Contents of this article
preface
Asset module is a module type that allows the use of resource files (fonts, icons, etc.) without configuring additional loader s.
Before webpack 5, we usually used:
- Raw loader imports the file as a string
- URL loader inline the file into the bundle as a data URI
- File loader sends the file to the output directory
Asset module type: replace all these loader s by adding four new module types:
- asset/resource sends a separate file and exports the URL. Previously, it was implemented by using file loader.
- asset/inline exports the data URI of a resource. Previously, it was implemented by using URL loader.
- asset/source export the source code of the resource. It was previously implemented by using raw loader.
- asset automatically selects between exporting a data URI and sending a separate file. Previously, it was realized by using URL loader and configuring resource volume limit.
The following examples verify the resource module type through different types of files.
Example structure:
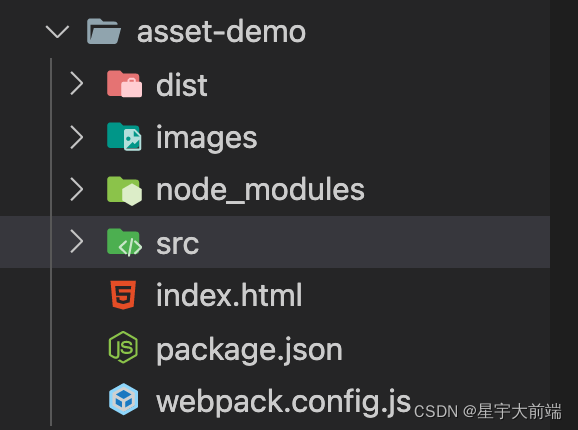
webpack basic configuration:
'use strict'; const path = require('path'); const svgToMiniDataURI = require('mini-svg-data-uri'); module.exports = { entry: './src/index.js', output: { path: path.resolve(__dirname, 'dist'), filename: 'bundle.js', }, module: { }, resolve: { }, devtool: 'source-map', plugins: [ ] };
index.html import the packaged js file:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> <script src="./dist/bundle.js"></script> </body> </html>
Resource mode
The function of Resource mode is to transport resources to the specified directory, modify the name, and its path will be injected into the bundle.
Example process description:
- Add img1 to the project PNG file
- webpack configures the loader and packages the png to the specified directory
- bundle.js automatically introduces the packaged image path
1. Import picture
Add img1 picture here
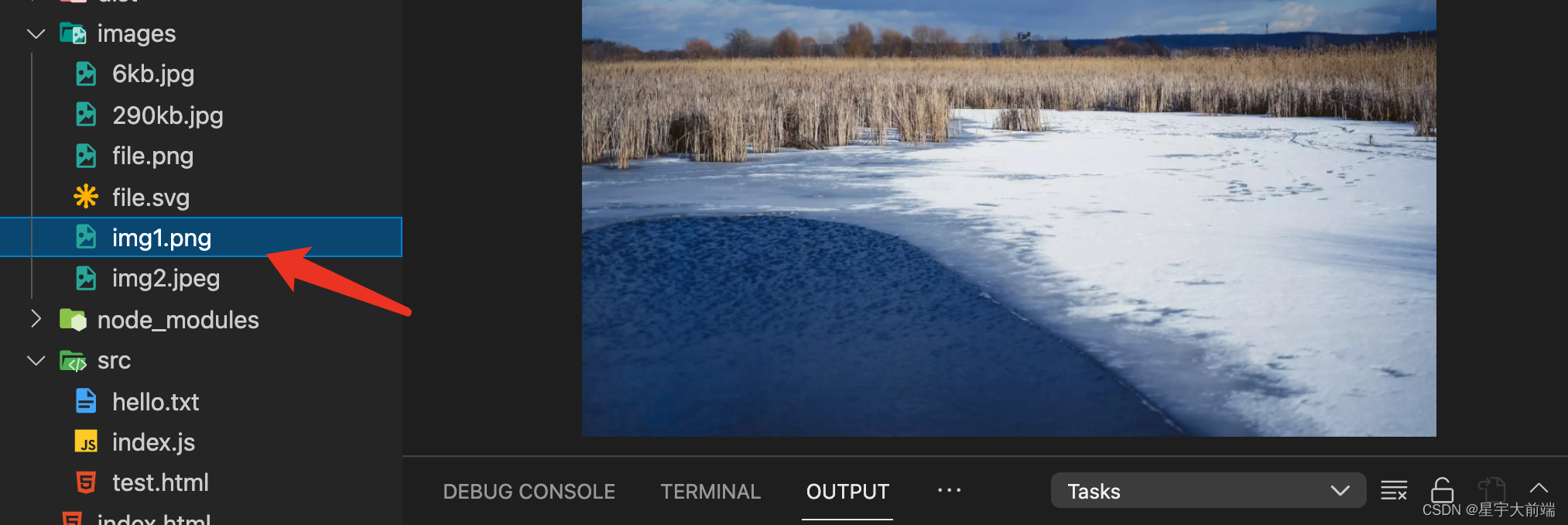
2,webpack.config configuration
webpack. In the config file, the configuration png file is in the Resource mode
module: { rules: [ { test: /\.png/, type: 'asset/resource' } }
Specify the folder after the pictures are packaged. If you do not specify the default packaging to the root directory.
