Python Basics - day 6
Slicing operations for lists
Indexing and slicing
Positive index: 0 ~ N-1 / negative index: - N ~ -1
nums = [1, 2, 3, 4, 5, 6] print(nums[2:]) # Gets the elements after index 2, including the elements with index 2 print(nums[::]) # Get all elements print(nums[::-1]) # Invert all elements print(nums[1:3]) # Gets the element between 1 - 3, excluding the element with index 3 print(nums[2:7:2]) # Get the element in the middle of 2 - 7 in steps of 2 print(nums[5:15]) # Only elements with index 5 can be obtained print(nums[1:2]) # Gets the element in the middle of 1 - 2
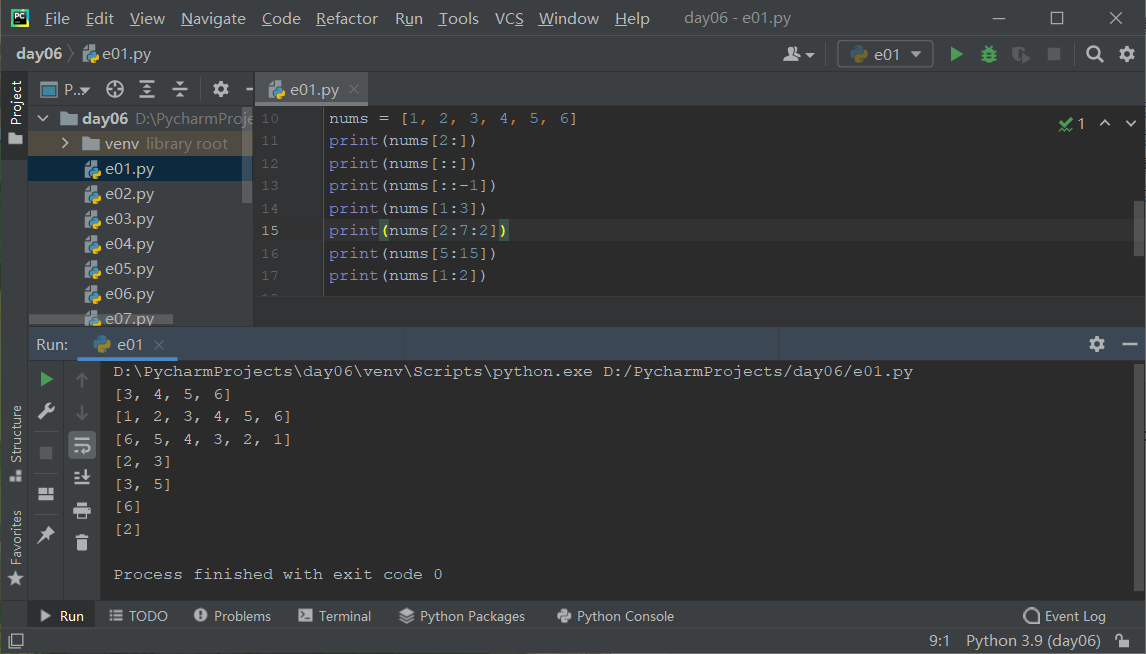
List related operations
# Create list method 1: literal syntax list1 = ['apple', 'orange', 'hello', 'world', 'pitaya'] print(list1) # Method 2 of creating list: constructor syntax list2 = list(range(1, 10)) print(list2) # Three ways to create a list: generative (deductive) syntax list3 = [i ** 2 for i in range(1, 10)] print(list3)
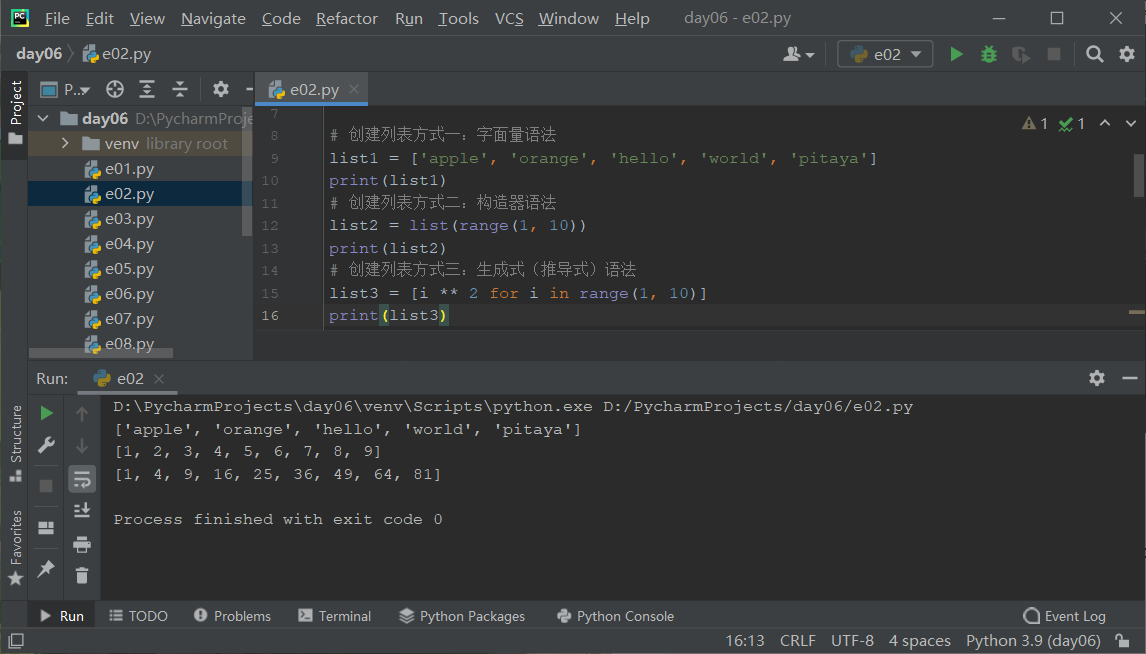
Using the list generation formula (derivation), you can quickly generate a list. You can deduce another list from one list, but the code is very concise.
