Introduction to Eureka
When subsystems and subsystems are not in the same environment, remote calls are needed. Remote calls may be implemented using httpClient,Web Service and other technologies. However, more and more services are available in distributed systems.
In this case, maintaining these static configurations manually is a nightmare.
In order to solve the problem of service instance maintenance in microservice architecture, a large number of service governance frameworks have been generated. In Spring Cloud, Eureka is commonly used in our service governance framework.
Mainstream service registration and discovery: Eureka and zookeeper
Working Principle of Eureka
There are three roles in Eureka
1. Service Registry
Eureka is divided into client and server, which provides service registration and discovery.
2. Service Providers
Applications that provide services are registered in the Eureka registry for discovery by other applications.
3. Service consumers
Consumers obtain service lists from service registries, poll service lists through client load balancing algorithm, and then call the services they need, that is, service providers of invoking objects.
Note: In some cases, services are both providers and consumers, such as A calls B,B calls C, then B is both service providers and service consumers.
Eureka's Service Governance Mechanism
Service Registry
1. Failure Elimination
Sometimes the service instance exception (memory overflow, network failure, etc.) is offline, when the service registry does not receive a "service offline" request. In order to remove these instances from the list that cannot provide services, Eureka Server creates a timing task at startup, defaulting to every interval of time (default is 60). Seconds), excludes the service that has not been renewed in the current list due to the timeout (default 90 seconds).
2. Self-protection
During operation, Eureka Server counts whether the percentage of heartbeat failures is less than 85% within 15 minutes. If so, Eureka Server protects the current instance registration information so that these instances do not expire.
Service Provider
1. Service Registration
When the "service provider" starts up, it registers itself on Eureka Server by sending REST requests, along with some metadata information of its own services.
2. Service renewal
After registration, the service provider maintains a heartbeat and tells Eureka Server, "I'm still alive" to prevent it from being rejected by Eureka Server. By default, the renewal task interval is 30 seconds, the service failure time is 90 seconds, and the time can be set through the configuration file.
Service consumers
1. Get a list of services
