Welcome to my column [General Knowledge of Artificial Intelligence]
More related articles Click June 2019 as Topic
Can children learn programming?I'm not talking about programming like Scratch, but really writing code, C++.
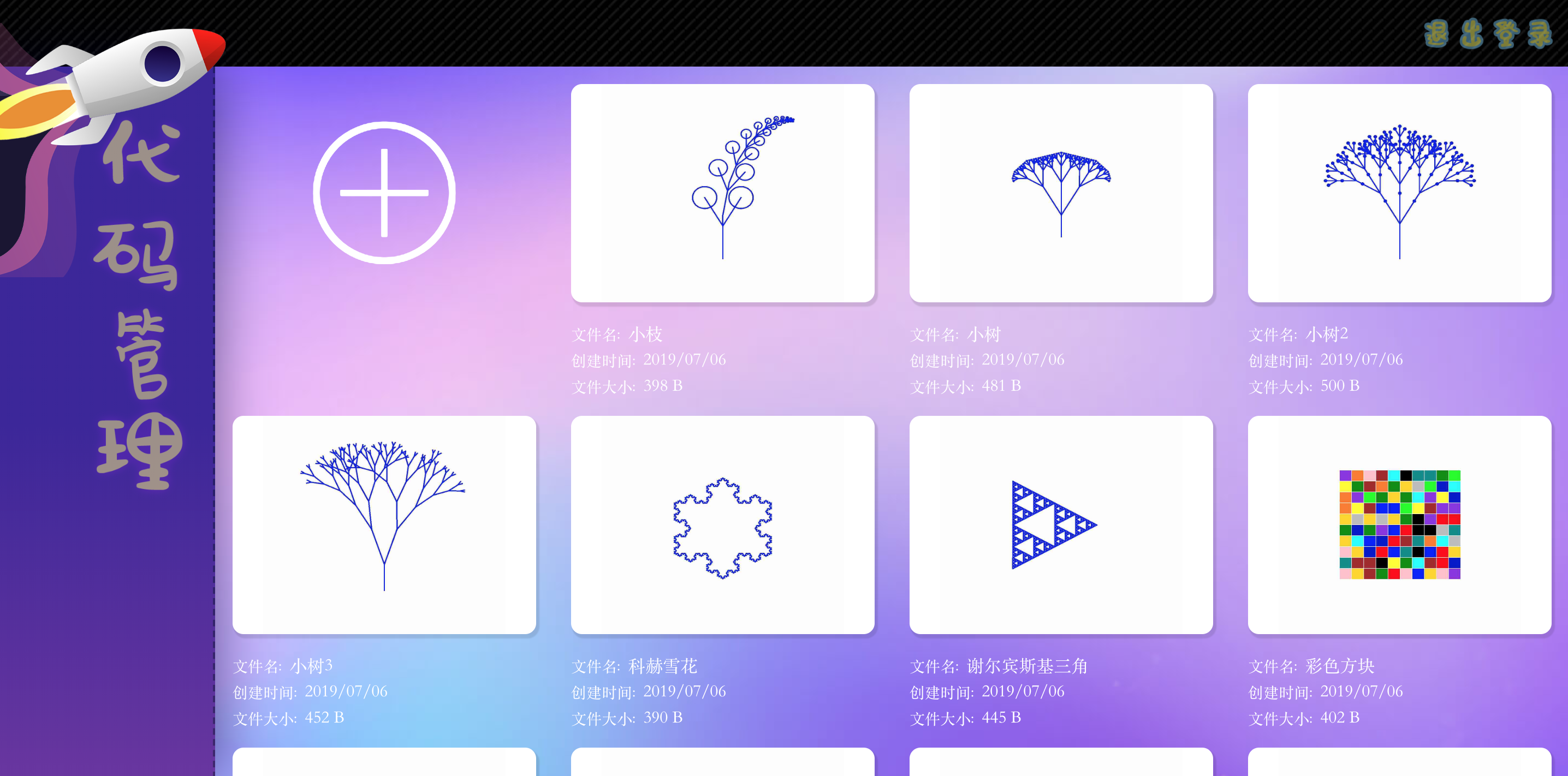
About GoC programming
GoC is an extension of the C language and an interesting programming introductory language developed specifically for elementary and middle school students.
GoC was developed by Jiang Tao, who has been engaged in information teaching in primary and secondary schools for more than 30 years. He is also one of the most famous Olympic informatics coaches in China.
Go C is characterized by its simplicity and preservation of the full grammatical structure of C/C++.
With regard to GoC programming, Taobao can purchase the book Fantastic Journey to the Magic Academy written by Mr. Jiang Tao, which is interesting and easy to learn.
About the Trainer website
hourofcode.cn, College of Trainers
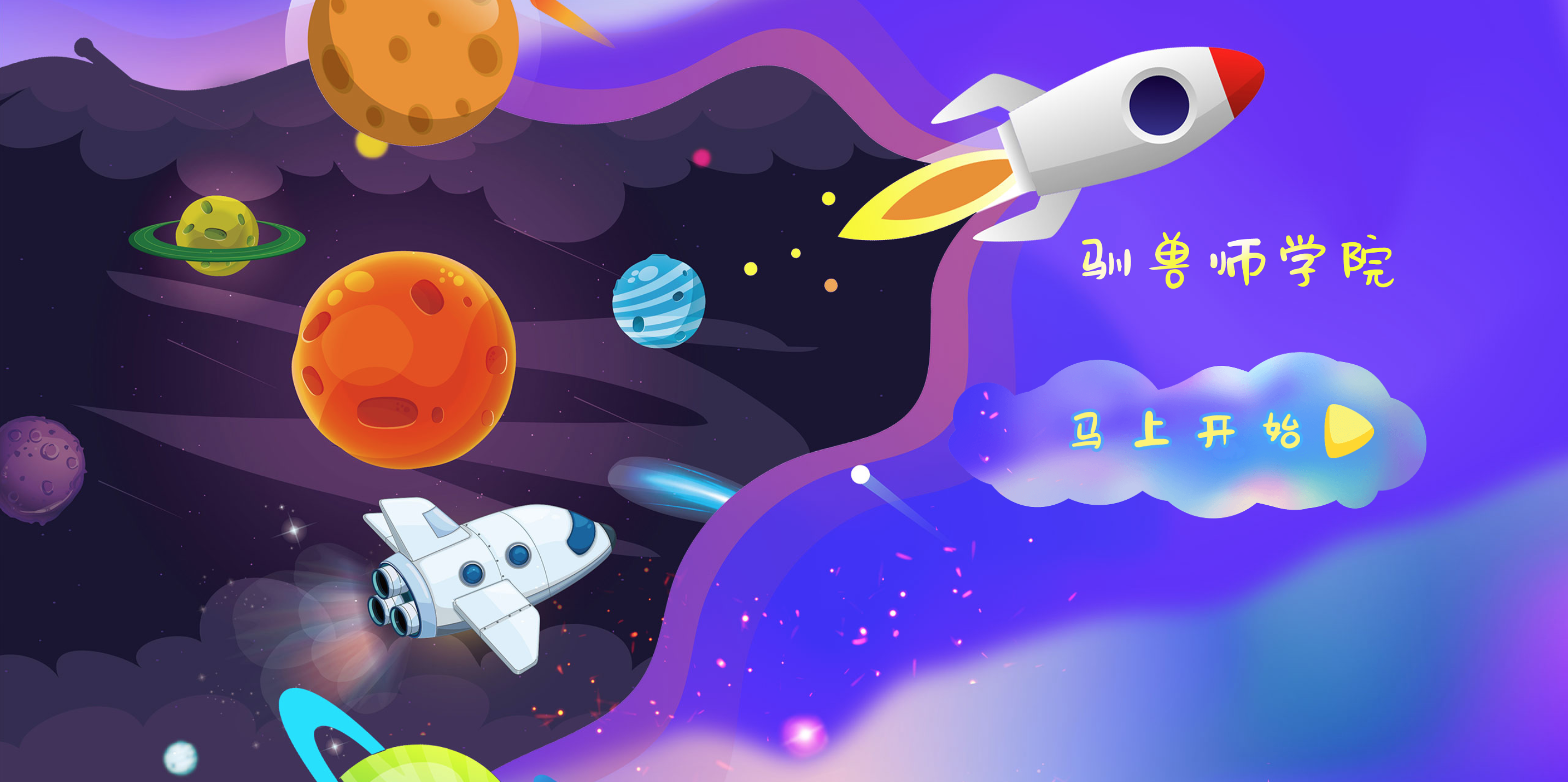
This is an online learning platform based on the GoC language Web version of Jiang Tao Teacher. It is simple and focused on enabling young children to quickly get started with GoC programming and get to touch with real code concepts.
