MongoDB learning notes
- 1, What is MongoDB?
- 2, What can MongoDB do?
- 3, Basic use of MongoDB
- 4, Java connection MangoDB
1, What is MongoDB?
1.1 introduction to mongodb
MongoDB is written in C + + language. It is an open source database system based on distributed file storage. It aims to provide scalable high-performance data storage solutions for WEB applications.
Baidu Encyclopedia introduction
As can be seen from the above description
- MangoDB is written in c + +, and its running efficiency is naturally not low
- Distributed file storage system
- Open source database system, anyone can use
1.2 characteristics of mongodb
Here are only the features that I think are more useful. There are many online features about MangoDB, so I won't list them one by one.
- MongoDB is a document storage oriented database, which is relatively simple and easy to operate.
- The file storage format is BSON (an extension of JSON), which supports the nesting of documents and arrays.
- Mongo supports rich query expressions. The query instruction uses JSON tags, which can easily query the embedded objects and arrays in the document.
- MongoDB supports various programming languages: RUBY, PYTHON, JAVA, C + +, PHP, c# and other languages
- wait
1.3 introduction to mongodb related terms
1.3.1 database
One MongoDB can establish multiple databases The default database of MongoDB is "db", which is stored in the data directory
1.3.2 Document
A document is a set of key value pairs (i.e. BSON). MongoDB documents do not need to set the same fields, and the same fields do not need the same data types, which is very different from relational databases, and it is also a very prominent feature of MongoDB.
Here is a simple document example
{"site": "https://www.gorit.cn", "name":"CodingGorit"}
Terms corresponding to RDBMS and MongoDB
RDBMS | MongoDB |
---|---|
database | database |
form | aggregate |
that 's ok | file |
column | field |
Table Union | embed document |
Primary key | Primary key (MangoDB provides a key of _id) |
1.3.3 assembly
A set is a MongoDB document group, similar to a table in RDBMS (Relational Database Management System).
The collection exists in the database and has no fixed structure, which means that you can insert data of different formats and types into the collection, but usually the data we insert into the collection will have a certain correlation.
For example, we can insert documents with the following different data structures into the collection
{"site": "https://www.gorit.cn"} {"site": "https://www.gorit.cn", "name":"CodingGorit"} {"site": "https://www.gorit.cn", "name":"CodingGorit","age":5}
When the first document is inserted, the collection is created
- Collection names cannot be empty strings
- The collection name cannot contain the \ 0 character (null character), which represents the end of the collection name.
- Collection names cannot start with 'system.' this is a reserved prefix for system collections.
- User created collection names cannot contain reserved characters. Some drivers do support including this character in collection names because some system generated collections contain this character. Do not appear $, unless you want to access such system created collections.
1.3.4 list
SQL terminology and concepts | MongoDB terminology and concepts | Explanation / explanation |
---|---|---|
database | database | database |
table | collection | Database tables / sets |
row | document | Data record line / document |
column | field | Data field / field |
index | index | Indexes |
primary key | primary key | Primary key MongoDB automatically sets the _id field as the primary key |
1.4 MongoDB data type
The following lists the common data types in MangoDB (from the rookie tutorial)
data type | describe |
---|---|
String | String, a common data class used to store data. Only UTF-8 encoded string in mangoDB is legal |
Integer | Integer value is used to store the value. It can be divided into 64 bits and 32 bits according to the server you adopt. |
Boolean | Boolean values, storing Boolean values (true and false) |
Double | Double precision floating-point value, used to store floating-point values |
Min,Max keys | Compare a value with the lowest and highest values of BSON (binary JSON) elements |
Array | Used to store multiple values of an array or list as a key |
TimeStamp | Timestamp, which records the specific time when a document is modified or added |
Object | For embedded documents |
Null | Used to create null values |
Date | Date and time: use UNIX time format to store the current date or time. You can specify your own date and time, create a date object, and pass in the year, month and day information |
. .
2, What can MongoDB do?
Let's see where online enterprises are and what mangoDB does
- Archive billions of records using MongoDB on Craiglist.
- FourSquare, a location-based social networking site, uses MongoDB to share data on Amazon EC2 servers.
- Shutterfly, an Internet-based social and personal publishing service, uses MongoDB's various persistent data storage requirements.
- bit.ly, a Web-based URL shortening service, uses MongoDB to store its own data.
- spike.com, an affiliate of MTV network, uses MongoDB's.
- Intuit, a software and service provider for small businesses and individuals, uses MongoDB to track user data for small businesses.
- sourceforge.net, the resource website to find, create and publish open source software for free, using MongoDB's back-end storage.
- etsy.com, a website for buying and selling handmade items, uses MongoDB. The New York Times, one of the leading online news portals, uses MongoDB.
- The data of CERN, the famous Institute of particle physics and the Large Hadron Collider of the European Center for nuclear research use MongoDB.
3, Basic use of MongoDB
3.1 downloading and configuring MongoDB
MongoDB thunderbolt network disk Extraction code: 2JOq
MongoDB Baidu cloud disk Extraction code: g9lu
I will omit the specific installation process. There are many tutorials on the Internet, which I won't show here
After downloading and installing, remember to configure environment variables
- D:\Program Files\mangoDB\bin
Remember to create a data folder in the root path, and our data files will be stored here
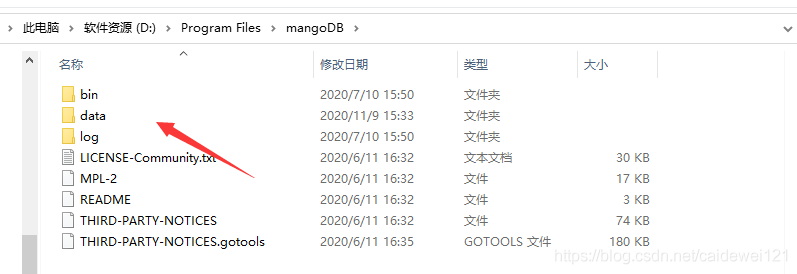
3.2 MongoDB common commands
3.2.1 database related operations
mangoDB command | Command function | Execution effect |
---|---|---|
show dbs | Displays a list of all data | xx01 |
db | Displays the current database or collection | xx02 |
use xxx (xxx is the database name) | You can connect to a specified database. If the database does not exist, it will be created | xxx03 |
db.dropDatabase() | To delete a database, you must use use use xxx to enter the database and then delete it | xxx04 |
3.2.2 collection operation
- Create collection
MongoDB command | Command function | Execution effect |
---|---|---|
db.createCollection(name, options) | Create collection | xxx01 |
show collections & show tables | View collection | xxx02 |
Create a fixed collection mycol. The size of the entire collection space is 6142800 KB, and the maximum number of documents is 10000.
db.createCollection("mycol", { capped : true, autoIndexId : true, size : 6142800, max : 10000 } ) { "ok" : 1 }
- Delete collection
- db.collection.drop()
- Collection is the name of the collection
3.2.3 document operation
1 insert document
show dbs; // view the database use test; // If the test database does not exist, test is created // Insert a record. If the set does not exist, first create the set and insert data db.user.insert({"id":"1","name":"Gorit","age":16}); // Insert multiple records at once db.user.insert({"id":"5","name":"Gorit555","age":16},{"id":"6","name":"Gorit6666","age":18}); // Insert multiple at once db.user.insertMany([{"id":"4","name":"Gori333","age":16},{"id":"4","name":"Gorit4444","age":18}])
Insert document and
- db.collection_name.insertOne()
- db.collection_name.insertMany()
2. Update documents
grammar
db.collection.update( <query>, <update>, { upsert: <boolean>, multi: <boolean>, writeConcern: <document> } )
Parameter Description:
- Query: query criteria of update, similar to the query criteria after where in sql update query.
