Through two blog posts, we learned that reflection accesses objects and members in objects through non instantiation (new). Not only that, reflection can also break through the restriction of access modifiers and spy on all members (fields, attributes and methods) inside objects from the perspective of God, including private members, It provides a strong and powerful support for us to design program architecture from another level and loose module coupling.
In this blog case, the separation is more thorough and completely from the perspective of God. Let's talk about the previous synopsis: for His manufacturers, there is only one His, but the medical insurance that His wants to connect may vary, and His can't connect all medical insurance. Therefore, here we need to decouple. First, we need to define an interface to complete the standard definition, As long as both His and Medicare interfaces comply with this specification, the interface appears:
using System;
namespace HisMedical
{
/// <summary>
/// HIS
/// </summary>
public interface IHis
{
/// <summary>
/// his Registration number
/// </summary>
string RegisterID { set; }
/// <summary>
/// Hospitalization registration
/// </summary>
/// <returns></returns>
dynamic Register();
/// <summary>
/// pay
/// </summary>
/// <returns></returns>
dynamic Fee();
}
}
This interface is encapsulated into a separate DLL called hismedical dll
First of all, this interface should be implemented in His to complete the linkage call of all actions in His. There are two dynamics here: one is registration and hospitalization, and the other is hospitalization payment:
/// <summary>
/// his Registration and hospitalization
/// </summary>
/// <param name="his"></param>
/// <returns></returns>
static string Register(IHis his)
{
var registerID = DateTime.Now.ToString("yyyyMMddHHmmss");
Console.WriteLine($"*****complete His Registration number:{registerID}");
his.RegisterID = registerID;
var result = his.Register();
return registerID;
}
/// <summary>
/// his pay
/// </summary>
/// <param name="his"></param>
/// <param name="registerID"></param>
/// <returns></returns>
static bool Fee(IHis his, string registerID)
{
Console.WriteLine($"*****complete His Settlement, registration number:{registerID}");
his.RegisterID = registerID;
var result = his.Fee();
return true;
}
Next, you can implement the dll of medical insurance interface:
Let's start with Neusoft:
using HisMedical;
using System;
namespace NeusoftMedical
{
/// <summary>
/// Neusoft interface
/// </summary>
public class NeusoftMedical : IHis
{
/// <summary>
/// his Registration number
/// </summary>
public string RegisterID { set; private get; }
/// <summary>
/// pay
/// </summary>
/// <returns></returns>
public dynamic Fee()
{
Console.WriteLine("-----Complete the hospitalization payment of Neusoft medical insurance");
return true;
}
/// <summary>
/// Hospitalization registration
/// </summary>
/// <returns></returns>
public dynamic Register()
{
Console.WriteLine("-----Complete the hospitalization registration of Neusoft medical insurance");
return true;
}
}
}
Take another look at the silver sea:
using HisMedical;
using System;
namespace YiHaiMedical
{
/// <summary>
/// Yinhai interface
/// </summary>
public class YinHaiMedical : IHis
{
/// <summary>
/// his Registration number
/// </summary>
public string RegisterID { set; private get; }
/// <summary>
/// pay
/// </summary>
/// <returns></returns>
public dynamic Fee()
{
Console.WriteLine("=====Complete the hospitalization payment of Yinhai medical insurance");
return true;
}
/// <summary>
/// Hospitalization registration
/// </summary>
/// <returns></returns>
public dynamic Register()
{
Console.WriteLine("=====Complete the hospitalization registration of Yinhai medical insurance");
return true;
}
}
}
The implementation of each interface depends on the RegisterID to organize the corresponding data from the Reading Library of His, so as to decouple it from His to the greatest extent.
How to call different interfaces in His? We can configure the path of medical insurance dll into a table or configuration file. When His starts, it will be ok to automatically load these DLLs. It depends on how the code is loaded.
static void Main(string[] args)
{
while (true)
{
Console.WriteLine("1,Neusoft 2. Yinhai");
var no = Console.ReadLine();
Console.WriteLine("1,Hospitalization registration 2. Hospitalization settlement");
var busNo = Console.ReadLine();
var path = "";
switch (no)
{
case "1":
path = @"C:\MyFile\Source\Repos\Asp.NetCoreExperiment\Asp.NetCoreExperiment\Architecture\NeusoftMedical\bin\Debug\netstandard2.0\NeusoftMedical.dll";
break;
case "2":
path = @"C:\MyFile\Source\Repos\Asp.NetCoreExperiment\Asp.NetCoreExperiment\Architecture\YiHaiMedical\bin\Debug\netstandard2.0\YiHaiMedical.dll";
break;
}
//Load the application set from the file and get the specific type
var medicalType = Assembly.LoadFile(path).GetTypes().FirstOrDefault(t => t.GetInterfaces().Where(s => s.Name == "IHis").Count() > 0);
IHis his = (IHis)Activator.CreateInstance(medicalType);
var registerID = "";
switch (busNo)
{
case "1":
registerID = Register(his);
break;
case "2":
if (registerID != "")
{
Fee(his, registerID);
}
else
{
Console.WriteLine("Please register for hospitalization first");
}
break;
}
}
}
The first switch in the code is the medical insurance interface selection. Look at the interface loaded from the configuration file. The second switch is equivalent to an operation executed in His. It is not difficult to see that the dll is loaded through reflection to decouple the interface between His and medical insurance to the greatest extent. Any new medical insurance interface to be docked in the future is insensitive to His (in this case, it may not be insensitive, because it is only a simple code, and there are no implementation of asynchronous call of medical insurance and special interface correspondence).
Through the three blog posts, we have a brief look at the ability of reflection, which can enable architects to play freely. In the first two articles, through the centralized development of two conversion methods, other development has become manual work (which can be realized by junior programmers). This article also allows architects to easily realize docking regardless of the implementation of specific medical insurance interfaces. Therefore, reflection is the magic weapon of architects
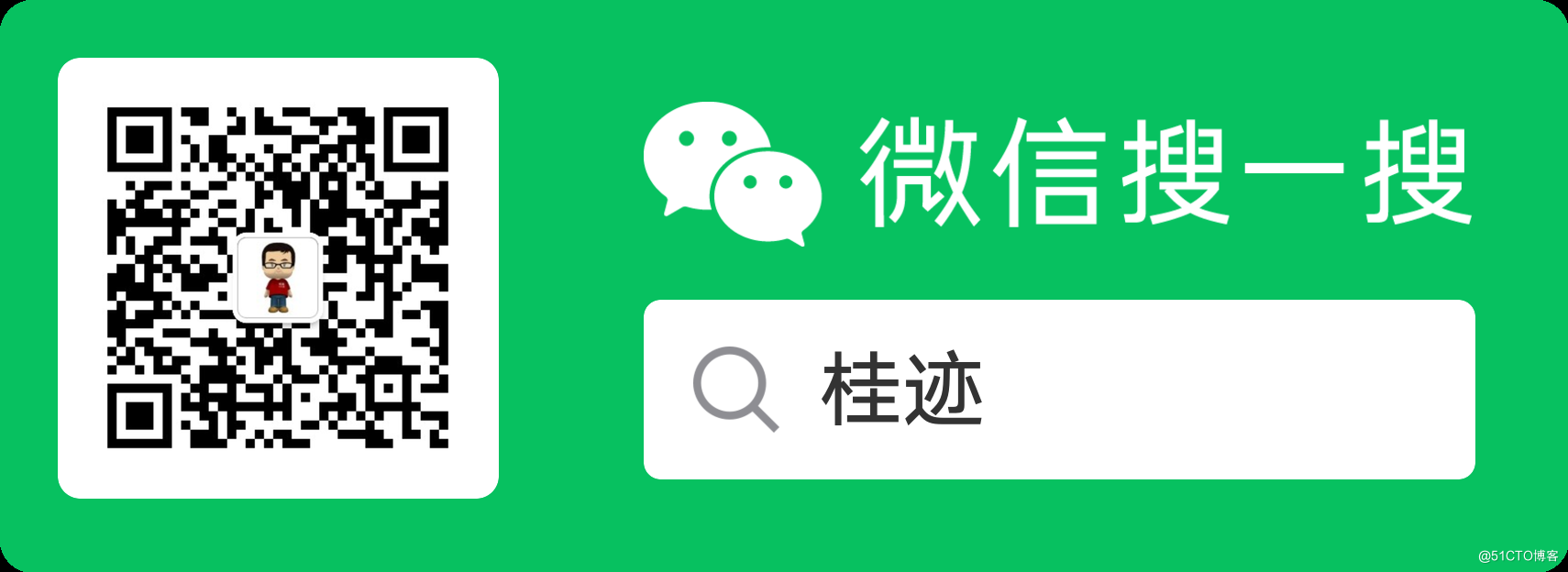