1, Preparations
1 download and install python
2 download and install VS code Editor
When installing, please check add to path
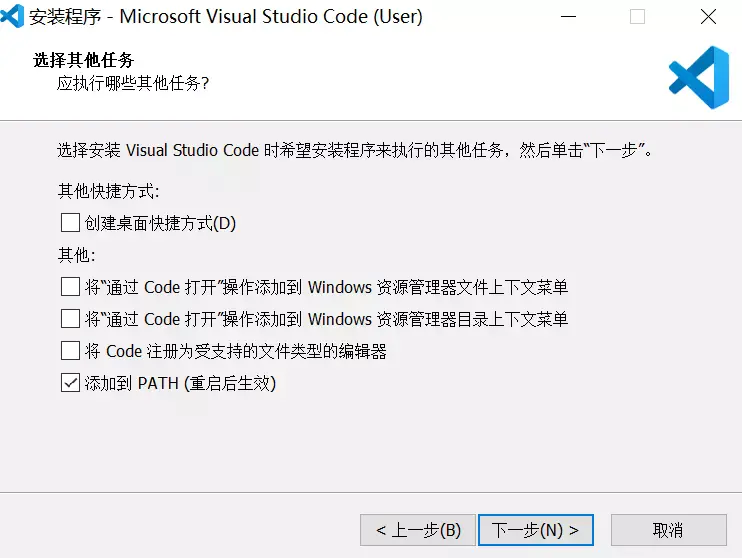
3 install pygame module
Note: many people will encounter various problems in the process of learning python, and no one will help answer the questions. For this reason, I have built a python full stack free Q & A exchange. Skirt: after a long time, I can find it under the conversion of liuyisi (homophony of numbers). The problems that I don't understand are solved by the old driver, and the latest Python tutorial project can be taken, so we can supervise each other and make progress together!
- Open the command line terminal in the top menu [terminal new temporary] of visual studio code, and then input the command python -m pip install --upgrade pip, enter and wait for completion.
-
Then enter the same command pip install pygame and wait for the installation to complete, which may take several minutes
2, Create project
<meta charset="utf-8">
-
Create a folder mygame on the desktop, then use the menu [file open folder] in VSCode, select the mygame folder, and the EXPLORER navigation bar will appear on the left side of VSCode.
-
In the left navigation bar, right click New File to create the file main.py.
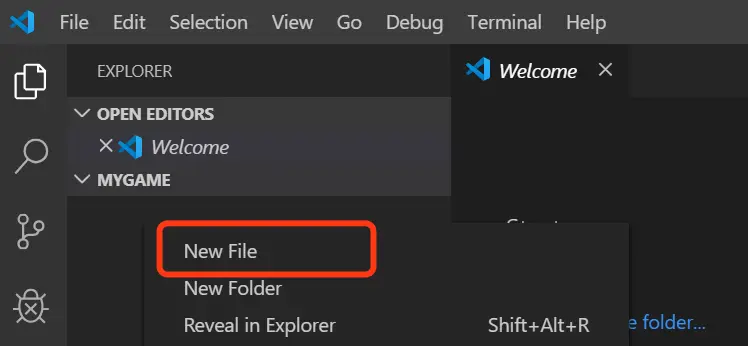
-Paste the following code into the 'main.py' file on the right.
import pygame
import sys
pygame.display.set_mode([600,400])
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
- Run the code.
*Still input the command 'python main.py' in the [terminal new terminal] terminal. This will run our above code, and a black window will pop up.
