Py4DS|3 list, tuple and dictionary
Content structure:
1 List knowledge
2-tuple knowledge
3 dictionary knowledge
Target management:
After reading this article, you can get:
1. Creation of three data structures: list, tuple and dictionary
2 Application of lists, tuples and dictionaries
Data structure is a way of data organization and storage.
Lists, tuples, and dictionaries are three data structures in Python.
01
list
Let's first review Python's atomic data types:
float: floating point type
int: integer
str: character type
bool: Boolean
A list is a non atomic data structure. It is an ordered and variable collection. Use brackets [] to create a list. The operations of the list include accessing the list elements by using the index method, traversing the list elements by using the circular operation, and adding, deleting, modifying and sorting the list by using some common methods.
# List knowledge and skills # 1 create list height_list = [] print(type(height_list)) print(height_list) height_list1 = [1.75, 1.62, 1.83, 1.94] print(height_list1) # 2 list operation # 2.1 use the index to access the elements of the list, starting from 0 lang_list = ["C", "Python", "R", "Java", "C++"] print("My first programming language in College:", lang_list[0]) print("I do data science programming language:", lang_list[1]) print("I do data science programming language:", lang_list[2]) # 2.2 the list is variable. Modify the element value of the list print("Before modification:", lang_list) lang_list[3] = "Go" print("After modification:", lang_list) # 2.3 elements of the convenience list using the for loop for language in lang_list: print("Computer programming language:", language) # 2.4 use the len() function to output the number of elements in the list print("Number of elements in the programming language list:", len(lang_list)) # 2.5 list addition and deletion # append() -- add element operation lang_list.append("SQL") print("After modification", lang_list) # insert() -- insert element at a specific location lang_list.insert(3, "Matlab") print("After modification", lang_list) # Use the remove(), pop(), del() methods to delete elements print("Complete list", lang_list) lang_list.remove("Go") print("use remove Method to delete the list after the element", lang_list) lang_list.pop(2) print("use pop Method to delete the list after the element", lang_list) del(lang_list[0]) print("use del Method to delete the list after the element", lang_list) # 2.6 sorting of lists # Use the sort() method print("List before sorting:", lang_list) lang_list.sort() print("Sorted list:", lang_list)
02
tuple
Tuples are another data type in the Python language.
Tuples are similar to lists, but they are essentially different.
First, tuples are invariant (emphasis); Secondly, tuples are marked and created with parentheses.
Due to the immutability of tuples, it is impossible to add, delete, modify and query them.
When do we use the list? When do I use tuples? You can choose according to whether the data structure needs to change.
# Knowledge and skills # 1 tuple creation gzh_name = ("Data science and artificial intelligence", "R language", "Data talent") print(type(gzh_name)) print(gzh_name) # 2 element operation for name in gzh_name: print("My official account is:", name) print("%s Focus on data science and AI content" % gzh_name[0]) print("%s Focus on R Content of language" % gzh_name[1]) print("%s It is a data talent service provider" % gzh_name[2])
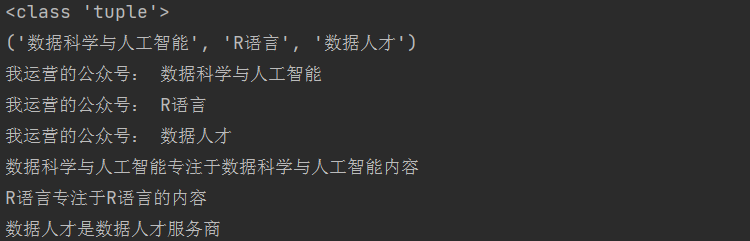
03
Dictionaries
A dictionary is a key value data structure.
Key is used to indicate the key value; Value is used to represent the value corresponding to the key value.
Key is unique.
Dictionaries are created by braces.
There are two ways to get the Value of Value from the dictionary. One way is to use the Key to obtain, and the other way is to use the get method to obtain.
# Dictionary knowledge and skills # 1. Creation of dictionary DS_AI_gzh = { "name": "Lu Qin", "content": "Focus on data science and artificial intelligence", "operation": "article+Community", "value": "Share and disseminate knowledge and skills, and use data to learn knowledge and create value", "wechat": "Welcome to wechat 1181906473 to exchange data science and artificial intelligence" } print(type(DS_AI_gzh)) print(DS_AI_gzh) # Dictionary operation # 1 get dictionary Value # 1) Use Key to get the Value of Value, 2) use get method print("Data science and AI official account number%s" % DS_AI_gzh["wechat"]) print("Data science and AI official account number%s" % DS_AI_gzh.get("wechat")) # 2. Modify dictionary Value # Improve the operation of official account number DS_AI_gzh["operation"] = "Image & Text+Community+Wechat Moments" print(DS_AI_gzh) # 3 traverse the contents of the dictionary # Use for loop operation # 3.1 output Key for gzh_info in DS_AI_gzh: print("Official account information contains Key", gzh_info) # 3.2 output Value for gzh_info in DS_AI_gzh.values(): print("Official account information contains Value", gzh_info) # 3.3 output key value for gzh_key_info, gzh_value_info in DS_AI_gzh.items(): print(gzh_key_info, gzh_value_info)
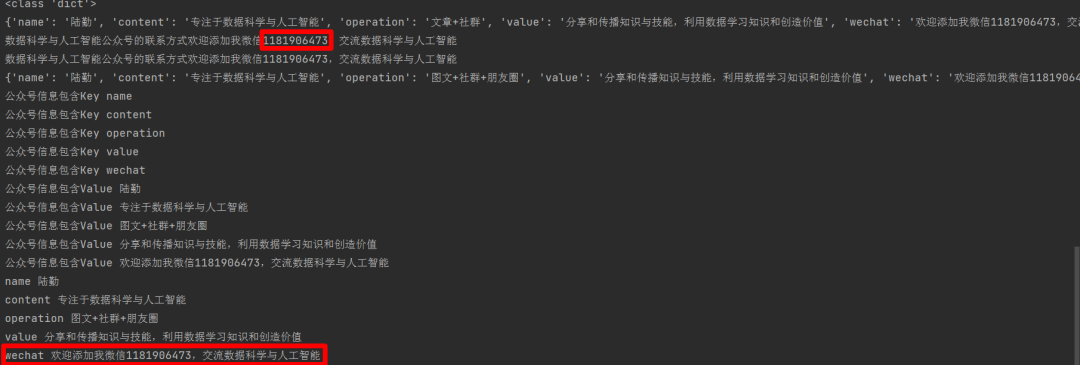