- Update: update object and some Update Operators (such as
inc...) can also be understood as the data after the set in the sql update query
- upsert: optional. This parameter means whether to insert objnew if there is no update record. True means insert. The default is false and not insert.
- multi: optional. mongodb is false by default. Only the first record found will be updated. If this parameter is true, all the records found by conditions will be updated.
- writeConcern: optional, the level of exception thrown.
// Add test data db.user.insert({"id":"5","name":"Gorit ","age":16},); // Modify data db.user.update( {"name":"Gorit"}, {$set:{"name":"Zhang San","age":17}} ); // This will only modify the first condition record with the same name. If you want to batch modify records with the same name, you can do so db.user.update( {"name":"Gorit"}, {$set:{"name":"Zhang San","age":17}}, {multi:true} ); // More condition modifications Update only the first record: db.col.update( { "count" : { $gt : 1 } } , { $set : { "test2" : "OK"} } ); Update all: db.col.update( { "count" : { $gt : 3 } } , { $set : { "test2" : "OK"} },false,true ); Add only the first: db.col.update( { "count" : { $gt : 4 } } , { $set : { "test5" : "OK"} },true,false ); Add all: db.col.update( { "count" : { $gt : 5 } } , { $set : { "test5" : "OK"} },true,true ); Update all: db.col.update( { "count" : { $gt : 15 } } , { $inc : { "count" : 1} },false,true ); Update only the first record: db.col.update( { "count" : { $gt : 10 } } , { $inc : { "count" : 1} },false,false );
3. Delete document
db.collection.remove( <query>, <justOne> ) // mangoDB version is after version 2.6 db.collection.remove( <query>, { justOne: <boolean>, writeConcern: <document> } )
use
// Delete all data db.collection_ name.remove({}) // Deletes the record for the specified field db.collection_name.remove({"id":"1"}) // Delete the first entry of the specified record db.collection_name.remove({"id":"1"},1) // The new version deletes all collections db.collection_name.deleteMany({}) // Delete multiple with status A in the new version db.collection_name.deleteMany({ status : "A" }) // The new version deletes a collection with status D db.inventory.deleteOne( { status: "D" } )
4. Query documents
4.1 query criteria
Corresponding to where condition query
operation | format | Example | Corresponding statements in RDBMS |
---|---|---|---|
be equal to | { key:value } | db.user.find({"name":"Gorit111"}) | where age = 17 |
less than | { key : { $lt : value } } | db.user.find({"age":{$lt:20}}); | where age < 20 |
Less than or equal to | { key : { $lte : value } | db.user.find({"age":{$lte:20}}); | where age <= 20 |
greater than | { key : { $gt : value } | db.user.find({"age":{$gt:16}}); | where age > 16 |
Greater than or equal to | { key : { $gte : value } | db.user.find({"age":{$gte:16}}); | where age >=16 |
Not equal to | { key : { $ne : value } | db.user.find({"age":{$ne: 16}}) | where age <> 16 |
//View all document records db.collection_name.find() // Query a document db.collection_name.findOne(); // Condition query // Condition query== db.user.find({"name":"Gorit111"}) // where name = "Zhang San" all match // Condition query is less than db.user.find({"age":{$lt:17}}); // where age < 17 // Condition query is less than or equal to db.user.find({"age":{$lte:20}}); // where age <= 20 // Condition query greater than db.user.find({"age":{$gt:16}}); // where age > 10 // Condition query is greater than or equal to db.user.find({"age":{$gte:16}}); // where age >= 16 // Condition query is not equal to db.user.find({"age":{$ne: 16}})
4.2 logical query
// Logic and query db.user.find({"age":17},{"id":"1"}) // Logical or query db.user.find( { $or: [ {"age":17}, {"id":"1"} ] } ) // Combined query exercise where age <=20 or (name in ("Zhang San","Gorit111") db.user.find( { "age": {$lte: 20}, $or: [ { "name": "Zhang San" },{ "name": "Gorit111" }] } )
4.3 paging query
- Paging parameter Limit()
db.collection_name.find().limit(number); // Query three records in the database. If the database does not specify limit(), query all data by default db.user.find().limit(3);
- Use skip() to skip a specified amount of data
// Skip the first record and query the next three records db.user.find().limit(3).skip(1);
4.4 sorting
In MangoDB, the sort() method is used to sort the data. The sort() method can specify the sorting fields through parameters, and use 1 and - 1 to determine the sorting method. 1 represents ascending sequence and - 1 represents descending sequence
// Ascending by age db.user.find().sort({"age":1}) // In descending order of age db.user.find().sort({"age":-1});
4.5 polymerization
Aggregation in MangoDB is mainly used to process data (such as average, sum, etc.) and return the calculated results
However, it seems that my version does not support aggregate query, so I won't hide it here.
