Transformation of demand message center
The shopping center business here currently has more than 70 million users, and the news is about two or three million a day. The old business system may not be good enough for various reasons. Recently, the leaders want to transform the information center Before the development, consider the development of the first official account. Consider a relatively small problem, the channel problem. At present, the news is mainly pushed to several channels, such as APP, public address, H5, short message and so on. Here we must consider how each situation is sent, and there will be various channels from behind, so we should consider expansibility. Here, the policy mode is used to judge a large number of if else switches in provinces and regions
demo:
Message entity
/** * @description: news * @author: zyh * @create: 2021-06-09 18:56 **/ @Data public class MsgEntity { private final String msgType; private final String openId; private final String name; }
Abstract policy class
public interface CustomerStrategyAbstract { void pushStrategy(MsgEntity msg); }
Two strategies
short message
public class SmsPushStrategy implements CustomerStrategyAbstract{ @Override public void pushStrategy(MsgEntity msg) { System.out.println(String.format("adopt openid:%s ,Send SMS to: %s",msg.getOpenId(),msg.getName())); } }
WeChat:
public class WeiXinPushStrategy implements CustomerStrategyAbstract { @Override public void pushStrategy(MsgEntity msg) { System.out.println(String.format("adopt openid:%s ,Push wechat messages to: %s",msg.getOpenId(),msg.getName())); } }
Processing class
public class MsgDeal { //Here, the channel can maintain an enumeration type; /** * SMS channel */ private static String sms="sms"; /** * Wechat push channel */ private static String weixin="weixin"; // The first way to write /** * Cache all policies, which are currently stateless, and can share policy class objects */ private static final Map<String, CustomerStrategyAbstract> strategies = new HashMap<>(4); static { strategies.put(sms, new SmsPushStrategy()); strategies.put(weixin, new WeiXinPushStrategy()); } public static CustomerStrategyAbstract getPushStrategy_1(MsgEntity msg) { final String type = msg.getMsgType(); if (type == null || type.isEmpty()) { throw new IllegalArgumentException("type should not be empty."); } return strategies.get(type); } //The second way to write public static CustomerStrategyAbstract getPushStrategy(MsgEntity msg){ if (sms.equals(msg.getMsgType())){ return new SmsPushStrategy(); }else if (weixin.equals(msg.getMsgType())){ return new WeiXinPushStrategy(); } return null; } //The third way is to write the same prefix for the three channels, and write the channel name for the suffix, and then put the names of the three channels into the bean container, such as modelprovider_ 1,ModelProvider_ 2,ModelProvider_ three public static CustomerStrategyAbstract getPushStrategy(MsgEntity msg){ return applicationContext.getBean("ModelProvider_" + msg.getMsgType(), CustomerStrategyAbstract.class); } }
Test:
public class MsgPushStrategyTest { public static void main(String[] args) { final MsgEntity msgEntity = new MsgEntity("weixin", "123897123", "zyh"); CustomerStrategyAbstract pushStrategy = MsgDeal.getPushStrategy(msgEntity); Optional.ofNullable(pushStrategy).ifPresent(item->{ pushStrategy.pushStrategy(msgEntity); }); } }
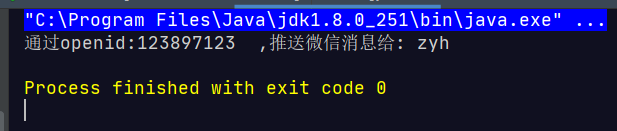
test result
summary
- At the request side, we can't see so many if else judgments. We just need to pass in the corresponding policy method to achieve the idea of behavior decoupling. If we want to add channels, add a class to write logic in it, and add a processing channel in the deal class
- In our common source code, for example, the rejection policy of thread pool is a policy mode, arrays Sort () requires a sort policy type and a policy pattern to be passed in. Comparator acts as an abstract policy role, while specific sub implementation classes act as specific policy roles.
- In addition: we generally deal with situations that avoid a large number of classifications Factory mode , the difference between the two is that one is the behavior pattern and the other is the creation pattern
- Factory pattern is a kind of creation design pattern, which is mainly used to create different objects for different types to achieve decoupling objects.
- Strategy mode is a behavioral design mode, which mainly makes corresponding behaviors for different strategies to achieve behavior decoupling