Author: AXYZdong Automation Engineering Male
File and file path
Two key attributes of a file: file name and path (indicating the location of the file on the computer)
On Windows, the path is written with a backslash \ as the separator between folders
On OS X and Linux, the forward slash / is used as the separator of the path.
Current working directory
Each program running on a computer has a "current working directory", or cwd. If there is no file name or path starting from the root folder, it is assumed to be in the current working directory. os.getcwd() function obtains the string of the current working path. You can use OS Chdir () changes it.
>>>os.getcwd() # Get current work path, cwd(current work directory): current work path 'D:\\Python Study'
Absolute path and relative path
- Absolute path: always start from the root folder.
- Relative path: relative to the current working directory of the program.
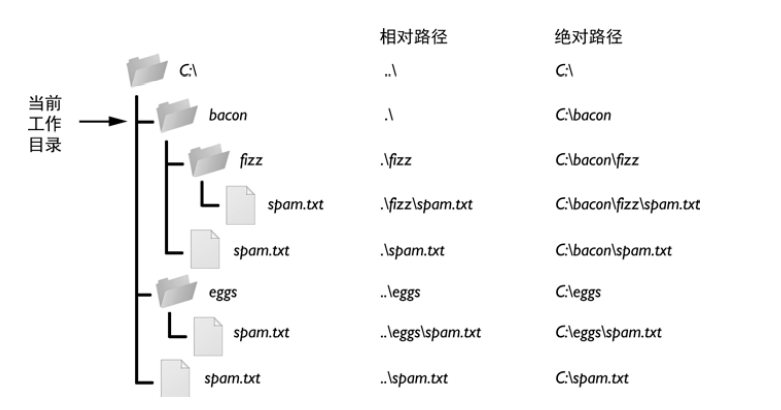
Use OS Makedirs() creates a new folder
>>>import os >>>os.makedirs('.\\read1') >>>os.makedirs('D:\\Python study\\read2')
os.makedirs('.\read1'): relative path, which means to create the read1 folder under the current working directory.
os.makedirs('D:\Python study\read2 '): absolute path. Create read2 folder under Python Study folder on disk D.
To ensure that the full pathname exists, if the intermediate folder does not exist, OS Makedirs () will create all necessary intermediate folders.
os.path module
os. The path module contains many useful functions related to file names and file paths. os.path is a module in the OS module. You can import it by importing OS. os. Complete documentation of the path module: http://docs.python.org/3/library/os.path.html .
Handle absolute and relative paths
- os.path.abspath(path) returns the string of the absolute path of the parameter to facilitate the conversion from relative path to absolute path.
- os.path.isabs(path) determines whether the parameter is an absolute path. If yes, it returns True; otherwise, it returns False.
- os.path.relpath(path,start) will return the string of the relative path from start to path. If start is not provided, the current working directory will be the default starting path.
- os.path.dirname(path) will return a string containing everything before the last slash in the path parameter. (that is, return the directory name)
- os.path.basename(path) will return a string containing everything after the last slash in the path parameter. (that is, return the base name)
- os.path.split(path) returns the directory name and basic name of a path at the same time, and obtains the tuple containing these two strings.
>>>import os >>>path = 'C:\\Windows\\System32\\calc.exe' >>>os.path.abspath(path) 'C:\\Windows\\System32\\calc.exe' >>>os.path.isabs(path) True >>>os.path.relpath(path,'C:\\Windows') 'System32\\calc.exe' >>>os.path.dirname(path) 'C:\\Windows\\System32' >>>os.path.basename(path) 'calc.exe' >>>os.path.split(path) ('C:\\Windows\\System32', 'calc.exe')
View file size and folder contents
- os.path.getsize(path) returns the size of the folder under the path.
- os.listdir(path) returns a list of file name strings under the path.
>>>path = os.getcwd() >>>os.path.getsize(path) 4096 >>>os.listdir(path) ['.idea', 'Data Visualization', 'demo', 'demo.py', 'demo_1.py', 'paper.png', 'PDF synthesis', 'text.py', 'turtle', 'watermark', 'wordcloud', '__pycache__', 'lantern.py', 'Climb picture.py', 'veil.jpg']
Check the validity of the path
- os.path.exists(path) determines whether the file or folder specified by the path parameter exists. If it exists, it returns True; otherwise, it returns False.
- os.path.isfile(path) determines whether the path parameter is a file. If yes, it returns True; otherwise, it returns False.
- os.path.isdir(path) determines whether the path parameter is a folder. If yes, it returns True; otherwise, it returns False.
>>>path = os.getcwd() >>>os.path.exists(path) True >>>os.path.exists('D:\\read') False >>>os.path.exists('D:\\Python study') True >>>os.path.isfile(path) False >>>os.path.isdir(path) True >>>os.path.isfile('D:\\Python Study\\demo.py') True
Using OS path. The exists() function determines whether a DVD or flash drive is currently connected to the computer. (there is no F disk on my current computer)
>>>os.path.exists('F:\\') False
File reading and writing process
Plain text file: contains only basic text characters and does not contain font, size and color information. For example: with txt extension text file with Python script file with py extension.
Three steps for reading and writing files:
- Call the open() function to return a File object.
- Call the read() or write() method of the File object.
- Call the close() method of the File object to close the File.
open Several modes of opening files Character Meaning --------- --------------------------------------------------------------- 'r' open for reading (default) 'w' open for writing, truncating the file first 'x' create a new file and open it for writing 'a' open for writing, appending to the end of the file if it exists 'b' binary mode 't' text mode (default) '+' open a disk file for updating (reading and writing) 'U' universal newline mode (deprecated)
Create one in the current directory TXT text file, and then write to Hello world! And my name is axyzdong.
>>>onefile = open('one.txt','w') #Open text in write mode >>>onefile.write('Hello world!\n') 13 >>>onefile.close() >>>onefile = open('one.txt','a') #Open text in add mode >>>onefile.write('my name is axyzdong') 19 >>>onefile.close() >>>onefile = open('one.txt') >>>content = onefile.read() >>>onefile.close() >>>print(content) Hello world! my name is axyzdong
Save variables with shelve module
Use the shell module to save the variables in the Python program to the binary SHELL file.
Steps:
- import shelve
- Call the function shelve Open () and pass in a file name, and then save the return value (shell value) in a variable. (you can modify the shell value of this variable, just like a dictionary)
- Invoke the close() method in the returned object.
shelve. The file opened by open () can be read and written by default. Like the dictionary, the shelf value has the keys() and values() methods to return the keys and key values in the shelf.
Use pprint The pformat() function saves the variable and writes it to the py file
Usage: suppose there is a dictionary stored in a variable, and you want to save the variable and its contents, then you can use pprint Pformat () function to save this variable and write it to py file. This file will become a module that can be called when needed.
>>>import pprint >>>characters = {'one':'a','two':'b'} >>>pprint.pformat(characters) "{'one': 'a', 'two': 'b'}" >>>file = open('characters.py','w') >>>file.write('characters = '+ pprint.pformat(characters) + '\n') 38 >>>file.close() >>>import characters >>>characters.characters {'one': 'a', 'two': 'b'} >>>characters.characters['one'] 'a'
In this way, there will be a character in the current working directory Py file, which contains only one line of code characters = {'one':'a ',' two ':'b'}
Create a The advantage of py file is that py file is a text file. Anyone can use a simple text editor to read and modify the contents of the file.
reference
1: Python Programming gets started quickly: automating cumbersome work / (US) written by A1 sweigart; Translated by Wang Haipeng Beijing: People's Posts and Telecommunications Press, July 2016
that's all for this sharing