python operates MongoDB database
MongoDB is a database composed of multiple collections, and each collection is composed of multiple documents.
File:
#Be similar to
{
"_id" : ObjectId("5d2944d421b631f231d08056"),
"username" : "Li Yang",
"password" : "123456",
"age" : 25,
"gender" : "male",
"dept" : [
{
"title" : "Production department"
},
{
"title" : "Quality department"
}
]
}
#This is called a document
#Documents are nested
#In document key Values are unique, case sensitive, and non repeatable
#Key value pairs in documents are ordered
Collection:
#A collection is a set of documents
#Documents are like lines in a relational Library
#A set is similar to a table in a relational Library
#Documents in a collection do not need a fixed structure (non relational)
I. environmental installation
1. pymongo installation
(automatic) C:\Users\Administrator>pip install pymongo
2. Check whether the installation is successful
(automatic) C:\Users\Administrator>python
Python 3.5.2 (v3.5.2:4def2a2901a5, Jun 25 2016, 22:18:55) [MSC v.1900 64 bit (AM
D64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import pymongo
>>>
II. python operation MongoDB

from pymongo import MongoClient
from datetime import datetime
from bson.objectid import ObjectId
class MongoDatabase:
def __new__(cls, *args, **kwargs):
"""Singleton mode"""
if not hasattr(cls,"instance"):
cls.instance = super(MongoDatabase,cls).__new__(cls, *args, **kwargs)
return cls.instance
def __init__(self):
"""
//Connect to database
"""
self.client = MongoClient()
self.db = self.client['test']
def add_one(self):
"""
//Add a piece of data
:return:
"""
post = {
"author": "aaa",
"text": "bbb",
"date": datetime.utcnow()
}
return self.db.table1.insert_one(post) # Database oriented test Of table1 Add data to table
def get_one(self):
"""
//Query a piece of data
:return:
"""
return self.db.table1.find_one()
def get_more(self):
"""
//Query multiple data
:return:
"""
return self.db.table1.find({'author': 'aaa'})
def get_one_from_id(self, id):
"""
//Query data by id
:return:
"""
obj = ObjectId(id)
return self.db.table1.find_one({"_id": obj})
def update_one_data(self):
"""
//Update a piece of data by id
:return:
"""
result=self.db.table1.update_one({'text':'bbb'},{'$inc':{'x':10}})#find text=bbb This data and x Numerical plus 10
return result
def update_many_data(self):
"""
//Update multiple data
:return:
"""
result=self.db.table1.update_many({},{'$inc':{'x':10}})#Find all the data and put the x Numerical plus 10
return result
def delete_one(self):
"""
//Delete one piece of data: if more than one piece of data meets the conditions, only the first piece will be deleted.
:return:
"""
result=self.db.table1.delete_one({'text':'bbb'})
return result
def delete_many(self):
"""
//Delete multiple data
:return:
"""
result=self.db.table1.delete_many({})
return result
def main():
mongo_obj = MongoDatabase()
print(mongo_obj)
# New data
# result=mongo_obj.add_one()
# print(result.inserted_id)#5d286c7bbf18433e81e4f16f
# Query data
# result=mongo_obj.get_one()
# print(result) #Dictionary type, result['id ']
# Query multiple data
# result=mongo_obj.get_more()
# # for item in result:
# # print(item['text'])
# adopt id Query data
# result = mongo_obj.get_one_from_id('5d286c7bbf18433e81e4f16f')
# print(result)
#Update a piece of data
# result=mongo_obj.update_one_data()
# print(result.matched_count)
# print(result.modified_count)
#Delete a piece of data
# result=mongo_obj.delete_one()
# print(result.delete_count)
if __name__ == '__main__':
main()
Implementing mongodb database by python in singleton mode
III. ODM operation
1. Install mongoengine module
(automatic) C:\Users\Administrator>pip install mongoengine
2. Check whether the installation is successful
(automatic) C:\Users\Administrator>python
Python 3.5.2 (v3.5.2:4def2a2901a5, Jun 25 2016, 22:18:55) [MSC v.1900 64 bit (AM
D64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import mongoengine
>>>
3. Model introduction
mongoengine module is equivalent to SQL alchemy module in mysql database. It is more convenient to operate the database by object than by native operation.
(1) data type
BinaryField
BooleanField
ComplexDateTimeField
DateTimeField
DecimalField
DictField
DynamicField
EmailField
EmbeddedDocumentField
EmbeddedDocumentListField
FileField
FloatField
GenericEmbeddedDocumentField
GenericReferenceField
GenericLazyReferenceField
GeoPointField
ImageField
IntField
ListField
MapField
ObjectIdField
ReferenceField
LazyReferenceField
SequenceField
SortedListField
StringField
URLField
UUIDField
PointField
LineStringField
PolygonField
MultiPointField
MultiLineStringField
MultiPolygonField
Parameters can be set for each field type:
db_field (Default: none)
#MongoDB Field name.
required (Default value: False)
#If set to True If the field is not set on the document instance, the ValidationError When a document is validated, the a .
default (Default: none)
#The value used when no value is set for this field.
unique (Default value: False)
#If so True,No document in the collection has the same value for this field.
unique_with (Default: none)
#When a field name (or a list of field names) is used with this field, there will not be two documents in the collection with the same value.
primary_key (Default value: False)
#If so True,Use this field as the primary key for the collection. DictField and EmbeddedDocuments Are supported as primary keys for documents.
choices (Default: none)
#Should limitThe value of the field can be selected iteratively (for example, list, tuple, or set).
#For example:
SIZE = (('S' , 'Small' ),
('M' , 'Medium' ),
('L' , 'Large' ),
('XL' , 'Extra Large' ),
('XXL' , 'Extra large' ))
class Shirt (Document ):
size = StringField (max_length = 3 , choices = SIZE )
**kwargs (Optional) #Other metadata can be provided as any other key parameter. However, you cannot overwrite existing properties. Common choices include help_text and verbose_name,They are commonly used by forms and widget libraries.
(2) create model
from mongoengine import connect, Document, StringField, IntField, EmbeddedDocument, ListField, EmbeddedDocumentField
connect('userinfo') #Connect to database, connect to local by default
GENDER_CHOICES = (
('male', 'male'),
('female', 'female'),
)
class Dept(EmbeddedDocument):
"""
//Departmental table
"""
title = StringField(max_length=32, required=True)
class User(Document):
"""
//User table
"""
username = StringField(max_length=32, required=True)
password = StringField(max_length=64, required=True)
age = IntField(required=True)
gender = StringField(choices=GENDER_CHOICES, required=True)
dept = ListField(EmbeddedDocumentField(Dept)) # Related department table
meta = {
'collection': 'user' # Specify what document to save in
}
The User table above is equivalent to a collection. It inherits the Document class, while dept is actually a field in the User table. Each User instance is equivalent to a Document (a row of data in the collection). See the generated specific data for details:
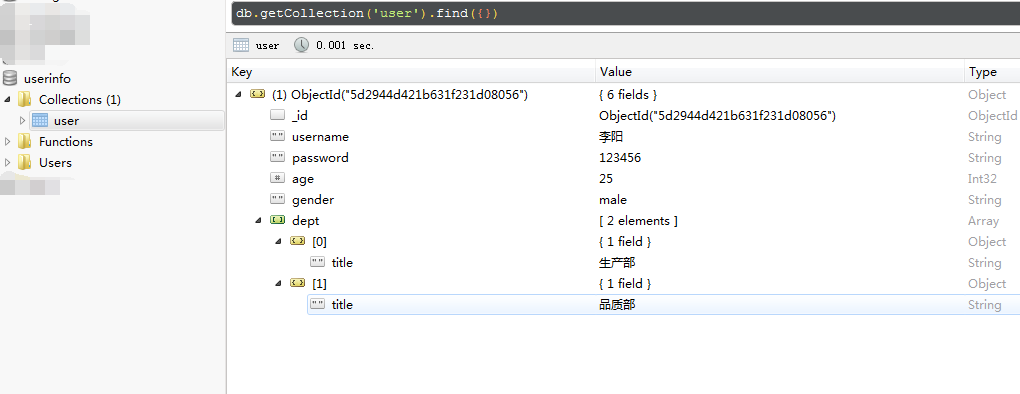
4. Operation model
After creating the model, you need to CURD the data in the model and create a class to operate:
class MongoEngine(object):
def add_one(self):
"""
//Add a piece of data
:return:
"""
product_department = Dept(
title="Production department"
)
quality_department = Dept(
title="Quality department"
)
user_obj = User(
username='Li Yang',
password='123456',
age=20,
gender='male',
