1, Environment introduction
MCU model: STC89C52
Programming software: keil5
Programming language: C language
Mobile APP: QT design is adopted, and the program supports cross platform compilation and operation (Android, IOS, Windows and Linux can be compiled and run, corresponding to the establishment of QT environment on the platform, which has been explained in the blog before)
Full source code download address: https://download.csdn.net/download/xiaolong1126626497/19766960
The information package contains: 51 MCU source code, Android mobile APP source code, executable file apk, various alarm sounds, description documents and download tools.
2, Function and hardware introduction
The single chip microcomputer adopts STC89C52, which is very rich in data. keil can choose AT89C52 when building a project.
Bluetooth for communication with mobile phone: HC05 serial port Bluetooth.
Function introduction:
The anti loss function mainly depends on Bluetooth to judge whether it has been disconnected.
1. After the development board is reset, if the Bluetooth is not connected, the buzzer will alarm after 5 seconds
2. Once Bluetooth is successfully connected, it needs to send the specified data to the device at the frequency of 300ms to keep it alive.
3. If no data is sent to the device after Bluetooth connection, the buzzer will alarm after 300ms.
4. You can manually click on the app to trigger the alarm
5. The MCU can clear the alarm by pressing the reset key
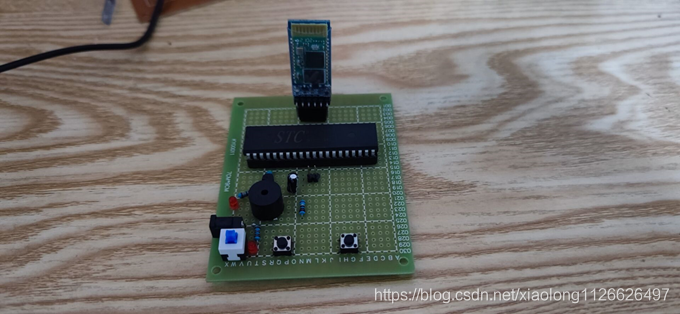
APP interface:
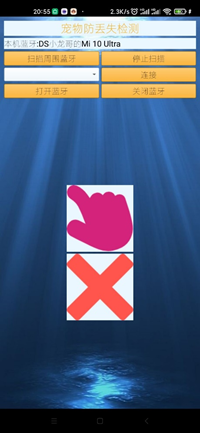
If you don't want to weld and want to build a modular environment directly, you can buy the system board + module directly on Taobao:
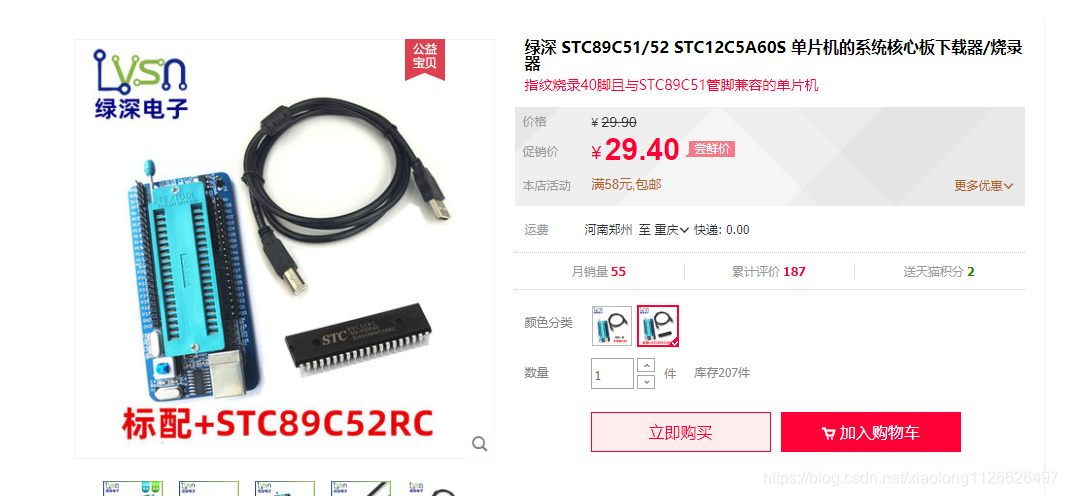
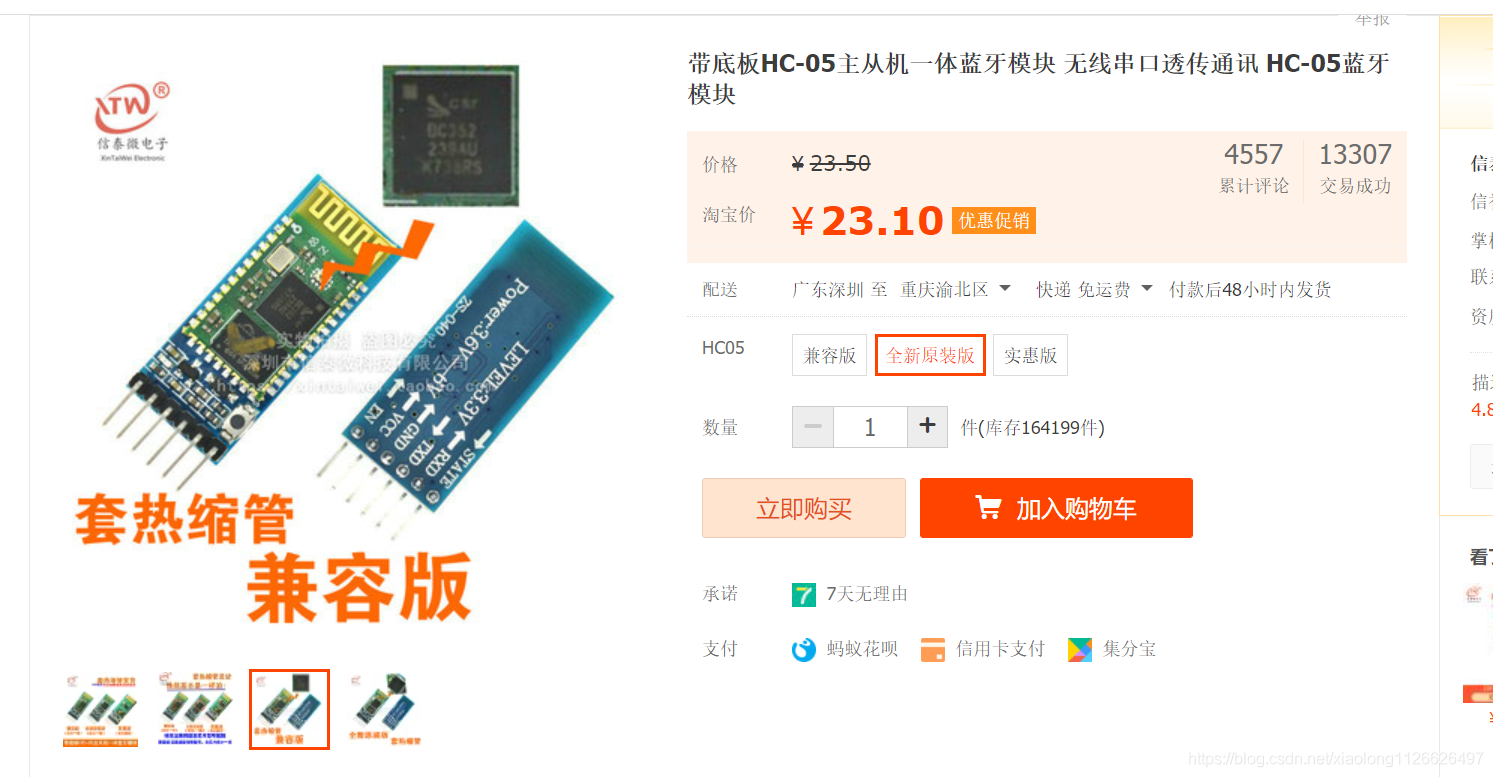
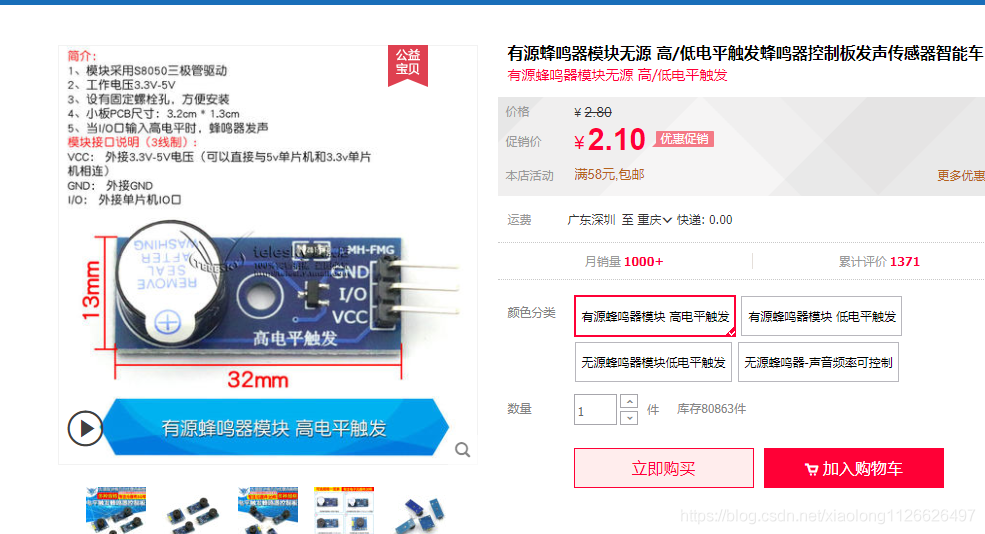
Bluetooth HC05 is a master-slave Bluetooth serial port module. In short, when the Bluetooth device is successfully paired and connected with the Bluetooth device, we can ignore the internal communication protocol of Bluetooth and directly use Bluetooth as a serial port. When a connection is established, the two devices use the same channel, that is, the same serial port. One device sends data to the channel, and the other device can receive the data in the channel.
In order to simplify the programming code of 51 single chip microcomputer, you can first use the computer serial port debugging assistant to configure the parameters of HC05, and then connect it to 51 single chip microcomputer. Configure HC05 Bluetooth to slave mode and set the pairing password.
