Another concept about OOP is "inheritance". Based on one class, "inherit" its methods and properties to build another class.
catalogue
1, Why is there the concept of "inheritance"?
1.1. Inherit in multiple classes
2, Better control of objects - using slots
2.1. We specify the type of Python storage
1, Why is there the concept of "inheritance"?
Understand "inheritance" in one picture
class Parent class: def Methods in the parent class(self): #Do things class Subclass(Parent class): #The subclass inherits from the parent class, and the subclass has all the methods of the parent class ..... zi = Subclass() To create a subclass object is to create a subclass instance zi.Methods in the parent class #Execute the method of the parent class
Considering many similar data types with a few key differences, you may think of using OOP to process this data. You can create different classes for each data type, but there will be a lot of code duplication, which is not very efficient
A better approach is to define a "base class" that contains common elements, and then define "subclasses" that inherit properties from the base class ji.
If you need to write a game or simulation program involving different types of vehicles, what properties do you want to define for each type of vehicle?
Vehicles have something in common and differ from:
1. All vehicles have X and Y coordinates and speeds on the screen, and all vehicles also have common moving and rendering methods, just as we used classes to write pinball game programs Two ways to write pinball games , these can be put into the base class "Vehicle" 2. Then we can start to consider subclasses of specific vehicles. For example, excavators need all the functions of "Vehicle" and also include special methods that only excavators have. For example, excavators have shovels, and their function is "excavation". Helicopters must also be based on "Vehicle" but need their own "z_pow (height)" to leave the ground Look at the following code:
class Vehicle: def __init__(self,x,y): self.x_pos =x self.y_pos =y self.x_speed = 0 self.y_speed = 0 def update(self): print("Moving...") self.x_pos +=self.x_speed self.y_pos +=self.y_speed def render(self): print("Drawing...") class Digger(Vehicle): #We inherit methods and properties from the base class "Vehicle" def __init__(self,x,y): Vehicle.__init__(self,x,y) #First initialize the "Vehicle" section def dig(self):#Method of adding excavator specific classes print("Digging...") class Helicopter(Vehicle): def __init__(self,x,y,height): Vehicle.__init__(self,x,y) self.z_pow = height #Helicopter specific attributes car = Vehicle(10,20) car.update() car.render() digger = Digger(50,90) digger.dig()#Only excavators can call this method chopper = Helicopter(200,400,50) chopper.update() chopper.render()
1.1. Inherit in multiple classes
This program clearly shows us how to inherit methods and properties from another class, but we can also inherit from multiple classes. We only need to put parentheses in the class definition, put multiple base classes, and then separate them with commas. However, this is generally recommended
2, Better control of objects - using slots
Better control of objects, to be precise, is to control the properties they have. For example, when you create an object, you can add additional properties to the program, even if these properties are not in the class definition. Take a look at the example program below
class Myclass: def __init__(self,passed_number): self.number = passed_number x = Myclass(10) print(x.number) x.text = "Hello,everybody" print(x.text) print(x.__dict__)

Here we create a new class definition, including the attribute "number". When we create the instance of x = Myclass(10), set its attribute to 10. Everything is going well. What the hell is x.text = "Hello, everyone" after that? Here we create a new attribute - string attribute, which is not specified in the class definition.
Although we have created a new attribute, the disadvantages are also obvious. The attribute is saved in the dictionary, which takes time to process, and the memory utilization is not very effective. The unique dictionary with this phenomenon is the "_dict_", and the operation results in the third line also show the contents of the dictionary
2.1. We specify the type of Python storage
To save space, we can specify that Python saves instance properties without a dictionary. You can specify which properties are allowed to be created so that no other properties will be created. This function can be realized through "slot s". See the following code for details
class Myclass(object): __slots__ = ["number","name"] def __init__(self,passed_number): self.number = passed_number x = Myclass(10) print(x.number) x.name = "Bob" print(x.name) x.text = "Hello"
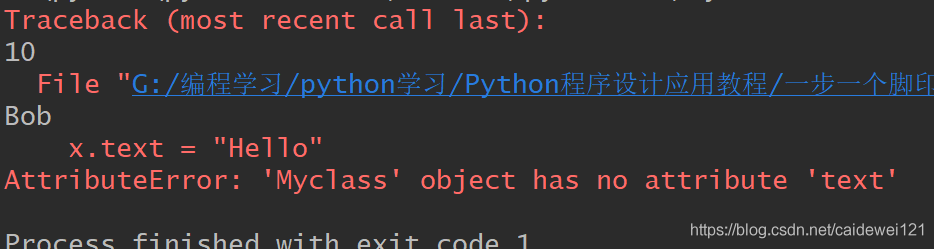
10 and "Bob" are printed normally, but the program error tells us that we have not defined the "text" attribute. In fact, what I want to say is not here
When the amount of data we need to process is very large, but storing data in a dictionary is very wasteful. We can use "_slot _" to save the data we need to store, which can greatly save memory