import java.util.ArrayList; public class Test { public static void main(String[] args) { Object obj1 = new Object(); Object obj2 = new Object(); System.out.println(obj1.hashCode()); // 460141958 System.out.println(obj2.hashCode()); // 1163157884 String str = new String(); System.out.println(str.hashCode()); // 0 ArrayList<Integer> arr = new ArrayList<>(); System.out.println(arr.hashCode()); // 1 } }
Generally, obj 1 must be met equals(obj2) == true. Can launch obj 1 hash Code() == obj2. hashCode(), but equal hashcodes do not necessarily satisfy equals. However, in order to improve efficiency, we should try to make the above two conditions close to equivalence.
wait()
The wait() method puts the current thread into a wait state. Until another thread calls the notify() method or notifyAll() method of this object.
notify() wakes up a thread waiting on the object.
notifyAll() wakes up all threads waiting on the object.
import java.util.Date; class WaitTest { public static void main(String[] args) { ThreadA threadA = new ThreadA("threadA"); synchronized (threadA) { try { // Start thread threadA.start(); System.out.println(Thread.currentThread().getName() + " wait() " + new Date()); // The main thread waits for ta to wake up via notify. threadA.wait();// Instead of making the ta thread wait, the thread currently executing the wait waits System.out.println(Thread.currentThread().getName() + " continue " + new Date()); } catch (Exception e) { e.printStackTrace(); } } } } class ThreadA extends Thread { public ThreadA(String name) { super(name); } @Override public void run() { synchronized (this) { try { Thread.sleep(1000); // Block the current thread for 1 second } catch (InterruptedException e) { e.printStackTrace(); } System.out.println(Thread.currentThread().getName() + " call notify()"); this.notify(); } } }
Output results:
main wait() Sat Jul 24 13:03:57 CST 2021 threadA call notify() main continue Sat Jul 24 13:03:58 CST 2021
finalize()
The finalize() method is used for the operation triggered when the instance is collected by the garbage collector.
class Test { public static void main(String[] args) { User u1 = new User(1); User u2 = new User(2); User u3 = new User(3); u2 = u3 = null; System.gc(); } } class User { private int id; public User(int id) { this.id = id; System.out.println("User Object " + id + " is created"); } protected void finalize() throws java.lang.Throwable { super.finalize(); System.out.println("User Object " + id + " is disposed"); } }
About garbage collection:
-
Objects may not be garbage collected. As long as the program is not on the verge of running out of storage space, the space occupied by objects will not be released.
-
Garbage collection is not equal to "Deconstruction".
-
Garbage collection is only memory related. The only reason to use garbage collection is to recycle memory that is no longer used by the program.
Purpose of finalize():
No matter how the object is created, the garbage collector is responsible for freeing all the memory occupied by the object.
This limits the need for finalize() to a special case where storage space is allocated to objects in a way other than the way they are created. However, this situation usually occurs when the local method is used, and the local method is a way to call non Java code in Java.
In Java, strings belong to objects. Java provides String classes to create and manipulate strings.
Creation method
class Test { public static void main(String[] args) { // The object created by direct assignment is the constant pool in the method area String str1 = "hello"; // The string object created by the construction method is in heap memory String str2 = new String("hello"); // Reference passing, str3 directly points to the heap memory address of st2 String str3 = str2; String str4 = "hello"; System.out.println(str1 == str2); // false System.out.println(str1 == str3); // false System.out.println(str3 == str2); // true System.out.println(str1 == str4); // true } }
common method
String judgment
|Method description|
| — | — |
|boolean equals(Object obj) | whether the contents of the comparison string are the same|
|boolean equalsIgnoreCase(String str) | compare whether the contents of strings are the same, ignoring case|
|boolean startsWith(String str) | judge whether the string object starts with the specified str|
|boolean endsWith(String str) | judge whether the string object ends with the specified str|
String interception
|Method description|
| — | — |
|int length() | gets the length of the string, which is actually the number of characters|
|char charAt(int index) | gets the character at the specified index|
|int indexOf(String str) | get the index of the first occurrence of str in the string object|
|String substring(int start) | intercept string from start|
|String substring(int start,int end) | intercept the string from start to end. Including start, excluding end|
String conversion
|Method description|
| — | — |
|char[] toCharArray() | convert string to character array|
|String toLowerCase() | convert string to lowercase string|
|String toUpperCase() | convert string to uppercase string|
Other methods
|Method description|
| — | — |
|String trim() | remove spaces at both ends of the string|
|String[] split(String str) | splits the string according to the specified symbol|
StringBuilder and StringBuffer
StringBuilder is a variable character sequence. It inherits from AbstractStringBuilder and implements the CharSequence interface.