# Get the number of list elements -- > len() print(len(list3))
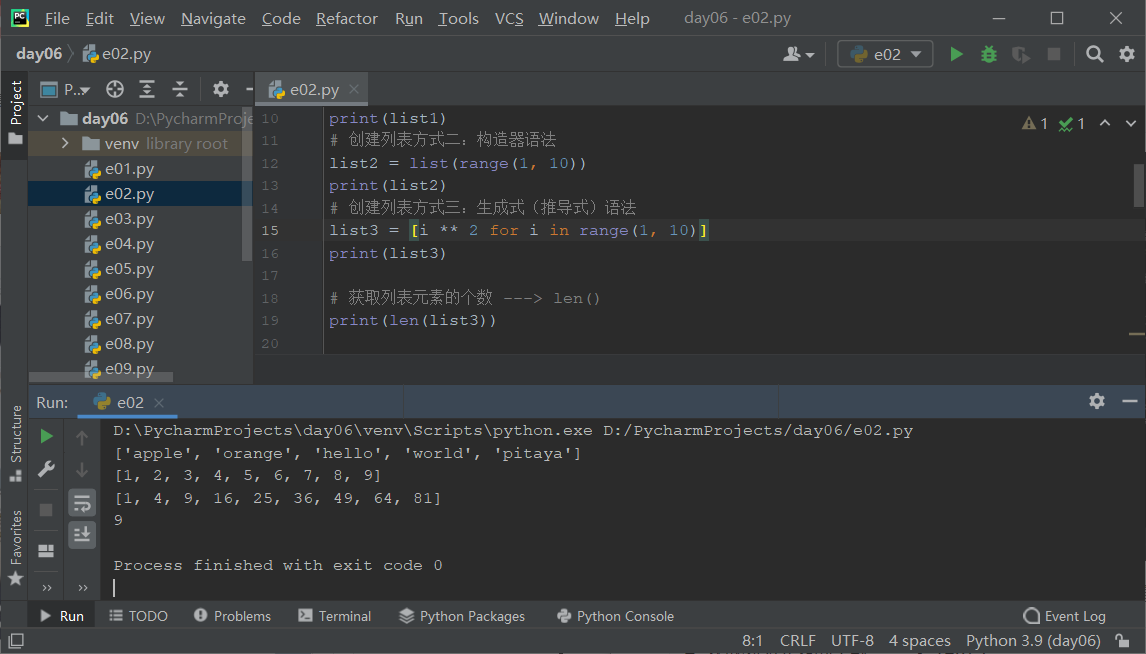
# Traverse the elements in the list for i in range(len(list1)): print(list1[i]) for x in list1: print(x) for i, x in enumerate(list1): print(i, x)
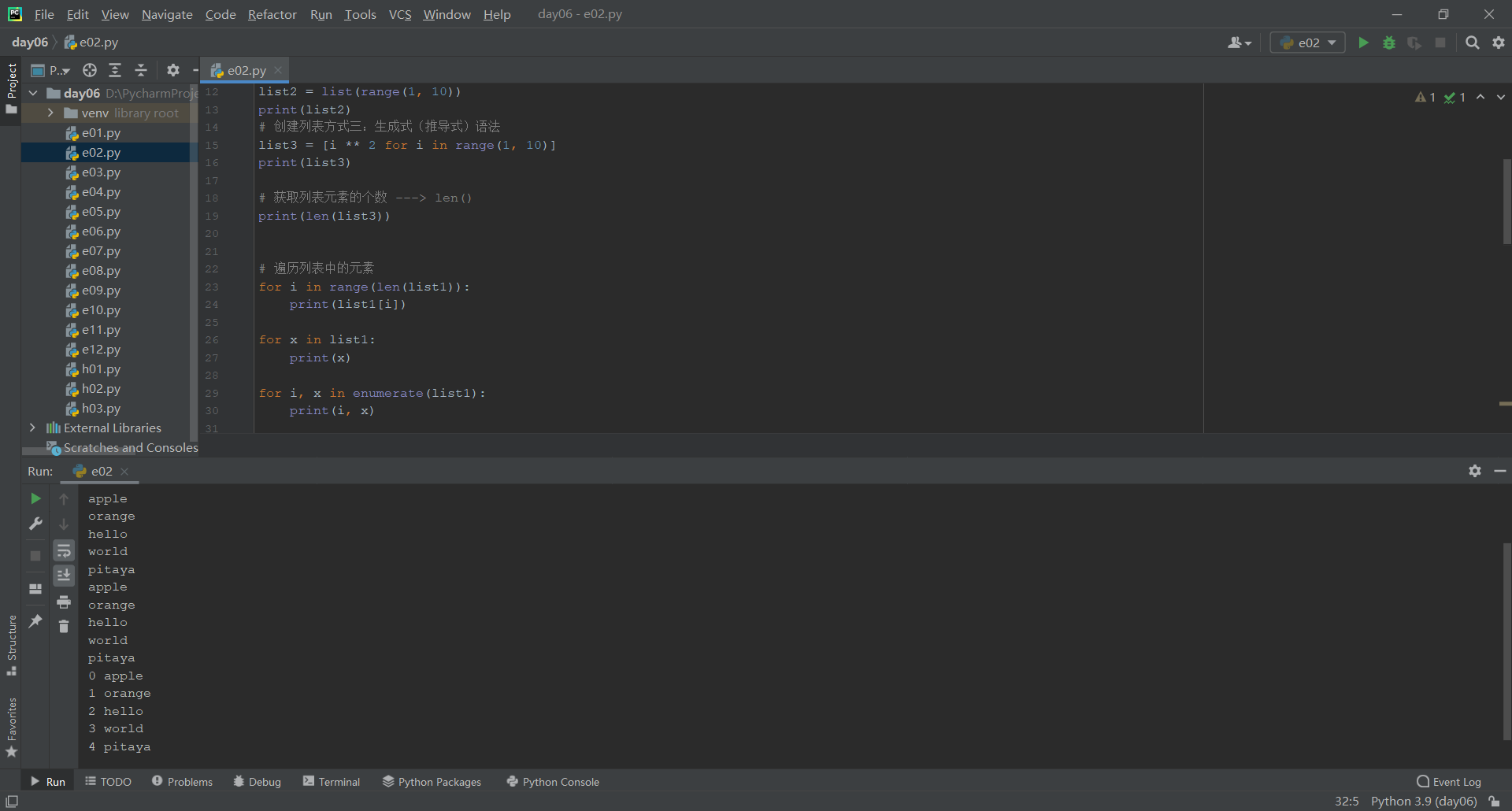
List dependent operation
# Repeat operation list4 = [1, 10, 100] * 5 print(list4) # Member operation -- > in / not in -- > true / false print(10 in list4) print(5 in list4) print(5 not in list4) print('apple' in list1) # merge list5 = [1, 2, 2, 3] list6 = [2, 3, 4] temp = list5 + list6 print(temp) list5 += list6 print(list5) list6.extend(list5) print(list6) # compare list7 = list(range(1, 8, 2)) list8 = [1, 3, 5, 7, 9] list9 = [0, 3, 5, 7] print(list7 == list8) # Compare the size of the elements corresponding to the two lists print(list7 > list8) print(list9 > list7)
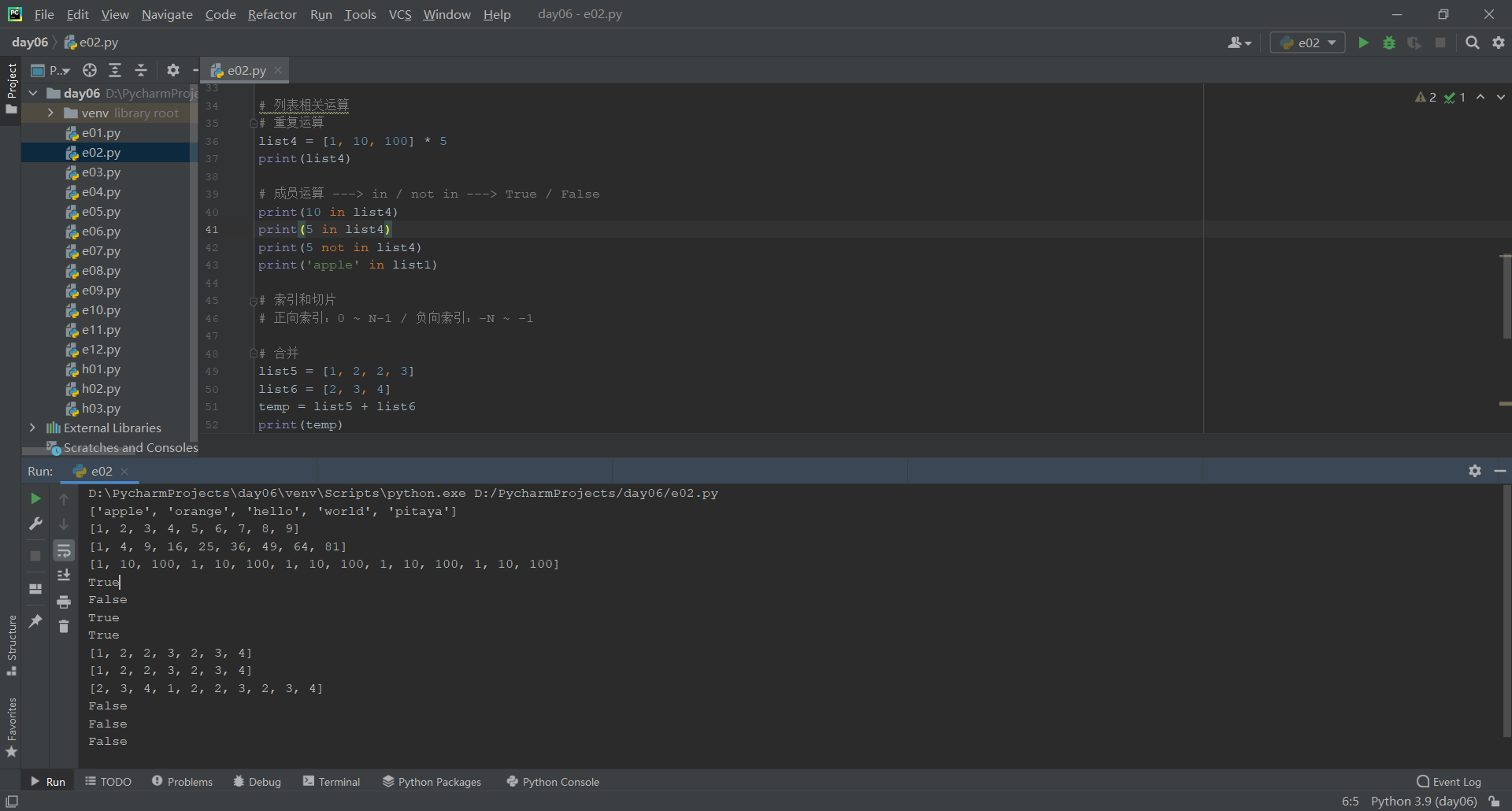
Chinese character viewing (0x4e00, 0x9fa6)
ord() - > view the encoding corresponding to the character
chr() - > process the encoding into corresponding characters
