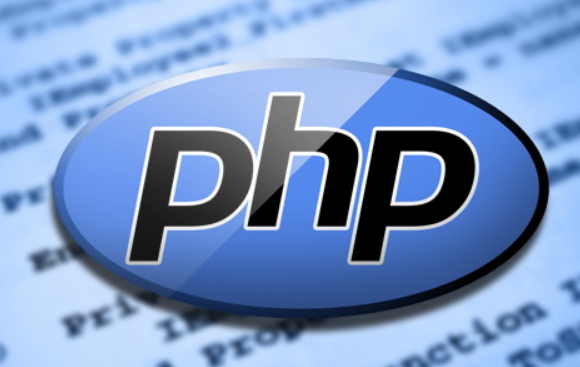
As you all know, php is constantly updated and expanded, and each version has a performance improvement. Next, I will explain the new features of php 7. X. I will explain the features of each version.
- New features of PHP7.0
- New features of PHP7.1
- New features of PHP7.2
- New features of PHP7.3
- New features of PHP7.4
New features of PHP7.0
1. Declaration of scalar type
Scalar type declarations have two modes: mandatory (default) and strict. You can now use the following type parameters (whether in forced or strict mode): string, int, float, and bool. They extend the other types introduced in PHP 5: class name, interface, array, and callback types.
PHP scalar contains: string, int, float, and bool.
For example, let's define a parameter whose formal parameter is an integer. (correct as follows)
<?php //Enter your code here, enjoy! function sumOfInts(int ...$ints) { return array_sum($ints); } var_dump(sumOfInts(2, '3', 4.1));
Output:
int(9)
For example, let's define a parameter whose formal parameter is an integer. (wrong as follows)
<?php //Enter your code here, enjoy! function sumOfInts(int ...$ints) { return array_sum($ints); } var_dump(sumOfInts(2, 'error', 4.1));//There is a string in the parameter. We declare an integer
Output error message:
<br /> <b>Fatal error</b>: Uncaught TypeError: Argument 2 passed to sumOfInts() must be of the type integer, string given, called in [...][...] on line 8 and defined in [...][...]:3 Stack trace: #0 [...][...](8): sumOfInts(2, 'error', 4.1) #1 {main} thrown in <b>[...][...]</b> on line <b>3</b><br />
PHP 7 adds support for return type declaration. Similar to the parameter type declaration, the return type declaration indicates the type of the function return value. The types available are the same as those available in parameter declarations.
For example, let's define a function whose return value is an array.
<?php function arraysSum(array ...$arrays): array { return array_map(function(array $array): int { return array_sum($array); }, $arrays); } print_r(arraysSum([1,2,3], [4,5,6], [7,8,9]));
Array ( [0] => 6 [1] => 15 [2] => 24 )
Due to the fact that a lot of ternary expressions and isset() are used at the same time in daily use, we add the syntax sugar of null merge operator (??). If the variable exists and the value is not null, it will return its own value, otherwise it will return its second operand.
4. Spaceship operator (combination comparator)<?php // If $_GET['user '] does not exist, execute no body assignment to $username $username = $_GET['user'] ?? 'nobody'; // The above statement is equivalent to the following statement $username = isset($_GET['user']) ? $_GET['user'] : 'nobody'; // Coalesces can be chained: this will return the first // defined value out of $_GET['user'], $_POST['user'], and // 'nobody'. $username = $_GET['user'] ?? $_POST['user'] ?? 'nobody'; ?>
The spaceship operator is used to compare two expressions. When $a is less than, equal to, or greater than $b, it returns - 1, 0, or 1, respectively. The principle of comparison is to follow the regular comparison rules of PHP.
<?php // integer echo 1 <=> 1; // 0 echo 1 <=> 2; // -1 echo 2 <=> 1; // 1 // Floating point number echo 1.5 <=> 1.5; // 0 echo 1.5 <=> 2.5; // -1 echo 2.5 <=> 1.5; // 1 // Character string echo "a" <=> "a"; // 0 echo "a" <=> "b"; // -1 echo "b" <=> "a"; // 1 ?>
Constants of type Array can now be defined through define(). Only const can be defined in PHP 5.6.
<?php define('ANIMALS', [ 'dog', 'cat', 'bird' ]); echo ANIMALS[1]; // Output "cat" ?>
6. anonymous category
Now it is supported to instantiate an anonymous class through new class, which can be used to replace some complete class definitions of "burn after use".
