhost:1000/eureka/
spring. application. The name attribute specifies the name of the micro service, which can be used to access the service when calling. eureka. client. serviceUrl. The defaultzone property corresponds to the configuration content of the service registry and specifies the location of the service registry.
As you can see, the port here is set to 1001, which is because the service provider and service registry need to be tested on this machine, so the port attribute of the server needs to be set with different ports.
Finally, we write another interface to obtain all the service information of the registry in the client through the DiscoveryClient object.
1 @Controller 2 @RequestMapping("/eurekacenter") 3 public class EuServiceController { 4 5 @Autowired 6 DiscoveryClient discoveryClient; 7 8 /** 9 * Get registration service information 10 */ 11 @RequestMapping(value = "/service", method = {RequestMethod.GET}) 12 @ResponseBody 13 public String getServiceInfo() { 14 return "service:"+discoveryClient.getServices()+" , memo:"+discoveryClient.description(); 15 } 16 }
At this time, run for a try and continue to visit the previous address: http://localhost:1000/ , you can see that the Eureka registration center page already contains a service defined by us, which is the service of port 1001 newly created above.
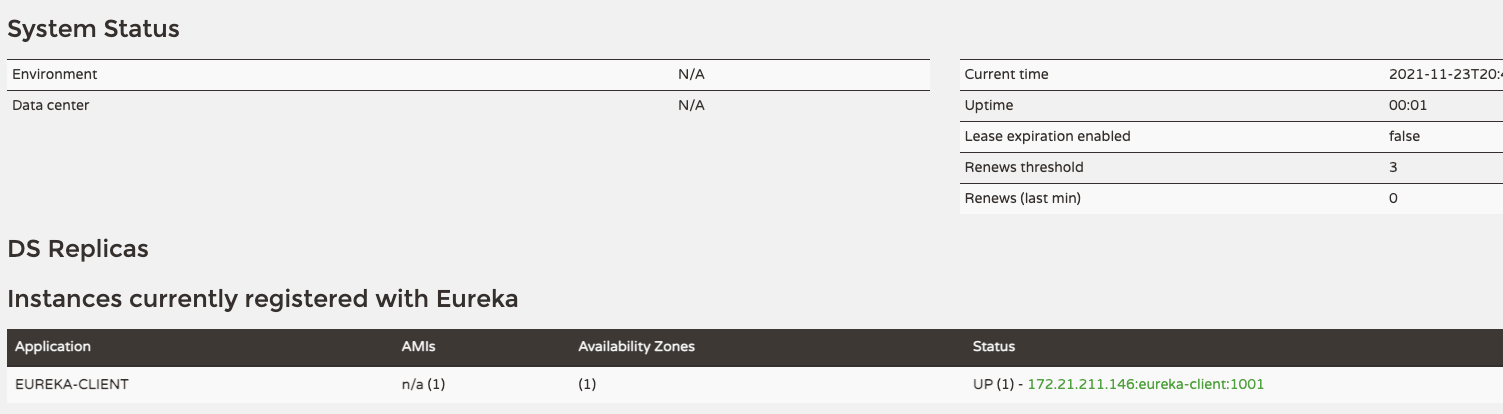
Similarly, we can call the above interface for obtaining registration service information to see how many services are registered in the registry from the perspective of service discovery. http://localhost:1001/eurekacenter/service
As shown in the figure above, the eureka client in square brackets obtains the List of all services in eureka's implementation through the getServiceInfo interface defined by Spring Cloud. It is a List of strings. If multiple providers are registered, they will all be displayed.
2.2 Spring Cloud Consul
Consul is used to realize service discovery and configuration of distributed systems. Compared with other distributed service registration and discovery schemes, consul's scheme has more "one-stop" characteristics. It has built-in service registration and discovery framework, distributed consistency protocol implementation, health check, Key/Value storage and multi data center scheme, and does not need to rely on other tools (such as ZooKeeper).
Spring cloud consult, as a whole, is a tool to provide service discovery and service configuration for our infrastructure in the micro service architecture.
2.2.1 advantages of consult
1. Using Raft algorithm to ensure consistency is more direct than complex Paxos algorithm.
2. It supports multiple data centers, and the services of internal and external networks are monitored by different ports. Multi data center cluster can avoid single point of failure of single data center, and its deployment needs to consider network delay, fragmentation and so on. zookeeper and etcd do not support multi data center functions, as shown in the table above.