output: { path: path.resolve(__dirname, 'dist'), filename: 'bundle.js', assetModuleFilename: 'images/[hash][ext][query]' },
3. Introduce photos and perform webpack packaging
The webpack package entry file is index JS, picture resources will be introduced here in the future.
index.js :
/* * @Author: ZY * @Date: 2022-02-16 10:44:46 * @LastEditors: ZY * @LastEditTime: 2022-02-16 14:55:09 * @FilePath: /webpack-demo/packages/asset-demo/src/index.js * @Description: Package resources */ import png from "../images/img1.png"; const container = document.createElement("div"); Object.assign(container.style, { display: "flex", flexDirection:'column', justifyContent: "center", }); document.body.appendChild(container); function createImageElement(title, src) { const div = document.createElement("div"); div.style.textAlign = "center"; const h2 = document.createElement("h2"); h2.textContent = title; div.appendChild(h2); const img = document.createElement("img"); img.setAttribute("src", src); img.setAttribute("width", "150"); div.appendChild(img); container.appendChild(div); } [png].forEach((src,index) => { const typeArray = ['resource'] createImageElement(typeArray[index], src); });
Results of webpack packaging:
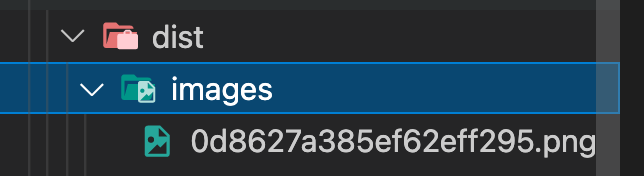
Self defined injection bundle JS:
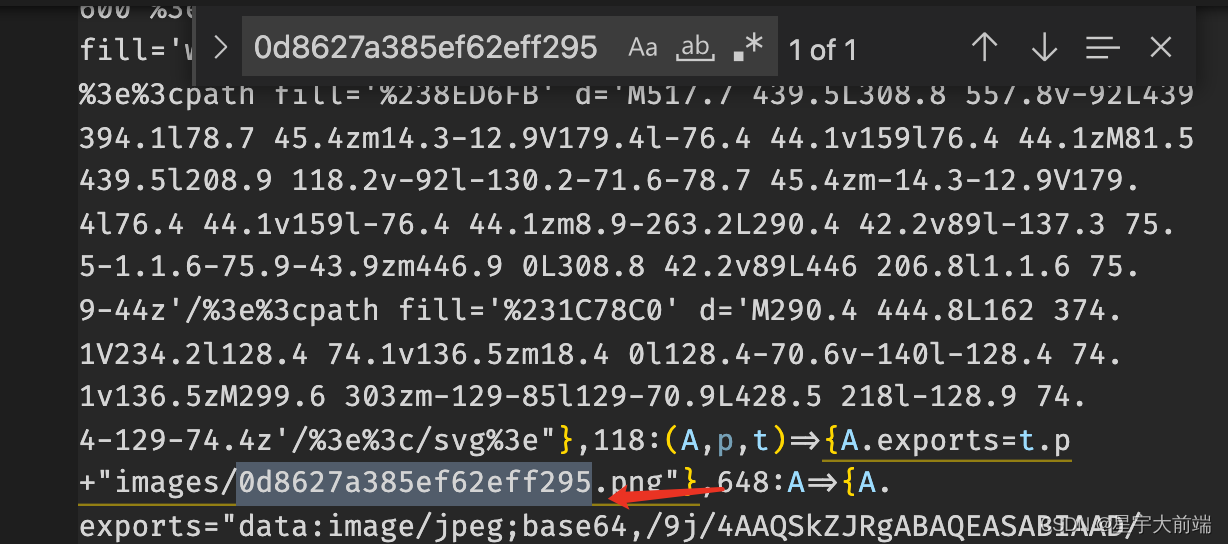
4. Another way to output file names
Take html files as an example. All html files will be output to the static Directory:
webpack.config file loader configuration
{ test: /\.html/, type: 'asset/resource', generator: { filename: 'static/[hash][ext][query]' } },
Execute webpack packaging results
Put a test and HTML file in the project directory and put it in the entry index JS file and execute webpack packaging.