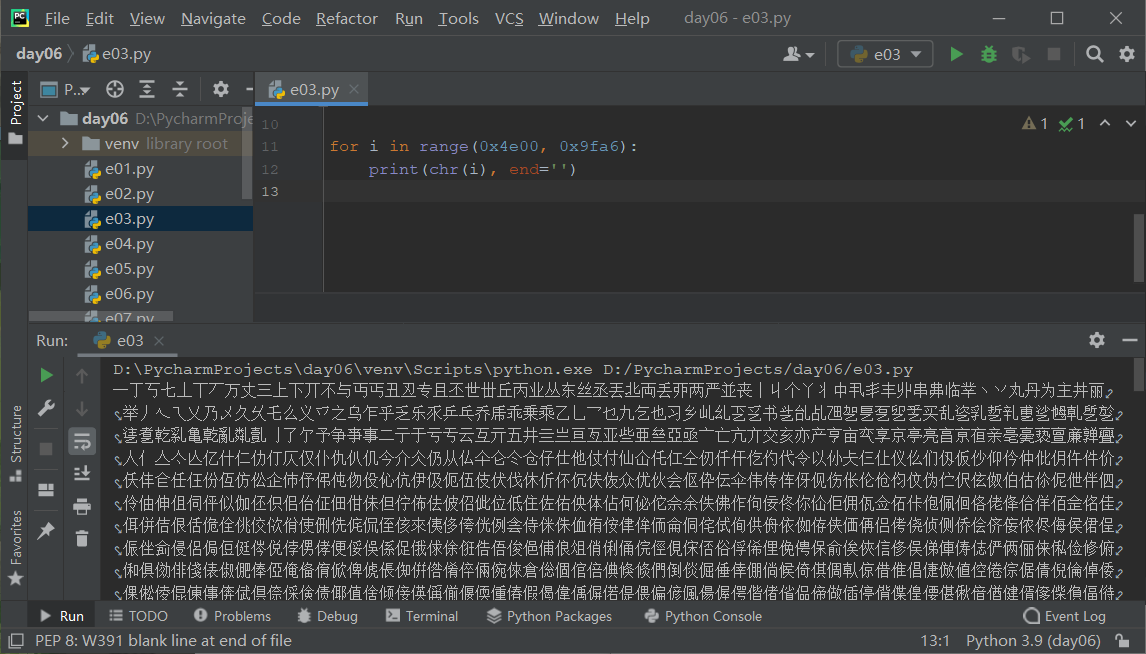
List related operations (find, count, add, delete, empty)
items = ['banana', 'grape', 'apple', 'waxberry', 'pitaya', 'apple'] # Index() --- > find the index (subscript) of the element in the list if 'strawberry' in items: print(items.index('strawberry')) if 'apple' in items: print(items.index('apple')) if 'apple' in items[3:]: print(items.index('apple', 3)) # Count() --- > counts the number of occurrences of elements in the list print(items.count('apple')) print(items.count('strawberry')) # Add element items.append('blueberry') items.insert(1, 'watermelon') print(items) # Delete element items.pop() items.pop(4) del items[0] while 'apple' in items: items.remove('apple') print(items) # Empty list elements items.clear() print(items)
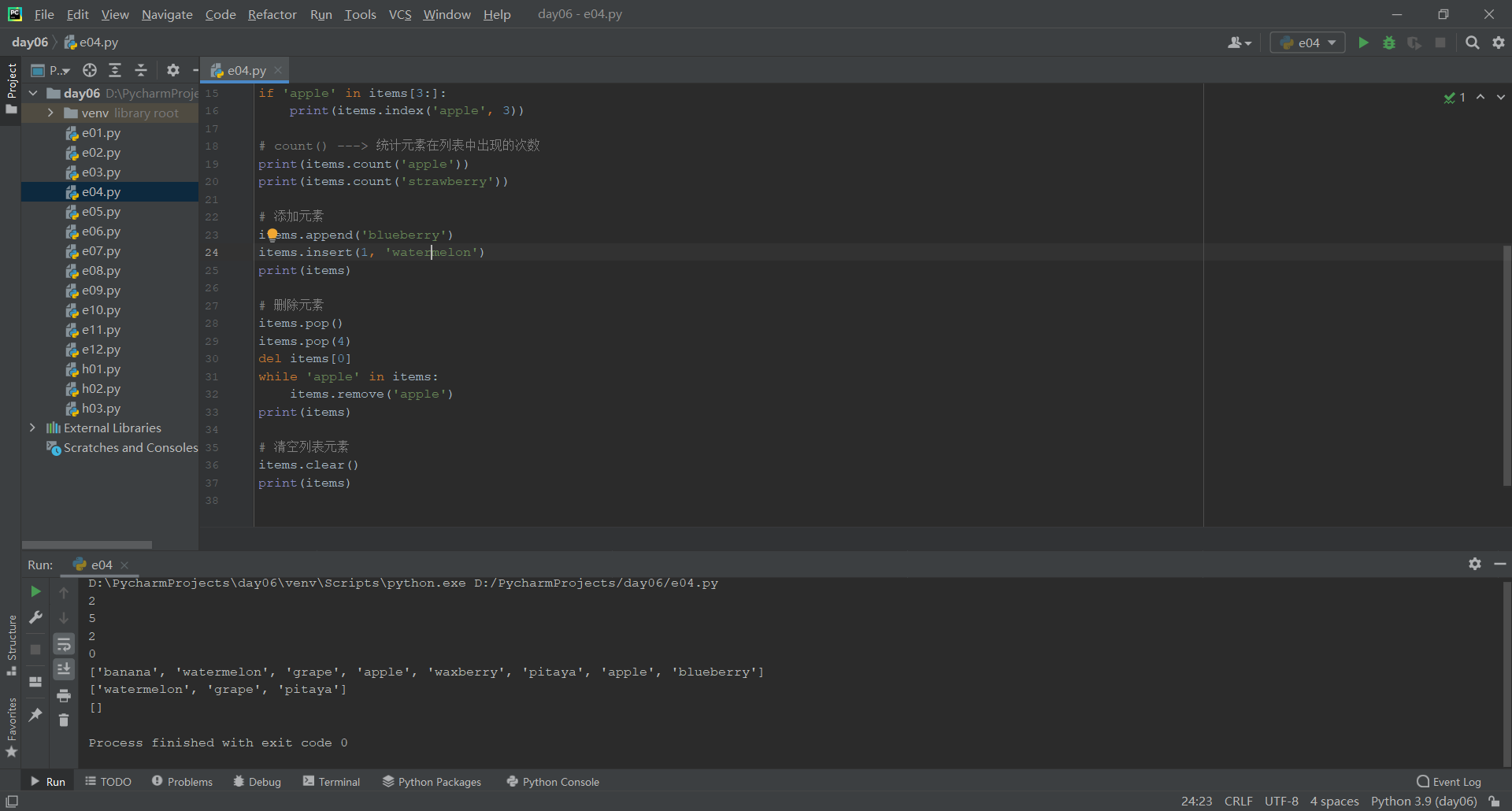
List operations (reverse and sort)
items = ['banana', 'grape', 'apple', 'waxberry', 'pitaya', 'apple'] # reversal items.reverse() print(items) # Sorting (you can modify the revers e parameter to control ascending or descending sorting) # items.sort(reverse=True) items.sort() print(items) nums = ['1', '10', '234', '2', '35', '100'] nums.sort(key=int) print(nums)
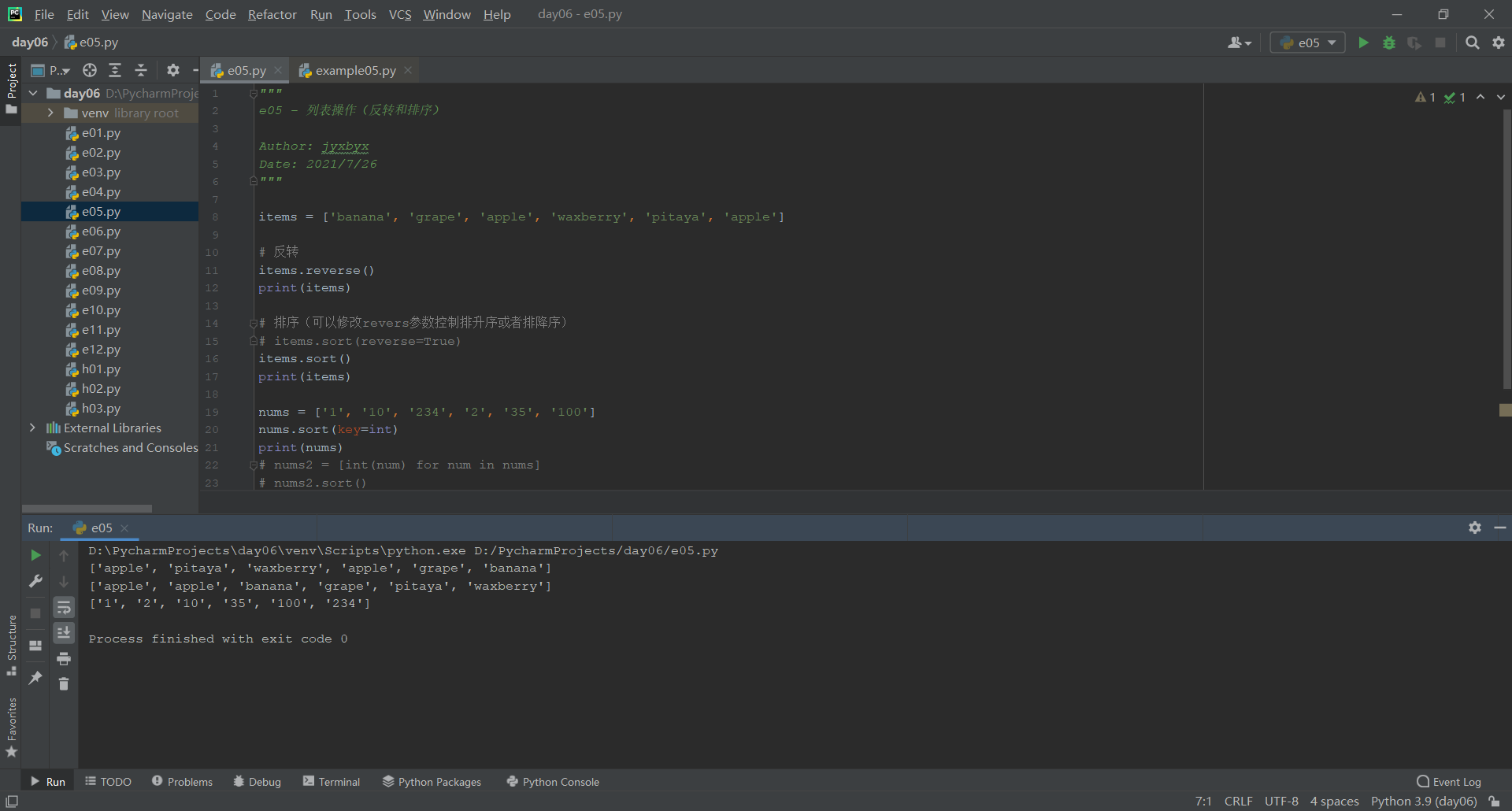
Simple selection sorting:
Select the smallest value from the remaining elements each time
nums = [35, 12, 99, 58, 67, 42, 49, 31, 73] for i in range(len(nums) - 1): # Suppose the first element is the minimum min_value, min_index = nums[i], i # Loop to find if there is a smaller value and note its location for j in range(i + 1, len(nums)): if nums[j] < min_value: min_value, min_index = nums[j], j # Change the minimum value to the front position nums[i], nums[min_index] = nums[min_index], nums[i] print(nums)
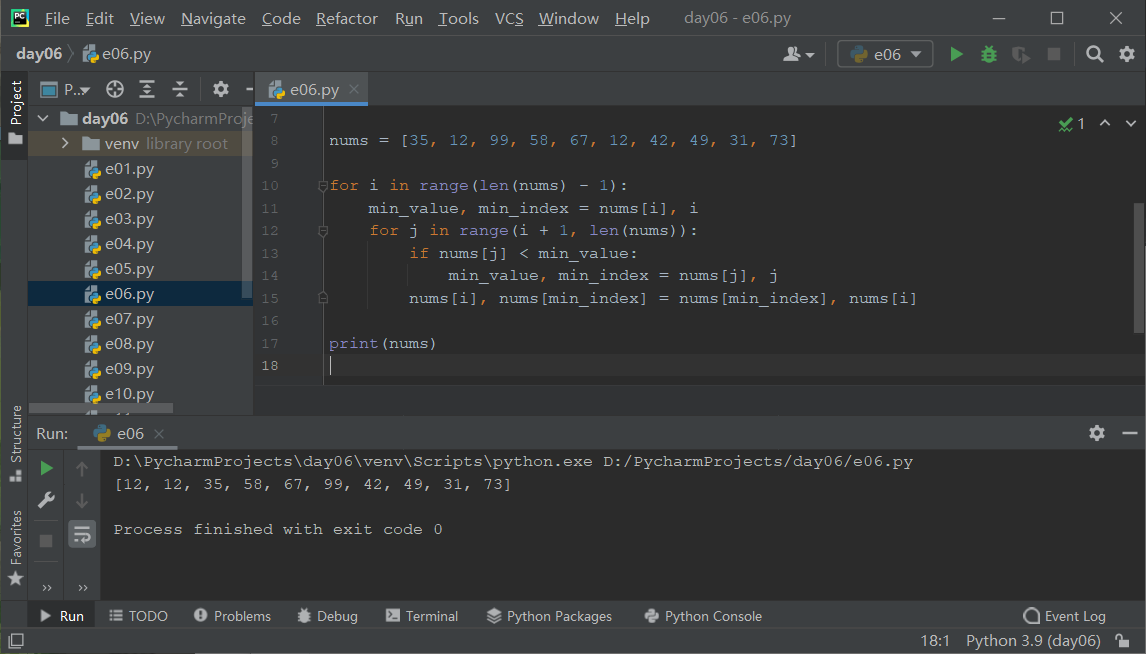
Bubble sort:
Elements are compared in pairs. If the previous element is larger than the subsequent element, the positions of the two elements are exchanged
nums = [35, 12, 99, 58, 67, 42, 49, 31, 73] for j in range(1, len(nums)): swapped = False for i in range(0, len(nums) - j): if nums[i] > nums[i + 1]: nums[i], nums[i + 1] = nums[i + 1], nums[i] swapped = True if not swapped: break print(nums)
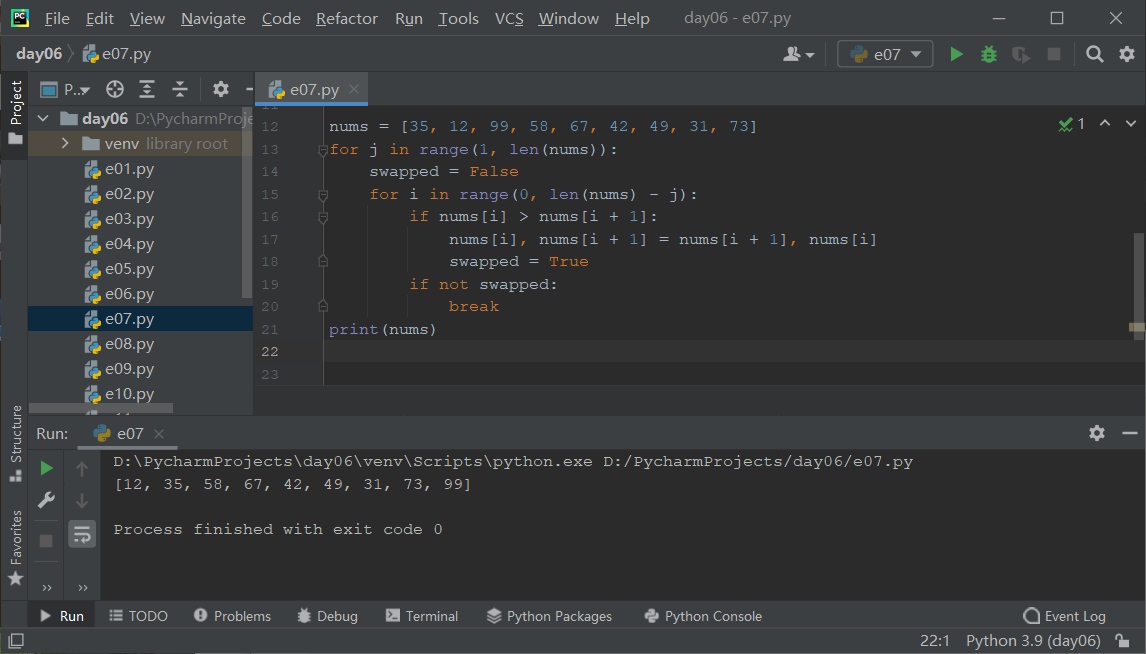
Random extraction and out of order
names = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'l', 'm'] # The sample function can sample list elements without putting them back print(random.sample(names, k=5)) # The choices function samples the list elements back print(random.choices(names, k=5)) # The choice function can randomly select an element from the list print(random.choice(names)) # shuffle function can realize random disorder of list elements random.shuffle(names) print(names)
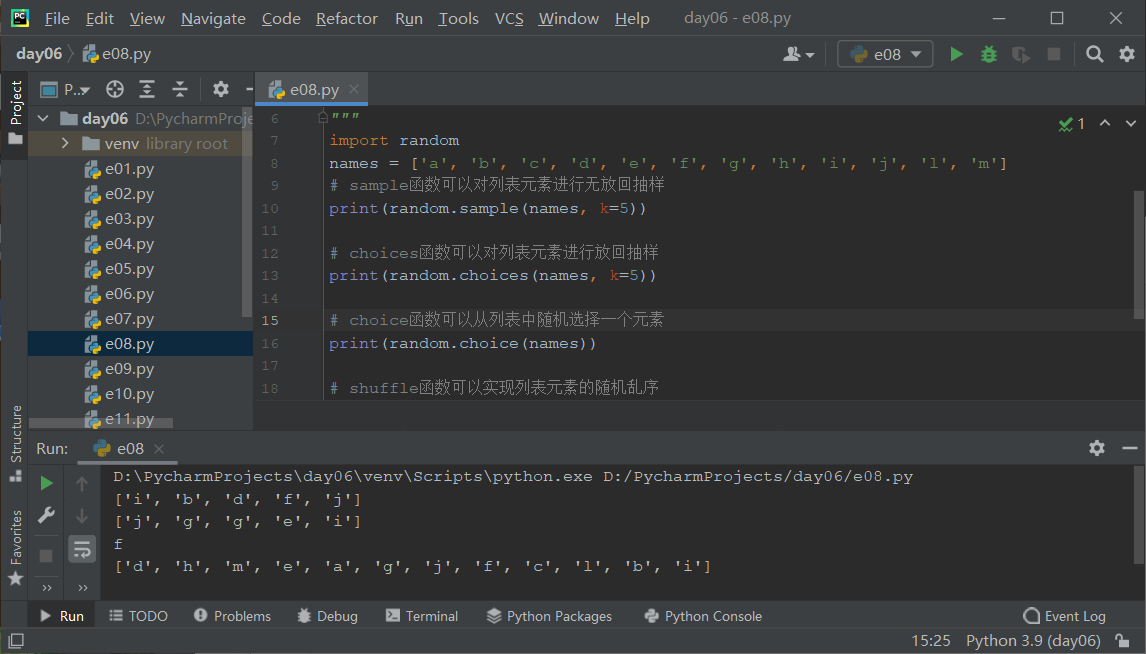
Exercise 1:
Lucky woman (Josephu ring)
15 men and 15 women went to sea by boat. The boat broke down and 15 of them needed to be thrown into the sea so that the others could survive; All people form a circle, and one person counts off from 1. The person who reports 9 is thrown into the sea, and the next person counts off from 1 until 15 people are thrown into the sea. Finally, 15 women survived and 15 men were thrown into the sea. Ask which positions were men and which positions were women.
Method 1:
persons = [True] * 30 index, counter, number = 0, 0, 0 while counter < 15: if persons[index]: number += 1 if number == 9: persons[index] = False counter += 1 number = 0 index += 1 if index == 30: index = 0 for person in persons: # Ternary conditional operation -- > the expression after if is True, take the value before if, otherwise go to the value after else print('female' if person else 'male', end=' ')
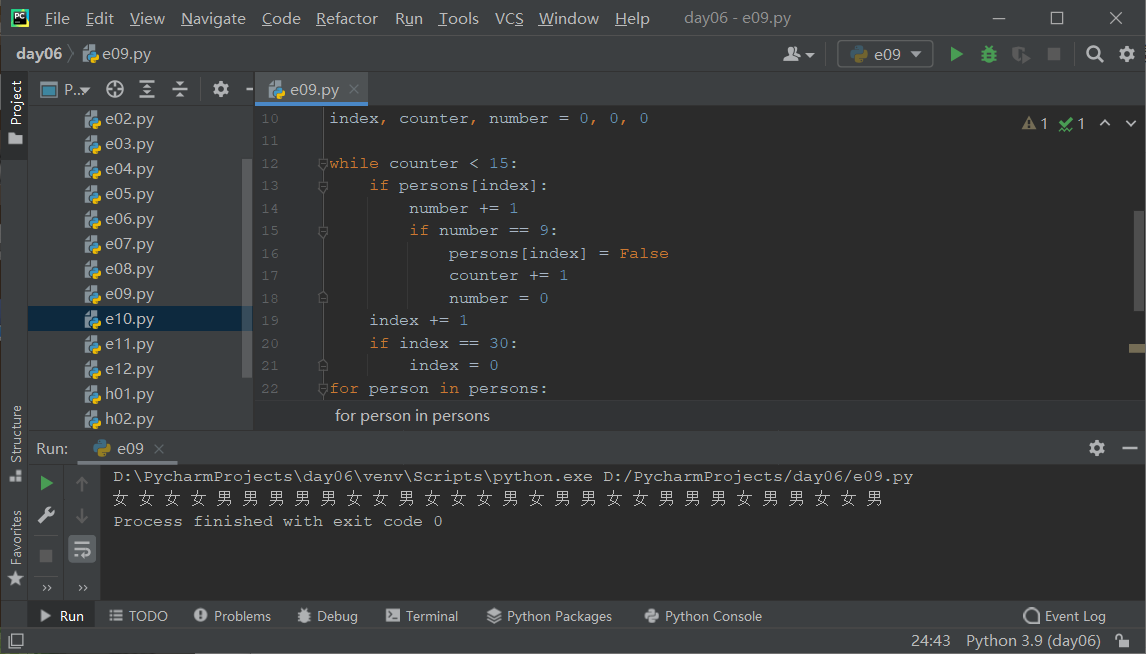
Method 2:
persons = [i for i in range(1, 31)] for _ in range(15): persons = persons[9:] + persons[:8] print(persons) for i in range(1, 31): print('female' if i in persons else 'male', end='')
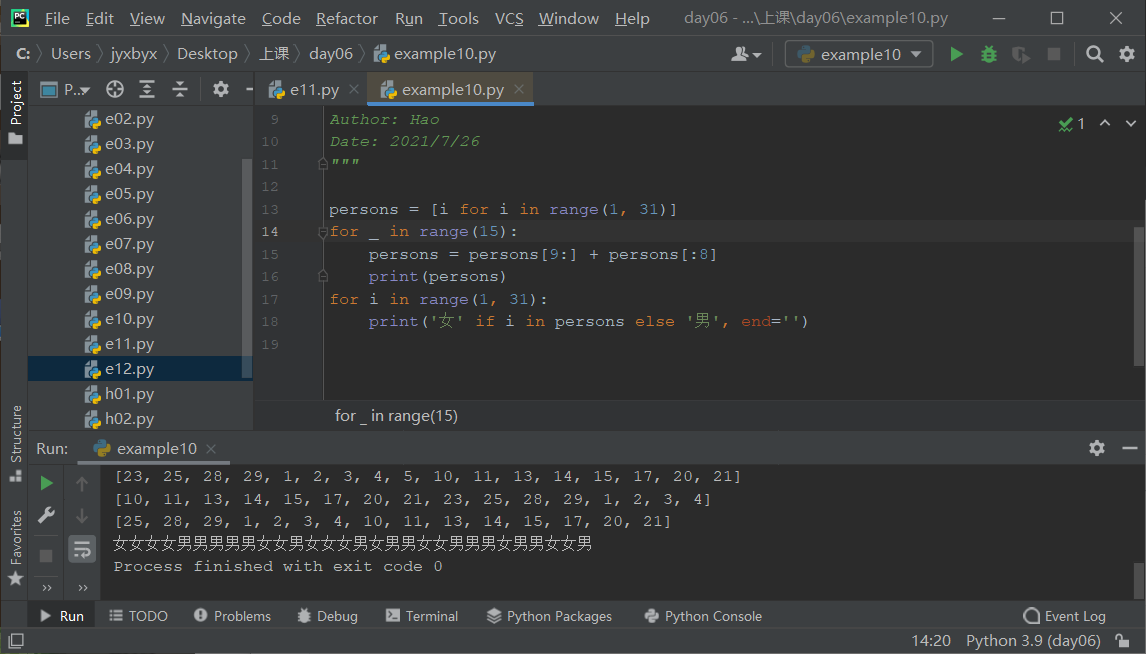
Exercise 2:
Save 54 playing cards in a list, shuffle, distribute the cards to three players according to the licensing method of fighting the landlord, and give the additional 3 cards to the first player (landlord), so as to display the cards in each player's hand
import random suites = ['♠', '🖤', '♣', '♦'] faces = ['A', '2', '3', '4', '5', '6', '7', '8', '9', '10', 'J', 'Q', 'K'] cards = [f'{suite}{face}' for suite in suites for face in faces] cards.append('king') cards.append('Xiao Wang') random.shuffle(cards) # players = [[], [], []] players = [[] for _ in range(3)] for _ in range(17): for player in players: player.append(cards.pop()) players[0].extend(cards) for player in players: player.sort(key=lambda x: x[1:]) for card in player: print(card, end=' ') print()
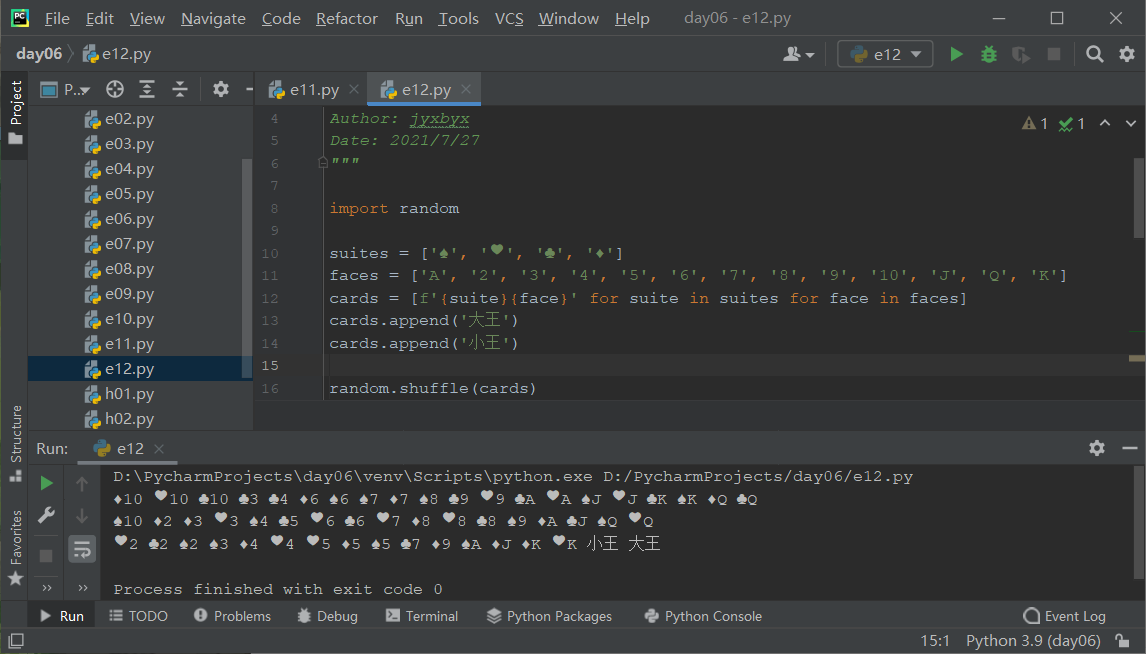
Exercise 3:
Enter three integers and output them in descending order
nums = [] for _ in range(3): temp = int(input('Please enter data:')) nums.append(temp) for j in range(2): for i in range(2): if nums[i] < nums[i + 1]: nums[i], nums[i + 1] = nums[i + 1], nums[i] print(nums)
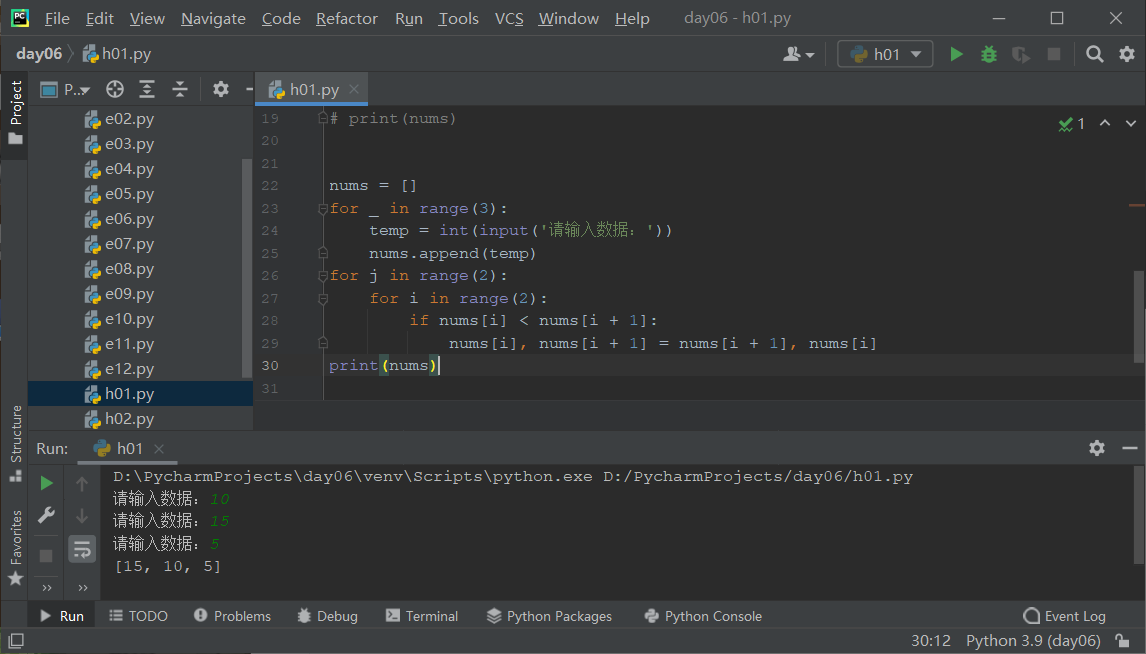
Exercise 4:
Add 10 random integers to the list and find the second largest element
[a, b) —> random.random() * (b - a) + a
