I. overview of HashMap;
1.HashMap is a hash table, which stores key value mapping;
2.HashMap inherits AbstractMap and implements Map, Cloneable and Serializable interfaces;
3. The implementation of HashMap is not synchronous and the thread is not safe, but it is efficient;
4.HashMap allows null key and null value, which is based on the Map interface of hash table;
5. Hash table is used to ensure the uniqueness of key;
6. The instance of HashMap has two parameters that affect its performance: initial test capacity and load factor. When the number of entries in the hash table exceeds the product of load factor and current capacity, rehash the hash table (that is, rebuild the internal data structure). The capacity expansion is about twice of the previous one, and the default value of load factor is 0.75;
Second, three traversal methods of HashMap;
The first one: traversing the set of entrySet key value pairs of HashMap
1. Get the set of key value pairs through HashMap.entrySet();
2. The key value and value value are obtained by Iterator traversing the key value pair set;
package com.xyfer; import java.util.HashMap; import java.util.Iterator; import java.util.Map; public class HashMapTest { public static void main(String[] args) { // Create a key and value All are String Of Map aggregate Map<String, String> map = new HashMap<String, String>(); map.put("1", "11"); map.put("2", "22"); map.put("3", "33"); // Key and value String key = null; String value = null; // Get iterator of key value pair Iterator it = map.entrySet().iterator(); while (it.hasNext()) { Map.Entry entry = (Map.Entry) it.next(); key = (String) entry.getKey(); value = (String) entry.getValue(); System.out.println("key:" + key + "---" + "value:" + value); } } }
Console print results:
The second is to traverse the Set set Set of the HashMap key to get the value;
1. Get the Set set of keys through HashMap.keySet();
2. Traversing the Set set of the key to get the value;
package com.xyfer; import java.util.HashMap; import java.util.Iterator; import java.util.Map; public class HashMapTest { public static void main(String[] args) { // Create a key and value All are String Of Map aggregate Map<String, String> map = new HashMap<String, String>(); map.put("1", "11"); map.put("2", "22"); map.put("3", "33"); // Key and value String key = null; String value = null; // Get iterator of key set Iterator it = map.keySet().iterator(); while (it.hasNext()) { key = (String) it.next(); value = (String) map.get(key); System.out.println("key:" + key + "---" + "value:" + value); } } }
Console print results:
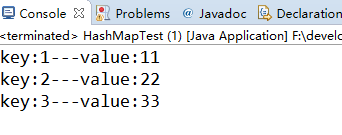
The third is to traverse the set of HashMap "values";
1. Get the set of "values" through HashMap.values()
2. Traversing the set of "values";
package com.xyfer; import java.util.HashMap; import java.util.Iterator; import java.util.Map; public class HashMapTest { public static void main(String[] args) { // Create a key and value All are String Of Map aggregate Map<String, String> map = new HashMap<String, String>(); map.put("1", "11"); map.put("2", "22"); map.put("3", "33"); // value String value = null; // Get iterator of value set Iterator it = map.values().iterator(); while (it.hasNext()) { value = (String) it.next(); System.out.println("value:" + value); } } }
Console print results: