The term "reactivity" refers to the programming model built around responding to change, availability, and processability - network components respond to I/O events, UI controllers respond to mouse events, resources are available, and so on. In this sense, non blocking is reactive because we are now in the mode of responding to notifications rather than being blocked because the operation is completed or data is available.
Reactive API
Reactive Streams plays an important role in interoperability. It is very interested in libraries and infrastructure components, but it is not very useful as an application API because it is too low-level. Applications need a more advanced, richer, and more powerful API to combine asynchronous logic -- similar to the Java 8 Stream API, but not limited to tables. This is the role of reactive libraries.
Project Reactor It is the preferred reaction library for Spring Data R2DBC. It provides Mono and Flux API type, which handles 0.. through a set of rich operators aligned with the ReactiveX operator vocabulary 1 (mono) and 0 Data sequence of n (flux). Reactor is a Reactive Streams library, so all its operators support non blocking back pressure. Reactor is very concerned about server-side Java. It was developed in close collaboration with Spring.
Spring Data R2DBC requires Project Reactor as the core dependency, but it can interoperate with other reactive libraries through the Reactive Streams specification. As a general rule, the Spring Data R2DBC repository accepts ordinary Publisher as input, internally adjusts it to the Reactor type, uses it, and returns aMono or aFlux as output. Therefore, you can take any Publisher as input and apply actions on the output, but you need to adjust the output to use with another reaction library. Spring Data will transparently adapt to RxJava or other reactive libraries whenever possible.
Personal understanding: through responsive WebFlux and reactive data sources, thread availability and system throughput can be improved in a non blocking way, but the response time is not much perceived.
2.R2DBC integration Mysql demo
Environmental Science:
jdk: adopt-openjdk-11
maven: 3.8.1
SpringBoot : 2.3.2.RELEASE
Pom file
<!-- lombok --> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> </dependency> <!-- r2dbc spring data--> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-r2dbc</artifactId> </dependency> <!-- r2dbc mysql library--> <dependency> <groupId>dev.miku</groupId> <artifactId>r2dbc-mysql</artifactId> <version>0.8.2.RELEASE</version> </dependency> <!-- r2dbc-pool --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-r2dbc</artifactId> <version>2.3.0.RELEASE</version> </dependency> <!-- webFlux --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-webflux</artifactId> </dependency>
application profile
### r2dbc spring: r2dbc: url: r2dbc:mysql://localhost/r2dbc?useUnicode=true&characterEncoding=UTF-8 username: root password: root pool: enabled: true max-size: 10 initial-size: 10 validation-query: select 1
Table structure
DROP TABLE IF EXISTS `user`; CREATE TABLE `user` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(255) CHARACTER SET utf8 COLLATE utf8_general_ci NULL DEFAULT NULL, `age` int(11) NULL DEFAULT NULL, `email` varchar(255) CHARACTER SET utf8 COLLATE utf8_general_ci NULL DEFAULT NULL, `phone_number` int(11) NULL DEFAULT NULL, PRIMARY KEY (`id`) USING BTREE ) ENGINE = InnoDB AUTO_INCREMENT = 6 CHARACTER SET = utf8 COLLATE = utf8_general_ci ROW_FORMAT = Dynamic; -- ---------------------------- -- Records of user -- ---------------------------- INSERT INTO `user` VALUES (1, 'admin', 12, '123@163.com', 145448546); INSERT INTO `user` VALUES (2, 'tom', 18, 'tom@163.com', 12346789); INSERT INTO `user` VALUES (5, 'jerry', 25, 'jerry@163.com', 154646126); SET FOREIGN_KEY_CHECKS = 1;
Entity class
/** * @author ZhaoJiu * @since: 2021/7/22 * @desc: User entity class */ @Table("user") @Data public class User { @Id private String id; private String name; private Integer age; private String email; private Integer phoneNumber; }
Repository in JPA
/** * @author ZhaoJiu * @since: 2021/7/22 * @desc: */ public interface PersonRepository extends R2dbcRepository<User,String> { }
Final code
/** * @author ZhaoJiu * @since: 2021/7/22 * @desc: r2dbc Operation mysql CRUD */ @RestController @RequestMapping("user") public class UserController { @Resource PersonRepository repository; **Java Interview core knowledge notes** Which includes JVM,Lock, concurrency Java Reflection Spring Principles, microservices Zookeeper,A large number of knowledge points such as database and data structure. 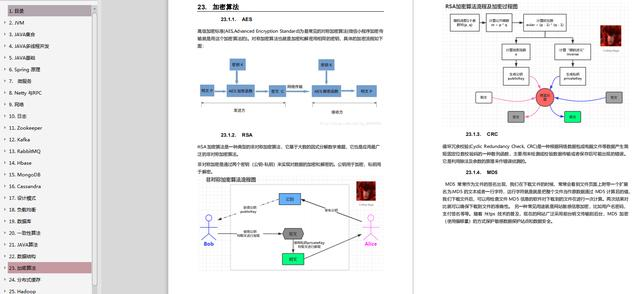 **Java Arrangement of high frequency examination sites for middle and senior interview** 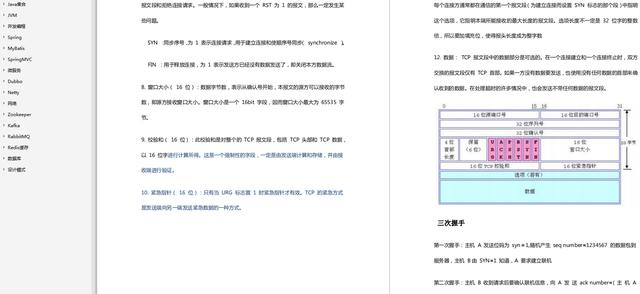 **more Java Advanced knowledge notes and document sharing are good learning materials for both interview and learning** **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** 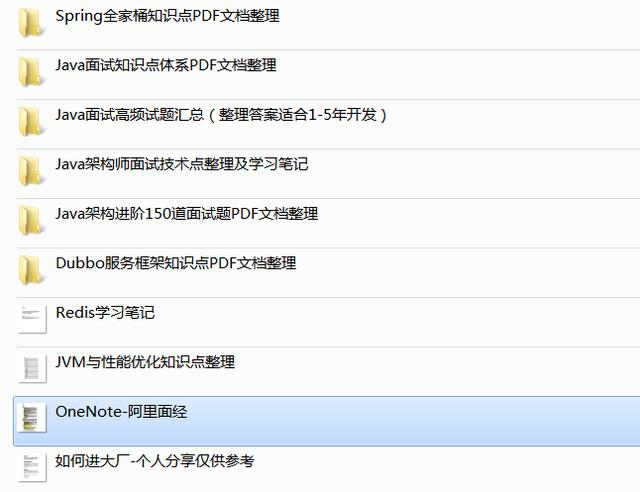 **Finally, share Java Video teaching for advanced learning and interview** 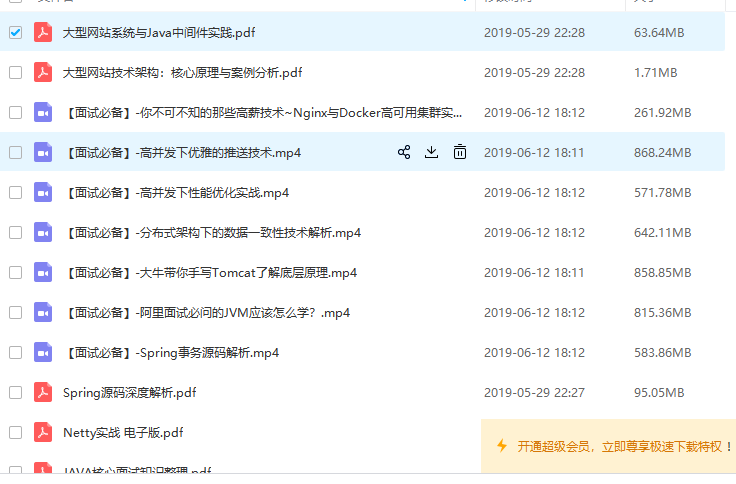 Chain picture transfer...(img-v8IfpEZu-1630911837863)] **more Java Advanced knowledge notes and document sharing are good learning materials for both interview and learning** **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** [External chain picture transfer...(img-gjBOFhug-1630911837864)] **Finally, share Java Video teaching for advanced learning and interview** [External chain picture transfer...(img-5K4DU12K-1630911837866)]