The verification results are as follows:
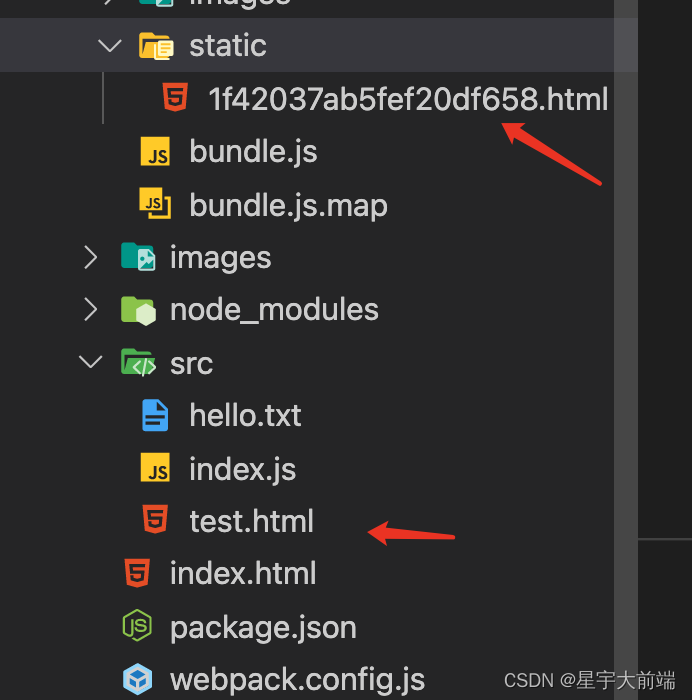
inline resource model
In the inline resource mode, by default, the image programming base64 format is injected into the bundle JS internal.
Inline mode takes jpeg type files as an example.
1. Import jpeg file
Import img2,jpeg file
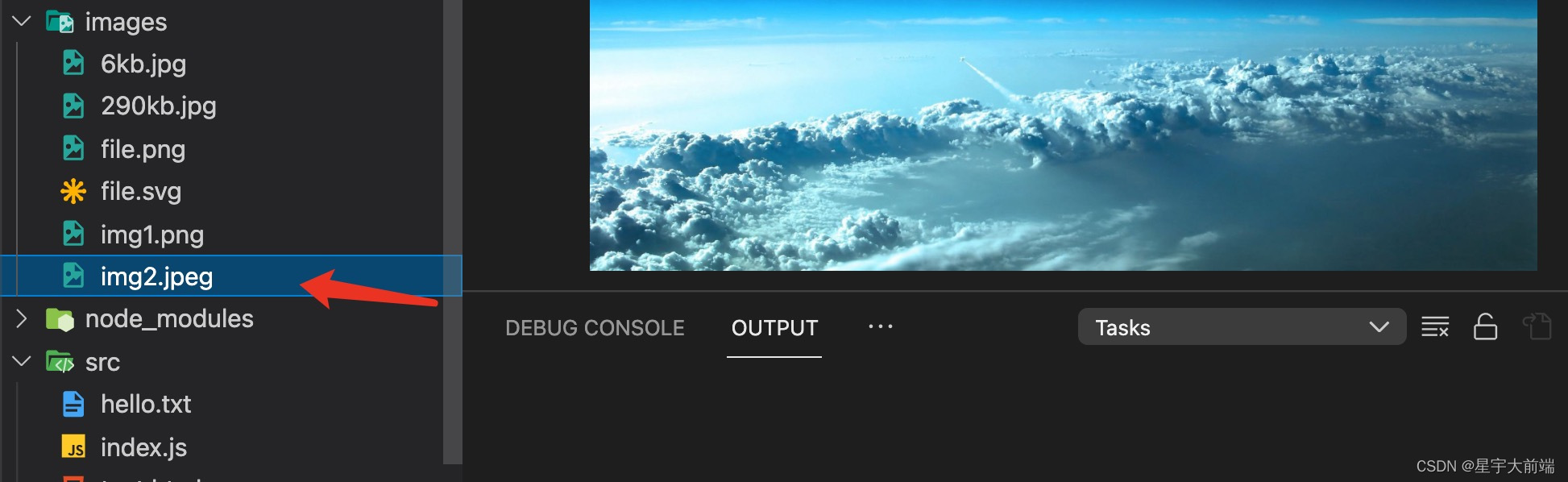