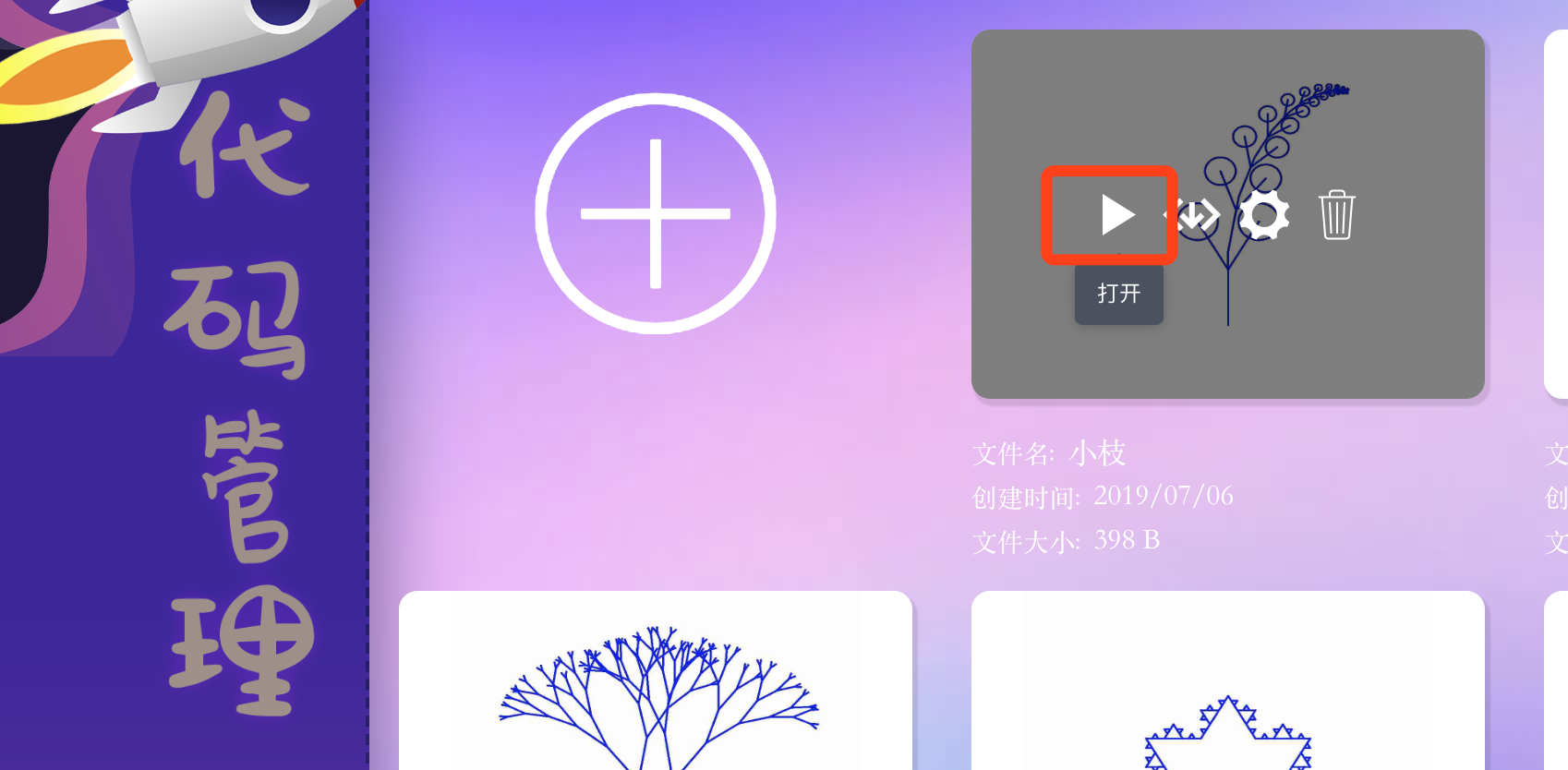
Use process:
-
Sign in and start right away.
-
Code management, new file.
-
Open the file and write the code.
-
Click Run to see the results.
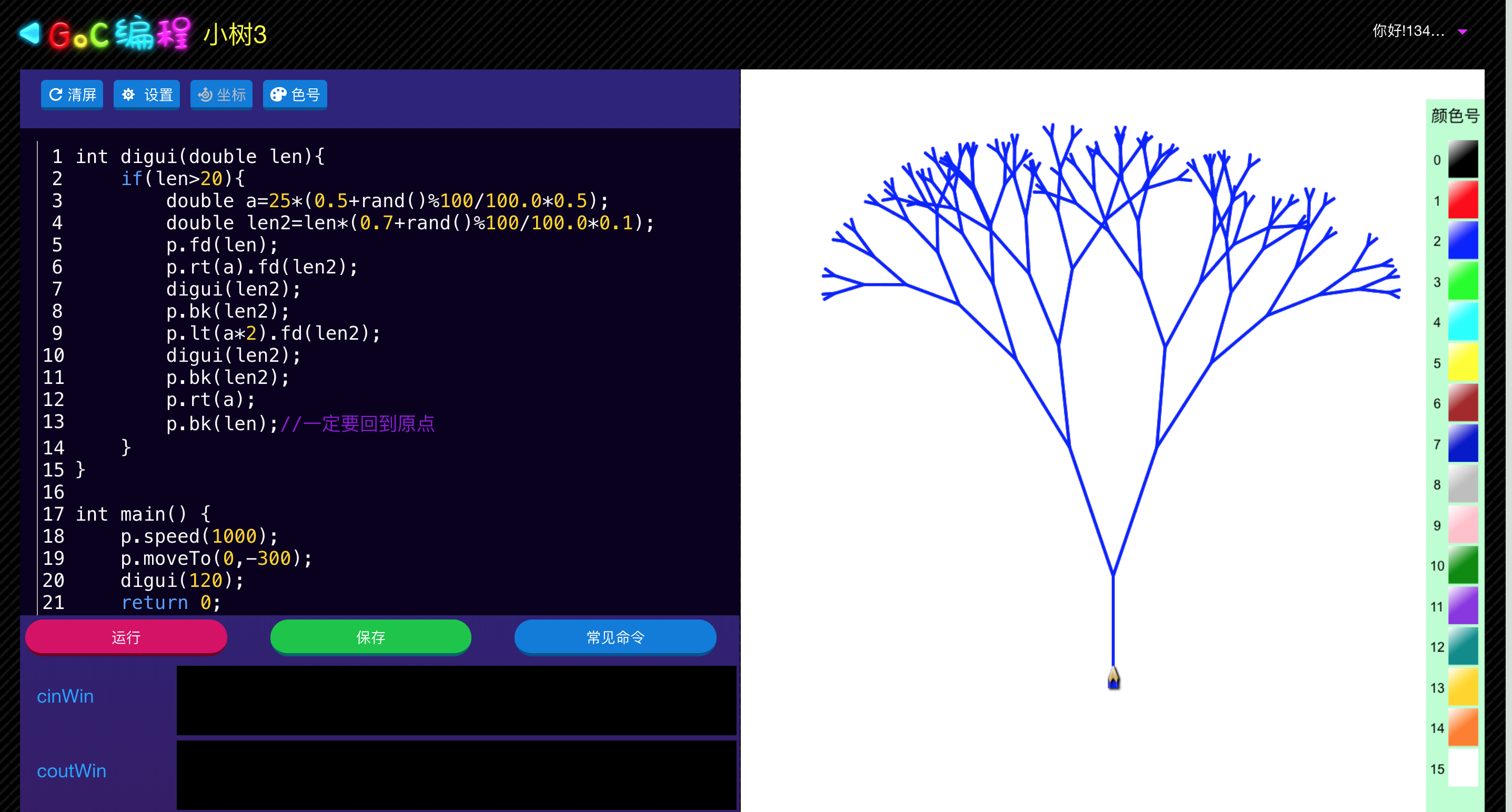
Click the Save button to save the current code, or click View Commands to browse common commands.
The purpose of learning programming is to domesticate the computer, control the computer, master the computer, and use the computer as a powerful tool to enhance the learning, cognitive and logical abilities of adolescents.
Here are some simple introductory cases. If you and your child do not have a formal programming foundation, it is recommended that you refer to the Magic Academy's Magic Trip for learning and practice.
Video tutorials are currently being developed on the Trainer College website and will be available soon, free of charge.