3. Support health examination.
4. Support http and dns protocol interfaces. The integration of zookeeper is complex. etcd only supports http protocol.
5. The official web management interface is provided, but etcd does not have this function.
2.2.2 characteristics of consult
1. Service discovery
2. Health examination
3. Key/Value storage
4. Multi data center
2.2.3 installation of Consul registry
1. Official download version 64: https://www.consul.io/downloads.html
2. Unzip and copy to the directory / usr/local/bin
3. Start the terminal and see what version first
1 wengzhihua@B000000147796DS ~ % consul --version 2 Consul v1.10.4 3 Revision 7bbad6fe 4 Protocol 2 spoken by default, understands 2 to 3 (agent will automatically use protocol >2 when speaking to compatible agents)
4. Execute the installation command, and you can see that the port of his Client Addr is 8500. So visit the 8500 port site, http://127.0.0.1:8500/ui/dc1/services
1 wengzhihua@B000000147796DS ~ % consul agent -dev 2 ==> Starting Consul agent... 3 Version: '1.10.4' 4 Node ID: '6db154b4-62ff-e67d-e745-1a7270fa1ce8' 5 Node name: 'B000000147796DS' 6 Datacenter: 'dc1' (Segment: '<all>') 7 Server: true (Bootstrap: false) 8 Client Addr: [127.0.0.1] (HTTP: 8500, HTTPS: -1, gRPC: 8502, DNS: 8600) 9 Cluster Addr: 127.0.0.1 (LAN: 8301, WAN: 8302) 10 Encrypt: Gossip: false, TLS-Outgoing: false, TLS-Incoming: false, Auto-Encrypt-TLS: false
We can see that there is no client registered, only one instance of itself.
2.2.4 create a service provider
Due to the implementation of the spring cloud consult project, we can easily register the microservice application based on Spring Boot to consult, and realize the service governance in the microservice architecture through this.
We create a new cloud project consumer client under the micro service center. The pom file of the project is added as follows:
1 <!-- Add parent project name to child project--> 2 <parent> 3 <groupId>com.microservice</groupId> 4 <artifactId>center</artifactId> 5 <version>1.0.0</version> 6 </parent> 7 8 <dependencies> 9 <!-- Consul Service discovery--> 10 <dependency> 11 <groupId>org.springframework.cloud</groupId> 12 <artifactId>spring-cloud-starter-consul-discovery</artifactId> 13 </dependency> 14 <!-- Consul health examination--> 15 <dependency> 16 <groupId>org.springframework.boot</groupId> 17 <artifactId>spring-boot-starter-actuator</artifactId> 18 </dependency> 19 </dependencies>
Then modify the application For the configuration information of YML, write the consumption configuration, and the comments should be clear, as follows:
1 spring: 2 application: 3 name: consul-producer # The name of the current service 4 cloud: 5 consul: # The following is the address of the Consuk registry. If the host and port are not installed, they can be adjusted here 6 host: localhost 7 port: 8500 8 server: 9 port: 8501 # Port of the current service
Similarly, we need to add the @ EnableDiscoveryClient annotation in the Application main class Application file, which ensures that the current service is found by Consul as a provider.
You can see that this approach is the same as Eureka. Because Spring Cloud makes a layer of abstraction for service governance, it can shield the implementation details of Eureka and consult service governance,
There is no need to change the program. Just introduce different service governance dependencies and configure relevant configuration attributes. You can easily integrate microservices into each service governance framework of Spring Cloud.
1 @SpringBootApplication 2 @EnableDiscoveryClient 3 public class ConsulClientApplication { 4 public static void main(String[] args) { 5 SpringApplication.run(ClientApplication.class, args); 6 } 7 }
After the modification is completed, we can start the service Provider, and then go to the registration center to check the registration of the service, and you can see the registered Provider (consumer producer):
3 Summary
In addition to Eureka and Consul, there are other registry technologies, such as Zookeeper and Nocas. However, no matter what kind of registry technology, it is essentially to solve the following problems in microservices:
Decouple the details of interdependencies between services
We know that remote calls between services must know each other's IP and port information. We can directly configure the IP and port of the caller on the caller. The caller relies on IP and port directly. There are obvious problems. If the IP and port are changed, the calling method should be modified synchronously.
Through service discovery, the dependence of IP and port between services is transformed into the dependence of service name. The service name can be identified according to the micro service business. Therefore, shielding and decoupling the dependency details between services is the first problem to be solved by service discovery and registration.
Dynamic management of microservices
In the microservice architecture, there are many services, and the interdependence between services is also complex. Whether the services stop actively, hang up unexpectedly, or expand the service implementation due to the increase of traffic, the dynamic changes in these service data or status need to be notified to the callee as soon as possible, and the callee can take corresponding measures. Therefore, for service registration and discovery, it is necessary to manage the data and status of the service in real time, including the registration and online, active offline and elimination of abnormal services.