1, Foreword
this article mainly explains the basic operation of files in Python. It is the basic explanation of file operation. It will be used in subsequent automation tests. Below is the portal of a series of articles. Interested partners can also go to check it. Let's have a look at it together~
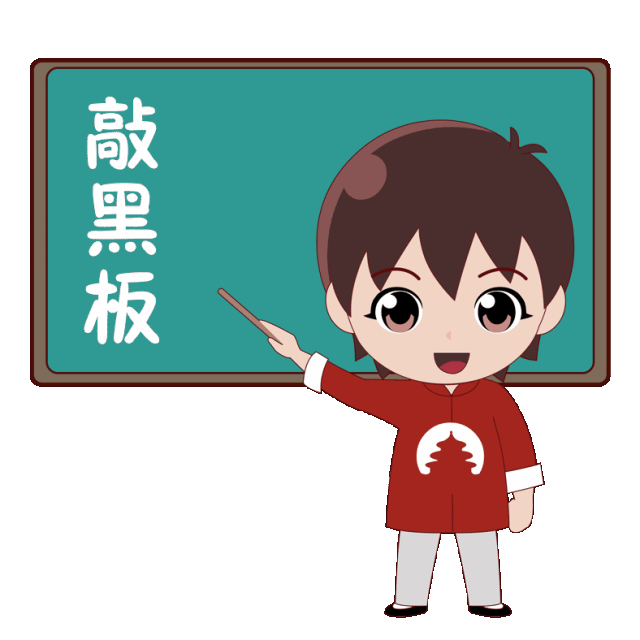
Series of articles:
Series 1: [Python automated test 1] meet the beauty of Python
Series 2: [Python automated test 2] Python installation configuration and basic use of PyCharm
series 3: [Python automated test 3] get to know data types and basic syntax
series 4: [Python automated test 4] string knowledge summary
Series 5: [Python automated test 5] list and tuple knowledge summary
series article 6: [Python automated test 6] dictionary and collection knowledge summary
series article 7: [Python automated test 7] data operator knowledge collection
series 8: [Python automated test 8] explanation of process control statement
series article 9: [Python automated test 9] function knowledge collection
2, Collection of file operations
2.1 explanation of open function
2.1.1 creation of open function
The open function is mainly used to open a file and create a file object. The most basic usage is as follows:
# There is a location parameter in the open function. We need to pass file and file name f = open("love.txt") # The open function also has a return value, which returns a file object print(f)
2.1.2 read all files
when we want to open a file, we always need to obtain the data in the file for use or reading. We can read the file through the read method in the open function. The read file type is string type:
# There is a location parameter in the open function. We need to pass file and file name f = open("love.txt") # To read a file, we use read, which can get the contents of the file read_my_file = f.read() print(read_my_file)
when the file is all in English, you can directly open it. If the file contains Chinese, Japanese, Korean and other contents, you must add utf-8 or utf8 after the open function, otherwise the error of Unicode decodeerror will appear in printing:
""" Wrong version, no utf-8 """ # There is a location parameter in the open function. We need to pass file and file name f = open("love.txt") # To read a file, we use read, which can get the contents of the file read_my_file = f.read() print(read_my_file) """ Correct version, plus utf-8,Able to identify non Chinese content """ # There is a location parameter in the open function. We need to pass file and file name f = open("love.txt", encoding="utf-8") # To read a file, we use read, which can get the contents of the file read_my_file = f.read() # The open function also has a return value, which returns a file object print(read_my_file)
2.1.3 read the first line of the file
in addition to reading all the contents of the file, we can also get the first line of the file. We can get the first line of the file through readline. Let's take a look at an error example first.