StringBuffer is also a subclass inherited from AbstractStringBuilder;
However, StringBuilder is different from StringBuffer. The former is non thread safe and the latter is thread safe.
common method
|Method description|
| — | — |
|public StringBuffer append(String s) | appends the specified string to this character sequence|
|public StringBuffer reverse() | replace this character sequence with its inverted form|
|public delete(int start, int end) | removes the characters in the substring of this sequence|
|public insert(int offset, int i) | inserts the string representation of the int parameter into this sequence|
|insert(int offset, String str) | insert the string of str parameter into this sequence|
|replace(int start, int end, String str) | replace the characters in the substring of this sequence with the characters in the given String|
class Test { public static void main(String[] args) { StringBuilder sb = new StringBuilder(10); // StringBuffer sb = new StringBuffer(10); sb.append("Test.."); System.out.println(sb); // Test.. sb.append("!"); System.out.println(sb); // Test..! sb.insert(6, "Java"); System.out.println(sb); // Test..Java! sb.delete(4, 6); System.out.println(sb); // TestJava! } }
The usage of StringBuilder is similar to that of StringBuffer.
Differences among String, StringBuilder and StringBuffer:
The first thing to note is:
-
String constant
-
StringBuilder string variable (non thread safe)
-
StringBuffer string variable (thread safe)
In most cases, the three are compared in terms of execution speed: StringBuilder > StringBuffer > string
Summary of the use of the three:
-
If you want to manipulate a small amount of data, use = String
-
Single thread operation large amount of data in string buffer = StringBuilder
-
Multithreaded operation: operate a large amount of data in the string buffer = StringBuffer
All wrapper classes (Integer, Long, Byte, Double, Float, Short) are subclasses of the abstract class Number.
Packaging
|Packaging class | basic data type|
| — | — |
| Boolean | boolean |
| Byte | short |
| Short | short |
| Integer | int |
| Long | long |
| Character | char |
| Float | float |
| Double | double |
usage method
Realize the conversion between int and Integer
class Test { public static void main(String[] args) { int m = 500; Integer obj = new Integer(m); int n = obj.intValue(); System.out.println(n); // 500 Integer obj1 = new Integer(500); System.out.println(obj.equals(obj1)); // true } }
Converts a string to an integer
class Test { public static void main(String[] args) { String[] str = {"123", "123abc", "abc123", "abcxyz"}; for (String str1 : str) { try { int m = Integer.parseInt(str1, 10); System.out.println(str1 + " Can be converted to an integer " + m); } catch (Exception e) { System.out.println(str1 + " Cannot convert to integer"); } } } }
Output results:
123 Can be converted to integer 123 123abc Cannot convert to integer abc123 Cannot convert to integer abcxyz Cannot convert to integer
Converts an integer to a string
class Test { public static void main(String[] args) { int m = 500; String s = Integer.toString(m); System.out.println(s); // 500 String s2 = m + "a"; System.out.println(s2); // 500a } }
Java's Math contains properties and methods for performing basic mathematical operations, such as elementary exponents, logarithms, square roots, and trigonometric functions.
constant value
|Constant description|
| — | — |
| Math.PI | recorded pi|
| Math.E | record the constant of e|
common method
|Method description|
| — | — |
| Math.abs | returns the absolute value of the parameter|
| Math.sin | find the sine of the specified double type parameter|
| Math.cos | find the cosine value of the specified double type parameter|
| Math.tan | find the tangent value of the specified double type parameter|
| Math.toDegrees | converts an angle to radians|
| Math.ceil | get the largest integer not less than a certain number|
| Math.floor | get the maximum integer not greater than a certain number|
| Math.IEEEremainder 𞓜 remainder|
| Math.max | returns the maximum of the two parameters|
| Math.min | returns the minimum of the two parameters|
| Math.sqrt | find the arithmetic square root of the parameter|
| Math.pow | find any power of a number and throw arithmetexception to handle overflow exception|
| Math.exp | returns the parameter power of the base e of a natural number|
| Math.log10 | base 10 logarithm|
| Math.log | returns the logarithm of the natural base of the parameter|
| Math.rint | find the integer nearest to a certain number (which may be larger or smaller than a certain number)|
| Math.round | same as above. It returns int type or long type (the previous function returns double type)|
| Math.random | returns a random number between 0 and 1|
The random() method is used to return a random number in the range of 0.0 = < math random < 1.0.
common method
|Method description|
| — | — |
|protected int next(int bits) | generates the next pseudo-random number|
|boolean nextBoolean() | returns the next pseudo-random number, which is a uniformly distributed boolean value from this random number generator sequence|
|void nextBytes(byte[] bytes) | generate random bytes and place them in the byte array provided by the user|
|double nextDouble() | returns the next pseudo-random number, which is the double value evenly distributed between 0.0 and 1.0 from this random number generator sequence|
|float nextFloat() | returns the next pseudo-random number, which is the float value evenly distributed between 0.0 and 1.0 taken from this random number generator sequence|
|double nextGaussian() | returns the next pseudo-random number, which is the double value in Gaussian ("normal") distribution taken from the random number generator sequence, with an average of 0.0 and a standard deviation of 1.0|
|int nextInt() | returns the next pseudo-random number, which is the evenly distributed int value in the sequence of this random number generator|
|int nextInt(int n) | returns a pseudo-random number, which is an int value taken from this random number generator sequence and evenly distributed between (including) and the specified value (excluding)|
|long nextLong() | returns the next pseudo-random number, which is a uniformly distributed long value taken from this random number generator sequence|
|void setSeed(long seed) | seed this random number generator with a single long seed|
import java.util.Random; class Test { public static void main(String[] args) { System.out.println(Math.random()); // 0.6456063107220328 System.out.println(Math.random()); // 0.579336669972285 Random rand = new Random(); int i = rand.nextInt(100); System.out.println(i); // Generate an integer with a random number of 0-100, excluding 100 } }
java. The util package provides a Date class to encapsulate the current Date and time. The Date class provides two constructors to instantiate a Date object.
common method
|Method description|
| — | — |
|boolean after(Date date) | if the Date object calling this method returns true after the specified Date, otherwise it returns false|
|boolean before(Date date) | if the Date object calling this method returns true before the specified Date, otherwise it returns false|
|int compareTo(Date date) | compare the Date object when this method is called with the specified Date. Returns 0 when the two are equal. The calling object returns a negative number before the specified Date. The calling object returns a positive number after the specified Date|
|int compareTo(Object obj) | if obj is of type Date, the operation is equivalent to compareTo(Date). Otherwise, it throws ClassCastException|
|long getTime() | returns the number of milliseconds represented by this Date object since January 1, 1970, 00:00:00 GMT|
|oid setTime(long time) | set the time and date in milliseconds since January 1, 1970 00:00:00 GMT|
import java.text.SimpleDateFormat; import java.util.Date; class Test { public static void main(String[] args) { Date date = new Date(); System.out.println(date.toString()); // Sat Jul 24 14:44:45 CST 2021 long time1 = date.getTime(); long time2 = date.getTime(); System.out.println(time1 == time2); // true // SimpleDateFormat format format time SimpleDateFormat ft = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss"); System.out.println(ft.format(date)); // 2021-07-24 02:46:37 } }
Calendar class is an abstract class. It implements the objects of specific subclasses in actual use. The process of creating objects is transparent to programmers. You only need to use getInstance method to create objects.