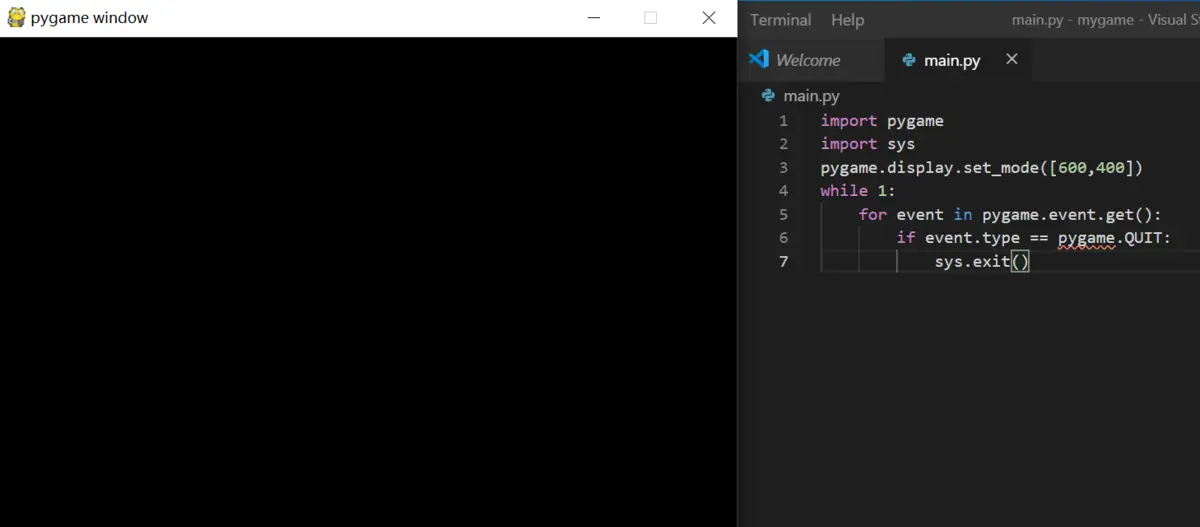
3, Optional actions
-
When performing the above operations, the bottom right corner of VSCode will often pop up some prompts. If there is the word "Install", you can click it to install more content.
-
You can also click the icon in the left column to open [EXTENSIONS], Search @ id:ms-python.python, click the found result, and then click the [Install] button on the right side to Install. After installation, the triangle run button will appear in the upper right corner of the main.py file. Click it to run the code, which is equivalent to entering python main.py in the terminal.
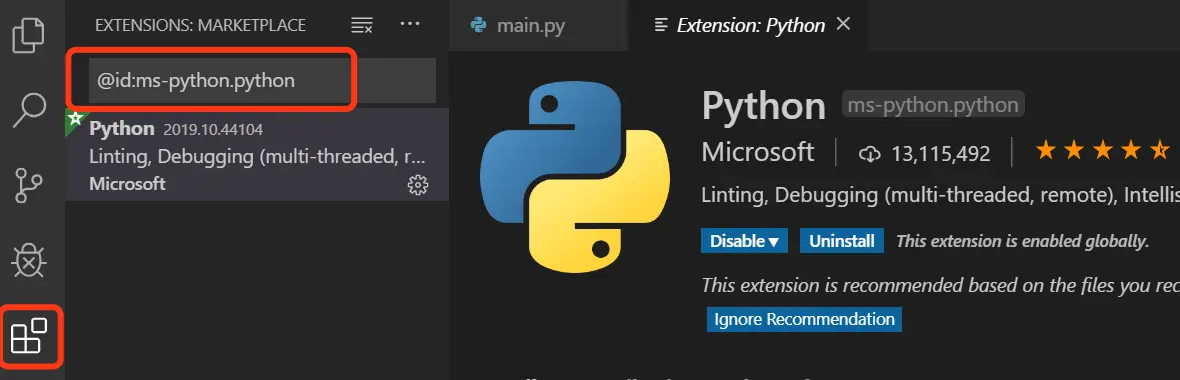
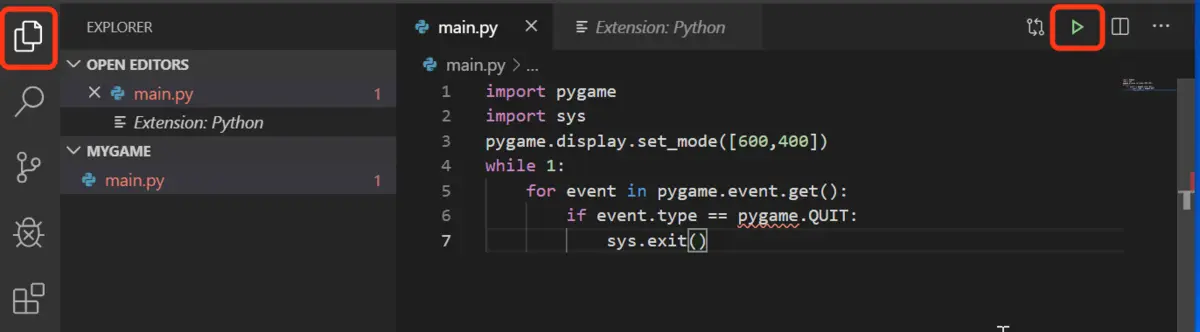
-
pip install... The install command is too slow. For Windows users, you can download the pip.ini file from the above network disk, create the PIP folder under the [C disk user user name] folder, copy the downloaded pip.ini file, and then run pip install... The installation speed will be much faster.
-
It's a lot of trouble for Apple users. First, execute cd ~ on the terminal to switch to the user folder, and then execute MKDIR. Pip to create the. PIP folder. It is invisible. We open visit, execute [go to folder...] from the menu, go to the ~ /. test directory, paste the downloaded pip.conf file into it, and finish.
pip.ini or pip.conf file is to download and install PIP from abroad by default instead of from home, so the speed will be much faster.
-
Among them, import is to import the external code module we want to use, pygame is of course necessary, Sys is the abbreviation of the system, because our game is to run on the system (windows or Mac OS), so we will use some commands of the system, such as the following command sys.exit().
-
Pyagme. Display. Set [mode ([600400]), where [600400] is a pair of numbers combined, called binary array, where it represents a rectangle with width of 600 and height of 400. The whole sentence is to set the size of the window to pop up, display display, set setting, mode mode mode.
-
When 1:... When it is 1, it means that 1 is correct, true and existing in the code. On the contrary, 0 means wrong, false and nonexistent. while 1:do something then something will be done, if while 0: do something then it will not be done.
-
For... Sys. Exit() is a fixed format. As long as you know that it means that when the game program is finished, the system will close the window, clean up the computer and leave no trace, and exit.
Ideas of game development
There are fixed routines in game development. Whether it's a big game like playing gophers, angry birds, watermelon ninjas, or even King glory, they generally follow the following ideas:
-
Create a map scene. There may be some props on it.
For example, there are several ground mouse holes, some wooden boxes that can hold pigs, and even very complex valley terrain, with many wild monsters on them.
The elements on these maps are generally passive, that is, if you don't approach or provoke wild monsters, they won't fight each other and kill each other. Similarly, when the birds haven't been launched, the wooden box won't collapse by itself. -
Create at least one element that the player can control that interacts with the map scene.
This controllable element is called the player character. The character is a hammer in the groundhog game. The character in the angry bird is actually a slingshot. The pop-up bird is actually a prop. In the king's glory game, the player's character is his hero.
-
There must be criteria to measure the outcome.
Player controlled characters interact with map scenes and react accordingly. There must also be a criterion, such as calculating the number of times to hit the hamster in 3 minutes, the number of green pigs killed, or the experience gained from fighting against the monsters. These rules must be clear and cannot contradict each other.
Most of the games have win or lose, and win or lose is usually only whose score first reaches a certain critical point. It can be the change of some key props, such as the tower is destroyed in the duel game, or it can be the attribute change of the player's character, such as being killed in the duel game, or it can be just a pure point evaluation, using the leaderboard instead of winning or losing.
Technical points of game development
-
To be able to draw in the window.
It can be drawing geometry directly with code or loading pictures to display content.
-
Be able to control the animation of each element (props and characters) with code.
Animation is a group of pictures constantly changing. To be able to use code control to play and stop the animation of each element, and to quickly switch between different animations.
-
Be able to receive user control and influence the elements in the game.
Know when the user pressed the keyboard, when he clicked the mouse, which key he pressed, left mouse button or right mouse button? We often call these operations interactive events.
-
It can calculate and manage the effective data generated by various elements in the game.
If the player's character cuts down, the monster's HP is reduced by 100 points. This is the data, and it is very useful data. Without this data, the monster may never die.
Sometimes these data should be saved so that users can still see that their level and equipment still exist the next time they open the game. Sometimes the data should be cleaned up in time. For example, when a new game starts again, the props and characters on the map should be restored as they are.
Ground mouse game
We can simplify the classic game of Groundhog as follows:
- Maps and props: gophers at random locations
- Interactive character: control the hammer figure and click the mouse figure to make it disappear
- Winning or losing points: number of times to hit the hamster figure in a limited time
The core play method is simplified into a sentence: Click to randomly display the figure.
Draw hamster
We use a blue circle to represent the hamster. How to draw a circle in the window?
Baidu [pyGame circle drawing] keyword can be used. You can find the pygame.draw.circle statement to be used. Its specific syntax can be found in the official document, Please click here for details.
We found that its syntax is:
pygame.draw.circle()
circle(surface, color, center, radius) -> Rect
This means that draw.circle() needs four parameters: surface, color, center point, radius.
Let's continue to see the description of the surface parameters:
surface (Surface) -- surface to draw on
It sounds like a canvas - you have to have a canvas before you can draw circles on it.
Click on the Surface link for further instructions:
Surface((width, height), flags=0, depth=0, masks=None) -> Surface
At the end of the sentence - "Surface means Surface((width...)" can generate a Surface. We can use the following statement to capture the generated Surface:
sur=pygame.Surface((600, 400)
In this way, sur is the surface we generate.
Color and location
Return to the color parameter:
color (Color or int or tuple(int, int, int, [int]))
-- color to draw with,
the alpha value is optional if using a tuple (RGB[A])
It's obviously a circle of what color to draw. tuple(int, int, int, [int]) indicates that three integers int are needed to represent color. RGB refers to red red, green green green, blue blue and alpha transparency
clr=(0,0,255) #blue
For the center position, we can also use the same method. Here, Vector2 represents the binary vector and the positions of horizontal x and vertical y:
pos=pygame.vector2(300,200) #Window center
Draw a circle
With all the parameters, you can start drawing circles. Run the following code:
import pygame
import sys
pygame.init() # initialization
window = pygame.display.set_mode([600, 400]) # Settings window
sur = pygame.Surface([600, 400]) # Draw background container
clr = (0, 0, 255)
pos = (300,200)
rad = 100
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
# Code executed in cycles per frame
pygame.draw.circle(sur, clr, pos, 100# Draw a circle
# Refresh screen
window.blit(sur, (0, 0))
pygame.display.flip()
Note that the bottom two lines of the refresh screen, in which window.blit(sur, (0, 0)) means to refresh the surface sur we have drawn into the window; pygame.display.flip() means to refresh the window.
Random occurrence
Random appearance is random position. We must make sure that the pos position of each flower circle is different, and it should be a fixed position of several gopher holes. ——Don't forget we're going to play the game of Groundhog.
Suppose that there are six positions of hamster pos, namely [200200], [300200], [400200], [200300], [300300], [400300], how to randomly select one of them? That is, how to randomly take a number from 1 to 6.
We can find from Baidu [Python random number] that we need to use random module, which is a module of python, and we don't need to pip install again.
If you search [python random document], you can find Official grammar description , as follows:
random.randint(a, b) Return a random integer N such that a <= N <= b. Alias for randrange(a, b+1).
This means that a number between a and b can be randomly generated. Or from Chinese rookie tutorial
Learn this knowledge.
Create a new test.py file. Let's test it:
import random
a = random.randint(0, 5)
print(a)
Each run generates a different number.
Continue testing:
import random
a = random.randint(0, 6)
pos6=[[200,200],[300,200],[400,200],[200,300],[300,300],[400,300]]
print(pos6[a])
The pos6[a] here represents the A in the six positions of pos6. Running this code will generate different locations each time.
