Python While Loop Statement
In Python programming, the while statement is used to execute a program in a loop, that is to say, under certain conditions, a program is executed in a loop to deal with the same tasks that need to be processed repeatedly. Its basic form is:
The while judgment condition: Execution statement...
Execution statements can be a single statement or block of statements. The judgment condition can be any expression, and any non-zero or null value is true.
When the condition false false false is judged, the loop ends.
The execution flow chart is as follows:
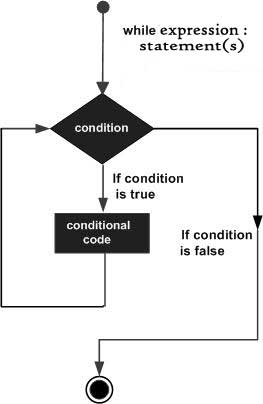
Gif demonstrates the Python while statement execution process
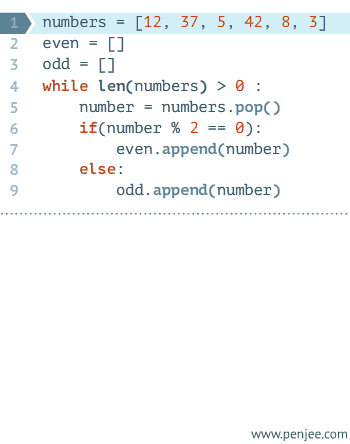
Example
Running examples »
The above code executes the output:
The count is: 0 The count is: 1 The count is: 2 The count is: 3 The count is: 4 The count is: 5 The count is: 6 The count is: 7 The count is: 8 Good bye!
There are two other important commands in the while statement: continue, break to skip the loop, continue to skip the loop, break to exit the loop, and "judgment condition" can also be a constant, which means that the loop must hold. The specific usage is as follows:
Infinite cycle
If the conditional judgement statement is always true, the loop will continue indefinitely, as shown in the following example:
Example
The output of the above example is as follows:
Enter a number :20 You entered: 20 Enter a number :29 You entered: 29 Enter a number :3 You entered: 3 Enter a number between :Traceback (most recent call last): File "test.py", line 5, in <module> num = raw_input("Enter a number :") KeyboardInterrupt
Note: The above infinite loops can be interrupted by CTRL+C.
Cyclic use of else statements
In python, while... Else executes an else statement block when the loop condition is false:
Example
The output of the above example is as follows:
0 is less than 5 1 is less than 5 2 is less than 5 3 is less than 5 4 is less than 5 5 is not less than 5
Simple statement group
Similar to the syntax of if statements, if you have only one statement in your while loop, you can write that statement on the same line as while, as follows:
Example
Note: The above infinite loops can be interrupted by CTRL+C.
Classic Comments:
-
#!/usr/bin/python # -*- coding: UTF-8 -*- import random s = int(random.uniform(1,10)) #print(s) m = int(input('Input integer:')) while m != s: if m > s: print('Big') m = int(input('Input integer:')) if m < s: print('Small') m = int(input('Input integer:')) if m == s: print('OK') break;
I'm a lovely boy. Sleeping is the best man in the world.I'm a lovely boy, sleeping is the world's man
col***iansy@Outlook.com
-
June
438***@qq.com
Guess boxing games
#!/usr/bin/python # -*- coding: UTF-8 -*- import random while 1: s = int(random.randint(1, 3)) if s == 1: ind = "stone" elif s == 2: ind = "Scissors" elif s == 3: ind = "cloth" m = raw_input('Input stones, scissors, cloth,input"end"End the game:') blist = ['stone', "Scissors", "cloth"] if (m not in blist) and (m != 'end'): print "Input error, please re-enter!" elif (m not in blist) and (m == 'end'): print "\n Game Exit..." break elif m == ind : print "The computer is out: " + ind + ",It ends in a draw!" elif (m == 'stone' and ind =='Scissors') or (m == 'Scissors' and ind =='cloth') or (m == 'cloth' and ind =='stone'): print "The computer is out: " + ind +",You win!" elif (m == 'stone' and ind =='cloth') or (m == 'Scissors' and ind =='stone') or (m == 'cloth' and ind =='Scissors'): print "The computer is out: " + ind +",You lost!"
Test results:
Input stone, scissors, cloth, enter "end" to end the game: stone Computer out: Stone, draw! Input stone, scissors, cloth, enter "end" to end the game: stone The computer is out: scissors, you win! Enter stone, scissors and cloth, and enter "end" to end the game:
JuneJune
438***@qq.com
-
Sunset Morning Injury
112***5110@qq.com
Shake the Sieve Game
#!/usr/bin/env python3 # -*- coding: utf-8 -*- import random import sys import time result = [] while True: result.append(int(random.uniform(1,7))) result.append(int(random.uniform(1,7))) result.append(int(random.uniform(1,7))) print result count = 0 index = 2 pointStr = "" while index >= 0: currPoint = result[index] count += currPoint index -= 1 pointStr += " " pointStr += str(currPoint) if count <= 11: sys.stdout.write(pointStr + " -> " + "Small" + "\n") time.sleep( 1 ) # Sleep for a second else: sys.stdout.write(pointStr + " -> " + "large" + "\n") time.sleep( 1 ) # Sleep for a second result = []
Sunset Morning InjurySunset Morning Injury
112***5110@qq.com
-
(+__+)
lk0***21428@126.com
Decimal to binary
#!/usr/bin/python # -*- coding: UTF-8 -*- denum = input("Input decimal number:") print denum,"(10)", binnum = [] # Binary Number while denum > 0: binnum.append(str(denum % 2)) # Stack pressing denum //= 2 print '= ', while len(binnum)>0: import sys sys.stdout.write(binnum.pop()) # No space output print'(2)'