1. Define profile information
Sometimes we put some variables into the yml configuration file for unified management
for example
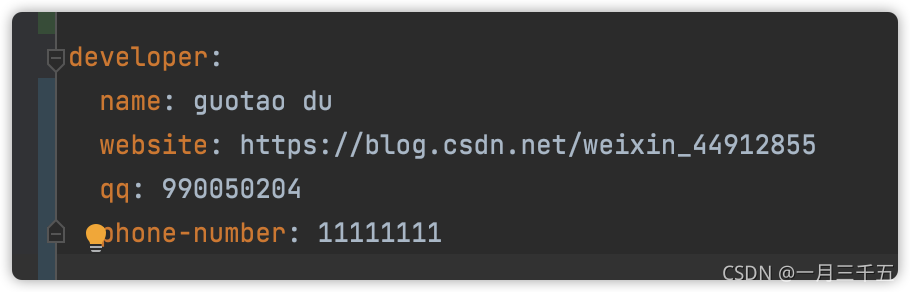
Replace @ Value with @ ConfigurationProperties
usage method
Define the entity of the corresponding field
@Data // Specify prefix @ConfigurationProperties(prefix = "developer") @Component public class DeveloperProperty { private String name; private String website; private String qq; private String phoneNumber; }
Inject this bean when using
@RestController @RequiredArgsConstructor public class PropertyController { final DeveloperProperty developerProperty; @GetMapping("/property") public Object index() { return developerProperty.getName(); } }
2. Replace @ Autowired with @ RequiredArgsConstructor
We all know that there are three ways to inject a bean (set injection, constructor injection and annotation injection). spring recommends that we use the constructor to inject beans
Let's take a look at what the previous code looks like after compiling
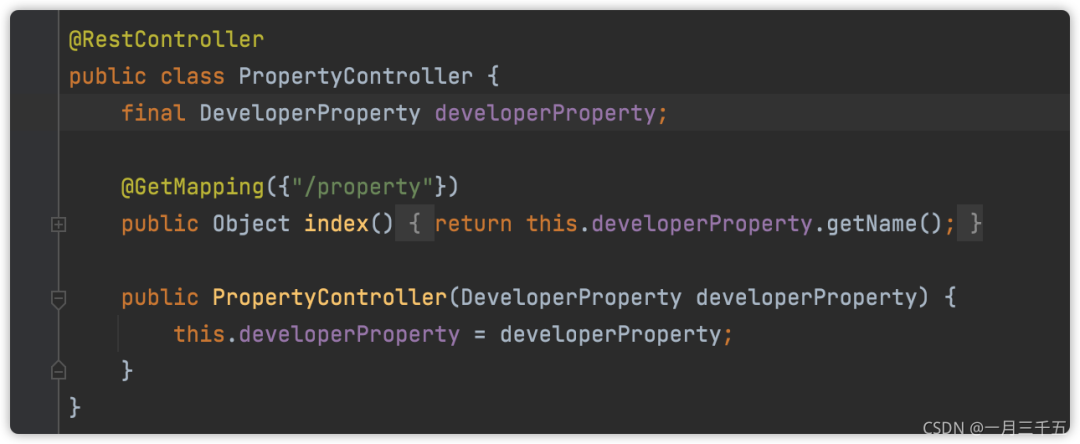