2,webpack.config configuration
{ test: /\.jpeg/, type: 'asset/inline' },
3. Import and package verification results
The picture becomes base64 and appears in bundle JS Li
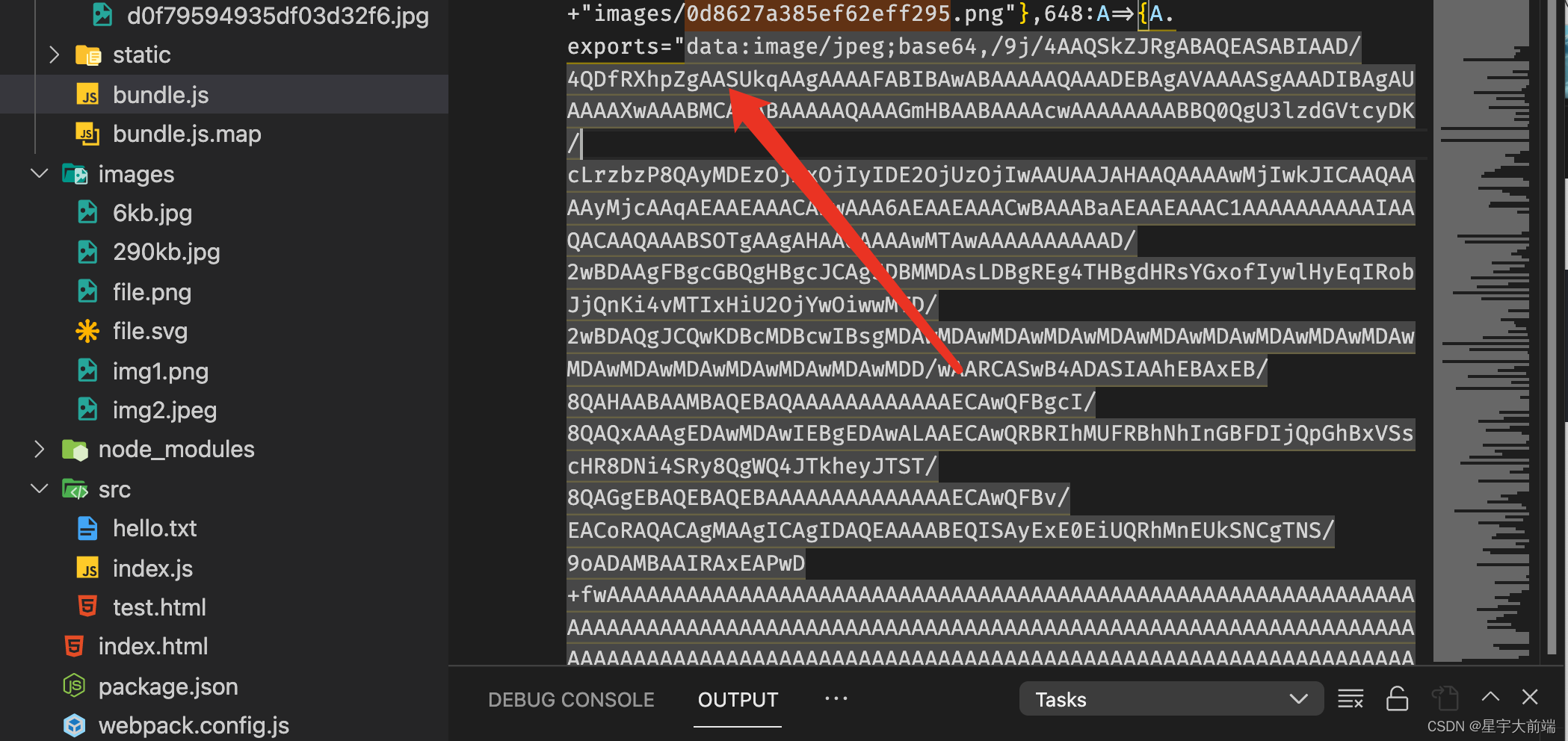
4. Custom data URI generator
The data URI output by webpack is presented as the file content encoded by Base64 algorithm by default.