The sum of the outer angles of the polygon is 360 degrees
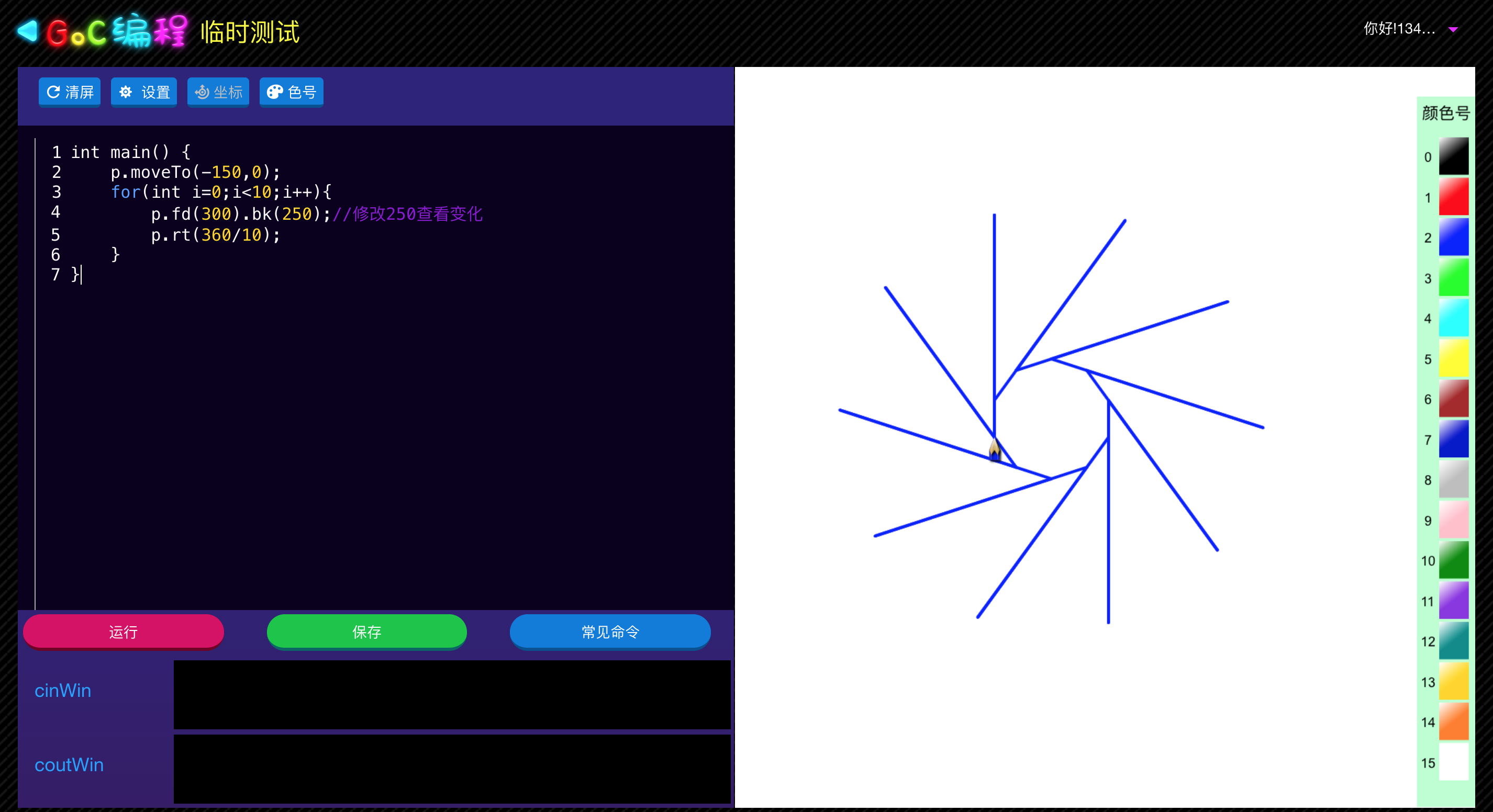
int main() { p.moveTo(-150,0); for(int i=0;i<10;i++){ p.fd(300).bk(250);//Modify 250 to see changes p.rt(360/10); } }
Draw Pentagon
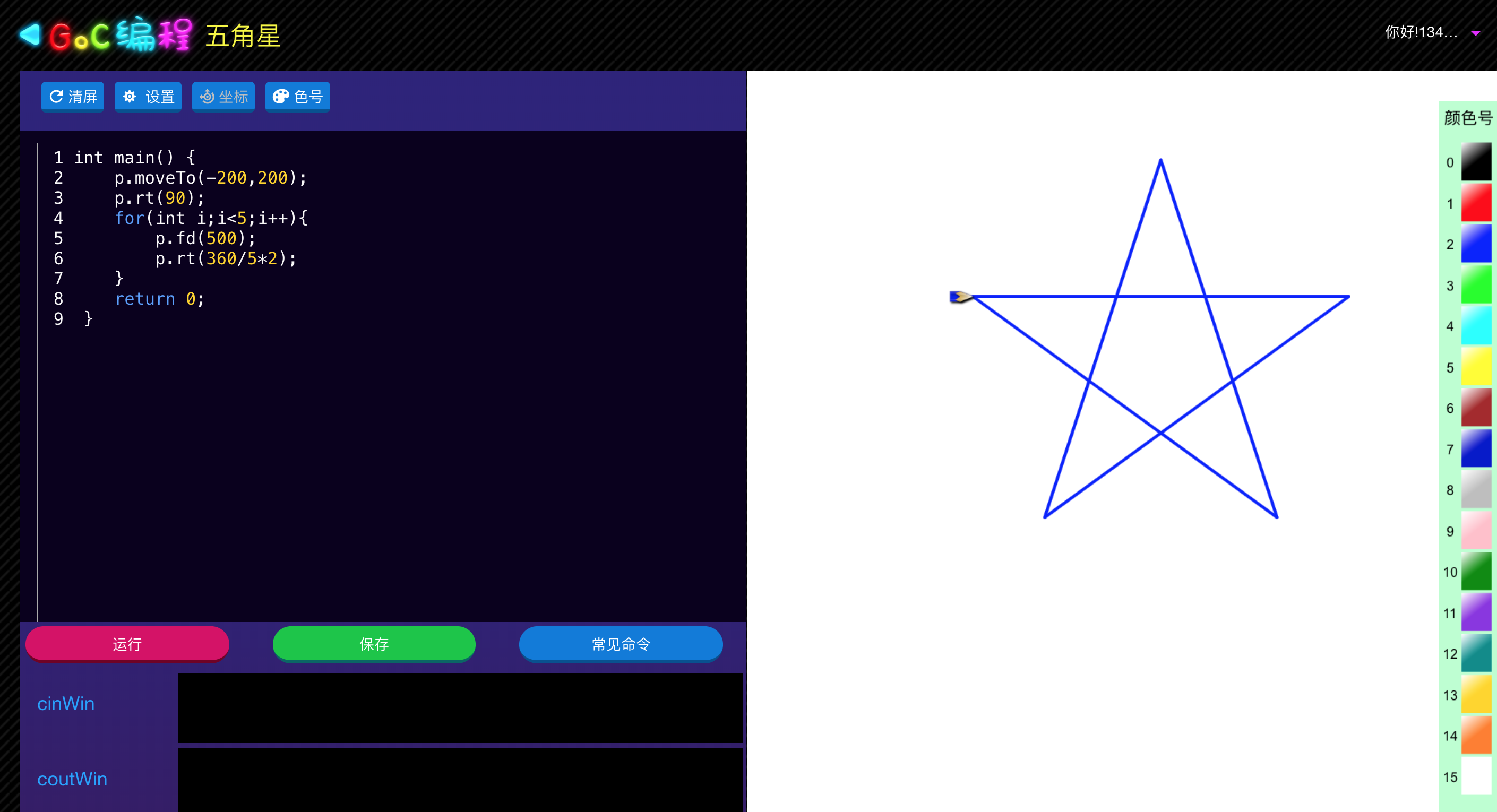
int main() { p.moveTo(-200,200); p.rt(90); for(int i;i<5;i++){ p.fd(500); p.rt(360/5*2); } return 0; }
Draw a polygon star
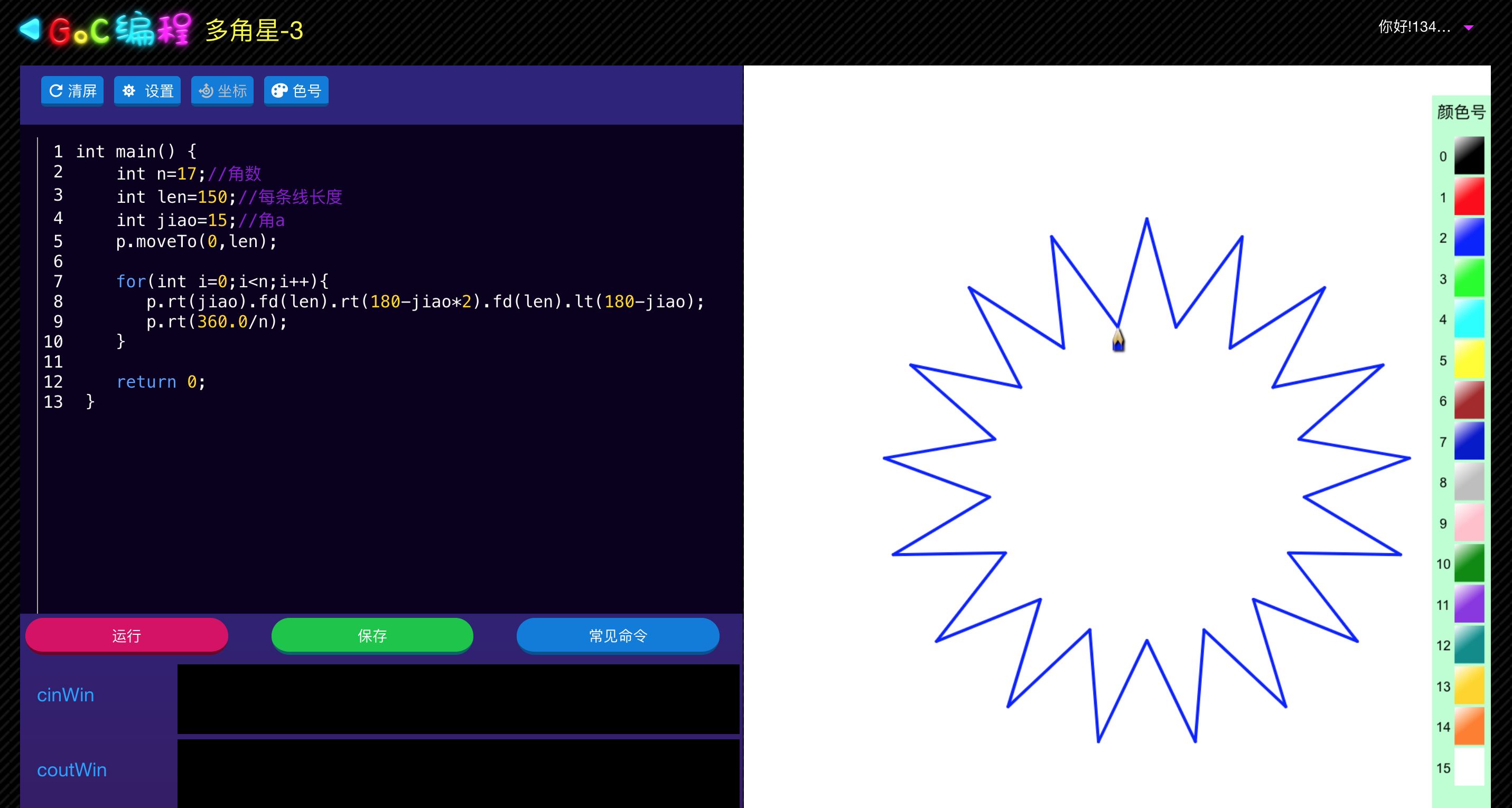
int main() { int n=17;//Angle number int len=150;//Length of each line int jiao=15;//Angle a p.moveTo(0,len); for(int i=0;i<n;i++){ p.rt(jiao).fd(len).rt(180-jiao*2).fd(len).lt(180-jiao); p.rt(360.0/n); } return 0; }
Draw inner polygonal star
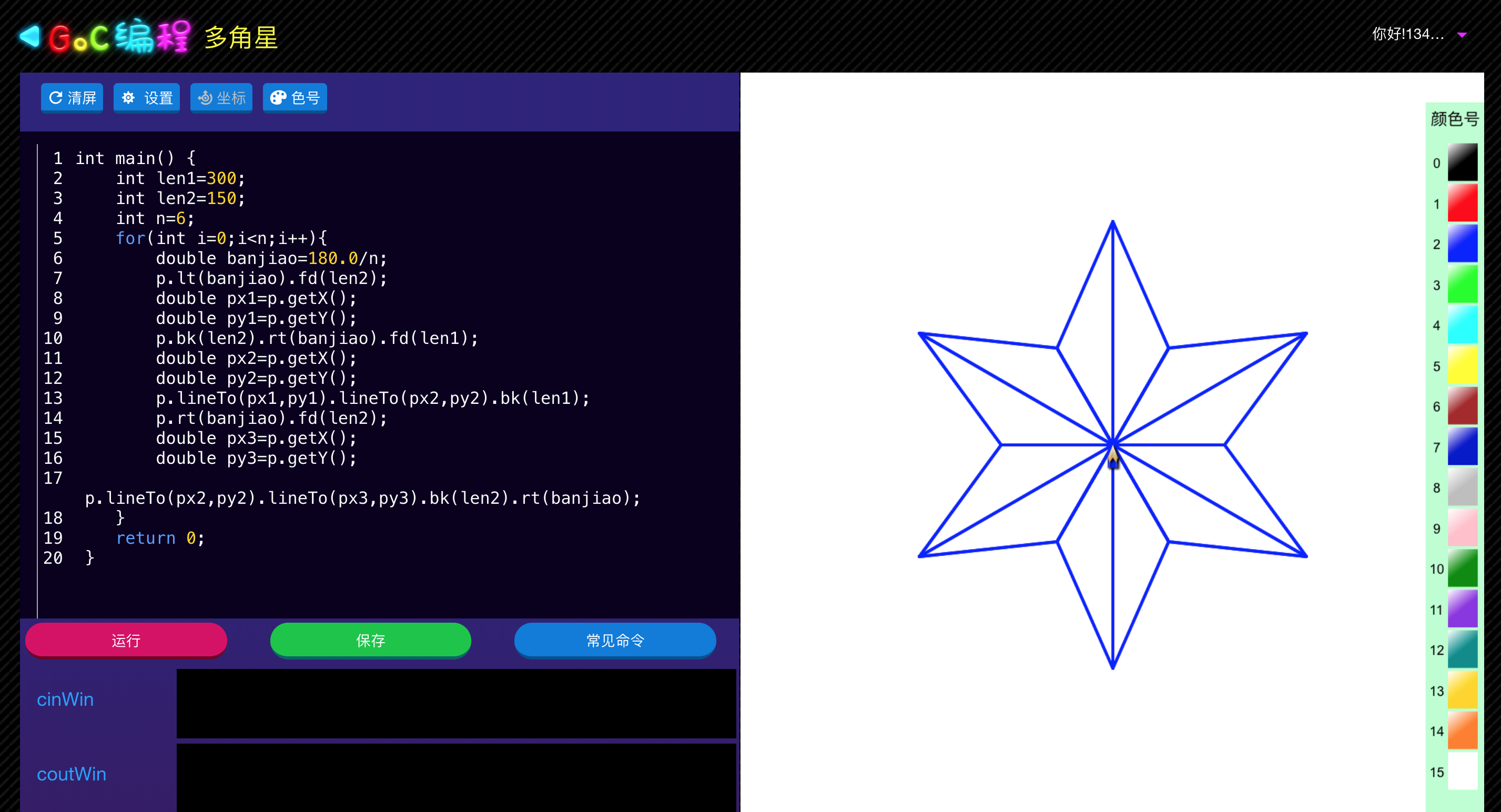
int main() { int len1=300; int len2=150; int n=6; for(int i=0;i<n;i++){ double banjiao=180.0/n; p.lt(banjiao).fd(len2); double px1=p.getX(); double py1=p.getY(); p.bk(len2).rt(banjiao).fd(len1); double px2=p.getX(); double py2=p.getY(); p.lineTo(px1,py1).lineTo(px2,py2).bk(len1); p.rt(banjiao).fd(len2); double px3=p.getX(); double py3=p.getY(); p.lineTo(px2,py2).lineTo(px3,py3).bk(len2).rt(banjiao); } return 0; }
Color square array
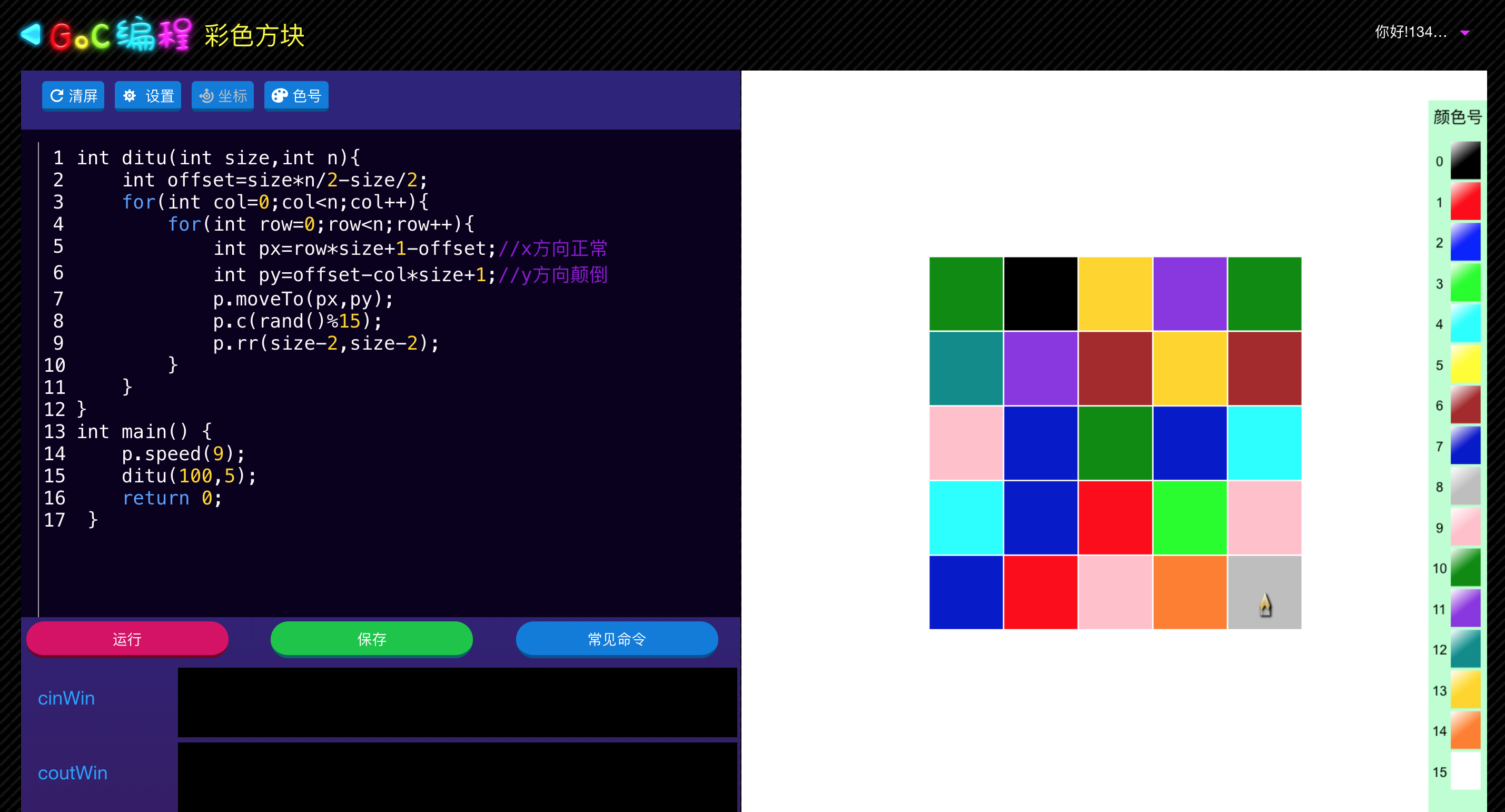
int gezi(int size,int n){ int offset=size*n/2-size/2; for(int col=0;col<n;col++){ for(int row=0;row<n;row++){ int px=row*size+1-offset; int py=offset-col*size+1; p.moveTo(px,py); p.c(rand()%15); p.rr(size-2,size-2); } } } int main() { p.speed(9); gezi(100,5); return 0; }
Count points to calculate the circumference
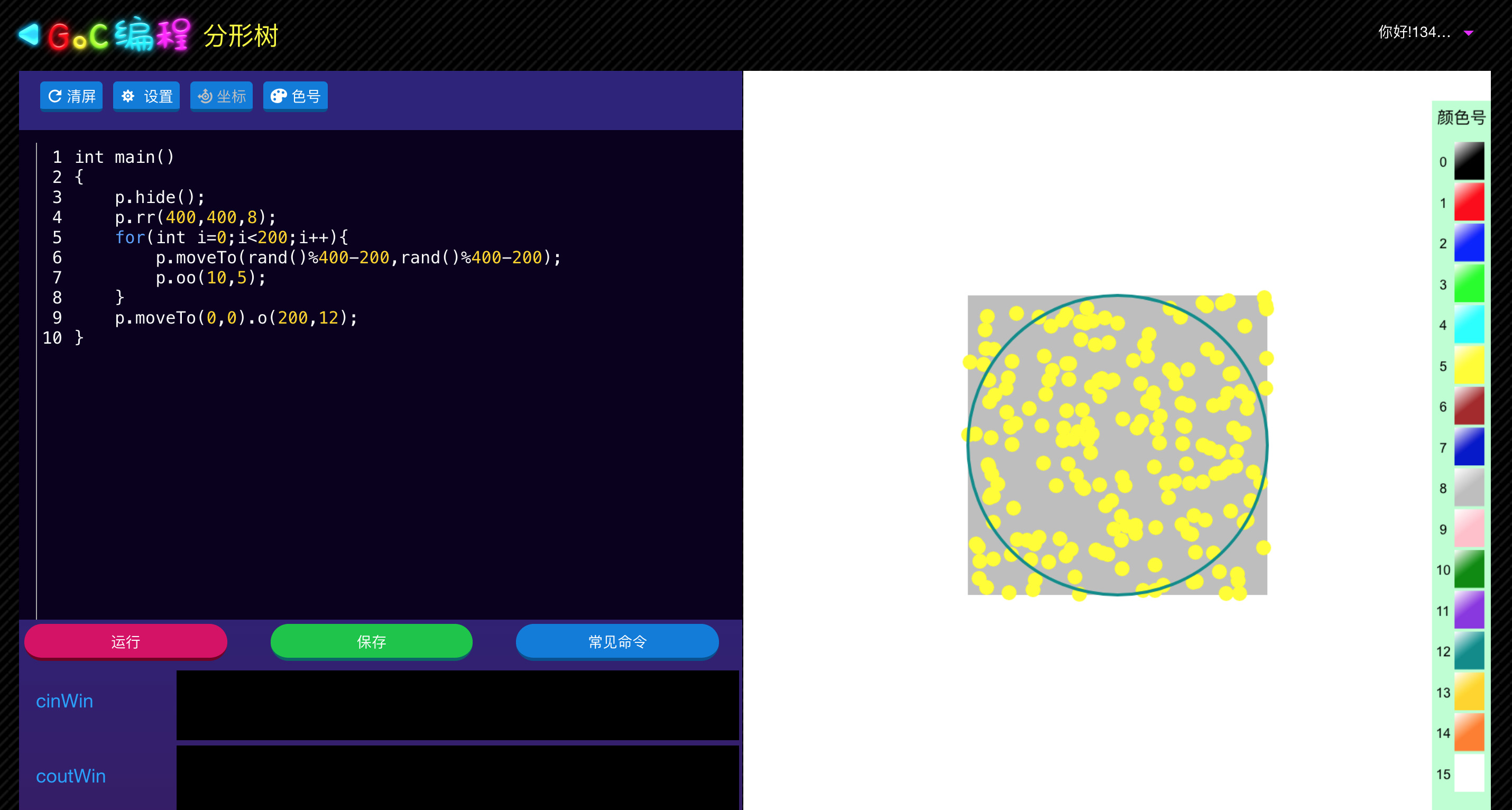
int main() { p.hide(); p.rr(200*2, 200*2, 8); //Here Short Book 400 is a sensitive word, so change to 2000*2 for(int i=0;i<200;i++){ p.moveTo(rand()%400-200,rand()%400-200); p.oo(10,5); } p.moveTo(0,0).o(200,12); }
Number lattice to calculate the circumference
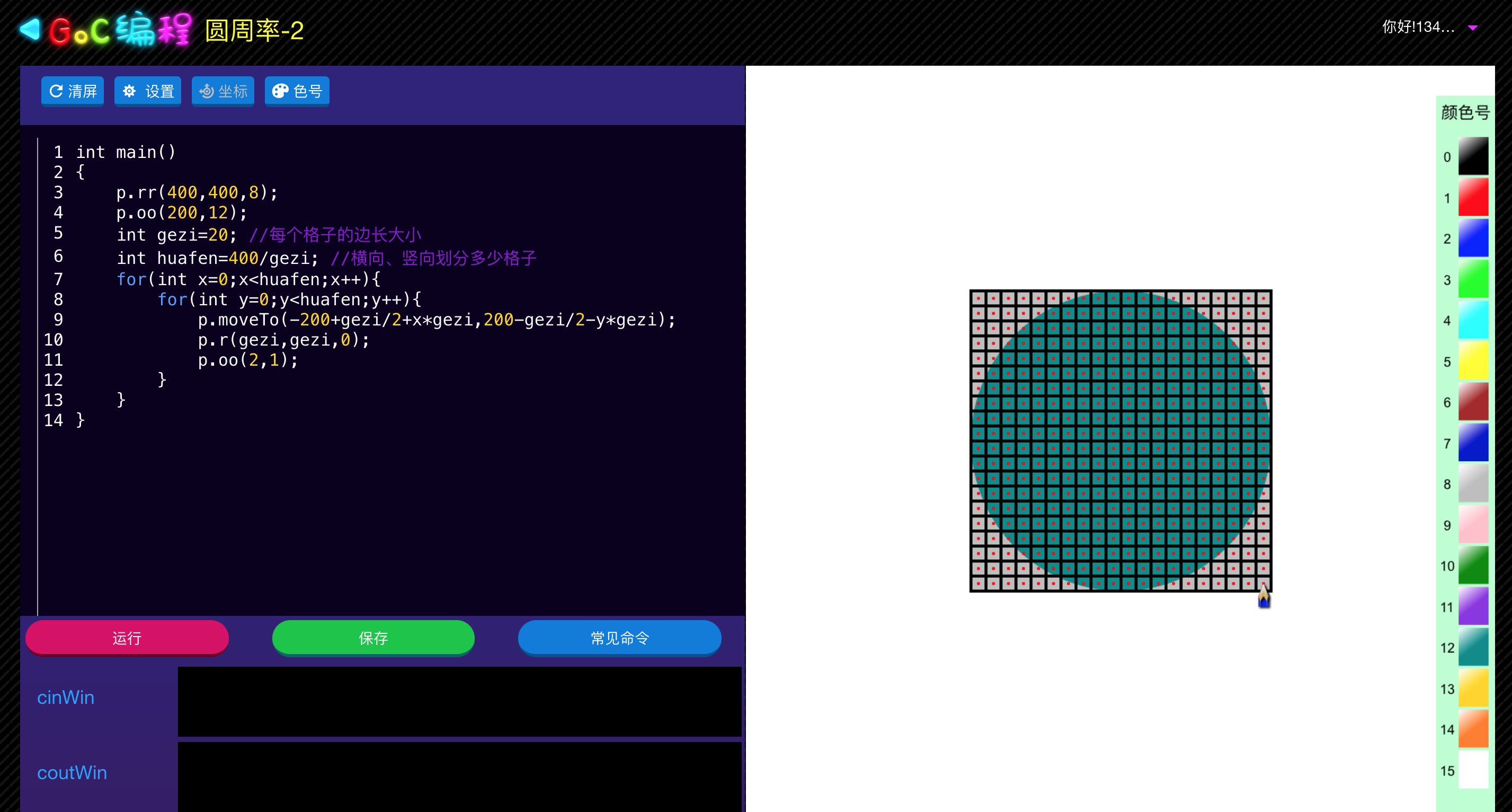
int main() { p.rr(200*2,200*2,8); p.oo(200,12); int gezi=20; //Size of edges per grid int huafen=400/gezi; //How many grids to divide horizontally and vertically for(int x=0;x<huafen;x++){ for(int y=0;y<huafen;y++){ p.moveTo(-200+gezi/2+x*gezi,200-gezi/2-y*gezi); p.r(gezi,gezi,0); p.oo(2,1); } } }
Polygon Side Length for Circumference Calculation
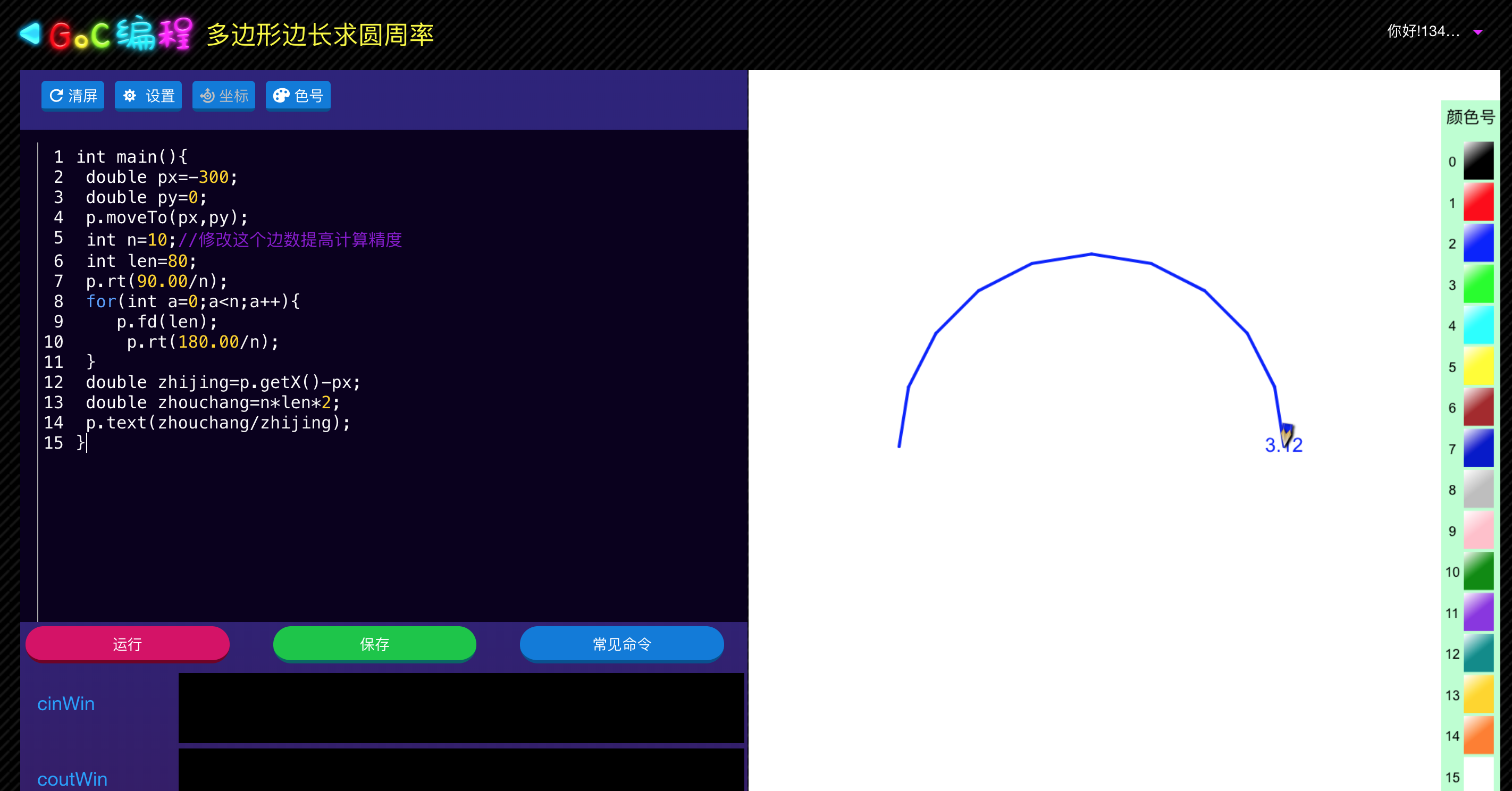
int main(){ double px=-300; double py=0; p.moveTo(px,py); int n=10;//Modify this number of edges to improve calculation accuracy int len=80; p.rt(90.00/n); for(int a=0;a<n;a++){ p.fd(len); p.rt(180.00/n); } double zhijing=p.getX()-px; double zhouchang=n*len*2; p.text(zhouchang/zhijing); }
Branch
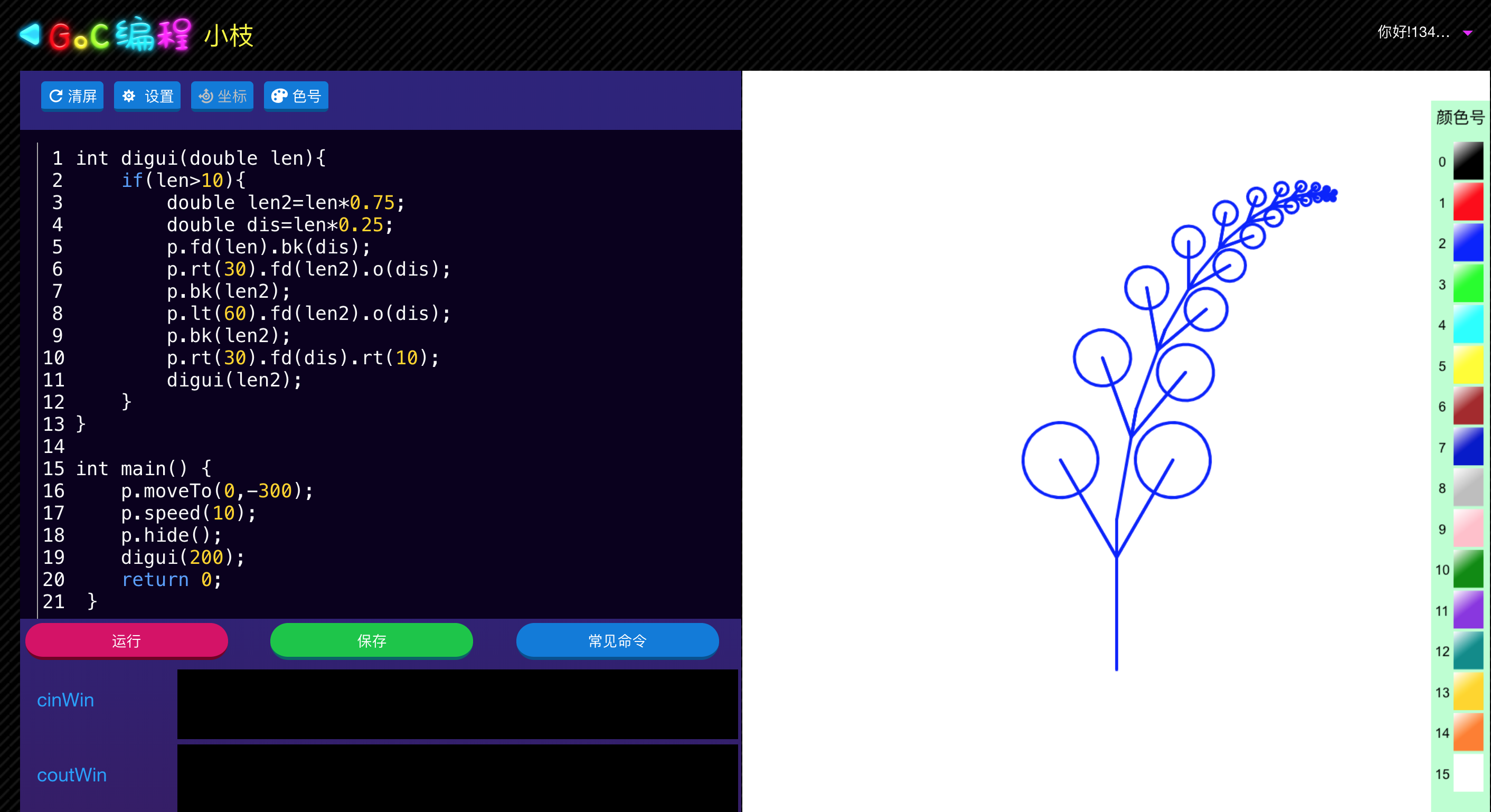
int aa(double len){ if(len>10){ double len2=len*0.7; //7 and five are sensitive words together in the short book, so use 7 here double dis=len*0.25; p.fd(len).bk(dis); p.rt(30).fd(len2).o(dis); p.bk(len2); p.lt(60).fd(len2).o(dis); p.bk(len2); p.rt(30).fd(dis).rt(10); aa(len2); } } int main() { p.moveTo(0,-300); p.speed(10); p.hide(); aa(200); return 0; }
Fractal Tree
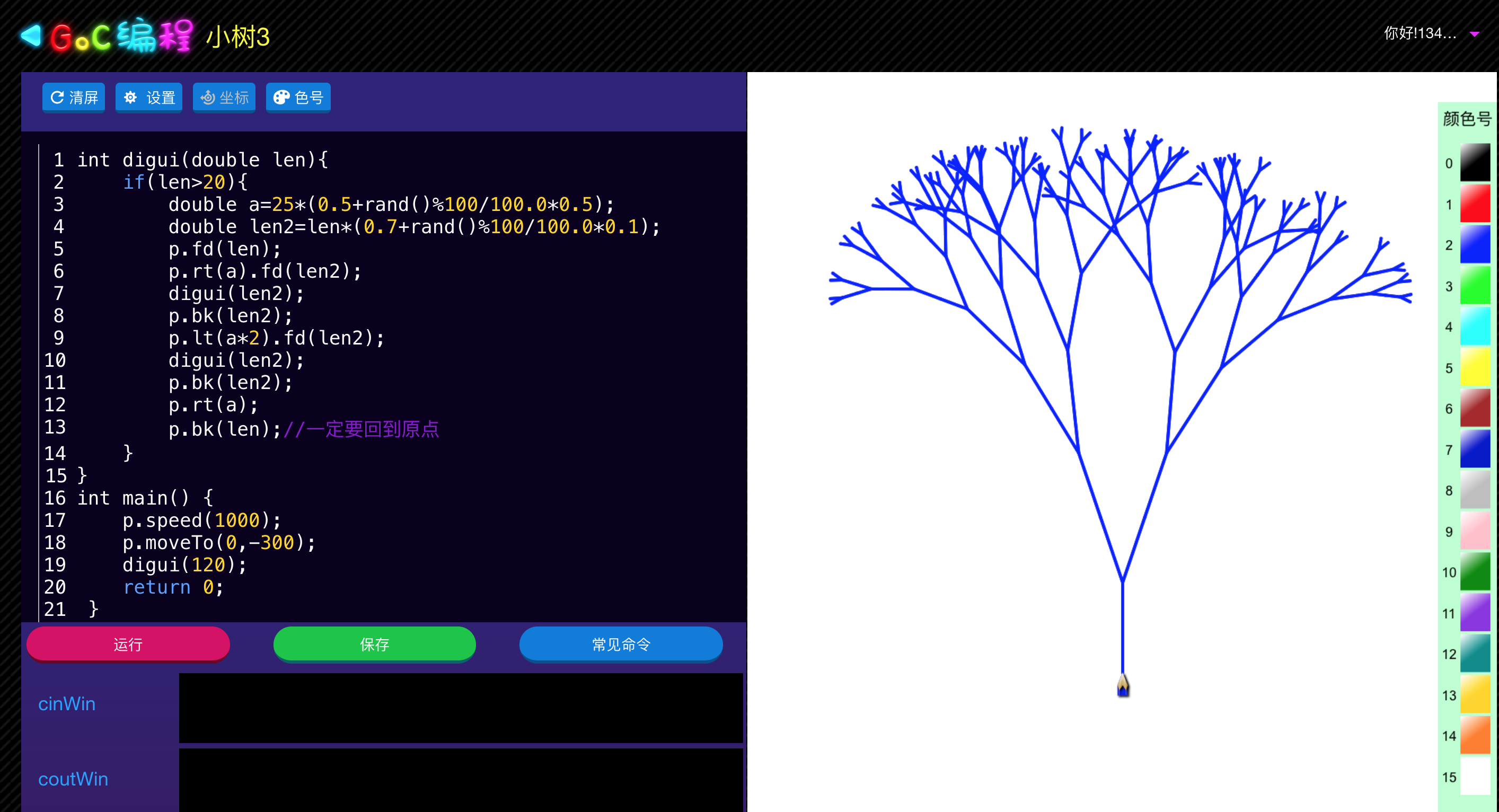
int aa(double len){ if(len>20){ double a=25*(0.5+rand()%100/100.0*0.5); double len2=len*(0.7+rand()%100/100.0*0.1); p.fd(len); p.rt(a).fd(len2); aa(len2); p.bk(len2); p.lt(a*2).fd(len2); aa(len2); p.bk(len2); p.rt(a); p.bk(len);//Be sure to go back to the origin } } int main() { p.speed(1000); p.moveTo(0,-300); aa(120); return 0; }
Sierpinski triangle
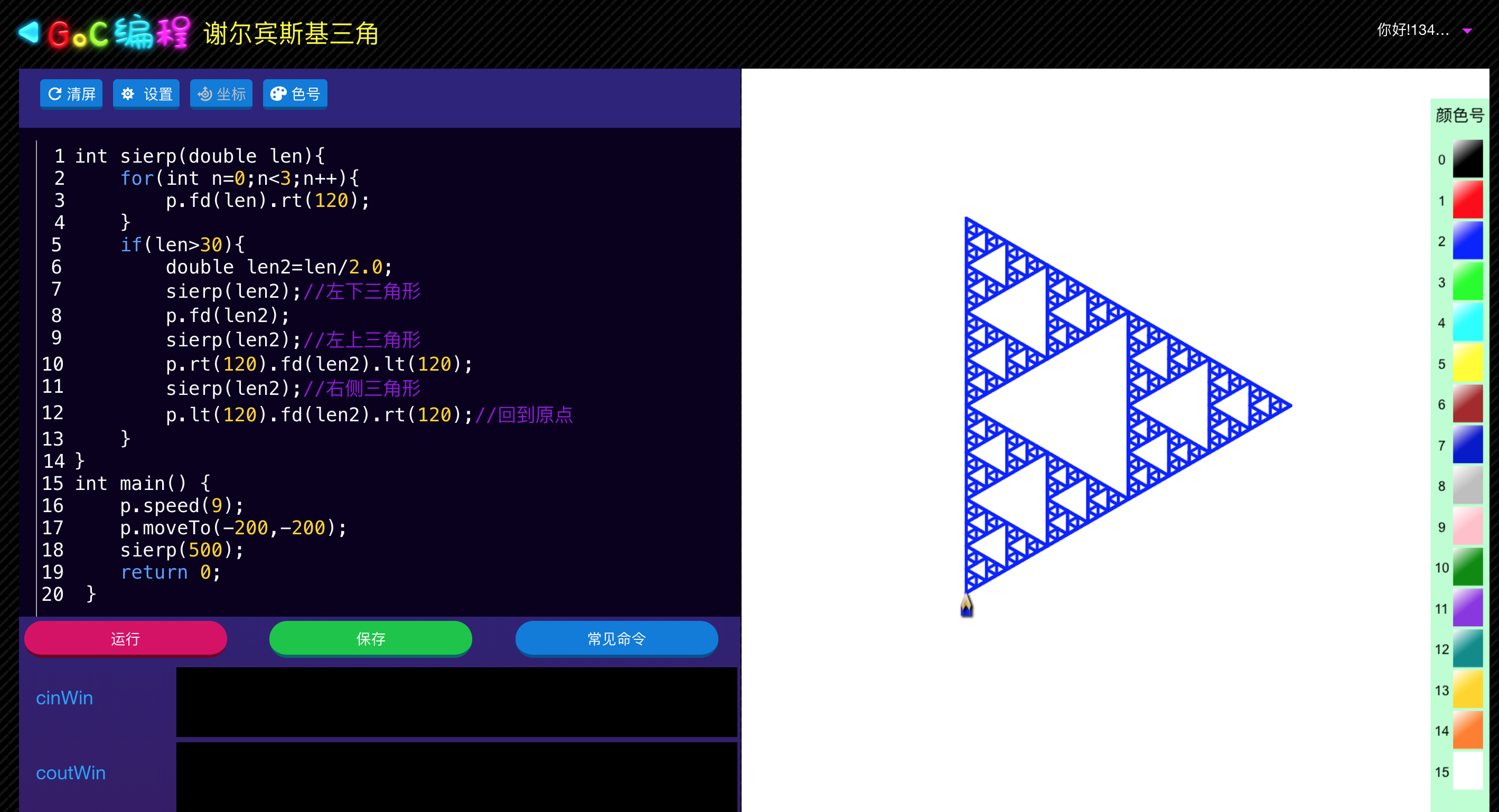
int sierp(double len){ for(int n=0;n<3;n++){ p.fd(len).rt(120); } if(len>30){ double len2=len/2.0; sierp(len2);//Lower left triangle p.fd(len2); sierp(len2);//Upper left triangle p.rt(120).fd(len2).lt(120); sierp(len2);//Right triangle p.lt(120).fd(len2).rt(120);//Back to Origin } } int main() { p.speed(9); p.moveTo(-200,-200); sierp(500); return 0; }
Koch Snowflake
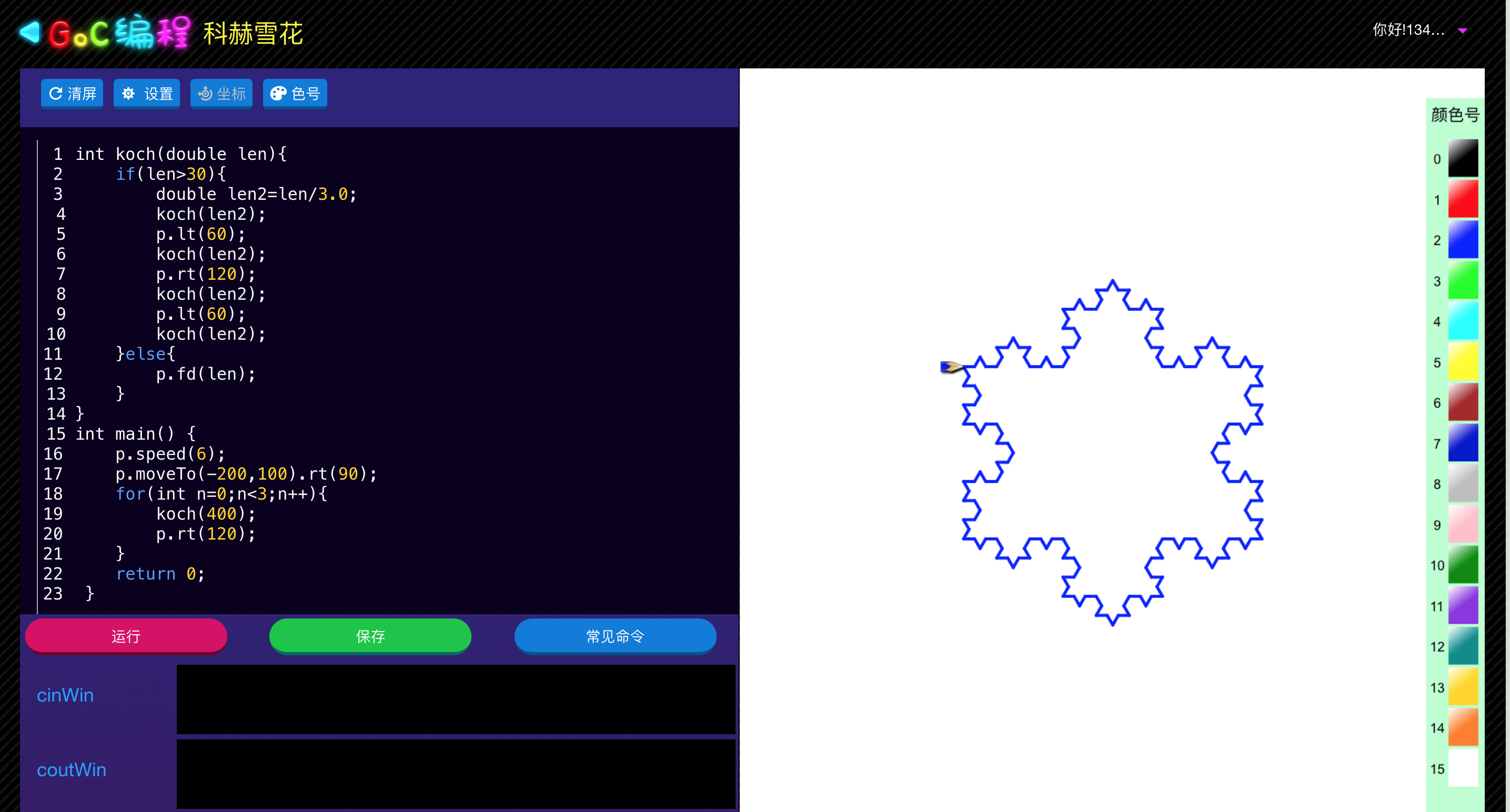
int koch(double len){ if(len>30){ double len2=len/3.0; koch(len2); p.lt(60); koch(len2); p.rt(120); koch(len2); p.lt(60); koch(len2); }else{ p.fd(len); } } int main() { p.speed(6); p.moveTo(-200,100).rt(90); for(int n=0;n<3;n++){ koch(400); p.rt(120); } return 0; }
More articles to watch my small collection Teenager Programming
Welcome to my column [General Knowledge of Artificial Intelligence]
More related articles Click June 2019 as Topic
A New Age of Intelligence for Everyone
If you find an error in the article, please leave a message to correct it.
If you find it useful, please like it;
If you find it useful, please reload ~
END