<?php interface Logger { public function log(string $msg); } class Application { private $logger; public function getLogger(): Logger { return $this->logger; } public function setLogger(Logger $logger) { $this->logger = $logger; } } $app = new Application; $app->setLogger(new class implements Logger { public function log(string $msg) { echo $msg; } }); var_dump($app->getLogger()); ?>
7.Unicode codepoint translation syntax
This takes a Unicode codepoint in hexadecimal form and prints out a string in UTF-8 encoding surrounded by double quotes or heredoc. Any valid codepoint can be accepted, and the first 0 can be omitted.
echo "\u{aa}"; echo "\u{0000aa}"; echo "\u{9999}";
ª ª (same as before but with optional leading 0's) fragrant
8.Closure::call()
Close:: call() now has better performance by briefly and concisely temporarily binding a method to an object and calling it.
<?php class A {private $x = 1;} // Code before PHP 7 $getXCB = function() {return $this->x;}; $getX = $getXCB->bindTo(new A, 'A'); // Middle closure echo $getX(); // PHP 7 + and later $getX = function() {return $this->x;}; echo $getX->call(new A);
The above routine will output:
1 1
9.unserialize() provides filtering
This feature is designed to provide a more secure way to unpack unreliable data. It prevents potential code injection through a white list.
// Convert all objects to PHP incomplete class objects $data = unserialize($foo, ["allowed_classes" => false]); // Convert all objects except MyClass and MyClass2 to PHP incomplete class objects $data = unserialize($foo, ["allowed_classes" => ["MyClass", "MyClass2"]); // By default, all classes are acceptable, equivalent to omitting the second parameter $data = unserialize($foo, ["allowed_classes" => true]);
The above routine will output:
<?php printf('%x', IntlChar::CODEPOINT_MAX); echo IntlChar::charName('@'); var_dump(IntlChar::ispunct('!'));
10ffff COMMERCIAL AT bool(true)
11. expectations
The expectation is to use both backward and enhance the previous assert() method. It makes it zero cost to enable assertions in the production environment and provides the ability to throw specific exceptions when assertions fail.
The old API will continue to be maintained for compatibility purposes. assert() is now a language structure that allows the first parameter to be an expression, not just a string to be evaluated or a boolean to be tested.
<?php ini_set('assert.exception', 1); class CustomError extends AssertionError {} assert(false, new CustomError('Some error message')); ?>
Fatal error: Uncaught CustomError: Some error message
12.Group use declarations
Classes, functions, and constants imported from the same namespace can now be imported once through a single use statement.
<?php // Code before PHP 7 use some\namespace\ClassA; use some\namespace\ClassB; use some\namespace\ClassC as C; use function some\namespace\fn_a; use function some\namespace\fn_b; use function some\namespace\fn_c; use const some\namespace\ConstA; use const some\namespace\ConstB; use const some\namespace\ConstC; // PHP 7 + and later use some\namespace\{ClassA, ClassB, ClassC as C}; use function some\namespace\{fn_a, fn_b, fn_c}; use const some\namespace\{ConstA, ConstB, ConstC}; ?>
13. Generators can return expressions
This feature is built on the generator feature introduced in PHP version 5.5. It allows you to return an expression in the generator function by using the return syntax, but not to return the reference value. You can get the return value of the generator by calling the Generator::getReturn() method, but this method can only be called once the generator completes the production.
<?php $gen = (function() { yield 1; yield 2; return 3; })(); foreach ($gen as $val) { echo $val, PHP_EOL; } echo $gen->getReturn(), PHP_EOL;
1 2 3
14.Generator delegation
Now, just use yield from in the outermost generation to automatically delegate one generator to another generator, Traversable object or array.
<?php function gen() { yield 1; yield 2; yield from gen2(); } function gen2() { yield 3; yield 4; } foreach (gen() as $val) { echo $val, PHP_EOL; } ?>
1 2 3 4
15. Integer division function intdiv()
The new function intdiv() is used to divide the integer.
<?php var_dump(intdiv(10, 3)); ?>
The above routine will output:
int(3)
16. Session options
session_start() can take an array as a parameter to override the session configuration options set in the php.ini file.
When session start() is called, the session. Lazy write behavior is also supported in the passed in option parameters. By default, this configuration item is open. Its function is to control PHP to write to the session storage file only when the data in the session changes. If the data in the session does not change, PHP will close the session storage file immediately after reading the session data, without any modification. It can be realized by setting read and close.
For example, the following code sets session.cache'limiter to private and closes the session storage file as soon as the session data is read.
<?php session_start([ 'cache_limiter' => 'private', 'read_and_close' => true, ]); ?>
17.preg_replace_callback_array()
Before PHP 7, when the preg_replace_callback() function was used, the callback function was executed for each regular expression, which may lead to too much branch code. The new preg replace callback array() function can make the code more concise.
