1. Creation and initialization of one-dimensional array
1.1 creation of array
type_t arr_name [const_n]; //type_t is the element type of the index group //const_n is a constant expression that specifies the size of the array
int main() { /* int a = 1; int b = 2; int c = 3;*/ //Storage 1 ~ 100, array required //An array is a collection of elements of the same type int arr[100]={1,2,3,4,5,6,7,8,9,10}; int arr2[100] = { 0 }; return 0; }
1.2 initialization of array
The initialization of an array refers to giving some reasonable initial values (initialization) to the contents of the array while creating the array
int arr1[10] = { 1,2,3,4 };//Incomplete initialization int arr2[] = { 1,2,3,4 };//Specifies the size of the array based on the contents of the root array char ch1[] = { 'a','b','c' }; char ch2[] = { 'a',98,'c' };//The two are the same because the ascii value of b is equal to 98
When creating an array, if you want to not specify the determined size of the array, you have to initialize it. The number of elements of the array is determined according to the initialization content. But for the following code, we should distinguish how to allocate memory.
char arr1[] = "abc";//a b c \0 char arr2[] = { 'a','b','c' };//a b c
1.3 use of one-dimensional array
#include <stdio.h> int main() { int arr[100] = { 1,2,3,4,5,6 }; // 0 1 2 3 4 5 //Write code to assign a value of 1 ~ 100 //printf("%d\n", sizeof(arr));//400 //printf("%d\n", sizeof(arr[0]));//4 int sz = sizeof(arr) / sizeof(arr[0]);//How to calculate the number of array elements int i = 0; //assignment for (i = 0; i < sz; i++) { arr[i] = i + 1; } //Print for (i = 0; i < sz; i++) { printf("%d ", arr[i]); } return 0; }
int arr[10]; int sz = sizeof(arr)/sizeof(arr[0]);
1.4 storage of one-dimensional array in memory
//Storage of one-dimensional array in memory #include <stdio.h> int main() { int arr[10] = { 1,2,3,4,5,6,7,8,9,10 }; //Prints the address of each element of the array int i = 0; int sz = sizeof(arr) / sizeof(arr[0]); for (i = 0; i < sz; i++) { printf("&arr[%d]=%p\n", i, &arr[i]); } return 0; }
The output results are as follows:
Carefully observe the output results. We know that with the increase of array subscript, the address of the element is also increasing regularly. It can be concluded that arrays are stored continuously in memory.
//Storage of one-dimensional array in memory #include <stdio.h> int main() { int arr[10] = { 1,2,3,4,5,6,7,8,9,10 }; //Prints the address of each element of the array int i = 0; int sz = sizeof(arr) / sizeof(arr[0]); int* p = &arr[0]; for (i = 0; i < sz; i++) { printf("%d ", *(p + i)); } /*for (i = 0; i < sz; i++) { printf("&arr[%d]=%p<====> %p\n", i, &arr[i],p+i); }*/ return 0; } //%p-print address (hexadecimal) //%d - print integer (decimal) //Arrays can be accessed in the form of subscripts or pointers
2. Creation and initialization of two-dimensional array
2.1 creation of two-dimensional array
//Array creation int arr[3][4]; char arr[3][5]; double arr[2][4];
2.2 initialization of two-dimensional array
//Array initialization int arr[3][4] = {1,2,3,4};//Incomplete initialization int arr[3][4] = {{1,2},{4,5}}; int arr[][4] = {{2,3},{4,5}};//If the two-dimensional array is initialized, the row can be omitted and the column cannot be omitted
2.3 use of two-dimensional array
#include <stdio.h> int main() { int arr2[][5] = { {1,2},{4,5},{5,6} }; int i = 0; for (i = 0; i < 3; i++) { int j = 0; for (j = 0; j < 5; j++) { printf("%d ", arr2[i][j]); } printf("\n"); } return 0; }
#include <stdio.h> int main() { int arr2[][5] = { {1,2},{4,5},{5,6} }; int i = 0; for (i = 0; i < sizeof(arr2)/sizeof(arr2[0]); i++) { int j = 0; for (j = 0; j < sizeof(arr2[0])/sizeof(arr2[0][0]); j++) { printf("%d ", arr2[i][j]); } printf("\n"); } return 0; }
2.4 storage of two-dimensional array in memory
#include <stdio.h> int main() { int arr[3][5] = { {1,2},{4,5},{5,6} }; int i = 0; for (i = 0; i < 3; i++) { int j = 0; for (j = 0; j < 5; j++) { printf("&arr[%d][%d]=%p\n", i, j, &arr[i][j]); } } return 0; }
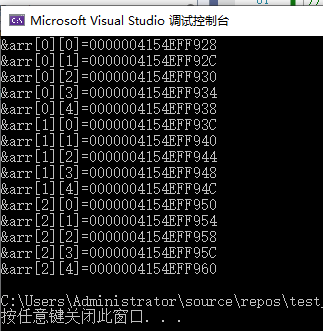
3. Array out of bounds
#include <stdio.h> int main() { int arr[10] = {1,2,3,4,5,6,7,8,9,10}; int i = 0; for(i=0; i<=10; i++) { printf("%d\n", arr[i]);//When i equals 10, the cross-border access } return 0; }
4. Array as function parameter
4.1 wrong design of bubble sorting function
//Method 1 #include <stdio.h> void bubble_sort(int arr[])//Array receiving { int sz = sizeof(arr) / sizeof(arr[0]); //Number of trips int i = 0; for (i = 0; i < sz - 1; i++) { //Each bubble sorting process int j = 0; for (j = 0; j <sz-1-i ; j++) { if (arr[j] > arr[j + 1]) { //exchange int tmp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = tmp; } } } } int main() { int arr[] = { 9,8,7,6,5,4,3,2,1,0 }; //Write a bubble sort function to sort the contents of the arr array // arr - the address of the first element of the // &arr[0] //Write a bubble sort function to sort the contents of the arr array //Bubble sort: compare two adjacent elements in the array. If they do not meet the conditions, they will be exchanged. The sort is ascending bubble_sort(arr); int i = 0; int sz = sizeof(arr) / sizeof(arr[0]); for (i = 0; i < sz; i++) { printf("%d ", arr[i]); } return 0; }
4.2 what is the array name?
#include <stdio.h> int main() { int arr[10] = { 1,2,3,4,5,6,7,8,9,10 }; printf("%p\n", arr); printf("%p\n", arr + 1); printf("%p\n", &arr[0]); printf("%p\n", &arr[0] + 1); printf("%p\n", &arr); printf("%p\n", &arr + 1); //Output results return 0; }
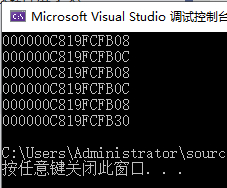
4.3 correct design of bubble sorting function
#include <stdio.h> void bubble_sort(int arr[],int sz)//Array receiving { //Number of trips int i = 0; for (i = 0; i < sz - 1; i++) { int flag = 1;//The assumption has been ordered //Each bubble sorting process int j = 0; for (j = 0; j <sz-1-i ; j++) { if (arr[j] > arr[j + 1]) { flag = 0; //exchange int tmp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = tmp; } } if (1 == flag) { break; } } } int main() { int arr[] = { 9,8,7,6,5,4,3,2,1,0 }; //Write a bubble sort function to sort the contents of the arr array // arr - the address of the first element of the // &arr[0] //Write a bubble sort function to sort the contents of the arr array //Bubble sort: compare two adjacent elements in the array. If they do not meet the conditions, they will be exchanged. The sort is ascending int sz = sizeof(arr) / sizeof(arr[0]); bubble_sort(arr,sz); int i = 0; for (i = 0; i < sz; i++) { printf("%d ", arr[i]); } return 0; }