3.3 connect MangoDB with database connection tool
There are many tools that can be connected. I use the database connection software provided by Jetbrains: DataGrid
Of course, you can also use the one provided by idea
Or use other tools
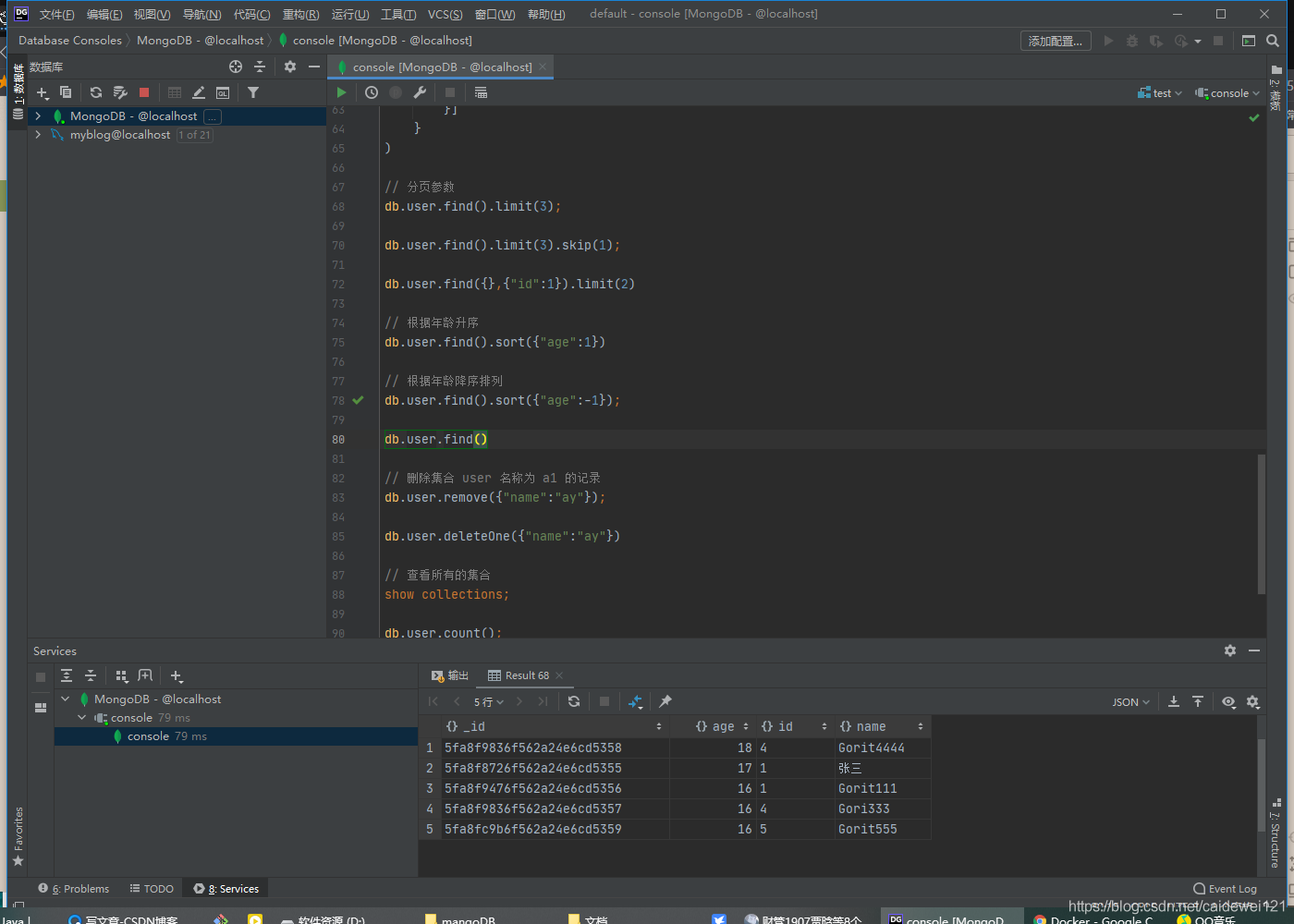
4, Java connection MangoDB
As a java developer, how can we connect mangoDB without Java?
Next, enter the actual combat part
4.1 SpringBoot integration MongoDB
SpringBoot integration MongoDB integration
- Dependency import
<dependency> <groupId>org.springframework.bootgroupId> <artifactId>spring-boot-starter-data-mongodbartifactId> dependency>
- Configuration file writing
For the convenience of testing, I'm here
# Application service WEB access port server: port: 8080 # apply name spring: application: name: demo resources: # spring static resource scanning path static-locations: classpath:/static/ data: mongodb: database: test # Database to connect to host: localhost # Database address port: 27017 # port #authentication-database: admin # Verify login information base #username: # Account connected to the database #password: # Password to connect to the library
- actual combat
Writing entity classes
package cn.gorit.entity; /** * @Classname Book * @Description TODO * @Date 2020/11/9 21:48 * @Created by CodingGorit * @Version 1.0 */ public class Book { private Integer id; private String name; private String author; // getter setter constructor omitted }
Write BookDao
