Port listening
The essence of the server is port communication, so you only need to listen to port 80 to carry out Http communication. This article uses the express module to listen
//Introducing express module const Express = require("express"); //Create server application const App = Express(); //Listen to port 80 App.listen(80); Now a server application is created and opened on the computer.0.0.1,If you see"Cannot GET /",It means that the server is running normally Processing requests //Introducing express module const Express = require("express"); //Create server application const App = Express(); App.get('/',(request, response)=>{ response.write("Hello World!");//Write web content response.end();//End response }); //Listen to port 80 App.listen(80);
Output the content of the web page in the response, and end() to end the response.
The end () method makes the server think that all data has been sent, and force the connection to be disconnected whether the client receives it or not. If you try to send data after end(), an error will be generated
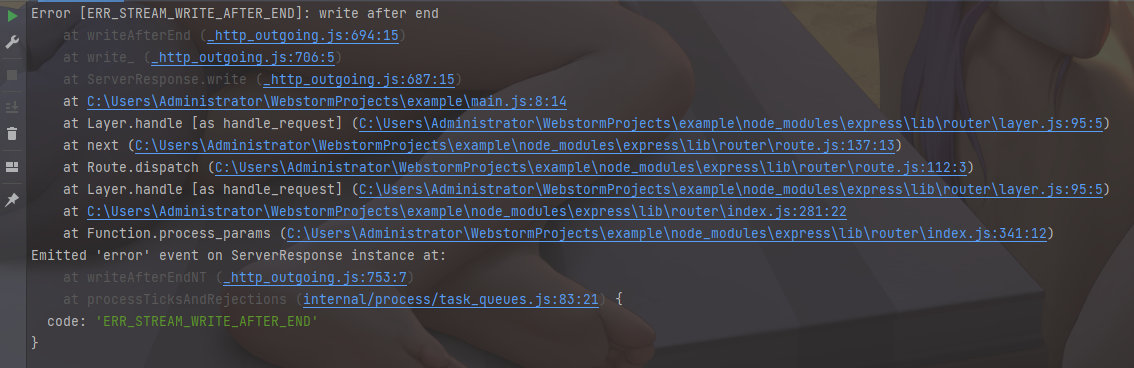
console output
You can use console to output data on the console
//Introducing express module const Express = require("express"); //Create server application const App = Express(); App.get('/',(request, response)=>{ console.log("this is a log"); console.error("this is an error"); response.end();//End response }); //Listen to port 80 App.listen(80);
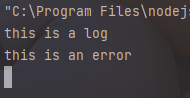
Url parsing
The request contains variables about the url, request Hostname means host name (domain name in the public network), request The url represents the address after the host name
with https://www.dearxuan.top/404?from=csdn take as an example
- url: /404?from=csdn
- hostname: www.dearxuan.top
In addition to getting the url, you can also use query to parse the parameters in the url
Use & split between parameters. If a parameter appears multiple times, it will be automatically saved as an array
Note that spaces and empty characters are also included
//Introducing express module const Express = require("express"); //Create server application const App = Express(); App.get('/',(request, response)=>{ const dic = request.query; console.log(dic); response.end();//End response }); //Listen to port 80 App.listen(80);
Access with browser: http://localhost/?a=1&b=&&&a=2 , it will be output on the console
{ a: [ '1', '2' ], b: '' }
response
Relocation
response.redirect("https://www.dearxuan.top")
Response header
The following code changes the response header to 404. Even if the page exists, it will show that the page cannot be found on the client
//Introducing express module const Express = require("express"); //Create server application const App = Express(); App.get('/',(request, response)=>{ response.writeHead(404,{})//404 Not Found response.end();//End response }); //Listen to port 80 App.listen(80);
subject
Send the web page content to the client with the send() method
//Introducing express module const Express = require("express"); //Create server application const App = Express(); App.get('/',(request, response)=>{ response.send("<html><body>Hello World</body></html>"); response.end();//End response }); //Listen to port 80 App.listen(80);
route
To facilitate the management of different addresses, express supports setting different functions for different routes
Project structure
In order to enhance the scalability of the code, the corresponding methods of all routes are stored in the "router" folder. For example, there is a main in the "router" folder JS file, which is used to process the url path starting with / main, but / main / * is not in this range
The document structure of the project is as follows:
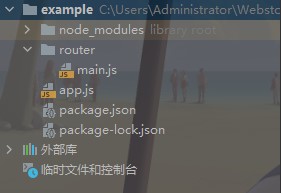
Custom processing function
module.exports = { //The MainPage function can be exported MainPage } function MainPage(request, response){ response.write("Main"); response.end(); }
Set route
const Express = require("express"); const Main = require("./router/main"); const App = Express(); //Use main Mainpage function to handle the get method under the route App.get('/main',Main.MainPage); App.listen(80);
It can now be accessed normally http://localhost/main , but access http://localhost/main/a Will go wrong
Use app Get ('/ main / a', main_a.func) is a good method, but if you want to access http://localhost/main/a/a perhaps http://localhost/main/abc/a/b , this method will appear clumsy
wildcard
"*" (asterisk) means that all characters match, so if you write down app Get ('/ m *', main. Mainpage), then all URLs starting with m will be processed by the MainPage() function, whether or not http://localhost/ma or http://localhost/main/a/b
placeholder
To match what looks like http://localhost/page/12 You can use placeholders for the path of the
App.get('/page/:id',(request, response)=>{ console.log(request.params.id); response.end(); });
The console will output: 12
Adding a question mark indicates whether the placeholder can be present or not
For example, app get('/page/:a?/:b',func)
Then its corresponding url includes
localhost/page/12/34: a='12', b='34' localhost/page/34: a=undefined, b='34'
control power
Express compares all routes one by one according to the url until a matching route is encountered
When all routes cannot match the url, it will display Cannot GET /
In order to navigate the user to the specified error page, wildcards are used to match all URLs
App.get('/main',func1); App.get('/page',func2); //Match all URLs App.get('*',(request, response)=>{ response.write("404 Not Found") response.end(); });
Note the order of routes. If wildcards are used for the first route, then all the next routes cannot obtain the request
Transfer of control
Calling the next() function in the function can give up its control and submit it to the following way to process the request.
App.get('/main',(request, response, next)=>{ next(); }); App.get('*',(request, response)=>{ response.write("404 Not Found") response.end(); });
Static web page
express supports direct return of static files without cumbersome file reading and writing
//Directly return to index html App.use('/main',Express.static('index.html'));