dept=[product_department, quality_department]
)
user_obj.save()
return user_obj
def get_one(self):
"""Query a piece of data"""
return User.objects.first()
def get_more(self):
"""Query multiple data"""
return User.objects.all()
def get_from_id(self, id):
"""according to id Query"""
return User.objects.filter(pk=id).first()
def update_one(self):
"""Modify single data"""
return User.objects.filter(gender='male').update_one(inc__age=5)
def update_more(self):
"""Modify multiple data"""
return User.objects.filter(gender='male').update(inc__age=5)
def delete_one(self):
"""Delete a piece of data"""
return User.objects.filter(gender='male').first().delete()
def delete_from_id(self,id):
"""according to id Deleting"""
return User.objects.get(pk=id).delete()
def delete_more(self):
"""Delete multiple data"""
return User.objects.filter(gender='male').delete()
5. Complete example

from mongoengine import connect, Document, StringField, IntField, EmbeddedDocument, ListField, EmbeddedDocumentField
connect('userinfo') #Connect to database, connect to local by default
GENDER_CHOICES = (
('male', 'male'),
('female', 'female'),
)
class Dept(EmbeddedDocument):
"""
//Departmental table
"""
title = StringField(max_length=32, required=True)
class User(Document):
"""
//User table
"""
username = StringField(max_length=32, required=True)
password = StringField(max_length=64, required=True)
age = IntField(required=True)
gender = StringField(choices=GENDER_CHOICES, required=True)
dept = ListField(EmbeddedDocumentField(Dept)) # Related department table
meta = {
'collection': 'user' # Specify what document to save in
}
class MongoEngine(object):
def add_one(self):
"""
//Add a piece of data
:return:
"""
product_department = Dept(
title="Production department"
)
quality_department = Dept(
title="Quality department"
)
user_obj = User(
username='Li Yang',
password='123456',
age=20,
gender='male',
dept=[product_department, quality_department]
)
user_obj.save()
return user_obj
def get_one(self):
"""Query a piece of data"""
return User.objects.first()
def get_more(self):
"""Query multiple data"""
return User.objects.all()
def get_from_id(self, id):
"""according to id Query"""
return User.objects.filter(pk=id).first()
def update_one(self):
"""Modify single data"""
return User.objects.filter(gender='male').update_one(inc__age=5)
def update_more(self):
"""Modify multiple data"""
return User.objects.filter(gender='male').update(inc__age=5)
def delete_one(self):
"""Delete a piece of data"""
return User.objects.filter(gender='male').first().delete()
def delete_from_id(self,id):
"""according to id Deleting"""
return User.objects.get(pk=id).delete()
def delete_more(self):
"""Delete multiple data"""
return User.objects.filter(gender='male').delete()
def main():
mongo_obj = MongoEngine()
# Add a piece of data
user_obj=mongo_obj.add_one()
print(user_obj.id)
# Query a piece of data
# user_obj=mongo_obj.get_one()
# print(user_obj.id,user_obj.username)
# Query all data
# queryset = mongo_obj.get_more()
# for user_obj in queryset:
# print(user_obj.id, user_obj.username)
# Modifying data
# result = mongo_obj.update_one()
# print(result)
# Delete data
# result = mongo_obj.delete_one()
# print(result)
# obj = mongo_obj.delete_from_id('5d2944a2a1bd1324ebe43d68')
# print(obj)
if __name__ == '__main__':
main()
Complete example