RequiredArgsConstructor: provided by lombok
3. Code modularization
Alibaba Java development manual says that the code of each method should not exceed 50 lines (if I remember correctly)
In the actual development, we should be good at splitting our own interfaces or methods, so that a method only deals with one logic. Maybe a function will be used in the future
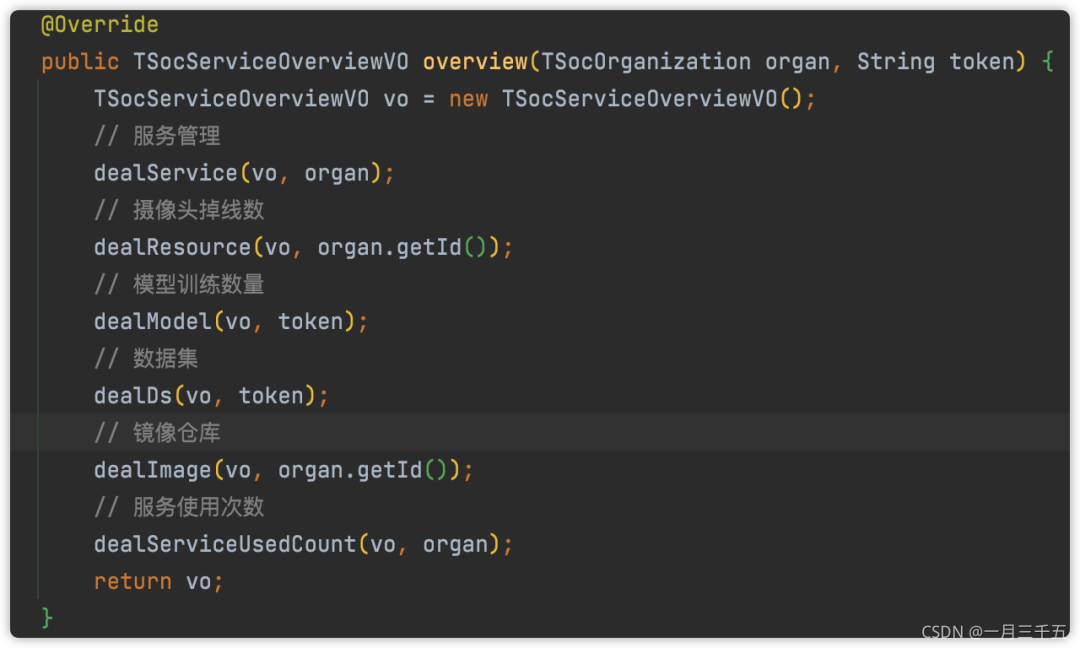
4. Throw exception instead of return
When writing business code, you often return different information according to different results. Try to minimize the return, which will make the code more chaotic
Counterexample
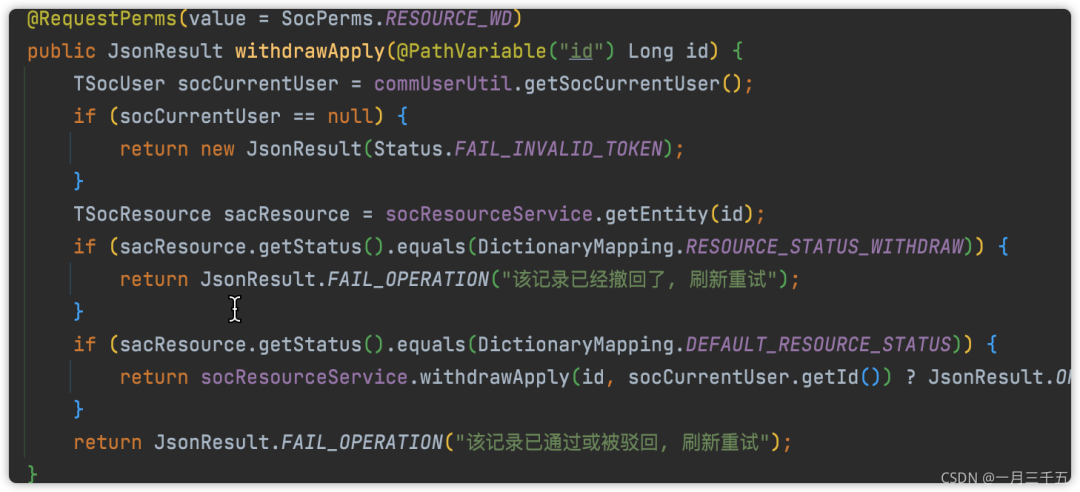
Positive example
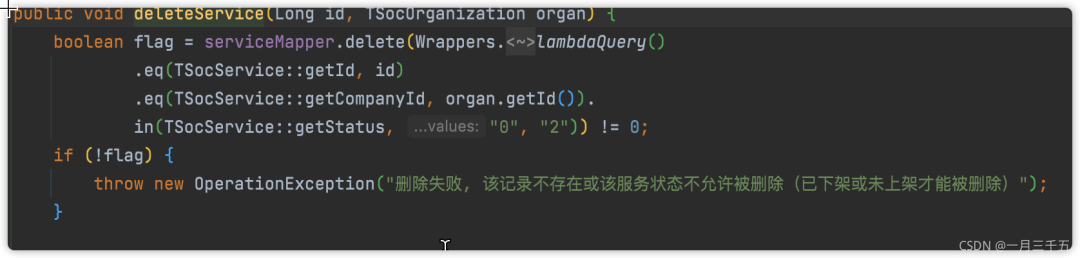
5. Reduce unnecessary costs
Reduce queries to the database as much as possible
For example
Delete a service (it can only be deleted if it is off the shelf or not on the shelf)
Before I read the code written by others, I will first query the record according to the id, and then make some judgments
Counterexample
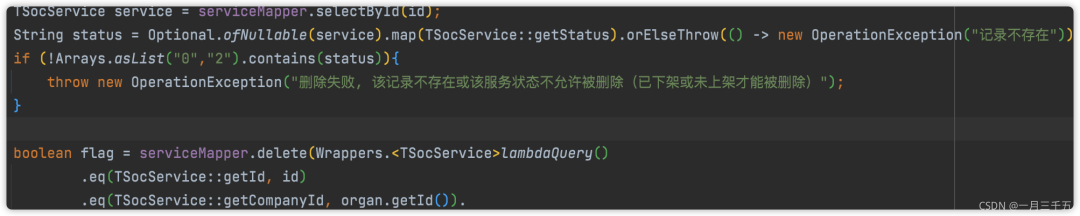
Positive example
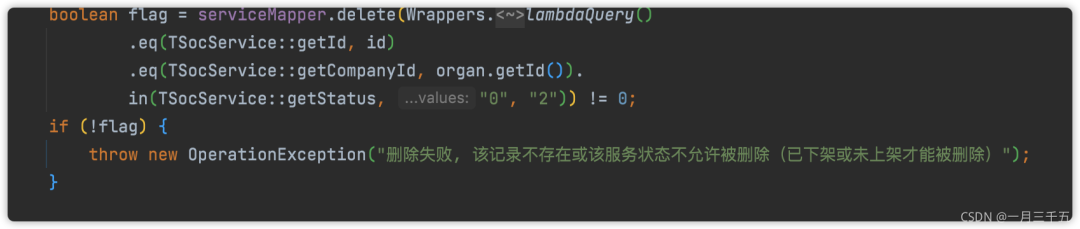
6. Don't return null
Counterexample
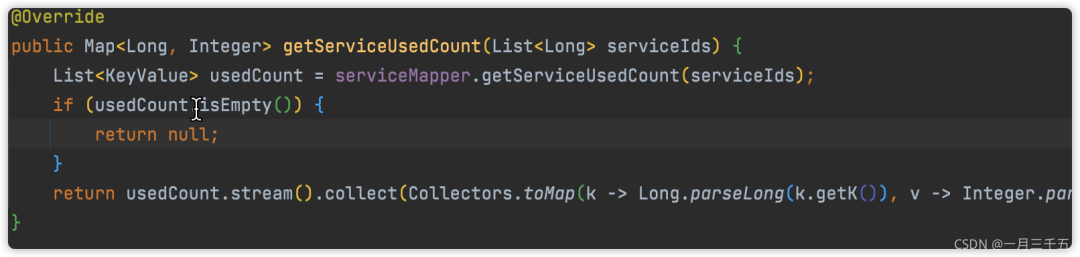
Positive example
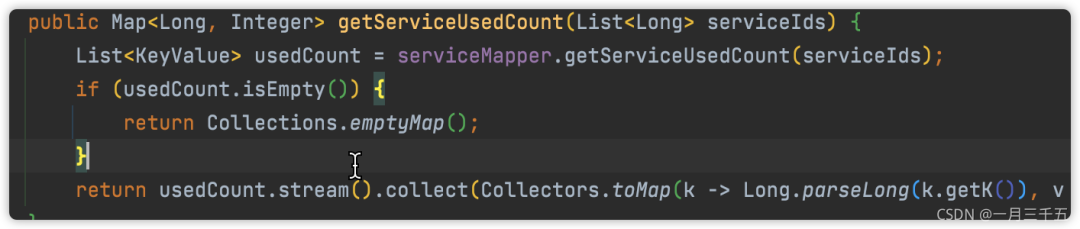
Avoid unnecessary null pointers when calling methods elsewhere
7. if else
Not too much if else if
You can try the strategy mode instead
8. Reduce the business code of controller
The business code should be handled in the service layer as much as possible. It is easy to maintain, operate and beautiful in the later stage
Counterexample
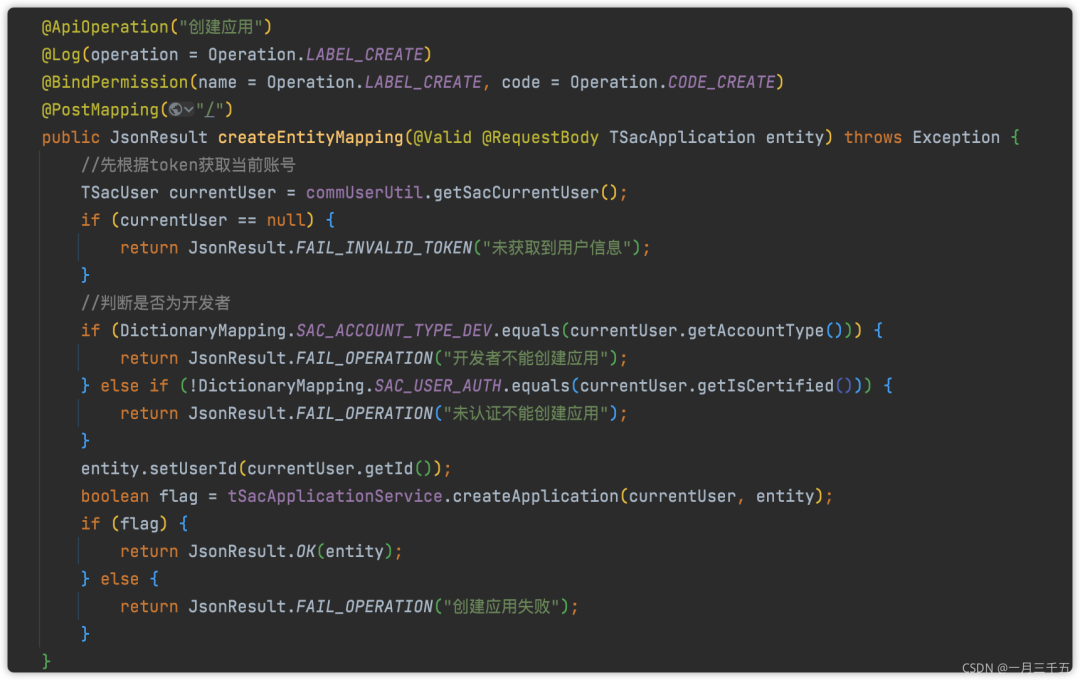
Positive example
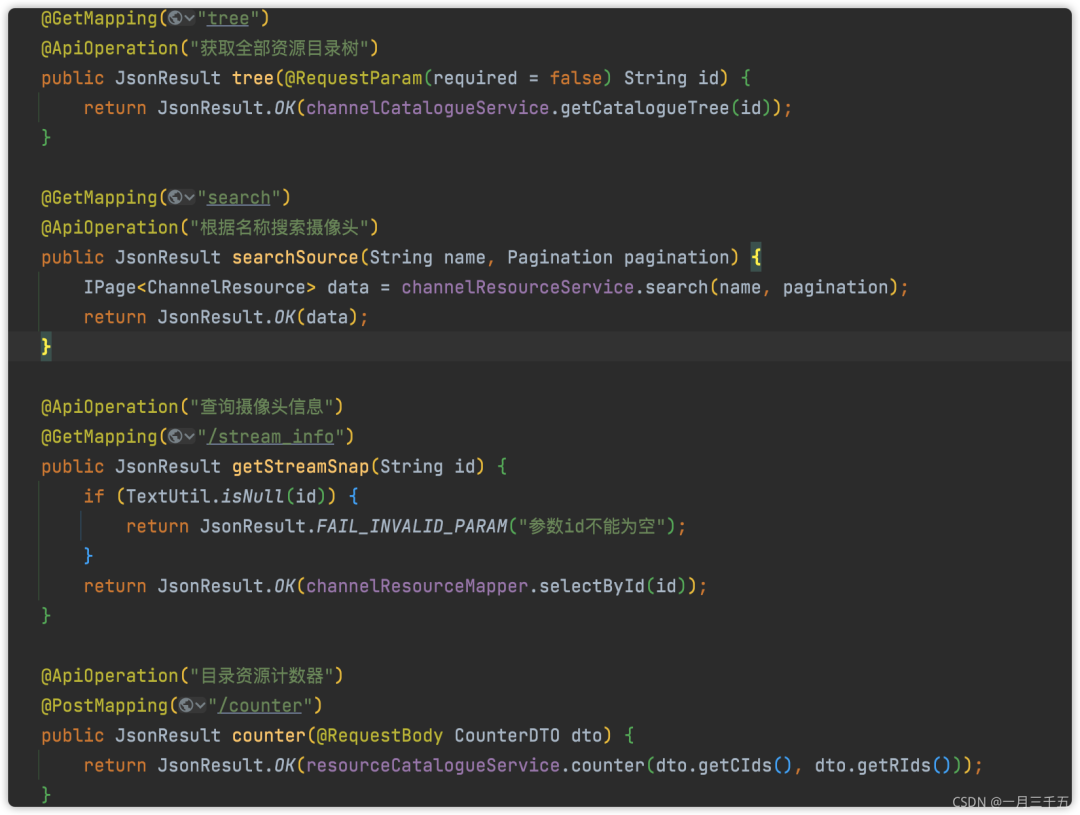
9. Make good use of Idea
So far, enterprises on the market basically use idea as a development tool
Take a small example
idea will judge our code and make reasonable suggestions
For example:

It recommends that we use the form of lanbda instead
Click replace

10. Read the source code
Be sure to develop the good habit of reading the source code, including stars: > 1000 on GitHub, an excellent open source project. You will learn a lot of knowledge from it, including its design idea of code and advanced API. Plus points in the interview (many interviewers are used to asking about the source code)
11. Design mode
For 23 design patterns, try to use the idea of design pattern in the code. The code written is standard, beautiful and tall.
12. Embrace new knowledge
For programmers with less working years like us, I think we should learn more knowledge beyond our own cognition. We can't crud every day. If we have the opportunity, we can use more difficult knowledge. If we don't have the opportunity (the project is more traditional), we can practice more relevant demo after work
13. Basic issues
map traversal
HashMap<String, String> map = new HashMap<>(); map.put("name", "du"); for (String key : map.keySet()) { String value = map.get(key); } map.forEach((k, v) -> { }); // recommend for (Map.Entry<String, String> entry : map.entrySet()) { }
optional blank judgment
//Get subdirectory list public List<CatalogueTreeNode> getChild(String pid) { if (V.isEmpty(pid)) { pid = BasicDic.TEMPORARY_DIRECTORY_ROOT; } CatalogueTreeNode node = treeNodeMap.get(pid); return Optional.ofNullable(node) .map(CatalogueTreeNode::getChild) .orElse(Collections.emptyList()); }
recursion
When recursing with a large amount of data, avoid the new object in the recursive method. You can try to pass the object as a method parameter
notes
Class interface method annotations. More complex method annotations should be written clearly. Sometimes they are written not for others, but for yourself
14. Judge whether the element exists
hashSet instead of list
list code to determine whether an element exists
ArrayList<String> list = new ArrayList<>(); // Determine whether a is in the list for (int i = 0; i < list.size(); i++) if ("a".equals(elementData[i])) return i;
It can be seen that its complexity is On
The bottom layer of hashSet uses hashMap as the data structure for storage, and the elements are placed in the key of map (i.e. linked list)
HashSet<String> set = new HashSet<>(); // Judge whether a is in set int index = hash(a); return getNode(index) != null
Thus, its complexity is O1
To be added