3, 51 MCU code
#include <REG52.H> #define uchar unsigned char #define uint unsigned long uchar buffer[8]; //Data cache array uchar rec_flag=0; //Data processing flag sbit BUZZER = P1^3; sbit KEY = P3^4; void delay1ms(void) //Error 0us { unsigned char a,b,c; for(c=1;c>0;c--) for(b=142;b>0;b--) for(a=2;a>0;a--); } void Delay(int ms) { while(ms--) { delay1ms(); } } /************************************************ ** Function name: void initiate (void) ** Function: serial port initialization function ** Input: None ** Output: None ** Note: timer 1 mode 2 baud rate generation mode, baud rate 9600bps, crystal oscillator 11.0592MHZ, error 0% **TMOD Timer counter register, SCON serial port register, PCON power control register, EA access external program memory control signal, ES serial port interrupt permission control bit, TR1 timer 1 operation control bit ************************************************/ void InitUART(void) { TMOD = 0x20; SCON = 0x50; TH1 = 0xFD; TL1 = 0xFD; PCON = 0x00; ES = 1; TR1 = 1; EA = 1; } /********************************************* **Name: void TxChar(uchar ch) **Function: serial port sending function **Enter: uchar ch > currently sent data **Output: None *********************************************/ void TxChar(unsigned char ch) { SBUF=ch; while(!TI); TI=0; } void putstring(uchar *puts) //send data { for(;*puts!=199;puts++) //Stop character 199 end encountered TxChar(*puts); } /************************************************ ** Function name: void ser(void) interrupt 4 ** Function function: serial port receiving interrupt function ** Input: None ** Output: None ** Description: process the data string sent from the serial port ************************************************/ void ser(void) interrupt 4 { static uchar i; if(RI==1) { RI = 0; if(rec_flag==0) { if(SBUF==0xff) { rec_flag=1; i=0; } } else { if(SBUF==0xff) { rec_flag=0; if(i==3) { //Communication_Decode(); } i=0; } else { buffer[i]=SBUF; i++; } } } } /************************************************ ** Function name: void main(void) ** Function function: main function ** Input: None ** Output: None Pet detection ** explain: ************************************************/ void main(void) { uint STOP=0,a=0,a1=0; uchar bueezr_flag=0,bueezr_count=0,buzzer_time=3;//Buzzer alarm variable uchar rxbuf[9]; InitUART(); rxbuf[0]='A'; rxbuf[1]=25; rxbuf[2]='B'; rxbuf[3]=99; rxbuf[4]='C'; rxbuf[5]=99; rxbuf[6]='D'; rxbuf[7]=99; rxbuf[8]=199; while(1) { putstring(rxbuf);//Send data function Delay(300); //Delay 300ms if(KEY==0 && !bueezr_flag){while(KEY==0);rxbuf[1]=22;bueezr_flag=1;} if(KEY==0 && bueezr_flag){while(KEY==0);rxbuf[1]=11;bueezr_flag=0;a=0;BUZZER=1;} if(!a)STOP++; //FF 00 00 01 FF // //Judge the data issued by APP if(buffer[2]==0x01) //Cancel buzzer { STOP=0; a1=1; buffer[2]=0x00; } //The data sent by APP has not been received for 15 300ms, and the alarm continues if(STOP>15 && a1) { bueezr_flag=1; } if(buffer[1]==0x01) //Manual mode alarm { bueezr_flag=1;//The buzzer rings directly a=1; //Manual mode flag bit } /*******Buzzer alarm********/ bueezr_count++;if(bueezr_count>buzzer_time*10)bueezr_count=buzzer_time+1; if(bueezr_count%buzzer_time==0 && bueezr_flag) { BUZZER=~BUZZER;//The buzzer reverses and gives a sound prompt } } }
4, Android mobile APP code
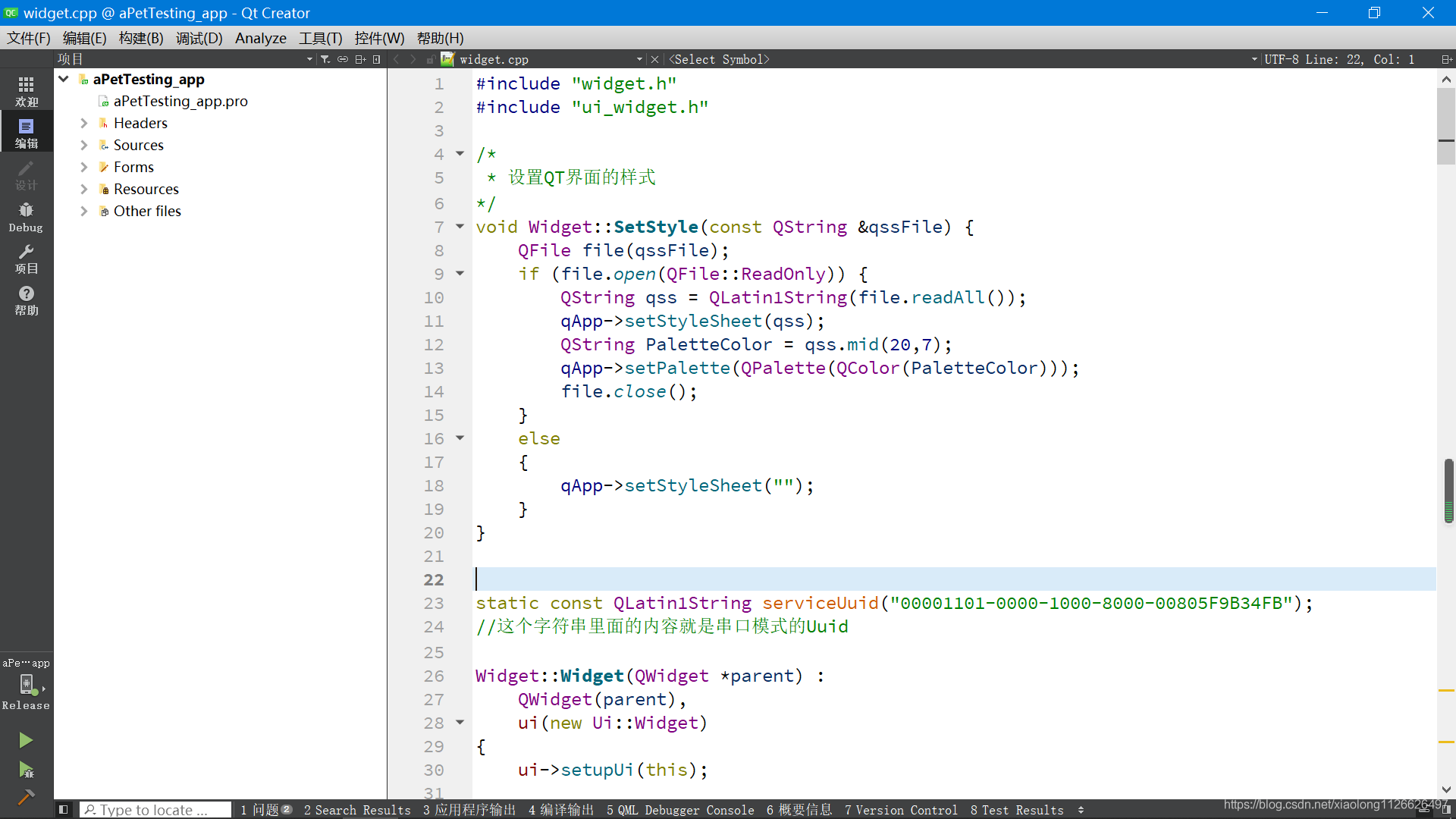
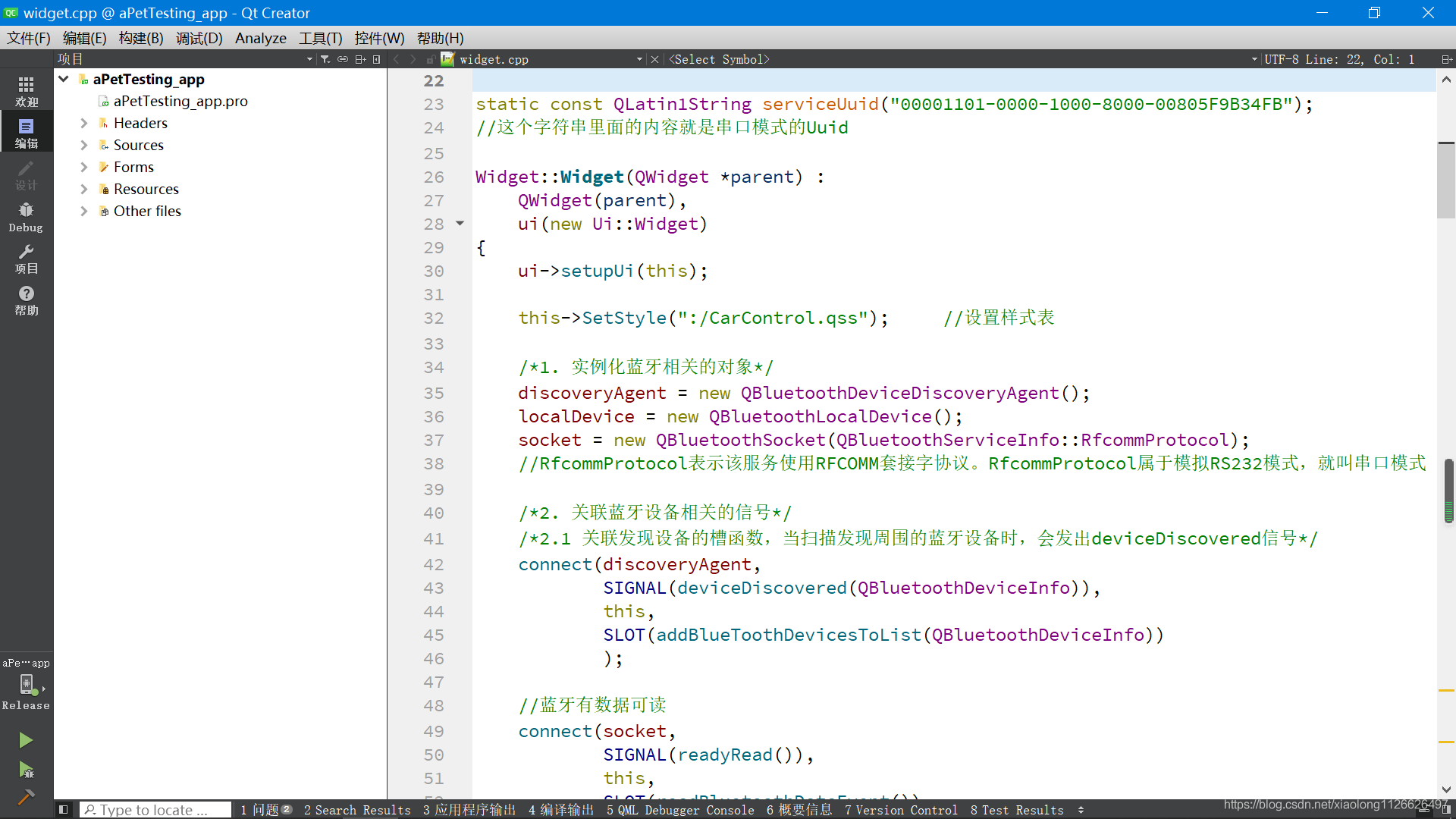
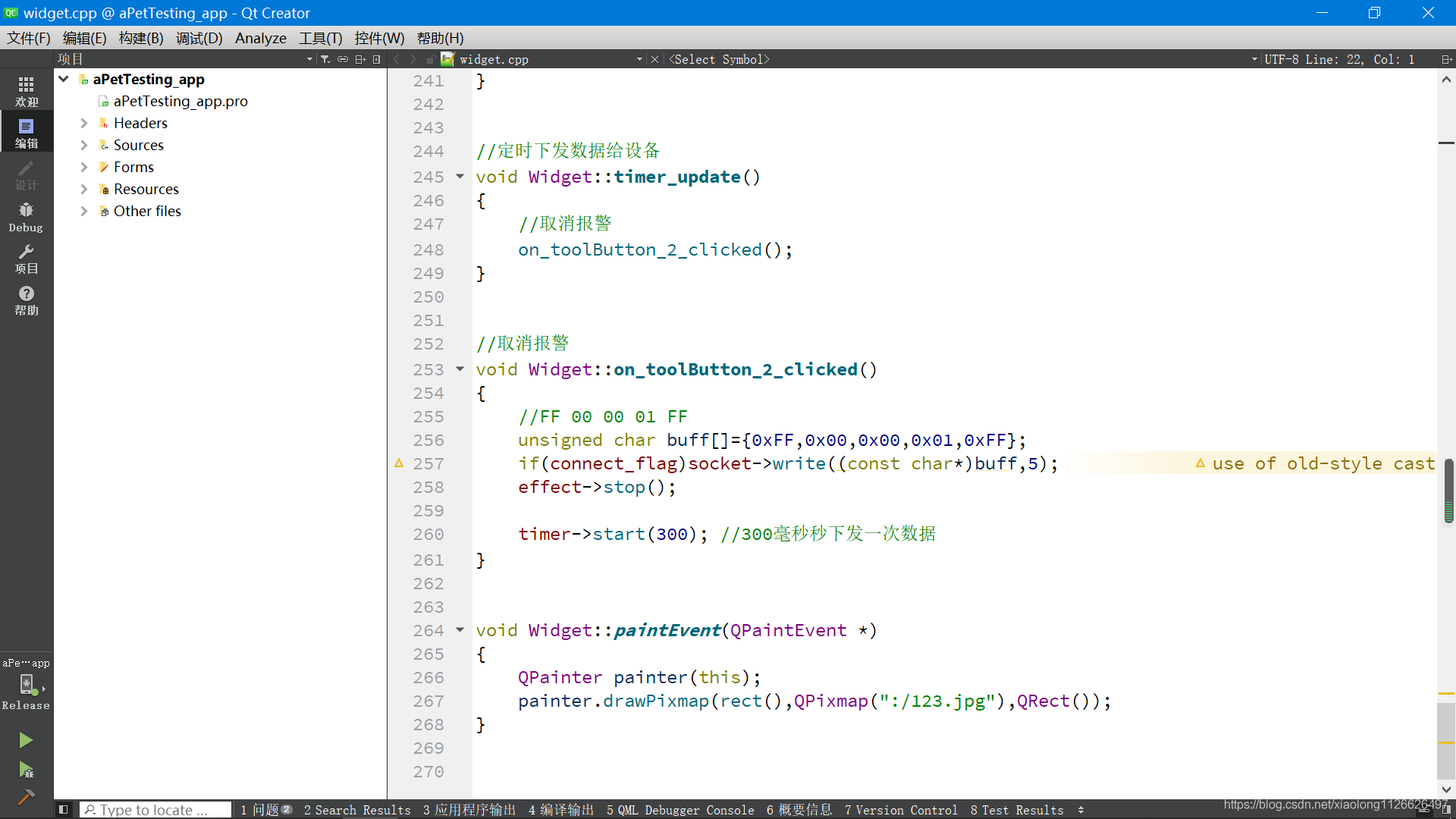
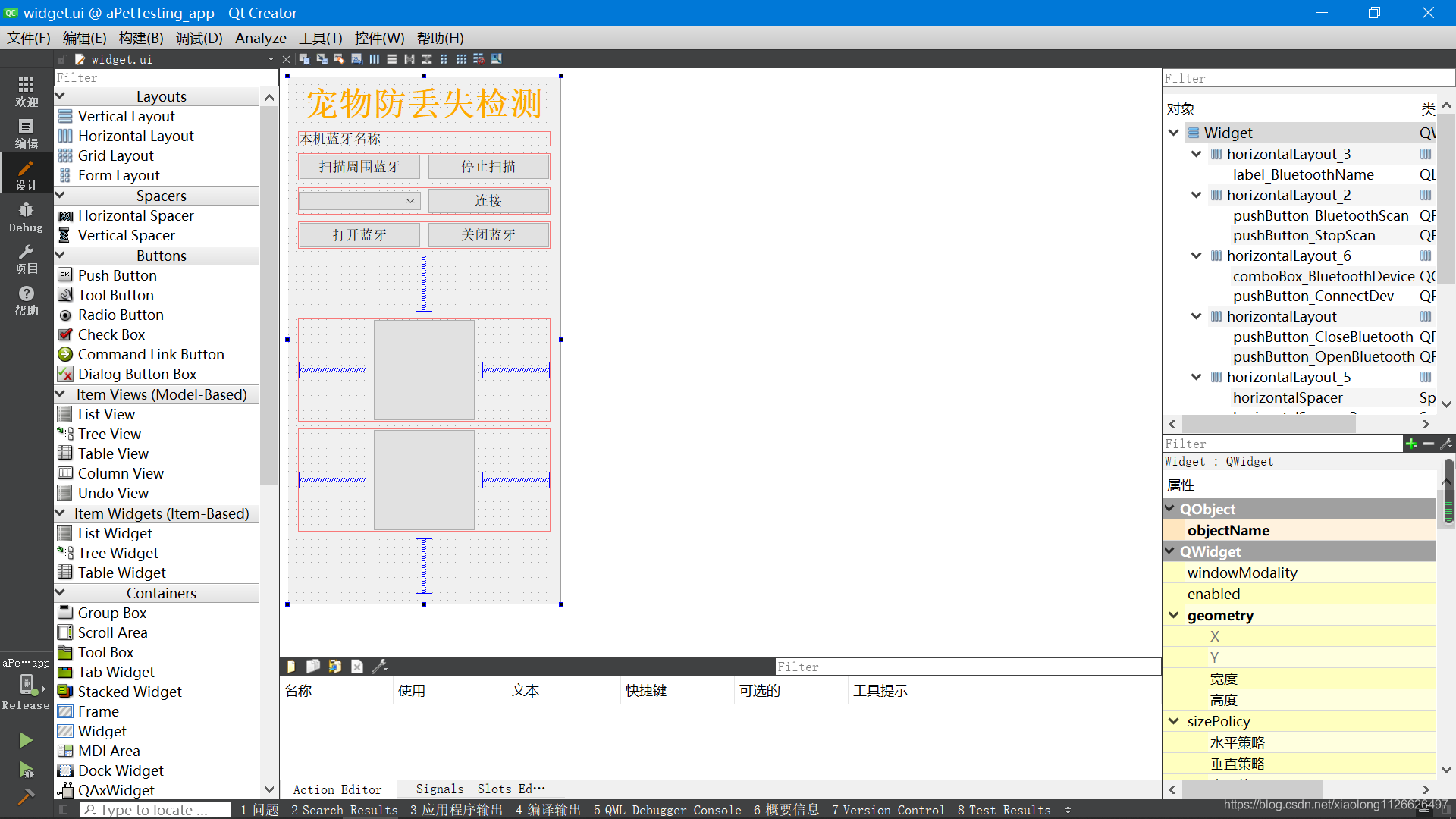