- 1. Overview of singleton mode
- 2. Structure and implementation of singleton mode
- 3. Application examples of singleton mode
- 4. Hungry man type single case and lazy man type single case
- Hungry Han type singleton
- Lazy singleton and double check locking
- A comparison between hungry man's single instance class and lazy man's single instance class
- Using static inner classes to implement singleton mode
- 5. Advantages, disadvantages and applicable environment of singleton mode
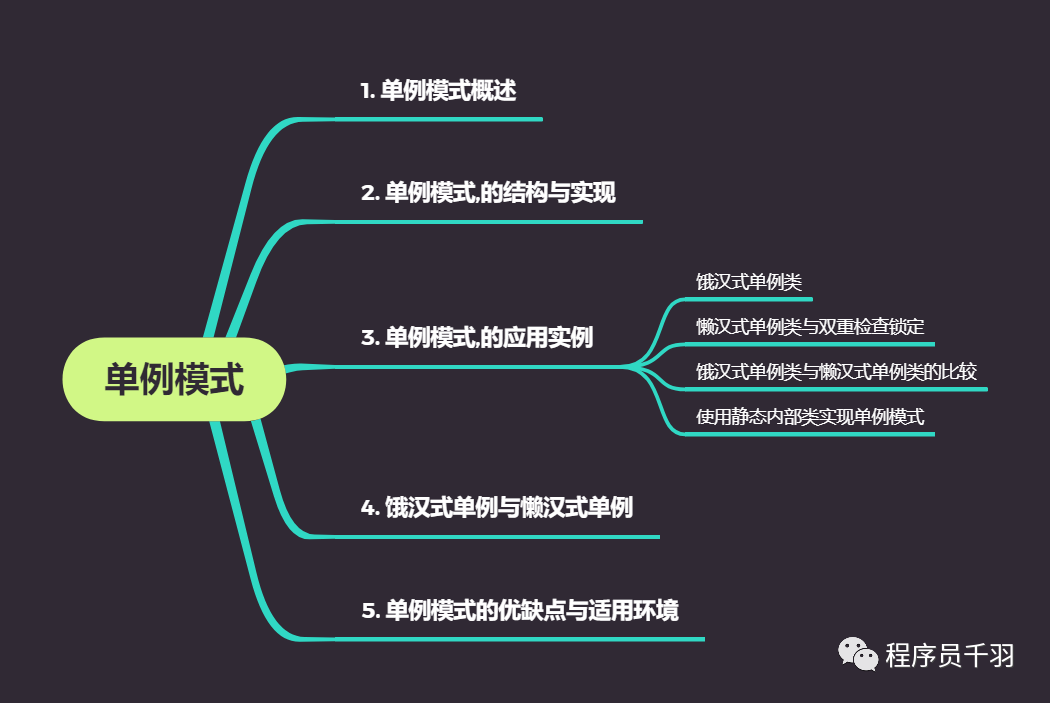
"Github: https://github.com/nateshao/design-demo/tree/main/JavaDesignPatterns/08-singleton
1. Overview of singleton mode
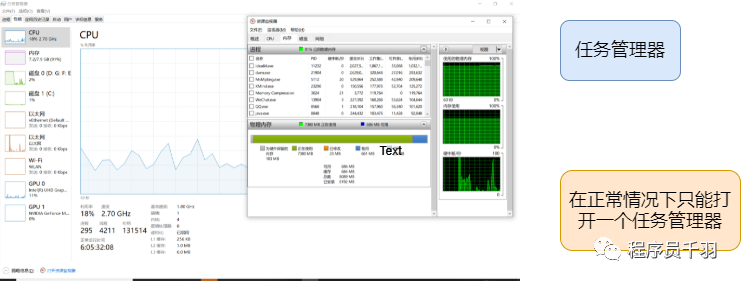
How to ensure that a class has only one instance and that the instance is easy to access?
- Global variable: it can ensure that objects can be accessed at any time, but it can not prevent the creation of multiple objects
- Let the class itself be responsible for creating and saving its unique instance, and ensure that no other instance can be created. It also provides a method to access the instance
Definition of singleton mode
"Singleton mode: ensure that there is only one instance of a class and provide a global access point to access this unique instance.
Object creation mode
main points:
- A class can only have one instance
- You must create this instance yourself
- You must provide this instance to the entire system yourself
2. Structure and implementation of singleton mode
Structure of singleton mode
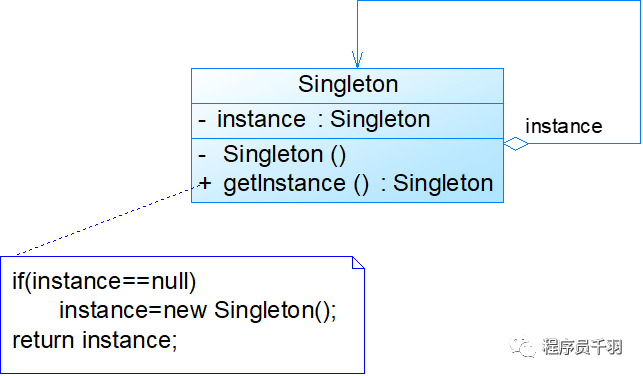
**The Singleton mode contains only one Singleton role: * * Singleton
Implementation of singleton mode
- private constructors
- Static private member variable (self type)
- Static public factory method
public class Singleton { private static Singleton instance = null; //Static private member variable //private constructors private Singleton() { } //Static public factory method that returns a unique instance public static Singleton getInstance() { if (instance == null) instance = new Singleton(); return instance; } }
3. Application examples of singleton mode
Example description:
A software company has undertaken the development of a server load balance software. The software runs on a load balance server and can distribute concurrent access and data traffic to multiple devices in the server cluster for concurrent processing, which improves the overall processing capacity of the system and shortens the response time. Because the servers in the cluster need to be dynamically deleted and the client requests need to be uniformly distributed, it is necessary to ensure the uniqueness of the load balancer. There can only be one load balancer to be responsible for server management and request distribution, otherwise it will lead to problems such as inconsistent server status and request allocation conflict. How to ensure the uniqueness of the load balancer is the key to the success of the software. Try to use the singleton mode to design the server load balancer.
Instance class diagram
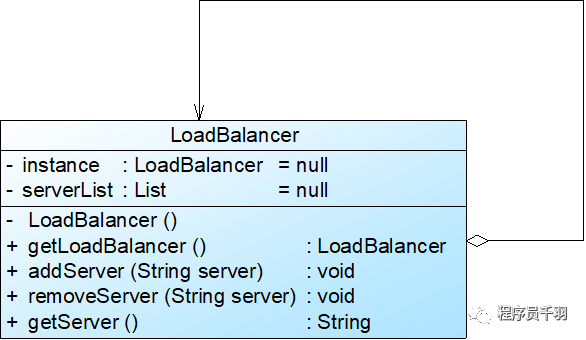
Example code
- LoadBalancer: load balancer class, which acts as a singleton
- Client: client test class
analysis:
//Determine whether the server load balancer is the same if (balancer1 == balancer2 && balancer2 == balancer3 && balancer3 == balancer4) { System.out.println("Server load balancer is unique!"); }
4. Hungry man type single case and lazy man type single case
Hungry Han type singleton
Hungry singleton
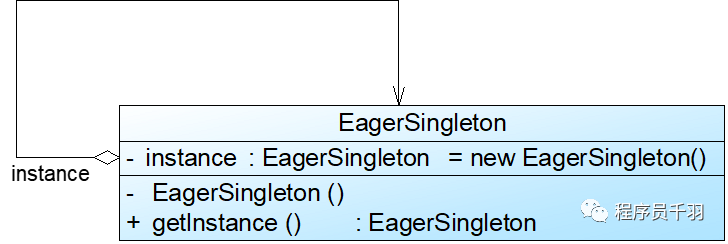
public class EagerSingleton { private static final EagerSingleton instance = new EagerSingleton(); private EagerSingleton() { } public static EagerSingleton getInstance() { return instance; } }
Lazy singleton and double check locking
Lazy singleton
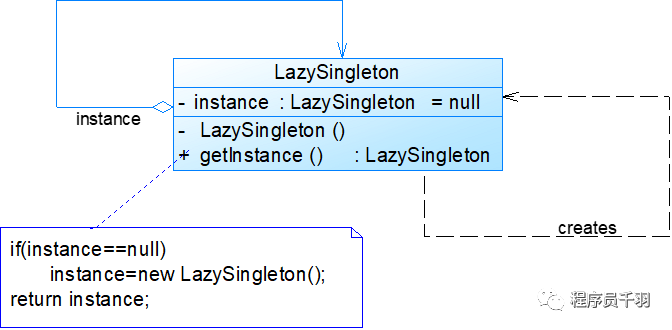
Delayed loading
public class LazySingleton { private static LazySingleton instance = null; private LazySingleton() { } public static LazySingleton getInstance() { if (instance == null) { instance = new LazySingleton(); } return instance; } }
Simultaneous access by multiple threads will result in the creation of multiple singleton objects! What should I do?
public class LazySingleton { private static LazySingleton instance = null; private LazySingleton() { } // Lock method synchronized public static LazySingleton getInstance() { if (instance == null) { instance = new LazySingleton(); } return instance; } }
...... public static LazySingleton getInstance() { if (instance == null) { // Lock code snippet synchronized (LazySingleton.class) { instance = new LazySingleton(); } } return instance; } ......
public class LazySingleton { private volatile static LazySingleton instance = null; private LazySingleton() { } // Double check locking public static LazySingleton getInstance() { //First judgment if (instance == null) { //Lock code block synchronized (LazySingleton.class) { //Second judgment if (instance == null) { instance = new LazySingleton(); //Create a singleton instance } } } return instance; } }
A comparison between hungry man's single instance class and lazy man's single instance class
Hungry Chinese singleton class: there is no need to consider the simultaneous access of multiple threads; The calling speed and reaction time are better than lazy single case; Resource utilization efficiency is not as good as lazy single case; The system may take a long time to load
Lazy singleton class: implements delayed loading; The problem of simultaneous access of multiple threads must be handled; It needs to be controlled through mechanisms such as double check locking, which will affect the system performance to a certain extent
Using static inner classes to implement singleton mode
- The best implementation in the Java language
- Initialization on Demand Holder (IoDH): use static inner class
//Initialization on Demand Holder public class Singleton { private Singleton() { } //Static inner class private static class HolderClass { private final static Singleton instance = new Singleton(); } public static Singleton getInstance() { return HolderClass.instance; } public static void main(String args[]) { Singleton s1, s2; s1 = Singleton.getInstance(); s2 = Singleton.getInstance(); System.out.println(s1==s2); } }
5. Advantages, disadvantages and applicable environment of singleton mode
Mode advantages
- Provides controlled access to unique instances
- It can save system resources and improve system performance
- Allow variable instances (multi instance classes)
Mode disadvantages
- Difficult to extend (lack of abstraction layer)
- The singleton class has too much responsibility
- Due to the automatic garbage collection mechanism, the state of shared singleton objects may be lost
Mode applicable environment
- The system only needs one instance object, or only one object is allowed to be created because of too much resource consumption
- A single instance of a client calling class is allowed to use only one public access point. In addition to the public access point, the instance cannot be accessed through other ways