Python Flask -- II (routing, jinji2 syntax)
1, Using routing and view functions
1.1 concept of routing
The client (Web browser) sends a network request to the Web server, and the Web server forwards the request to the flash program instance. The application instance needs to know which code to run for each URL request. Therefore, a mapping relationship between URL and Python function is saved. The program that handles the relationship between URLs and functions is called routing
1.2 define a route
To define a route, you need to use the app.route modifier to register the modified function as a route. Matched with it is the view function, which is used by Flask to process the client's request and return data
from flask import Flask app = Flask(__name__) @app.route("/") def hello(): return "Hello World" if __name__ == '__main__': app.run()
Here is an explanation of the above code. We use the hello() function to register the handler as the root address when the server receives a message from http://localhost:5000 For the network request, the flash example will find the view function (Hello function) under the root directory, and then give the return value to the client.
1.3 using dynamic routing
We can specify parameters in the passed url, and then the parameters we enter in the address bar can be displayed in the web page, which can be realized by using specific syntax
- Incoming dynamic parameters
- Pass in default parameters
A little more is added here. A view function can bind multiple URL s
from flask import Flask app = Flask(__name__) @app.route("/") def hello(): return "Hello World" # Here, we can pass in the name parameter in the address bar, and then receive this parameter in the view function and display the data @app.route("/user", defaults={'name':'Programmer'}) @app.route("/user/") def user(name): return f"Hello {name} " # Specify the parameter type passed in by the user. This can be int, float, and other types of data @app.route('/user/') def user1(num): return f"The parameter passed in is {num} " if __name__ == '__main__': app.run()

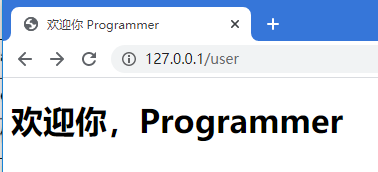
Common variable converters in URL processing
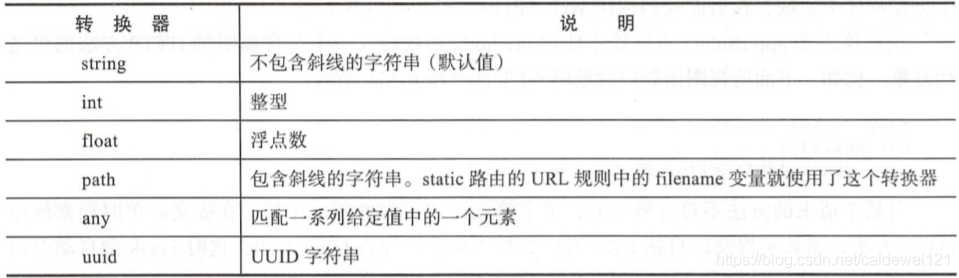
1.4 response of view function
Parameter 1: response content
After the 'flash' instance calls the view function, it will take its return value as the response content. The content of the response contains simple strings, json data, xml data, and html pages to the client
Parameter 2: http status code
But an important part of http protocol is the response status code. The default status code in flip is 200. In fact, we can change this manually, such as changing 404 manually to invalidate access
Parameter 3: header object
There is generally no need to set here, but if we want to return data in a specified format, such as html, we need to add content type: text / html in the header. json data is json, and there are many others
1.4.1 generate redirect response
When you visit this route, you will jump to Baidu's home page
from flask import Flask,redirect app = Flask(__name__) @app.route("/hello") def hello(): return redirect('http://www.baidu.com') @app.route('/hi') def hi(): return redirect(url_for('hello')) # Redirect to / hello app.run()
1.4.2 return JSON data
from flask import Flask, make_response import json app = Flask(__name__) # This method is not commonly used @app.route("/api/json1") def json1(): data = { "name":"Gelly", "age":18 } # Generates the specified response response = make_response(json.dumps(data)) # Specifies that the returned data is json response.mimetype = 'application/json' return response # json serialization is implemented using the json function provided by flash @app.route("/api/json2") def json2(): data = { "name":"Gelly", "age":18 } return jsonify(data) if __name__ == '__main__': app.run(host="127.0.0.1",debug=True,port=5000)
Return results

1.5 custom error page
Like regular routing, flash allows the program to customize the error page based on the template. The most commonly used status code is another 404500. The following is an example
@app.errorhandler(404) def page_not_found(e): return render_template('404.html'),404 @app.errorhandler(500) def internal_server_error(e): return render_template('500.html'),500
When we visit a page that does not exist, we will be prompted that the page does not exist
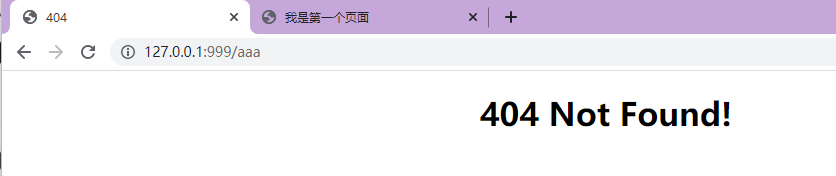
1.6 route request method listening (supplementary update)
By default, the route only listens to GET methods. If we need to listen to other request methods, we need to specify them in the attribute methods
We only need to add a methods parameter to the decorator to implement other requests
If we need to return different data according to different request methods, we can use the request.method method method
from flask import Flask,request app = Flask(__name__) @app.route("/", methods=['POST','GET']) def hello(): # If it is a post request, the following parameters are used # Specify the request and return different data if request.method == 'POST': return "Hello" else: return 'Hello World' app.run()
Two. Template syntax
2.1 initial experience of jinjia2 template
First, create a templates directory in the root directory of the flash project, and then write two simple HTML files, index.html and user.html
index.html
<html lang="en"> <head> <meta charset="UTF-8"> <title>I'm the first page title> head> <body> <h1>Hello Worldh1> body> html>
user.html
<html lang="en"> <head> <meta charset="UTF-8"> <title>Welcome {{name}}title> head> <body> <h1>Welcome,{{name}}h1> body> html>
Then modify the view function and use render_template causes the templates sub file in the flash program directory to search for the corresponding file.
render_ The first parameter of template corresponds to the template file name, and the subsequent parameters are key value pairs, representing the corresponding real values in the template
from flask import render_template @app.route("/") def hello(): # Render template return render_template('index.html') # Dynamic routing @app.route("/user/") def user(name): return render_template('user.html', name=name) # The first parameter of name corresponds to {{name}} in the template as the occupancy lease, and the second parameter corresponds to the value of the placeholder
Display effect (root page)

Template page
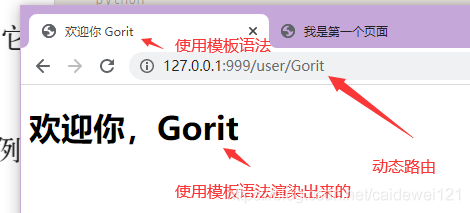
2.2 variables
In the above example, I used the {name}} structure to represent a variable, which belongs to a special placeholder. It will tell the template that the value of this position is obtained from the data used when rendering the template
Jinjia2 can recognize all types of variables, even some complex data structures, such as lists, dictionaries and objects. The following examples use
Content rendering of view function:
render_ The first parameter of template corresponds to the template file name, and the subsequent parameters are key value pairs, representing the corresponding real values in the template
@app.route("/test") def test(): isTrue = True data = { "name": "Xiao Hei", "age": 18 } ls = [1,2,3] return render_template('index.html',isTrue=isTrue,data=data,ls=ls)
index.html
<html lang="en"> <head> <meta charset="UTF-8"> <title>I'm the first page title> head> <body> <h1>Hello Worldh1> Display object content {{data}} <br> Displays the names in the object{{data.name}} <br> Displays the age in the object {{data.age}} <br> Show Boolean values {{isTrue}} <br> Display list {{ls}} <br> Displays the first data in the list {{ls[0]}} body> html>
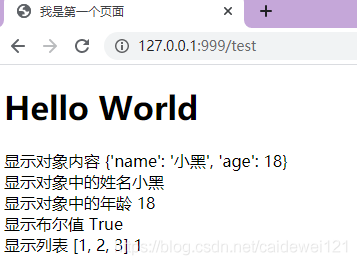
2.3 use of filters
In Jinjia2, you can use filters to modify variables
Basic format: displayed in the form of vertical bar + keyword
{{ name | capitalize}}
2.3.1 common filters
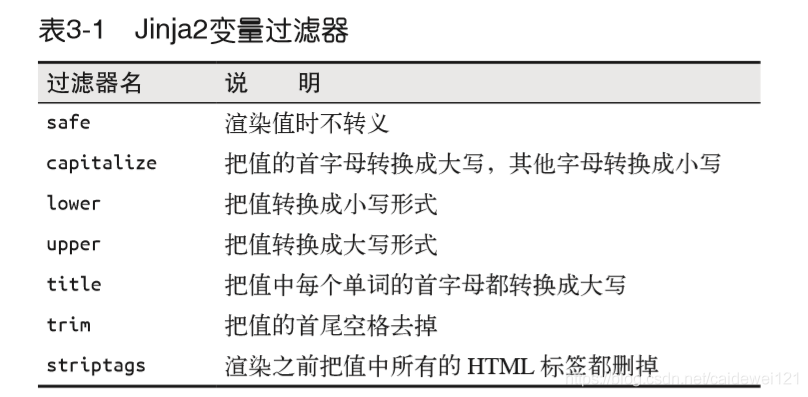
2.3.2 use of filters
@app.route("/test") def test(): isTrue = True data = { "name": "gorit smith", "age": 18 } ls = [1,2,3] return render_template('index.html',isTrue=isTrue,data=data,ls=ls)
<p>Use filter: initial uppercase, other lowercase:{{data.name | capitalize}}p> <p>upper: Convert values to uppercase{{data.name | upper}}p>

2.4 control structure
As can be seen from the following code, we can also use Python syntax to write in jinjia2, but add a judgment end to the condition judgment to tell the flash program that the judgment condition in the web page template is over.
jinjia2 The data is hidden{#Jinjia2#} <h2>if Judgment use h2> {% if data.age < 18 %} {{data.name}} {% elif data.age == 18 %} do Something {% else %} {{data.age}} <a href="">a> {% endif %}
2.5 circular syntax
The loop syntax in the Jiajia2 template is basically similar to the for loop in Python
<h2>for in loop h2> {% for foo in [1,2,3,4,5]%} {{foo}} <div>111div> {% endfor %} <h4>for Traversal dictionary h4> {% for key,value in data.items() %} {{key}} {{value}} <br> <div>111div> {% endfor %}
2.6 accessing static files
There are many static files, such as an html document, css file, js file, picture and so on. In Python flash, if we can access the static file, we need to add a static directory to the root directory, and then use the URL in flash_ The for () function specifies the path of the static file. Let's take a look at the specific example
I first define a view function named img of the class and let it load watch.html
@app.route('/watch') def img(): return render_template('watch.html')
Let me create a static file in the root directory to store some static files
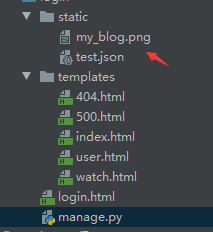
Next, write the watch.html file
<html lang="en"> <head> <meta charset="UTF-8"> <title>Titletitle> head> <body> <p>Show static files <img src="{{ url_for('static', filename='./my_blog.png') }}" width="50" >p> body> html>
Then we run flash and add / watch to the root directory to see the static files
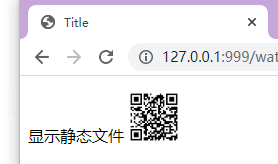
2.7 front end page outreach jump
When we use Flask to access a non-existent page, Flask will return us to a 404 page. Then we enter this page and click return to return to the home page. What should we do here?
It's actually very simple. We can use url_for('the name of the view function can be solved ')
<a href="{{url_for('web.index') }}">Punish bloggers a>
Explain why I added a web in front of me, because I defined a blueprint called web, so I need to add web to solve the problem of jumping to the home page.