# There is a location parameter in the open function. We need to pass file and file name f = open("love.txt", encoding="utf-8") # To read a file, we use read, which can get the contents of the file read_my_file = f.read() print(read_my_file) # Read the first line of the file first = f.readline() print(f"The data of the first row obtained is{first}")
as shown in the above code and results, no content is actually printed. Python reads the data according to the cursor position. The main reason is that when we read all the contents of the file, the cursor is at the end. If we want to read the data in the first line, there are two ways. The first is to close the file and read again, The second method is to move the cursor to the initial position and read it. Let's demonstrate the first method first:
# There is a location parameter in the open function. We need to pass file and file name f = open("love.txt", encoding="utf-8") # To read a file, we use read, which can get the contents of the file read_my_file = f.read() # The open function also has a return value, which returns a file object print(read_my_file) # Close file f.close() # Reread the file in utf-8 format f = open("love.txt", encoding="utf-8") # Read the first line of the file first = f.readline() print(f"The data of the first row obtained is{first}")
in addition to closing the file directly, we can also move the cursor to the beginning position by moving the cursor. Use the seek function:
# There is a location parameter in the open function. We need to pass file and file name f = open("love.txt", encoding="utf-8") # To read a file, we use read, which can get the contents of the file read_my_file = f.read() # The open function also has a return value, which returns a file object print(read_my_file) # # Close file # f.close() # Reread the file in utf-8 format f = open("love.txt", encoding="utf-8") # Move the cursor to the initial position """ seek Followed by 0 represents the initial position of the cursor. 1 and 2 do not represent the second and third positions. Each number has different meanings. Generally speaking, 0 is more used for automatic testing If there are small partners who need to expand, you can have an in-depth understanding """ f.seek(0) # Read the first line of the file first = f.readline() print(f"The data of the first row obtained is{first}")
2.1.4 read all lines of the file and save them as readlines
readlines saves all rows and saves the data to a list. Each list element is a row of data, and there will be a \ n at the end of each element, indicating line feed here:
f = open("love.txt", encoding="utf-8") first = f.readlines() print(f"The list data obtained is:{first}")
2.1.5 file write mode (write)
write file we use the write function to write the contents of the file. Let's look at the following error example:
f = open("love.txt", encoding="utf-8") f.write("I love CSDN ")
The default mode parameter in the open function is r. when we want to write data, we need to modify it to the write mode. If you already have the file and have data content, each time you open the file and write in the write mode, the previous write will be overwritten. If there is no file, write directly after creation:
# Default mode="r", change the mode to w f = open("love.txt", encoding="utf-8", mode="w") f.write("I love CSDN ")
I believe you already know the disadvantages of the write mode. When there is important data, it is obvious that we may overwrite the important data with the write mode. Often our writing is new, such as new remarks, test cases, etc., so we need to use the add mode. We only need to change the parameter of the mode to a:
f = open("love.txt", encoding="utf-8", mode="a") f.write("I love China ")
generally speaking, a test case and a comment line are one, and we need to wrap the line to write data, so we can add data after the content written, so as to wrap the line:
f = open("love.txt", encoding="utf-8", mode="a") f.write("I love ShenZhen\n") f.write("I love NBA\n")
2.1.6 auto close file (with)
remember to close a file after we open it, otherwise others will not be able to open and use it, as shown in the following code:
f = open("love.txt", encoding="utf-8", mode="r") read = f.read() print(read) # close closes the file so that it can be successfully opened and used when needed next time. Otherwise, an error will appear f.close()
people... After all, they will forget some things... Write 100 times and 1000 times, and there will always be one or two times. Python is afraid that we will forget, and carefully prepare the with statement to prevent forgetting.
the author also recommends using the with statement. The principle of the with statement is colon: after all sub codes are executed, they automatically execute close to prevent forgetting.
""" The first way of writing, which we need to use close Close this file manually """ f = open("love.txt", encoding="utf-8", mode="r") read = f.read() f.close() """ The second way of writing, through with Statement, you don't need to use close Close the file to prevent forgetting to close the file and causing some trouble of error reporting in the future Format: with open(File parameters,xxx,xxx) as Variable name: """ with open("love.txt", encoding="utf-8") as f: f.read()
OK ~ the above is all the content shared in this article. Have you learned it? I hope it can help you!