package cn.gorit.dao; import cn.gorit.entity.Book; import org.springframework.data.mongodb.repository.MongoRepository; import java.util.List; /** * @Classname BookDao * @Description TODO * @Date 2020/11/9 21:48 * @Created by CodingGorit * @Version 1.0 */ public interface BookDao extends MongoRepository<Book,Integer> { List<Book> findAllByAuthor(String author); Book findByNameEquals(String name); }
Write BookController
package cn.gorit.controller; import cn.gorit.dao.BookDao; import cn.gorit.entity.Book; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.mongodb.core.MongoTemplate; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; import java.util.ArrayList; import java.util.List; /** * @Classname BookController * @Description TODO * @Date 2020/11/9 21:51 * @Created by CodingGorit * @Version 1.0 */ @RestController public class BookController { // baseMongo provided by practical internal teeth @Autowired BookDao dao; // Practical built-in template @Autowired MongoTemplate template; @GetMapping("/test1") public void test1() { List<Book> books = new ArrayList<>(); books.add(new Book(1,"years","ls")); books.add(new Book(2,"Lou Ji","ls")); dao.insert(books); List<Book> b1 = dao.findAllByAuthor("ls"); System.out.println(b1.toString()); System.out.println(dao.findByNameEquals("Lou Ji")); } @GetMapping("/test2") public void test2() { List<Book> books = new ArrayList<>(); books.add(new Book(3,"A Town Besieged","Qian Zhongshu")); books.add(new Book(4,"Selected notes of Song Poetry","Qian Zhongshu")); template.insertAll(books); List<Book> list = template.findAll(Book.class); System.out.println(list); Book book = template.findById(3,Book.class); System.out.println(book); } }
Interface test
