preface
Recently, I occasionally wrote some small tools to improve the efficiency of the project team. Because the intermediate module interacting with physical devices is based on Python, it is difficult to communicate with it with Java. In order to facilitate everyone's daily use, I stared at the Python GUI tool, tried several GUI frameworks, and finally fell in love with PyQt5, a tool with more use and relatively complete documents~
PyQt5 is based on QT library, which is a C + + library and development tool, including graphical user interface, network, thread, regular expression, SQL database, SVG, OpenGL, XML, user and application settings, location and location services, short-range communication (NFC and Bluetooth), web browsing, 3D animation, charts 3D data visualization and interface with application store. PyQt5 implements more than 1000 of these classes as a set of Python modules.
In short, PyQt5 is a GUI tool similar to Java Swing, in which many advanced modules have been built. Moreover, using PyQt Desinger, you can draw the interface graphically and convert it into py script file again.
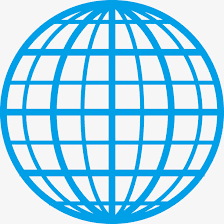
install
Omit the installation of Python 3 here and use pip tool to open CMD for installation:
pip install pyqt5 pip install pyqt5-tools
Shell
Copy
The first command is to install the core content of PyQt5. The following PyQt5 tools are powerful supporting tools such as QtDesigner and PyUIC.
Of course, in order to finally package as an exe tool, you also need to install the pyinstaller module:
pip install pyinstaller
Shell
Copy
In fact, the dependency library has been installed. Next, we configure the tools in PyCharm to open it quickly.
to configure
There are mainly two tools configured here. One is the drawing tool, which directly draws the UI of the tool in HTML form, and the other is the tool that converts the HTML interface into py file by one click.
Open pychart and click file -- > setting -- > tools -- > external tools
The right part is blank. Click the "+" sign to set the following settings:
Program: fill in the installation path of PyQtDesigner, that is, the installed designer The path where exe is located may be different for different versions. You have to find it under the Python installation path.
For example, my installed path is C: \ Python 39 \ lib \ site packages \ Qt5_ applications\Qt\bin\designer. exe
Fill in $FileDir $in the Working directory. For convenience, you can also click Insert Macro... On the right, Then find $FileDir $.
The second tool is PyUIC, which is the tool to convert the drawn interface UI into py file, or file -- > setting -- > tools -- > external tools. Open and add tools:
Program: is the installation path of Python
Arguments: fill in the following:
-m PyQt5.uic.pyuic $FileName$ -o $FileNameWithoutExtension$.py
Shell
Copy
Working directory: $FileDir$
So far, the basic setup has been completed, and we can start a simple test.
use
Click Tools - > external tools on the PyCharm navigation bar to open the QtDesigner configured above
The paint tool opens automatically:
We choose the default and click OK to get an initial interface
Of which:
- On the left is all the elements listed, including tables, input boxes, labels, check boxes, drop-down boxes, and so on
- In the middle is the drawing interface, which can move elements by itself
- On the top right is the object information of each element
- In the middle of the right is the attribute of the selected element. You can modify the size, default value, font, style, mouse over strategy and other information
- Below the right is the advanced attribute, that is, the signal slot of PyQt5, which can complete multi-threaded operation
Let's draw a simple form as follows:
You can click preview in the navigation bar above to preview the page:
Click Save as * ui file, open it with PyCharm, and convert it into py file with PyUIC tool:
Then a default Py file with the same name will be generated in the current directory:
To make the py file run, we need to add a main function as the startup entry, add the following code at the end of the py file just generated and introduce the corresponding dependencies:
if __name__ == '__main__': app = QApplication(sys.argv) MainWindow = QMainWindow() ui = Ui_Dialog() ui.setupUi(MainWindow) MainWindow.show() sys.exit(app.exec_())
Python
Copy
At this time, we can click Run py file to open this interface!
introduction
Now we use the above form to do such a thing. Click OK, the pop-up window will prompt "add successfully", and click "reset" to clear all the contents in the form. Therefore, we need to add dynamic operation in the py file. The whole logic is relatively simple, that is, when we click the "OK" button, we can get the filled contents of the above form and print in the background, It is added successfully in the pop-up window on the front desk, and when you click "reset", all the contents in the form will be cleared.
