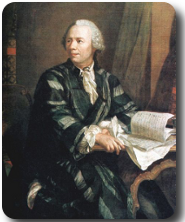
For more questions, see: https://www.jianshu.com/p/8c3ec805433d
84. Monopoly
The standard chessboard of the moneybags is roughly as follows:
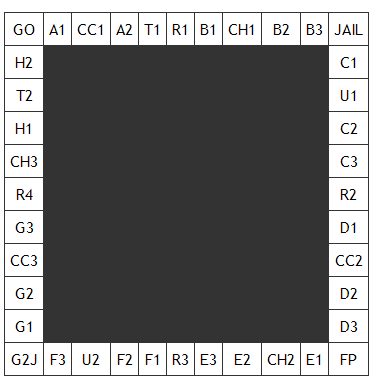
_Starting from the square marked with GO, throw two six-sided dice, and take the sum of the points as the number of steps forward in this round. If no other rules are added, after many rounds of play, the probability of falling on each grid should be close to 2.5%. However, due to the existence of G2J (imprisonment), CC (treasure box card) and CH (opportunity card), this probability distribution will change.
In addition to falling on G2J or possibly drawing jail cards on CC or CH, if players throw two identical points three times in a row, such as (3,3), (1,1), (5,5), they will not advance 5+5=10 steps on the third time, but go straight to jail.
At the beginning of the game, the cards in CC and CH will be randomly disrupted. When a player falls on CC or CH, they take a card from the top of CC and CH cards and move forward according to the meaning of the card, and put the card back to the bottom of the stack. The treasure box card and the opportunity card each have 16 cards, because we only care about the cards that will affect the movement, the other cards are invalid. The cards of treasure box card and opportunity card are as follows:
-
Treasure Box Card (2 cards, 14 other cards are invalid):
Back to the starting point GO
_Entering Jail JAIL -
Opportunity cards (10 cards, the other 6 are invalid):
Back to the starting point GO
_Entering Jail JAIL
Move to C1
Move to E3
Moving to H2
Move to R1
Move to the next R (Railway Company)
Move to the next R
Move to the next U (Public Service Company)
_three steps backward
_This question mainly examines the probability of stopping on a particular grid. Obviously, besides the possibility of stopping on G2J is 0, the possibility of stopping on CH is the smallest, because there is a 5/8 probability that the player will move to another grid. There is no distinction between being sent to prison or being left in prison, and regardless of the need to throw two identical points in order to get out of prison, it is assumed that the second round of entry into prison will be automatically released.
_Starting from the starting point GO, we can connect these two digits to represent the sequence of squares by marking the squares from 00 to 39 in turn.
Statistically speaking, the three largest stopping probability squares are:
__JAIL(6.24%): grid 10
__E3(3.18%): square 24
_GO(3.09%): square 00.
The three squares can be represented by a six-digit string: 102400
The above possibilities are obtained by using two six-sided dices. Now we use two four-sided dices to find a six-digit string consisting of three squares with the greatest stopping probability.
Python 3 Answer
import numpy as np import random #Computation of random numbers def Comnum(num, count):#Calculating the Random Numbers of Several Colors numlist = [] st = 0 while st < count: numlist.append(random.randint(1, num)) st += 1 return numlist #CH card chlist = list(np.array([0, 10, 11, 24, 39, 5, 99, 99, 999, 9999] +[99999] * 6)[random.sample(range(16), 16)])#99999 represents invalid cards and shuffles immediately chdict ={7: [15, 12], 22: [25, 28], 36: [5, 12]} #CC card bxlist = list(np.array([0, 10] +[99999] * 14)[random.sample(range(16), 16)])#99999 represents invalid cards and shuffles immediately bxdict = [2, 17, 33] #Define the function after taking card and playback card def Card(exlist): return exlist[1:] + [exlist[0]]#Place the cards at the bottom after you have taken them. #Counting dictionary countdict = {kk : 0 for kk in range(40)}#Each number represents a square. #Start simulation start = 0 jailsign = 0 monitimes = 10000#Number of simulations for moni in range(monitimes): #Beginning Random Number randomlist = Comnum(4, 2)#Calculating the Random Numbers of Two Four-Faced Chromosomes sezi = sum(randomlist)#Calculate the number of steps forward if randomlist[0] == randomlist[1]: jailsign += 1 else: jailsign = 0 if jailsign >= 3:#Throw the same number of points three times in a row countdict[10] += 1#JAIL start = 10 else: busu = (sezi + start) % 40 if busu == 30:#G2J countdict[10] += 1#JAIL start = 10 else: if busu in bxdict:#CC card if bxlist[0] == 99999:#Stop Moving countdict[busu] += 1 start = busu else: countdict[bxlist[0]] += 1 start = bxlist[0] bxlist = Card(bxlist).copy() elif busu in chdict:#CH card if chlist[0] == 99:#next R nnum = chdict[busu][0] elif chlist[0] == 999:#next U nnum = chdict[busu][1] elif chlist[0] == 99999:#Stop Moving nnum = busu elif chlist[0] == 9999:#Backward 3 nnum = busu - 3 if nnum == 33:#CC card if bxlist[0] != 99999: # Stop Moving nnum = bxlist[0] bxlist = Card(bxlist).copy() else: nnum = chlist[0] countdict[nnum] += 1 start = nnum chlist = Card(chlist).copy() else: countdict[busu] += 1 start = busu #Select the first n maximum values and output the string def Str(exdict, N, M): sdict = sorted(exdict.items(), key = lambda x: x[1], reverse = True) ncount = 0 strkey = '' while ncount < N: item = sdict[ncount] if len(str(item[0])) < 2: strkey += '0%s'%item[0] print('0%s : %.4f%%\n'%(item[0], exdict[item[0]] / M * 100)) else: strkey += '%s' % item[0] print('%s : %.4f%%' % (item[0], exdict[item[0]] / M * 100)) ncount += 1 return strkey print('After the simulation, the number of stops per square:%s'%countdict) print(Str(countdict, 3, monitimes)) //Answer: //After the simulation is completed, the number of stops per square is: {0: 276, 1: 185, 2: 148, 2: 148, 3: 201, 4: 217, 4: 217, 5: 275, 6: 208, 6: 208, 7: 80, 8: 198, 9: 198, 9: 218, 9: 218, 10: 218, 10: 762, 11: 212, 12: 250, 13: 246, 13: 246, 14: 246, 14: 302, 14: 302, 15: 380, 16: 300, 16: 309, 17: 287, 18: 293, 19: 283, 19: 289, 20: 308, 21: 301, 21: 301, 22: 113, 23: 288, 24: 356, 24: 25, 25: 356, 28:281, 29:296, 30:0, 31:293, 32:225, 33:225, 34:248 35: 181, 36: 75, 37: 197, 38: 216, 39: 251} #Three squares with the highest stopping probability: 10 : 7.6200% 15 : 3.8000% 24 : 3.5600% #Six-digit string: 101524