import random # Generating formula of list (derivation) - > the writing method is concise and more efficient nums = [random.randrange(1, 100) for _ in range(10)] # nums = [i for i in range(1, 101, 2)] print(nums) m1, m2 = nums[0], nums[1] if m1 < m2: m1, m2 = m2, m1 for num in nums[2:]: if num > m1: m1, m2 = num, m1 elif num == m1: pass elif num > m2: m2 = num print(m2)
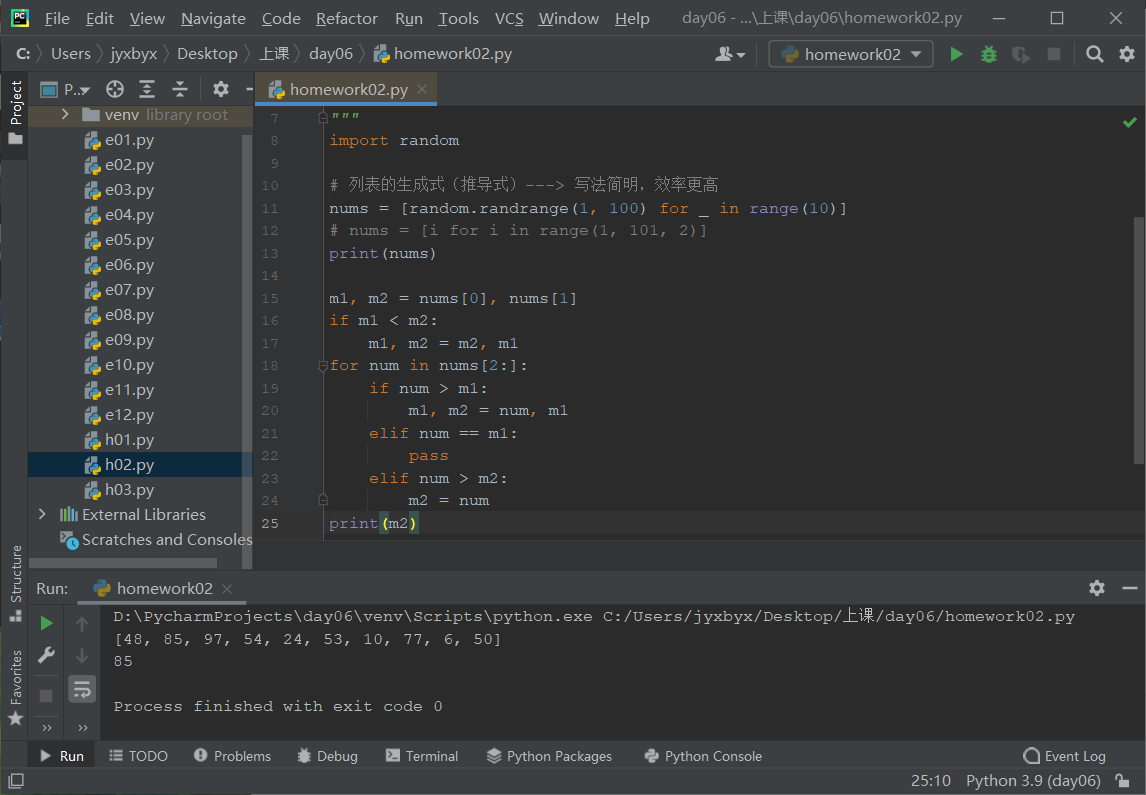
Exercise 5:
21 matches game: there are 21 matches. People and computers take turns to take them. People take them first (input several) and then the computer, at least one at a time and up to four at a time,
If you get the last match, you'll lose. Make sure the computer can win!
match = 21 while match > 0: user = int(input('Please enter:')) if 0 < user <= 4: match = match - user else: print('Input error') continue if match <= 0: print('You lost') break while match > 0: # computer = random.randrange(1, 5) computer = 5 - user print(f'Take the computer{computer}root') match = match - computer if match <= 0: print('The computer lost') break print('game over')
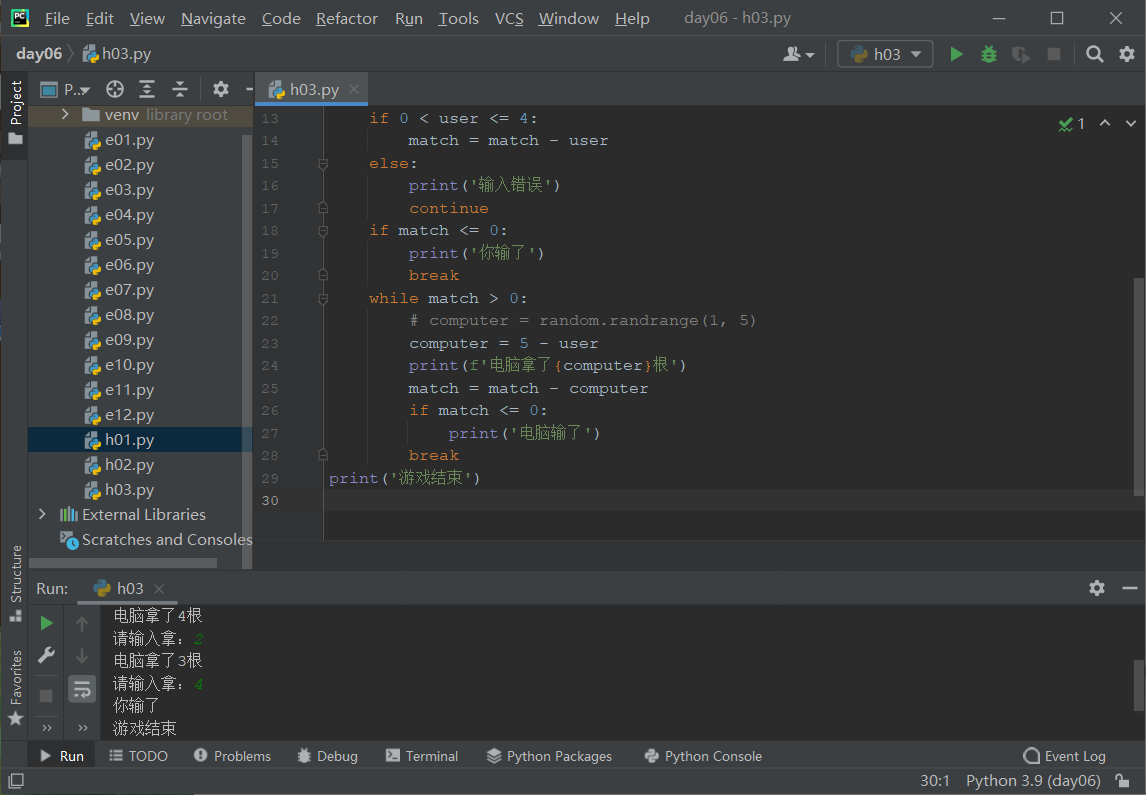