First, we add a binding event to the OK button:
self.pushButton.clicked.connect(lambda: self.submit_form())
Python
Copy
Then add the details of the binding event:
def submit_form(self): input_name = self.lineEdit.text() input_username = self.lineEdit_2.text() input_password = self.lineEdit_3.text() input_sex = self.comboBox.currentText() input_remark = self.textEdit.toPlainText() print(input_name) print(input_username) print(input_password) print(input_sex) print(input_remark) reply = QMessageBox.information(MainWindow, 'success', 'Submitted successfully', QMessageBox.Yes | QMessageBox.No, QMessageBox.Yes) if reply == QMessageBox.Yes: print('ok') else: print('cancel')
Python
Copy
Then the logical part is completed! As for the reset function, you can try it yourself~
All codes:
# -*- coding: utf-8 -*- # Form implementation generated from reading ui file 'untitled.ui' # # Created by: PyQt5 UI code generator 5.15.4 # # WARNING: Any manual changes made to this file will be lost when pyuic5 is # run again. Do not edit this file unless you know what you are doing. import sys from PyQt5 import QtCore, QtWidgets from PyQt5.QtWidgets import QApplication, QMainWindow, QMessageBox class Ui_Dialog(object): def setupUi(self, Dialog): Dialog.setObjectName("Dialog") Dialog.resize(463, 391) self.label = QtWidgets.QLabel(Dialog) self.label.setGeometry(QtCore.QRect(70, 40, 72, 15)) self.label.setObjectName("label") self.label_2 = QtWidgets.QLabel(Dialog) self.label_2.setGeometry(QtCore.QRect(70, 80, 72, 15)) self.label_2.setObjectName("label_2") self.label_3 = QtWidgets.QLabel(Dialog) self.label_3.setGeometry(QtCore.QRect(70, 120, 72, 15)) self.label_3.setObjectName("label_3") self.label_4 = QtWidgets.QLabel(Dialog) self.label_4.setGeometry(QtCore.QRect(70, 160, 72, 15)) self.label_4.setObjectName("label_4") self.label_5 = QtWidgets.QLabel(Dialog) self.label_5.setGeometry(QtCore.QRect(70, 200, 72, 15)) self.label_5.setObjectName("label_5") self.lineEdit = QtWidgets.QLineEdit(Dialog) self.lineEdit.setGeometry(QtCore.QRect(120, 80, 251, 21)) self.lineEdit.setObjectName("lineEdit") self.lineEdit_2 = QtWidgets.QLineEdit(Dialog) self.lineEdit_2.setGeometry(QtCore.QRect(120, 120, 251, 21)) self.lineEdit_2.setObjectName("lineEdit_2") self.lineEdit_3 = QtWidgets.QLineEdit(Dialog) self.lineEdit_3.setGeometry(QtCore.QRect(120, 40, 251, 21)) self.lineEdit_3.setObjectName("lineEdit_3") self.textEdit = QtWidgets.QTextEdit(Dialog) self.textEdit.setGeometry(QtCore.QRect(120, 190, 251, 87)) self.textEdit.setObjectName("textEdit") self.comboBox = QtWidgets.QComboBox(Dialog) self.comboBox.setGeometry(QtCore.QRect(120, 160, 87, 22)) self.comboBox.setObjectName("comboBox") self.comboBox.addItem("") self.comboBox.addItem("") self.pushButton = QtWidgets.QPushButton(Dialog) self.pushButton.setGeometry(QtCore.QRect(80, 320, 61, 28)) self.pushButton.setObjectName("pushButton") self.pushButton.clicked.connect(lambda: self.submit_form()) self.pushButton_2 = QtWidgets.QPushButton(Dialog) self.pushButton_2.setGeometry(QtCore.QRect(180, 320, 61, 28)) self.pushButton_2.setObjectName("pushButton_2") self.pushButton_3 = QtWidgets.QPushButton(Dialog) self.pushButton_3.setGeometry(QtCore.QRect(280, 320, 61, 28)) self.pushButton_3.setObjectName("pushButton_3") self.retranslateUi(Dialog) QtCore.QMetaObject.connectSlotsByName(Dialog) def retranslateUi(self, Dialog): _translate = QtCore.QCoreApplication.translate Dialog.setWindowTitle(_translate("Dialog", "Dialog")) self.label.setText(_translate("Dialog", "User:")) self.label_2.setText(_translate("Dialog", "account number:")) self.label_3.setText(_translate("Dialog", "password:")) self.label_4.setText(_translate("Dialog", "Gender:")) self.label_5.setText(_translate("Dialog", "remarks:")) self.comboBox.setItemText(0, _translate("Dialog", "male")) self.comboBox.setItemText(1, _translate("Dialog", "female")) self.pushButton.setText(_translate("Dialog", "determine")) self.pushButton_2.setText(_translate("Dialog", "Reset")) self.pushButton_3.setText(_translate("Dialog", "cancel")) def submit_form(self): input_name = self.lineEdit.text() input_username = self.lineEdit_2.text() input_password = self.lineEdit_3.text() input_sex = self.comboBox.currentText() input_remark = self.textEdit.toPlainText() print(input_name) print(input_username) print(input_password) print(input_sex) print(input_remark) reply = QMessageBox.information(MainWindow, 'success', 'Submitted successfully', QMessageBox.Yes | QMessageBox.No, QMessageBox.Yes) if reply == QMessageBox.Yes: print('ok') else: print('cancel') if __name__ == '__main__': app = QApplication(sys.argv) MainWindow = QMainWindow() ui = Ui_Dialog() ui.setupUi(MainWindow) MainWindow.show() sys.exit(app.exec_())