If there are two service registries and two service provider instances (e.g. schematic diagram), when starting a service consumer, it will first send a REST request to obtain the list of services returned by the service registry. For performance reasons, Eureka Server maintains a read-only list of services. Return to the client and update the list every 30 seconds.
2. Service invocation
After the service consumer gets the list of services, the instance name and metadata information of the service provider can be obtained by the service name. Because of the detailed information of these service instances, the client can decide which instance to invoke according to its own needs. In Ribbon, it will invoke by polling by default, so as to achieve load balancing of the client.
code implementation
The code implementation in this chapter only embodies the concepts of service registry and service provider.
Realization schematic diagram

Dead work
Note: Note readers need to have a certain understanding of multi-module projects!
1. Create the main project
Create a master Maven project and introduce dependencies into the POM file, which acts as the parent POM file and relies on version control. Other module projects inherit the pom.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.6.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.cjz</groupId> <artifactId>fenbushi</artifactId> <version>0.0.1-SNAPSHOT</version> <name>fenbushi</name> <!-- Essential information --> <description>Distributed Framework Learning</description> <modelVersion>4.0.0</modelVersion> <packaging>pom</packaging> <!-- Module Description: Multiple sub-modules are declared here --> <modules> <module></module> <module></module> </modules> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies> <build> <plugins> </plugins> </build> </project>
Implementing Eureka in spring cloud
1. Create a registered service center (eureka-server)
Right-click on the main project to create model - > select spring initialir - > Next step
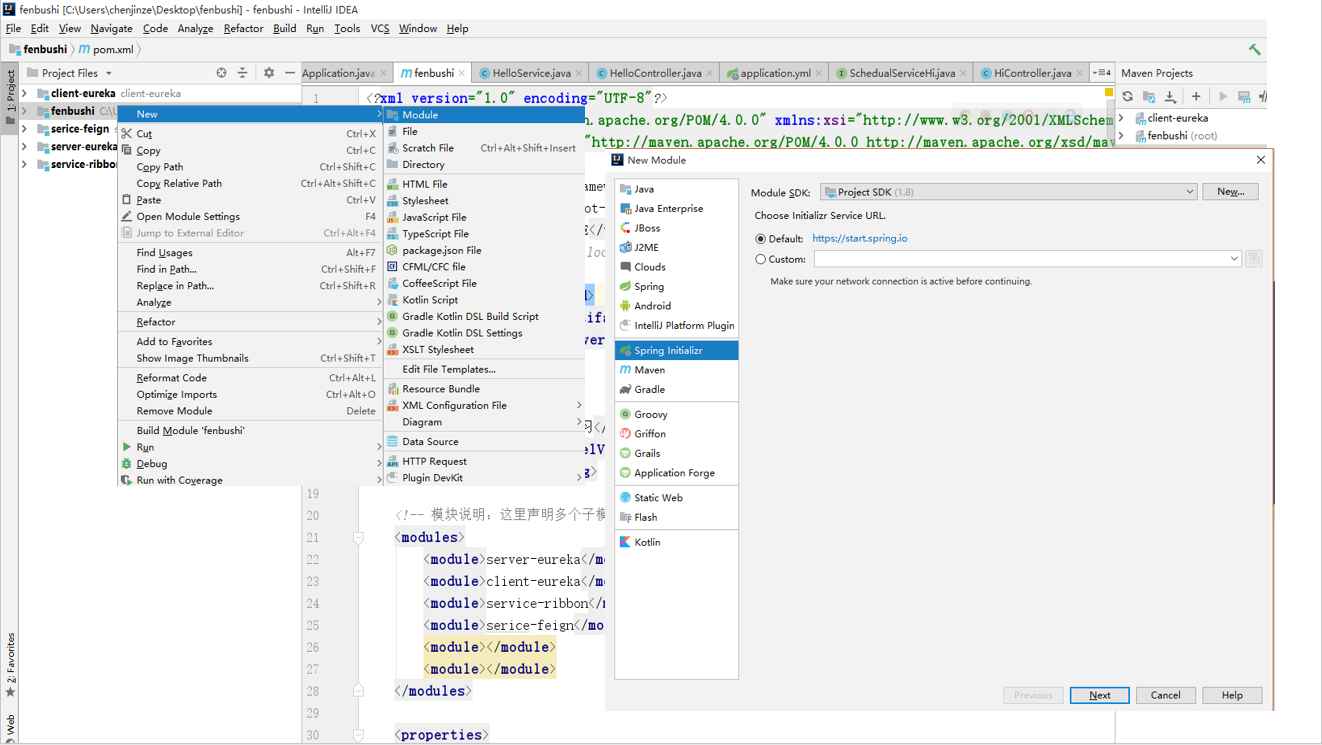
Select Cloud Discovery - > Eureka Server
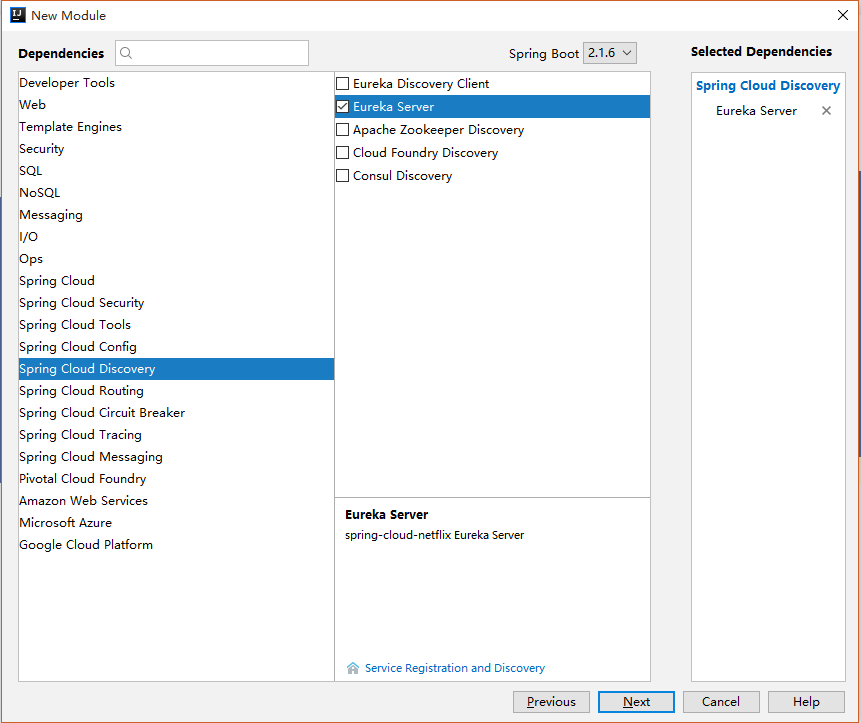
After the creation is completed, the parent pom file is inherited from the pom file of the eureka-server project and the dependency of spring-cloud-starter-netflix-eureka-server is checked.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <!-- Inheritance of the project's father project --> <parent> <groupId>com.cjz</groupId> <artifactId>fenbushi</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <groupId>com.cjz</groupId> <artifactId>server-eureka</artifactId> <version>0.0.1-SNAPSHOT</version> <name>server-eureka</name> <description>eureka - Service Registration and Discovery</description> <properties> <java.version>1.8</java.version> <spring-cloud.version>Greenwich.SR2</spring-cloud.version> </properties> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-server</artifactId> </dependency> </dependencies> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>${spring-cloud.version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
To start a service registry, you need an annotation @EnableEurekaServer, which needs to be added to the Startup application class of the springboot project:
@SpringBootApplication @EnableEurekaServer public class ServerEurekaApplication { public static void main(String[] args) { SpringApplication.run( ServerEurekaApplication.class, args ); } }
eureka is a highly available component. It has no backend cache. After each instance is registered, it needs to send a heartbeat to the registry (so it can be done in memory). By default, erureka server is also an eureka client, and a server must be specified. Ereka server configuration file appication.yml:
server: port: 8081 eureka: instance: hostname: localhost client: registerWithEureka: false fetchRegistry: false serviceUrl: defaultZone: http://${eureka.instance.hostname}:${server.port}/eureka/ spring: application: name: server-eurka
Express yourself as an eureka server through eureka.client.registerWithEureka: false and fetchRegistry: false.
Start the project and open browser access http://localhost:8081 Enter the Eureka interface.

No application available was not found because there was no registered service.
1. Create a service provider (eureka-client)
The creation process is similar to Cloud Discovery - > Eureka Discovery Client
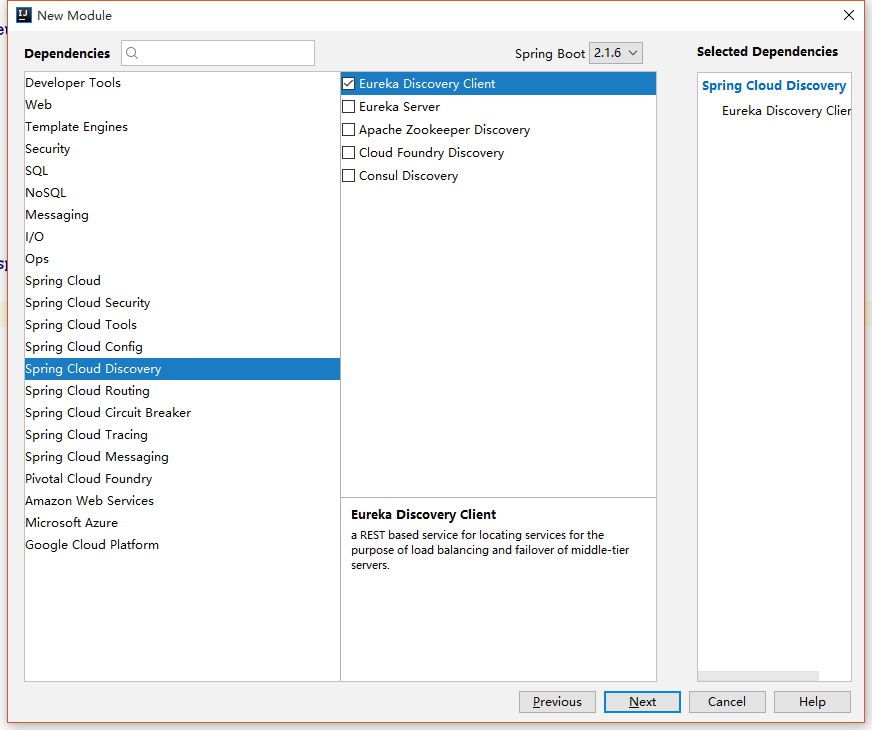
After the creation is completed, the parent pom file is inherited from the pom file of the eureka-client project and the dependency of spring-cloud-starter-netflix-eureka-client is checked.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <!-- Inheritance of the project's father project --> <parent> <groupId>com.cjz</groupId> <artifactId>fenbushi</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <groupId>com.cjz</groupId> <artifactId>client-eureka</artifactId> <version>0.0.1-SNAPSHOT</version> <name>client-eureka</name> <description>eureka - Service Provider</description> <properties> <java.version>1.8</java.version> <spring-cloud.version>Greenwich.SR2</spring-cloud.version> </properties> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> </dependencies> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>${spring-cloud.version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
The startup class adds the annotation @EnableEurekaClient to indicate that it is an eureka-client.
@SpringBootApplication @EnableEurekaClient public class ClientEurekaApplication { public static void main(String[] args) { SpringApplication.run( ClientEurekaApplication.class, args ); } }
Create a controller and add a service provided
@RestController public class HelloWordController { @Value( "${server.port}" ) private String prot; @RequestMapping("/hello") public String helloWord(){ return "hello ! I am " + prot + "Number Server."; } }
The address of your service registry is indicated in the configuration file. The application.yml configuration file is as follows:
#Port number server: port: 8082 #Service Provider Name spring: application: name: service-hello #Registered Service Registry Address eureka: client: serviceUrl: defaultZone: http://localhost:8081/eureka/
Start the project and open the eureka server's website: localhost:8081
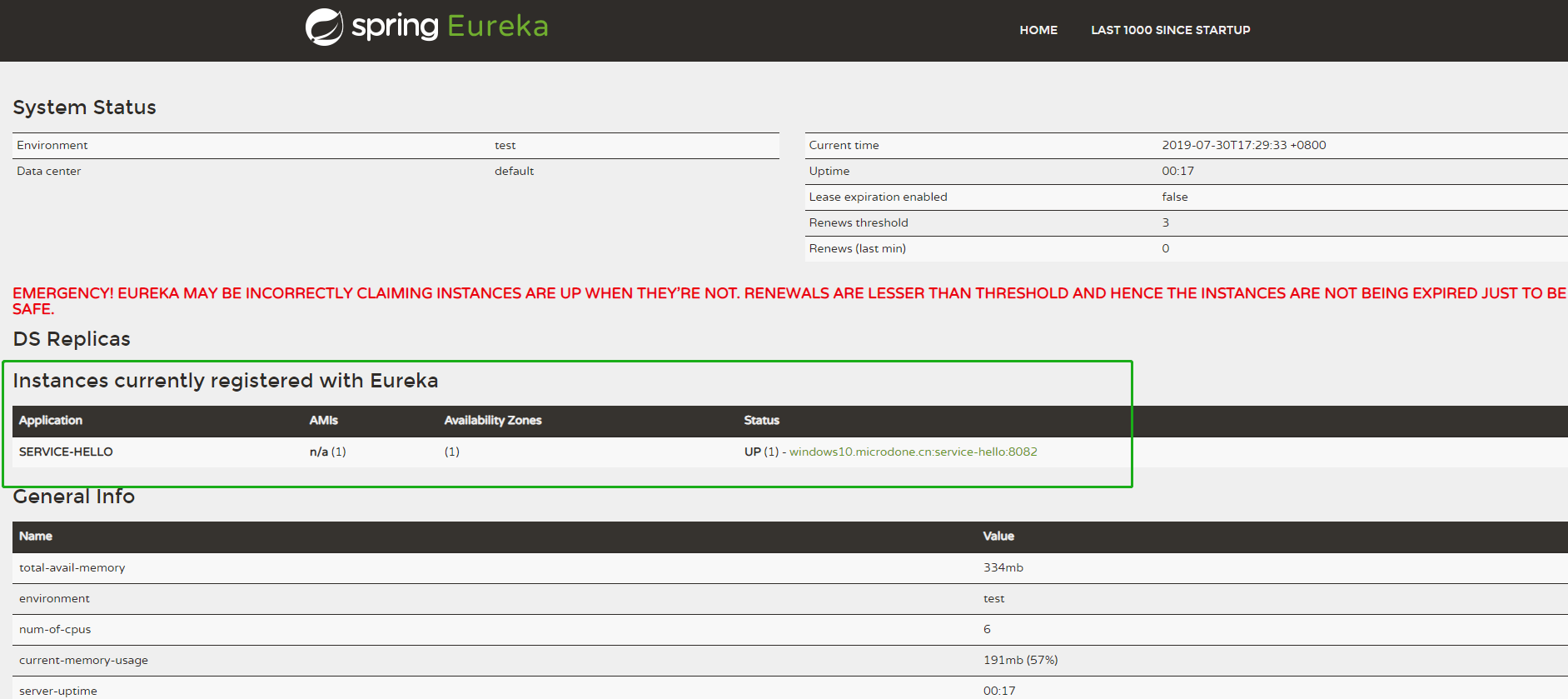
It was found that the service registry had registered a service named SERVICE-HELLO with a port of 8082.
Note: The red tips indicate that the self-protection mechanism has been opened.