Python object oriented programming
- 1, Some concepts in object oriented
- 2, Python uses object-oriented programming
- 3, Use inheritance, encapsulation, polymorphism
- 4, Properties and methods
- 5, Special method
After learning the basics of python, when I wanted to write some of my small projects through Python OOP, I found it very difficult, so I went back to learn the object-oriented idea in Python again this time
Learning materials reference: Portal
1, Some concepts in object oriented
1.1 what are classes?
Class: user-defined object prototype, which defines a set of attributes that can describe any object of this class. Attributes are data members (class variables and instance variables) and methods, which can be accessed through '.'. To put it simply, a class is a template that we can use to generate different concrete objects to complete the operations we want
1.2 what are examples?
Instance: a single object of a class. For example, we define a Person class, and specific people, such as Xiao Ming and Xiao Huang, are instances of the Person class
1.3 what are attributes?
Attributes: describe the characteristics of this class, such as human attributes, ID card, name, gender, height, weight, etc
1.4 what is the method?
Method: it is the behavior of this kind of object. For example, the boy can play basketball, the girl can sing, etc. all belong to methods. Attribute values in some classes are often changed through methods
2, Python uses object-oriented programming
This is an article written at the time of early learning foundation, which is for reference only Python object oriented programming (OOP) -- classes Python object oriented programming (OOP) -- value taking, assignment method and logic Python object oriented programming (OOP) -- inherit and use slots
2.1 define a class
A class defined in Python uses the keyword class, a simple instance
class Student: pass # Create object instance stu = Student() print(stu) print(property(stu)) print(type(stu))

When defining a class, we often make the first letter uppercase. Of course, lowercase is OK, but uppercase is used because it is a normative problem. The printed results can also be seen. The instance we create is in the memory address of my computer
2.2 adding basic attributes to classes
There are two types of attributes in a class
- Class properties
- Object properties
Let's take a look at the basic usage
# Careful students will find that I have added parentheses to the class here. In fact, if this parenthesis is passed as a parameter, we can pass it into another class. In this way, it becomes an inheritance relationship class Student(): student = "college student" def __init__(self,name,age): # Define two object properties, which are different in different objects self.name = name self.age = age stu1 = Student("Xiao Hong",18) stu2 = Student("Xiao Huang",19) print(f"{stu1.name}this year{stu1.age}year,yes{stu1.__class__.student}") print(f"{stu2.name}this year{stu2.age}year,yes{stu1.__class__.student}")
- A student attribute is defined in the class.student is a class attribute and can be accessed through the object. class.student. If the object attribute is changed in one place, it will be changed in other places
- Class defines a method named init. We call this method as a constructor. This method will be called automatically when an object is created in python. In the construction method, I define two object properties, so when I create the object below, I will pass in the properties of the two objects, and then we can get the object properties we set through the object
2.3 adding methods to classes
Adding a method is also very simple. Define a method using the def keyword, and then write the specific functions of the method in the method body. See the example below
class Student(): student = "college student" def __init__(self,name,age): self.name = name self.age = age def sing(self): print(f"{self.name} Can sing") def basketbal(self): print(f"{self.name} Can play basketball") stu1 = Student("Xiao Hong",18) stu2 = Student("Xiao Huang",19) print(f"{stu1.name}this year{stu1.age}year,yes{stu1.__class__.student}") print(f"{stu2.name}this year{stu2.age}year,yes{stu1.__class__.student}") stu1.sing() stu2.basketbal()
In the definition of the method in the class, the first parameter must be self, which is equivalent to the this keyword in the java constructor method. It refers to the current object. For example, stu1 is the current object, and self refers to stu1.
When we need to call an object method, we only need to use the object. Method ([parameter]), that is, stu1.sing() above
2.4 access control
In the process of learning java, we know that java has four access modifiers of public > Default > protected > private.
In Python, there is also an access modifier. To modify an attribute value in Python, you can modify it directly through the object attribute. This is problematic. For example, we set a person's age to 200. As normal people know, a person's maximum life expectancy will not exceed 150 years old, so in order to prevent this situation, We can set people's age as a private variable, so that the age attribute cannot be accessed directly from the outside. So we just need to prefix the age field with '' So we can't use object. Age or object outside__ I'm old
class Student(): # student is a class attribute that can be passed through an object__ class__.student access student = "college student" # init is the constructor of a class. This method will be called automatically when an object is created def __init__(self,name,age): # Define two object properties, which are different in different objects self.name = name if age>150: raise ValueError("People can't be over 150 years old") self.__age = age def sing(self): print(f"{self.name} Can sing") def basketbal(self): print(f"{self.name} Can play basketball") stu1 = Student("Xiao Hong",18) stu2 = Student("Xiao Huang",19) print(stu1.age) print(stu2.__age)

In this way, the age attribute cannot be accessed directly, so we need to create two methods, which are very similar to the setter and getter methods in java
- The value used to set the property
- The value used to provide access to the property
class Student(): student = "college student" def __init__(self,name,age): self.name = name if age>150: raise ValueError("People can't be over 150 years old") self.__age = age def getAge(self): return self.__age def setAge(self, age): if age > 150: raise ValueError("People can't reach the age of 150") self.__age = age stu1 = Student("Xiao Hong",18) stu2 = Student("Xiao Huang",19) stu1.setAge(20) print(stu1.getAge())
We can also use ''u' ' Decorate a method so that it is not externally accessible
3, Use inheritance, encapsulation, polymorphism
3.1 use inheritance
The example code is as follows
class Dog(): def __init__(self,name,age): self.name = name self.age = age # Define public methods def bark(self): print(f"{self.name} can bark") class pipi(Dog): def play(self): print(f"{self.name} Can roll") class dandan(Dog): def other(self): print(f"{self.name} Acrobatics") dog1 = pipi("Pippi",2) dog2 = dandan("very",3) dog1.play() dog1.bark() # Call public methods dog2.other()
In the above code, we find the one who wrote pipi class and dandan class respectively. They inherit from Dog class respectively, so they all have the properties and methods of Dog class, and then they can also have their unique properties and methods
3.2 method coverage
3.2.1 general method coverage
Suppose this is the case, the subclass and the parent have the same method name, but does the method we call belong to the parent or subclass? Let's change the code.
class Dog(): def __init__(self,name,age): self.name = name self.age = age def bark(self): print(f"{self.name} can bark") def eat(self): print(f"{self.name} I like chicken") class pipi(Dog): def play(self): print(f"{self.name} Can roll") def eat(self): print(f"{self.name} I like ham") dog1 = pipi("Pippi",2) dog1.eat()
Operation results:
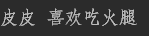
Therefore, we found that when the subclass and the parent method have the same name, the subclass method will override the parent method.
3.2.2 init method coverage
- When a subclass has no init method, it will directly inherit the init method of the parent class
- When the child class defines the init method, calling the init() method of the parent class in the init() of the subclass is similar to the above case. The difference is the timing of calling the parent class init() method.
- The subclass defines init()__ init__ () does not call the method of the parent class. At this time, note that the private property of the parent class cannot be called, and the child class will report an error when calling the get and set methods of the private property
3.3 packaging
The core of encapsulation: encapsulation is to hide some properties or methods in objects that do not want to be accessed externally
In the process of learning Java, when we perform encapsulation, we set the access permission of the property to private (only accessible in the current class), so we will use getter and setter methods to modify the value of the property. We can also use the same getter and setter methods in Python
3.3.1 degree of encapsulation (* *)
class Dog(): # Security problem. The attribute value cannot be changed arbitrarily def __init__(self,name,age): # Method 1: save with another attribute name self.hidden_name = name self.hidden_age = age def getAge(self): return self.hidden_age def setAge(self, age): if age < 0 or age > 150: raise ValueError('Unqualified age value') self.hidden_age = age
Although we do, we still don't want hidden_ The XX attribute is accessed externally, so a more advanced encapsulation is used
3.3.2 degree of encapsulation (* *)
class Retangle(): # Internal access, using hidden can still be accessed # Use__ As a private property, it is external and cannot be accessed def __init__(self,width, height): self.__width = width self.__height = height def setWidth(self,width): self.__width = width def getWidth(self): return self.__width def setHeight(self,height): self.__height = height def getHeight(self): return self.__height
We use ''u' ' As a hidden attribute, it is also a common method to make the outside invisible,
Next, explain the function of double underline:
In Python, double underscores exist as hidden attributes, but they can actually be accessed through methods. In Python, double underscores actually represent attributes in a more complex way than hidden_ The attribute is more complex. It actually puts__ xxx replaced with_ Class name__ Property name representation.
Therefore, encapsulation in Python is generally done in this step
3.3.3 degree of encapsulation (* * *)
Use decorators to better encapsulate getter s and setter s
class Person(): ''' __xxxx Become hidden attribute __name -> _Person__name use _xxx As a private attribute, do not modify the private attribute without special requirements Class generally uses properties or methods, which are not visible. You can use a single underscore ''' # Use one def __init__(self,name): self.__name = name @property def name(self): return self.__name ''' setter getter Better use of methods @property,Will one get Method to convert to object properties @Attribute name.setter Tell me one set Method to convert to object properties one can't do without the other ''' # Decorator for setter method: @ property name. Setter @name.setter def name(self, name): self.__name = name p = Person('Monkey Sai Lei') p.name = 'aaa' # Call setter s and getter s using properties print(p.name)
3.4 polymorphism
Polymorphism: it refers to a variable declared as type A, which may refer to an object of type A or any subclass object of type A
class Dog(): def __init__(self,name,age): self.name = name if age > 100: raise ValueError("Dogs can't be that old") self.__age = age def getAge(self): return self.__age def setAge(self,age): if age > 100: raise ValueError ("Dogs can't be that old") self.__age = age # Define public methods def bark(self): print(f"{self.name} can bark") def eat(self): print(f"{self.name} I like chicken") class pipi(Dog): def play(self): print(f"{self.name} Can roll") def eat(self): print(f"{self.name} I like ham") # Method coverage def bark(self): print(f"{self.name} Barking,Hee hee....") class dandan(Dog): def other(self): print(f"{self.name} Acrobatics") def dog_bark(dog): if isinstance(dog, Dog): dog.bark() d = Dog("Alaska",3) dog_bark(d) d1 = pipi("Small skin",2) dog_bark(d1) d2 = dandan("very",1) dog_bark(d2)
In the above code, we define a dog_ The bark () method can introduce the objects of the parent class or accept the objects of the child class
Using polymorphism, we do not need to define a method to call bark () for each subclass, pipi_bark(), dandan_bark(), you only need to define a dog_bark(), just pass the corresponding subclass object to it when calling.
3.5 summary of three object-oriented features
Three characteristics of object oriented
- Encapsulation (encapsulate some important attributes to prevent them from being viewed)
- Ensure data security
- Inheritance (extension)
- Ensure object access scalability
- Polymorphism (meeting the condition is some kind of transaction)
- Ensure program flexibility
4, Properties and methods
Updated and supplemented on March 23, 2020
In python object-oriented, there are five classes (attribute + method)
- Class properties
- Instance properties
- Class method
- Example method
- Static method
4.1 two types of attributes
class A(object): # Class properties count = 0 def __init__(self): self.name = 'swk' # Instance properties a = A() # A is an instance of the a object a.count = 10 # Create instance A.count = 100 # create a class object
Class properties:
- Attributes defined directly in a class are class attributes
- Class properties can be accessed directly through instances
- Class properties can only be modified through class objects, not instance objects
The third feature is that you can see the conclusion by testing the following two properties and printing them separately
a.count = 10 # Create instance A.count = 100 # create a class object
4.2 three methods
#!/usr/bin/python # -*- coding: utf-8 -- #@File: 35 properties and methods.py #@author: Gorit #@contact: gorit@qq.com #@time: 2020/3/18 17:30 class A(object): count = 0 # Class properties def __init__(self): self.name = 'swk' # Instance properties def test(self): ''' with self The methods for the first parameter are instance methods When the instance method is called, Python The calling object is used as the default self afferent Instance methods can be called through instances and classes - When invoked by instance, the current call object is automatically taken as an example. self afferent - When called through a class, it is not passed automatically self, At this point, we need to pass it manually self :return: ''' print('hello World') ''' Class method Use inside a class @classmethod The method to fix is a class method The difference between class method and instance method. The first parameter of instance method is self, The first parameter of a class method is cls Class methods can be called through classes or instances. There is no difference ''' @classmethod def test_2(cls): print('This is test2 method') ''' Static method: (can be called directly) Use in class @staticmethod The method modified by is a static method Static methods do not require any default parameters and can be called through classes and instances Static method: it is basically a method independent of the current class. It is just a function saved in the current class Static methods are all tool methods, which have nothing to do with the current method ''' @staticmethod def test_3(): print('test_3 Yes')
5, Special method
In Python, everyone should have used some internal functions 8, such as len(), str(). In fact, we can also define these methods in our own classes.
Special methods:
It has also become a magic method. Its use is also very simple. We use it__ The beginning and end can be used
Let's simply use it
class Test(): def __init__(self): print("I'm the initialization method") def __len__(self): return 55 def __str__(self): return "Hello World" t = Test() print(t) print(len(t)) print(str(t)) # There are many common ones. You can try them yourself ''' Special methods have also become magic methods Special methods to __ Beginning and end, such as __init__ Initialization method __str__() str() This special method is called when trying to convert an object to a string __rpr__() rpr() __len__() Get length len() __bool__() Returns a Boolean value bool() __pow__ __lshift__() __lt__() __add__() __and__ __or__ __eq__ __sub__ '''
Operation results
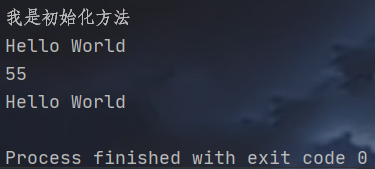