After the test is successful, we copy it into the circle drawing code just now and get:
import pygame
import sys
import random
pygame.init() # initialization
window = pygame.display.set_mode([600, 400]) # Settings window
sur = pygame.Surface([600, 400]) # Draw background container
clr = (0, 0, 255)
pos6 = [[200, 200], [300, 200], [400, 200], [
200, 300], [300, 300], [400, 300]] # !!Six positions
rad = 100
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
# Code executed in cycles per frame
sur.fill((0, 0, 0)) # !!Use black to cover the previous frame to refresh
a = random.randint(0, 5) # !!random0 reach5
pygame.draw.circle(sur, clr, pos6[a], 100) # !!Use random location
# Refresh screen
window.blit(sur, (0, 0))
pygame.display.flip()
Note the addition of sur.fill... Line, which uses black (0,0,0) to clean up the content of the previous frame and avoid multiple circles.
Refresh every n frames
The above code will see the blue circle jumping around after running. It's too fast. We hope to stop after changing the position and wait for us to hammer it.
We need the circle of the picture to change randomly every n frames instead of every frame now. The idea is as follows: we set a counter, which starts with 0, and each frame adds 1 to it, that is, 0,1,2,3,4... Until it increases to more than 50, then we change the position of the circle and reset the counter to 0 at the same time.
The code is as follows:
import pygame
import sys
import random
pygame.init() # initialization
window = pygame.display.set_mode([600, 400]) # Settings window
sur = pygame.Surface([600, 400]) # Draw background container
clr = (0, 0, 255)
pos6 = [[200, 200], [300, 200], [400, 200], [
200, 300], [300, 300], [400, 300]] # Six positions
rad = 100
tick=0 #!!Counter
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
# Code executed in cycles per frame
if tick>50: #each50 Refresh transform once
sur.fill((0, 0, 0)) # Use black to cover the previous frame to refresh
a = random.randint(0, 5) # random0 reach5
pygame.draw.circle(sur, clr, pos6[a], 100) # Use random location
tick=0
else: #!!When the transformation is not refreshed
tick=tick+1 #!!add counters
# Refresh screen
window.blit(sur, (0, 0))
pygame.display.flip()
Add interactive Click
When the user clicks on the screen, we need to know where it has clicked and whether it has clicked on the circle we drew.
Baidu search [pygame click] can find relevant resources, or Find it directly in the official documents.
The idea is that we add real-time monitoring of event.type event types, once we find a click event, we get the location coordinates. The code is as follows:
import pygame
import sys
import random
from pygame.locals import * # Bring in mouse event type
pygame.init() # initialization
window = pygame.display.set_mode([600, 400]) # Settings window
sur = pygame.Surface([600, 400]) # Draw background container
clr = (0, 0, 255)
pos6 = [[200, 200], [300, 200], [400, 200], [
200, 300], [300, 300], [400, 300]] # Six positions
rad = 100
tick = 0 # ! counter
pos = pos6[0] # !! Record the position of the circle outside
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == MOUSEBUTTONDOWN: # !! If it's a mouse down event
mpos = pygame.mouse.get_pos() # !! Get mouse position
print(mpos)
# Code executed in cycles per frame
if tick > 50: # Every 50 refreshes and transforms
sur.fill((0, 0, 0)) # Use black to cover the previous frame to refresh
a = random.randint(0, 5) # Random 0 to 5
pos = pos6[a] # !! Update the position of the circle of the external record
pygame.draw.circle(sur, clr, pos, 100) # !! Use random locations
tick = 0 # Reset counter
else: # !! When the transformation is not refreshed
tick = tick+1 # !! Increase counter
# Refresh screen
window.blit(sur, (0, 0))
pygame.display.flip()
Run this code, any click on the screen will print out the location of the schedule mouse click.
Distance measurement
Know the position pos of the current circle and the position mpos of the current click, so we can calculate the distance between the two points. If the distance is greater than the radius of the circle, there is no point to the hamster. If the distance is less than the radius, there is point to the hamster.
Baidu search [pygame two point distance] can find some methods to calculate the distance. Here we use the method provided by pygame official to test the following code:
import pygame
a=pygame.math.Vector2.length(pygame.math.Vector2(3,4))
print(a)
It will output 5. Here (3,4) is the difference between pos and mpos.
Take this idea into the original code and get:
import pygame
import sys
import random
from pygame.locals import * # Bring in mouse event type
pygame.init() # initialization
window = pygame.display.set_mode([600, 400]) # Settings window
sur = pygame.Surface([600, 400]) # Draw background container
clr = (0, 0, 255)
pos6 = [[200, 200], [300, 200], [400, 200], [
200, 300], [300, 300], [400, 300]] # Six positions
rad = 50
tick = 0 # Counter
pos = pos6[0] # Record the position of the circle outside
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == MOUSEBUTTONDOWN: # If it's a mouse down event
mpos = pygame.mouse.get_pos() # Get mouse position
dis = pygame.math.Vector2(
mpos[0]-pos[0], mpos[1]-pos[1]) # !!Calculate coordinate difference
len = pygame.math.Vector2.length(dis) # !!Calculate distance
if len < rad:
tick = 51 # !!Change position now
# Code executed in cycles per frame
if tick > 50: # each50 Refresh transform once
sur.fill((0, 0, 0)) # Use black to cover the previous frame to refresh
a = random.randint(0, 5) # random0 reach5
pos = pos6[a] # Update the position of the circle of the external record
pygame.draw.circle(sur, clr, pos, 100) # Use random location
tick = 0 # Reset counter
else: # When the transformation is not refreshed
tick = tick+1 # add counters
# Refresh screen
window.blit(sur, (0, 0))
pygame.display.flip()
Here we set that if the distance length len is less than the radius rad of the circle, then set tick=51 immediately to make it greater than 50, and perform random position transformation immediately.
By running the above code, the hamster (circle) can appear randomly and click to make it disappear, which also realizes the most basic logic function of the game. In the future, we will write more contents to make it more perfect.
Record scores
It's easy to increase the number. Set a score=0, and then increase it by 1 when you hit the hamster. But how to display it on the screen?
Baidu can search [pygame display text] and then find the general method. Let's do some tests first:
import pygame
pygame.init() # initialization
window = pygame.display.set_mode([600, 400]) # Settings window
# display text
pygame.font.init() # !! Initialize text
font = pygame.font.SysFont('Microsoft YaHei ', 30) # !! Set font and size
sur = font.render("Hello World!!{}".format(999), False, (255, 0, 0)) # !! Generate w text surface
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
window.blit(sur, (200, 10)) # !! Add fractional surface
pygame.display.flip()
In this code, you can see that pygame draws text in three steps:
- pygame.font.init() needs to be initialized first
- pygame.font.SysFont('microsoft YaHei ', 30) set font and font size
-
font.render("Hello World!!{}".format(999), False, (255, 0, 0)) generates a Surface
Finally, of course, don't forget to put the surface in the window
Run the above code to get a window as follows:
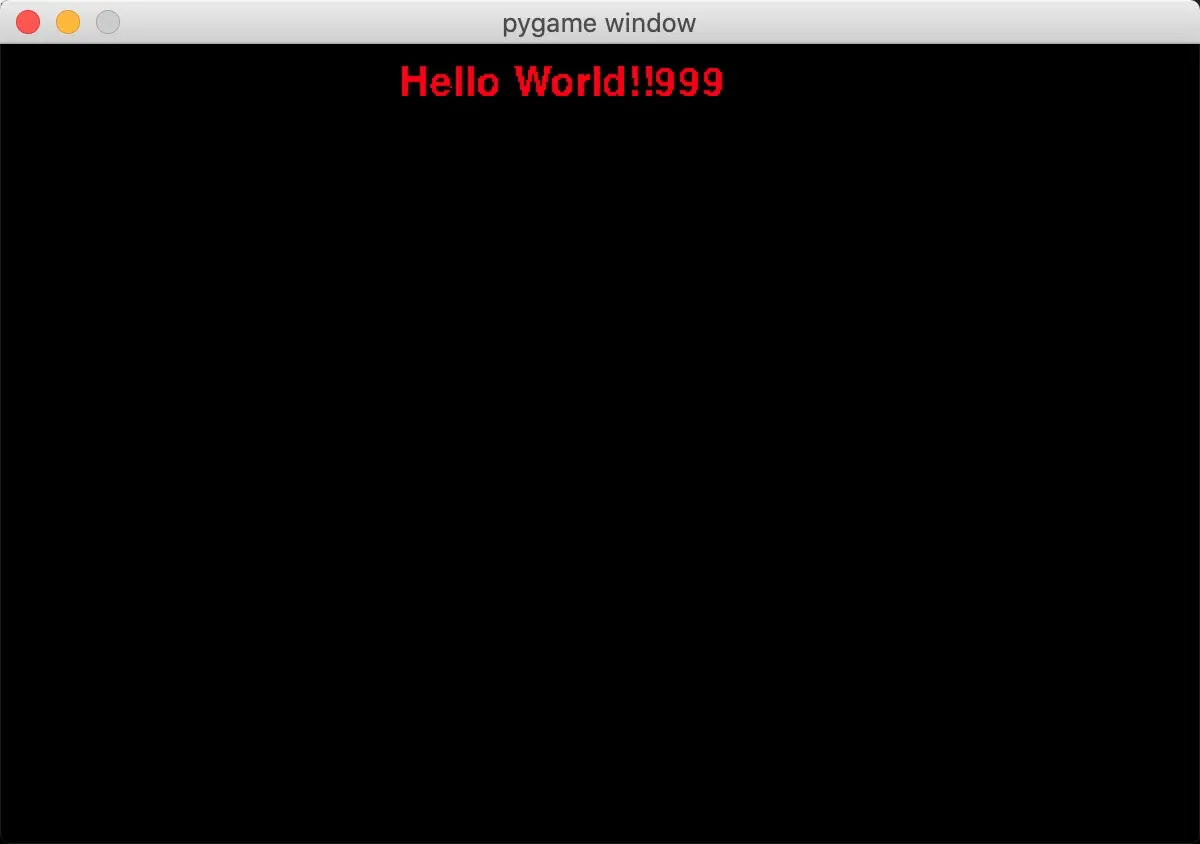
We improved the code based on this experience:
import pygame
import sys
import random
from pygame.locals import * # Bring in mouse event type
pygame.init() # initialization
window = pygame.display.set_mode([600, 400]) # Settings window
sur = pygame.Surface([600, 400]) # Draw background container
clr = (0, 0, 255)
pos6 = [[200, 200], [300, 200], [400, 200], [
200, 300], [300, 300], [400, 300]] # Six positions
rad = 50
tick = 0 # Counter
pos = pos6[0] # Record the position of the circle outside
# Fraction
score = 0 # !! Score count
pygame.font.init() # !! Initialize text
score_font = pygame.font.SysFont('Microsoft YaHei ', 30) # !! Set font and size
score_sur = score_font.render(str(score), False, (255, 0, 0)) # !! Generate count surface
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == MOUSEBUTTONDOWN: # If it's a mouse down event
mpos = pygame.mouse.get_pos() # Get mouse position
dis = pygame.math.Vector2(
mpos[0]-pos[0], mpos[1]-pos[1]) # Calculate coordinate difference
len = pygame.math.Vector2.length(dis) # Calculate distance
if len < rad:
tick = 1000 # Change position now
score = score+1 # Score increase
# Code executed in cycles per frame
if tick > 50: # Every 50 refreshes and transforms
score_sur = score_font.render(
str(score), False, (255, 0, 0)) # !! Regenerate fractional text surface
sur.fill((0, 0, 0)) # Use black to cover the previous frame to refresh
a = random.randint(0, 5) # Random 0 to 5
pos = pos6[a