target
- Concept of exception
- Catch exception
- Exception passing
- Throw exception
01. Concept of abnormality
- When the program is running, if the Python interpreter encounters an error, it will stop the execution of the program and prompt some error messages, which is an exception
- The action that the program stops executing and prompts an error message is usually called raising an exception
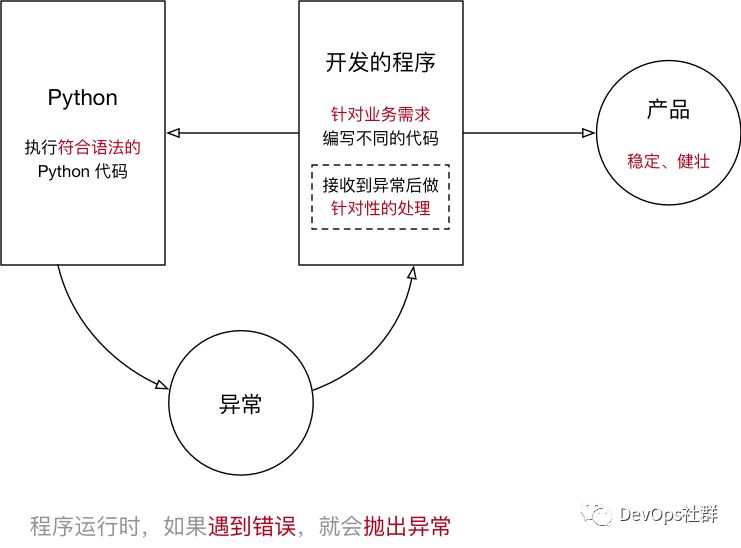
During program development, it is difficult to deal with all special cases. Through exception capture, we can deal with emergencies intensively, so as to ensure the stability and robustness of the program
02. Catch exception
2.1 simple exception capture syntax
- In program development, if the execution of some code is not sure whether it is correct, try can be added to catch exceptions
- The simplest syntax format for catching exceptions:
try: Code attempted to execute except: Handling of errors
- Try, write the code to try below, and you are not sure whether it can execute normally
- except if not, write the code below that the attempt failed
Simple exception capture exercise - fat boss: tell me how much you want to buy cigarettes
In [2]: try: ...: price = int(input("Fat boss: how much do you want to buy cigarettes:")) ...: except: ...: print("Please enter the correct number") ...: Fat boss: how much do you want to buy cigarettes:12 In [4]: try: ...: price = int(input("Fat boss: how much do you want to buy cigarettes:")) ...: except: ...: print("Please enter the correct number") ...: Fat boss: how much do you want to buy cigarettes:sad Please enter the correct number
2.2 error type capture
- During program execution, you may encounter different types of exceptions, and you need to make different responses to different types of exceptions. At this time, you need to catch the error type
- The syntax is as follows:
try: # Code attempted to execute pass except Error type 1: # Corresponding code processing for error type 1 pass except (Error type 2, Error type 3): # Corresponding code processing for error types 2 and 3 pass except Exception as result: print("unknown error %s" % result)
- When the Python interpreter throws an exception, the first word in the last line of error message is the error type
In [5]: price = int(input("Fat boss: how much do you want to buy cigarettes:")) Fat boss: how much do you want to buy cigarettes:asdasd --------------------------------------------------------------------------- NameError Traceback (most recent call last)
As can be seen from the above, the error type is NameError
####Exception type capture drill - fat boss: can you give an integer for the cigarette money you give?
demand
- Prompt for an integer
- Check whether NameError is abnormal
In [6]: try: ...: price = int(input("Fat boss: how much do you want to buy cigarettes:")) ...: except NameError: ...: print("NameError abnormal") ...: Fat boss: how much do you want to buy cigarettes:asdsa NameError abnormal In [7]:
Catch unknown error
- During development, it is still difficult to predict all possible errors
- If you want the program not to be terminated because the Python interpreter throws an exception regardless of any error, you can add another exception The syntax is as follows:
except Exception as result: print("unknown error %s" % result)
- 2.3 complete syntax of exception capture - fat boss asks for cigarette money, and the last complete exception version
- In actual development, in order to handle complex exception situations, the complete exception syntax is as follows:
Tips:
- The application scenarios of complete grammar will be better understood in the follow-up study combined with actual cases
- Now just have an impression of the grammatical structure
try: # Code attempted to execute pass except Error type 1: # Corresponding code processing for error type 1 pass except Error type 2: # Corresponding code processing for error type 2 pass except (Error type 3, Error type 4): # Corresponding code processing for error types 3 and 4 pass except Exception as result: # Print error message print(result) else: # Code that executes without exceptions pass finally: # Code that executes regardless of whether there are exceptions print("Code that executes regardless of whether there are exceptions")
- else code that executes only when there are no exceptions
- finally, the code will be executed whether there is an exception or not
- Then write a complete code to catch exceptions when the fat boss asks the price, as follows:
In [9]: try: ...: price = int(input("Fat boss: how much do you want to buy cigarettes:")) ...: except NameError: ...: print("NameError abnormal") ...: except Exception as result: ...: print("unknown error %s" % result) ...: else: ...: print("Fat boss: I want this%s The price, right" % price) ...: finally: ...: print("Fat boss: if you have no money, I can give you a bag.") ...: Fat boss: how much do you want to buy cigarettes:17 Fat boss: do you want this 17 price Fat boss: if you have no money, I can give you a bag. In [10]: try: ...: price = int(input("Fat boss: how much do you want to buy cigarettes:")) ...: except NameError: ...: print("NameError abnormal") ...: except Exception as result: ...: print("unknown error %s" % result) ...: else: ...: print("Fat boss: I want this%s The price, right" % price) ...: finally: ...: print("Fat boss: if you have no money, I can give you a bag.") ...: Fat boss: how much do you want to buy cigarettes:asdasd NameError abnormal Fat boss: if you have no money, I can give you a bag. In [11]:
03. Abnormal transmission - fat boss: how much do you want to buy cigarettes
- Exception passing - when an exception occurs in the execution of a function / method, the exception will be passed to the caller of the function / method
- If it is passed to the main program and there is still no exception handling, the program will be terminated
Tips
- In development, exception capture can be added to the main function
- Other functions that are called in the main function are passed to the exception capture of the main function as long as there is an exception.
- In this way, there is no need to add a large number of exception capture in the code, which can ensure the cleanliness of the code demand
- Define function demo1() fat boss: how much do you want to buy cigarettes
- Define function demo2() to call demo1()
- Call demo2() in the main program.
In [11]: def demo1(): ...: return int(input("Fat boss: how much do you want to buy cigarettes:")) ...: In [12]: def demo2(): ...: return demo1() ...: In [13]: In [13]: try: ...: print(demo2()) ...: except NameError: ...: print("NameError abnormal") ...: except Exception as result: ...: print("unknown error %s" % result) ...: Fat boss: how much do you want to buy cigarettes:asd NameError abnormal In [14]: try: ...: print(demo2()) ...: except NameError: ...: print("NameError abnormal") ...: except Exception as result: ...: print("unknown error %s" % result) ...: Fat boss: how much do you want to buy cigarettes:17 17 In [15]:
It can be seen from the above code that the exception of demo1 can be passed to demo2 for processing.
04. Throw a 'raise' exception - fat boss: collect enough money and throw an exception if it's not enough
Note: throw an exception, not a wink
4.1 application scenarios
- In development, in addition to code execution errors, the Python interpreter will throw exceptions
- You can also actively throw exceptions according to the unique business requirements of the application Example
- Prompt the user to enter cigarette money. If the price is less than 18, an exception will be thrown be careful
- The current function is only responsible for prompting the user to enter cigarette money. If the price of cigarette money is lower than 18 yuan, other functions are required for additional processing
- Therefore, exceptions can be thrown and caught by other functions that need to be handled
4.2 throw exception
- An Exception exception class is provided in Python
- During development, if you want to throw an exception when meeting specific business requirements, you can:
- Create an Exception object
- Throw an exception object using the raise keyword demand
- Define input_ The price function prompts the fat boss: collect the money
- If the user enters a price < 18, an exception is thrown
- If the user enters the price > = 18, return to fat boss: your cigarette is loyal to your lanli group
In [2]: def input_price(): ...: ...: # Collect money ...: price = input("Fat boss: take the money") ...: ...: # See if there's enough money ...: if int(price) >= 18: ...: return price ...: ...: # There is not enough money. You need to throw an exception ...: # 1> Create exception object - use the exception's error message string as a parameter ...: ex = Exception("The money is not enough") ...: ...: # 2> Throw exception object ...: raise ex ...: In [3]: In [3]: try: ...: price = input_price() ...: print(price) ...: except Exception as result: ...: print("Errors found:%s" % result) ...: Fat boss: charge 18 18 In [4]: try: ...: price = input_price() ...: print(price) ...: except Exception as result: ...: print("Errors found:%s" % result) ...: Fat boss: collect money 17 Error found: not enough money In [5]: