- Two files, cards_main.py and cards_ tools. py. The functions realized include adding business cards, displaying all business cards and querying business cards.
General idea:
- First, edit the main function to determine the functions in the main function. After the file needs to be executed, the menu function show is displayed directly_ Menu function, enter the operation to be performed, 1: add a business card; 2: Display all; 3: Query business cards; 0: exit the system. Then edit the content of the function according to the four functions.
- Add business card function NEW_ In card, first prompt the function as a new business card and prompt the user to enter information, then store the business card information in the dictionary and then store it in the list. After saving, you will be prompted that the saving is successful.
- Show all business card functions in_ In all, first prompt that the function is to display all business cards, and then output according to whether there is a business card record at present. If there is no record, prompt that there is no business card at present, and you need to add a business card and return the result; If there are records, first print the formatted header, and then traverse the information in the output dictionary in turn.
- In the search business card function search_ In card, first prompt the user for the name to be searched, and then traverse the business card list to query the name to be searched. If not, prompt the user to apologize for not finding it; If yes, output the name and information.
- To modify the found business card records, a deal is added_ Card function to process the found business card. First, prompt whether the operation to be performed is to modify, delete or return to the superior menu. If it is modified, enter the user's information according to the prompt. If the modification is successful, you will be prompted that the modification is successful; If it is deleted, use remove to delete the business card information.
- How to handle the business card information input during modification: add the function input_card_info, pass in the original value in the dictionary and the input prompt text. First prompt the user to enter the content, and then judge according to the user's input. If the content is entered, the result will be returned directly; If there is no input, the original value of the dictionary will be returned.
The code is as follows:
- cards_main.py file is as follows:
import cards_tools
# Infinite loop. The user decides when to exit the loop
while True:
# TODO comments
# TODO(amelia) display menu function
cards_tools.show_menu()
action_str = input("Please select the action you want to perform:")
print("The action you selected is[%s]" % action_str)
# 1,2,3 operations for business cards
if action_str in ["1", "2", "3"]:
# Add business card
if action_str == "1":
cards_tools.new_card()
# Show all
elif action_str == "2":
cards_tools.show_all()
# Query business card
elif action_str == "3":
cards_tools.search_card()
pass
# 0 quit the system
elif action_str == "0":
print("Welcome to [business card management system] again")
break
# If you don't want to write code inside the branch immediately when developing a program
# You can use the pass keyword to represent a placeholder, which can ensure the correct code structure of the program!
# When the program is running, the pass keyword will not perform any operation!
# pass
# The user needs to be prompted for other content input errors
else:
print("Your input is incorrect, please select again")
- cards _tools.py file is as follows:
# Record all business card Dictionaries
card_list = []
def show_menu():
"""Display menu"""
print("*" * 50)
print("")
print("1.Add business card")
print("2.Show all")
print("3.Search business card")
print("")
print("0.Exit the system")
print("*" * 50)
def new_card():
"""Add business card"""
print("-" * 50)
print("Add business card")
# 1. Prompt the user to enter the details of the business card
name_str = input("Please enter your name:")
phone_str = input("Please enter the phone number:")
qq_str = input("Please enter QQ: ")
email_str = input("Please enter email address:")
# 2. Create a business card dictionary using the information entered by the user
card_dict = {"name": name_str,
"phone": phone_str,
"qq": qq_str,
"email": email_str}
# 3. Add business card to the list
card_list.append(card_dict)
print(card_list)
# 4. Prompt the user to add successfully
print("add to %s Your business card is successful!" % name_str)
def show_all():
"""Show all business cards"""
print("-" * 50)
print("Show all business cards")
# Judge that there are business card records. If there are no business card records, prompt the user and return
if len(card_list) == 0:
print("There are no business card records currently. Please use the new features to add business cards!")
# return returns the execution result of a function
# The code below will not be executed
# If there is nothing after return, it means that it will return to the location of the calling function
# And will not return any results
return
# Print header
for name in ["full name", "Telephone", "QQ", "mailbox"]:
print(name, end="\t\t")
print()
# Print split lines
print("=" * 50)
# Traverse the business card list and output dictionary information in turn
for card_dict in card_list:
print("%s\t\t%s\t\t%s\t\t%s" % (card_dict["name"],
card_dict["phone"],
card_dict["qq"],
card_dict["email"]))
def search_card():
"""Search business card"""
print("-" * 50)
print("Search business card")
# 1. Prompt the user to enter the name to search
find_name = input("Please enter a name to search for:")
# 2. Traverse the business card list and query the name to be searched. If it is not found, the user needs to be prompted
for card_dict in card_list:
if card_dict["name"] == find_name:
print("full name\t\t Telephone\t\tQQ\t\t mailbox")
print("=" * 50)
print("%s\t\t%s\t\t%s\t\t%s" % (card_dict["name"],
card_dict["phone"],
card_dict["qq"],
card_dict["email"]))
# Modify and delete the business card records found
deal_card(card_dict)
break
else:
print("Sorry, not found %s" % find_name)
def deal_card(find_dict):
"""Process found business cards
:param find_dict:Business card found
"""
print(find_dict)
action_str = input("Please select the action to perform "
"1 Modify 2 delete 3 return to superior menu")
if action_str == "1":
find_dict["name"] = input_card_info(find_dict["name"], "full name:")
find_dict["phone"] = input_card_info(find_dict["phone"], "Telephone:")
find_dict["qq"] = input_card_info(find_dict["qq"], "QQ:")
find_dict["email"] = input_card_info(find_dict["email"], "mailbox:")
print("Business card modified successfully!")
elif action_str == "2":
card_list.remove(find_dict)
print("Delete business card successfully!")
def input_card_info(dict_value, tip_message):
"""Enter business card information
:param dict_value:Original value in dictionary
:param tip_message:Prompt text for input
:return:If the user enters the content, the content is returned; otherwise, the original value in the dictionary is returned
"""
# 1. Prompt the user to enter content
result_str = input(tip_message)
# 2. Judge the user's output. If the user inputs content, the result will be returned directly
if len(result_str) > 0:
return result_str
# 3. If the user does not use the input content, return the original value in the dictionary
else:
return dict_value
Operation process:
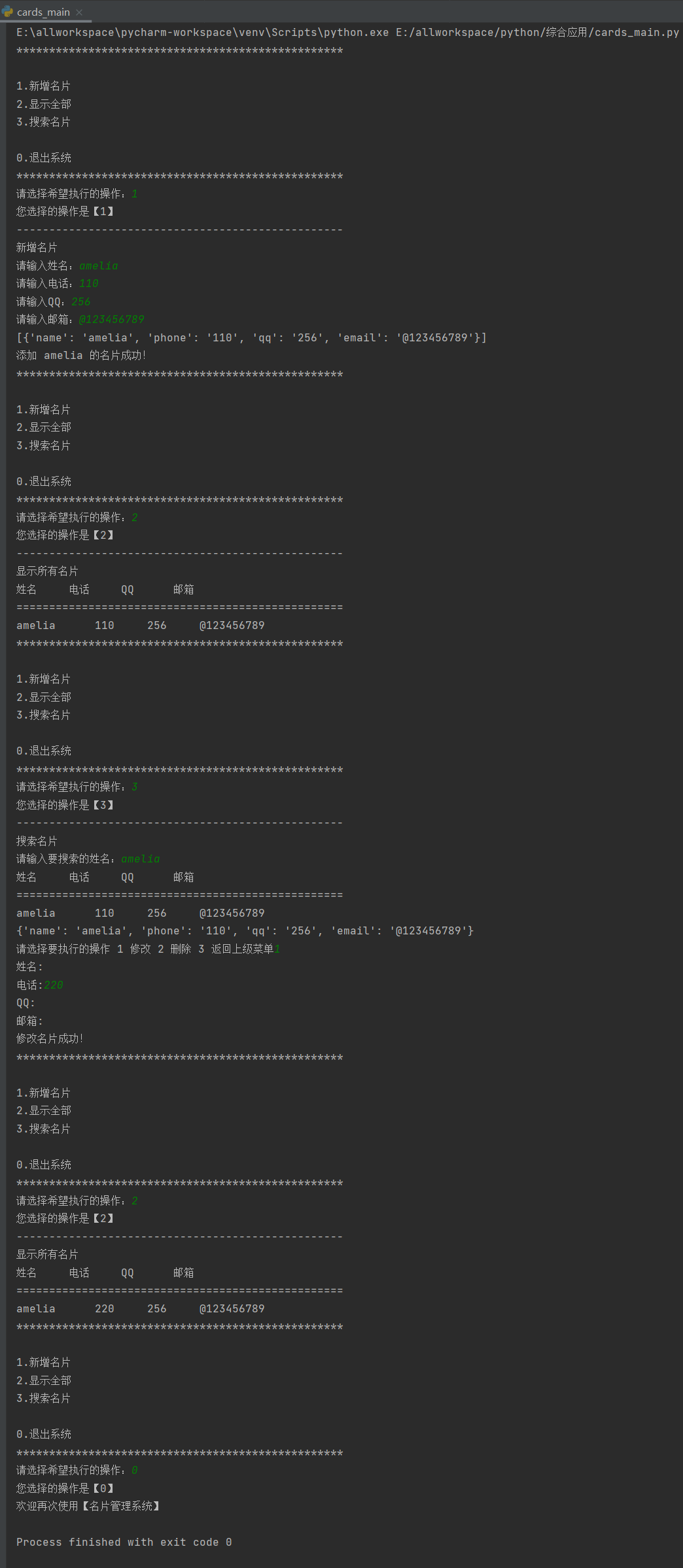