Now, you can use an associative array to register a callback function for each regular expression. The regular expression itself is the key of the associative array, and the corresponding callback function is the value of the associative array.
18.CSPRNG Functions
Two new cross platform functions are added: random_bytes() and random_int() to generate high security level random strings and random integers. You can use the list() function to expand the object ¶ that implements the ArrayAccess interface In the previous version, the list() function could not guarantee the correct expansion of the objects that implement the ArrayAccess interface. Now this problem has been fixed.
19. Other characteristics
Allow access to object members on clone expressions, for example: (clone $foo) - > bar().
New features of PHP7.1
1. Nullable type
Parameters and the type of the return value can now be allowed to be empty by prefixing the type with a question mark. When this feature is enabled, the result returned by the passed in parameter or function is either of the given type or null .
<?php function testReturn(): ?string { return 'elePHPant'; } var_dump(testReturn()); function testReturn(): ?string { return null; } var_dump(testReturn()); function test(?string $name) { var_dump($name); } test('elePHPant'); test(null); test();
The above routine will output:
string(10) "elePHPant" NULL string(10) "elePHPant" NULL Uncaught Error: Too few arguments to function test(), 0 passed in...
2.Void function
A new return value type void was introduced. A method whose return value is declared as void either omits the return statement or uses an empty return statement. NULL is not a legal return value for void functions.
<?php function swap(&$left, &$right) : void { if ($left === $right) { return; } $tmp = $left; $left = $right; $right = $tmp; } $a = 1; $b = 2; var_dump(swap($a, $b), $a, $b);
The above routine will output:
null int(2) int(1)
Trying to get the return value of a void method will get NULL without any warning. The reason for this is that you don't want to influence a higher level approach.
3. Class constant visibility
<?php class Sky8g { const PUBLIC_CONST_A = 1; public const PUBLIC_CONST_B = 2; protected const PROTECTED_CONST = 3; private const PRIVATE_CONST = 4; }
4.iterable pseudo class
Now a new one called iterable Pseudo classes of (and callable Similar). This can be used in parameter or return value types, which represent objects that accept arrays or implement the Traversable interface. As for subclasses, when used as parameters, subclasses can tighten the iterable Type to array Or an object that implements Traversable. For return values, subclasses can expand the array Or object return value type to iterable.
<?php function iterator(iterable $iter) { foreach ($iter as $val) { // } }
5. Multi exception capture processing
A catch statement block can now catch multiple exceptions through a pipe character (|). This is useful when you need to handle different exceptions from different classes at the same time.
<?php try { // some code } catch (FirstException | SecondException $e) { // handle first and second exceptions }
6.list() now supports key names
Now list() And its new [] syntax supports specifying key names inside it. This means that it can assign any type of array to some variables (similar to short array syntax)
<?php $data = [ ["id" => 1, "name" => 'Tom'], ["id" => 2, "name" => 'Fred'], ]; // list() style list("id" => $id1, "name" => $name1) = $data[0]; // [] style ["id" => $id1, "name" => $name1] = $data[0]; // list() style foreach ($data as list("id" => $id, "name" => $name)) { // logic here with $id and $name } // [] style foreach ($data as ["id" => $id, "name" => $name]) { // logic here with $id and $name }
7. Support negative string offset
Now all of the String manipulation function Both support accepting negative numbers as offsets, including through [] or {} operations String subscript . In this case, a negative offset is interpreted as an offset starting at the end of the string.
<?php var_dump("abcdef"[-2]); var_dump(strpos("aabbcc", "b", -3));
string (1) "e" int(3)
New features of PHP7.2
1. New object type
This new object type, object, introduces any object type that can be used for contravariant parameter input and covariant return.
<?php function test(object $obj) : object { return new SplQueue(); } test(new StdClass());
2. Load extension by name
Extension files no longer need to be specified by file loading (Unix with. so as file extension, Windows with. dll as file extension). You can enable it in the php.ini configuration file or using the dl() function.
3. Allow to override abstract method
When an abstract class inherits from another abstract class, the inherited abstract class can override the abstract methods of the inherited abstract class.
<?php abstract class A { abstract function test(string $s); } abstract class B extends A { // overridden - still maintaining contravariance for parameters and covariance for return abstract function test($s) : int; }
4. Using Argon2 algorithm to generate password hash
Argon2 has been added to the password hashing API (these functions start with "password"), and the following are the exposed constants:
- PASSWORD_ARGON2I
- PASSWORD_ARGON2_DEFAULT_MEMORY_COST
- PASSWORD_ARGON2_DEFAULT_TIME_COST
- PASSWORD_ARGON2_DEFAULT_THREADS
5. Add ext/PDO (PDO extension) string extension type
When you are ready to support multilingual character sets, PDO's string type has been extended to support internationalized character sets. Here are the extended constants:
- PDO::PARAM_STR_NATL
- PDO::PARAM_STR_CHAR
- PDO::ATTR_DEFAULT_STR_PARAM
These constants are calculated by PDO:: param ﹣ STR using a bitwise OR operation:
<?php $db->quote('über', PDO::PARAM_STR | PDO::PARAM_STR_NATL);
6. Add additional simulation debugging information for ext/PDO
Pdostatement:: debugdumparams() method has been updated. When sending SQL to the database, debugging information will be displayed in consistency and row query (including replacing binding placeholders). This feature has been added to analog debugging (available when analog debugging is turned on).
7.ext/LDAP (LDAP extension) supports new operation mode
The LDAP extension has added EXOP support. The extension exposes the following functions and constants:
- ldap_parse_exop()
- ldap_exop()
- ldap_exop_passwd()
- ldap_exop_whoami()
- LDAP_EXOP_START_TLS
- LDAP_EXOP_MODIFY_PASSWD
- LDAP_EXOP_REFRESH
- LDAP_EXOP_WHO_AM_I
- LDAP_EXOP_TURN
8.ext/sockets (sockets extension) adds address information
sockets extension now has the ability to find address information, and can connect to this address, or bind and parse. The following functions have been added for this purpose:
- socket_addrinfo_lookup()
- socket_addrinfo_connect()
- socket_addrinfo_bind()
- socket_addrinfo_explain()
9. Extended parameter type
Parameter types for overriding methods and interface implementations can now be omitted. However, this is still in line with LSP, because now this parameter type is inverted.
10. Allow trailing comma of grouping namespace<?php interface A { public function Test(array $input); } class B implements A { public function Test($input){} // type omitted for $input }
Namespaces can be introduced as groupings in PHP 7 using trailing commas.
New features of PHP7.3
1.Unicode 11 support
The multibyte string data table has been updated to Unicode 11.
2. Long string support
Multibyte string functions now properly support strings larger than 2GB.
3. Performance improvement
The performance of multibyte string extension has been improved significantly. The biggest improvement is the case conversion feature.
4. Support for custom naming
The MB? Ereg? Function now supports named snaps. Matching functions such as MB? Ereg () will now return the specified capture using their group number and name, similar to PCRE:
<?php mb_ereg('(?<word>\w+)', 'country', $matches); // =>[0 = > country, 1 = > country, word = > country]; ?>
In addition, MB? Ereg? Replace() now supports the \ k < > and \ k "symbols to refer to the specified capture in the replacement string:
<?php mb_ereg_replace('\s*(?<word>\w+)\s*', "_\k<word>_\k'word'_", ' foo '); // => "_foo_foo_" ?>
\k < > and \ k "can also be used for number references or group numbers greater than 9.
New features of PHP7.4
1. Type attribute
Class properties now support type declarations.
<?php class User { public int $id; public string $name; } ?>
The above example will enforce that $user - > ID can only be assigned to integer values, while $user - > name can only be assigned to string values.
2. Arrow function
Arrow functions provide a shorthand syntax for defining functions using implicit value range binding.
<?php $factor = 10; $nums = array_map(fn($n) => $n * $factor, [1, 2, 3, 4]); // $nums = array(10, 20, 30, 40); ?>
3. Limit return type and parameter type inverter
<?php class A {} class B extends A {} class Producer { public function method(): A {} } class ChildProducer extends Producer { public function method(): B {} } ?>
Full variance support is only available when using autoload. In a single file, only acyclic type references are possible, because all classes must be available before they can be referenced.
4.Null merge assignment operator
<?php $array['key'] ??= computeDefault(); // is roughly equivalent to if (!isset($array['key'])) { $array['key'] = computeDefault(); } ?>
5. New way to merge arrays
<?php $parts = ['apple', 'pear']; $fruits = ['banana', 'orange', ...$parts, 'watermelon']; // ['banana', 'orange', 'apple', 'pear', 'watermelon']; ?>
6. Numeric text separator
Numeric text can contain underscores between numbers.
<?php 6.674_083e-11; // float 299_792_458; // decimal 0xCAFE_F00D; // hexadecimal 0b0101_1111; // binary ?>
7. weak references
Weak references allow programmers to keep references to objects without preventing them from being destroyed.
If you don't understand, please leave a message. SKY8G website editors focus on the research and development of IT source code. I hope you will come next time. Your approval and message are our greatest support. Thank you!