constant value
|Constant description|
| — | — |
| Calendar.YEAR | year|
| Calendar.MONTH | month|
| Calendar.DATE | date|
| Calendar.DAY_OF_MONTH | date has exactly the same meaning as the field above|
| Calendar.HOUR | 12 hour system|
| Calendar.HOUR_OF_DAY | 24-hour system|
| Calendar.MINUTE | minutes|
| Calendar.SECOND | second|
| Calendar.DAY_OF_WEEK | day of week|
import java.util.Calendar; class Test { public static void main(String[] args) { ## summary The interview inevitably makes people anxious. Everyone who has experienced it knows. But it's much easier if you anticipate the questions the interviewer will ask you in advance and come up with appropriate answers. In addition, it is said that "the interview makes a rocket, and the work screws". For friends preparing for the interview, you only need to understand one word: brush! Brush me, brush hard! Since I'm here to talk about the interview today, I have to come to the real interview. It didn't take me 28 days to do a job“ Java Analytical collection of interview questions for senior positions in front-line large factories: JAVA Basics-intermediate-Advanced interview+SSM frame+Distributed+performance tuning +Microservices+Concurrent programming+network+Design pattern+Data structure and algorithm " 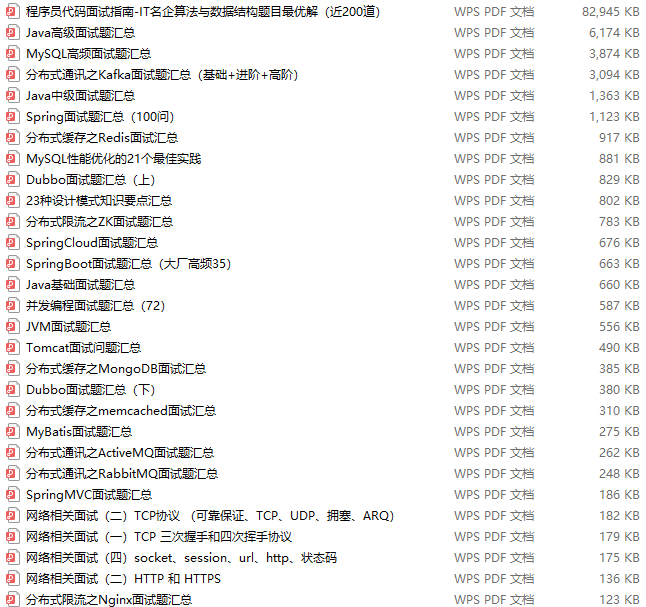 > **[Data collection method: click here to download for free](https://gitee.com/vip204888/java-p7)** In addition to simply brushing questions, you also need to prepare a book[ JAVA Advanced core knowledge manual]: JVM,JAVA Set JAVA Multithreading concurrency JAVA Foundation Spring Principles, microservices Netty And RPC,Network, log Zookeeper,Kafka,RabbitMQ,Hbase,MongoDB,Cassandra,Design pattern, load balancing, database, consistency algorithm JAVA Algorithm, data structure, encryption algorithm, distributed cache Hadoop,Spark,Storm,YARN,Machine learning and cloud computing are best used to check leaks and fill vacancies. | Calendar.MONTH | month | | Calendar.DATE | date | | Calendar.DAY\_OF\_MONTH | Date has exactly the same meaning as the field above | | Calendar.HOUR | 12 Hour in hour system | | Calendar.HOUR\_OF\_DAY | 24 Hour in hour system | | Calendar.MINUTE | minute | | Calendar.SECOND | second | | Calendar.DAY\_OF\_WEEK | What day is today? |
import java.util.Calendar;
class Test {
public static void main(String[] args) {
summary
The interview inevitably makes people anxious. Everyone who has experienced it knows. But it's much easier if you anticipate the questions the interviewer will ask you in advance and come up with appropriate answers.
In addition, it is said that "the interview makes a rocket, and the work screws". For friends preparing for the interview, you only need to understand one word: brush!
Brush me, brush hard! Since I'm here to talk about the interview today, I have to come to the real interview questions. It didn't take me 28 days to do a "collection of analysis of interview questions for high posts in large Java front-line factories: Java foundation - intermediate - Advanced interview + SSM framework + distributed + performance tuning + microservice + concurrent programming + Network + design pattern + data structure and algorithm, etc."
[external chain picture transferring... (img-PK2bmygP-1628143119875)]
Data collection method: click here to download for free
And in addition to simply brushing questions, You also need to prepare a copy [JAVA advanced core knowledge manual]: JVM, JAVA collection, JAVA multithreading concurrency, JAVA foundation, Spring principle, microservice, Netty and RPC, network, log, Zookeeper, Kafka, RabbitMQ, Hbase, MongoDB, Cassandra, design pattern, load balancing, database, consistency algorithm, JAVA algorithm, data structure, encryption algorithm, distributed cache, Hadoop, Spark , Storm, YARN, machine learning and cloud computing are best used to check leaks and fill vacancies.