If you want to use a custom encoding algorithm, you can specify a custom function to encode the file content,
Taking svg pictures as an example, mini svg data URI compression coding format is used
- Introducing svg pictures
- Introducing Mini SVG data URI package into npm
- Configure webpack config
/* * @Author: ZY * @Date: 2022-02-15 15:51:35 * @LastEditors: ZY * @LastEditTime: 2022-02-16 14:56:22 * @FilePath: /webpack-demo/packages/asset-demo/webpack.config.js * @Description: Document description */ 'use strict'; const svgToMiniDataURI = require('mini-svg-data-uri'); module.exports = { module: { rules: [ { test:/\.svg/, type: 'asset/inline', generator: { dataUrl: content => { content = content.toString(); return svgToMiniDataURI(content); } } }, ] }, };
source resource mode
Inject the file as it is into the packaged file, similar to the implementation of raw loader
Take txt file as an example
1. Create a new txt file and import it
New txt file

index.js import:
import helloText from "./hello.txt"; const text = document.createElement("h2"); text.textContent = helloText; container.appendChild(text);
2,webpack.config configuration
{ test:/\.txt/, type:'asset/source' },
3. Packaging validation
After packaging, the content is directly to the bundle js :
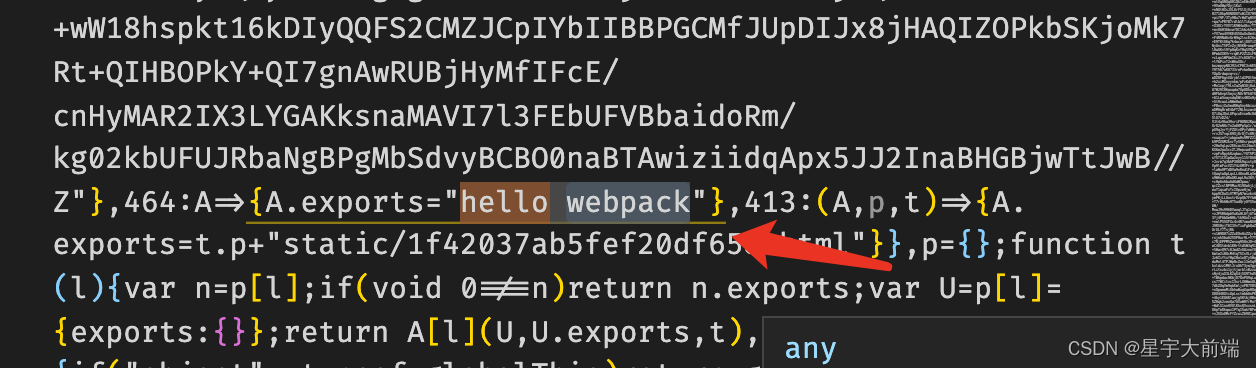
General mode
Now, webpack will automatically select between resource and inline according to the default conditions: files less than 8kb will be regarded as inline module type, otherwise they will be regarded as resource module type.
1. Import file
For better verification, files smaller than 8kb and files larger than 8kb are introduced
Take jpg file as an example
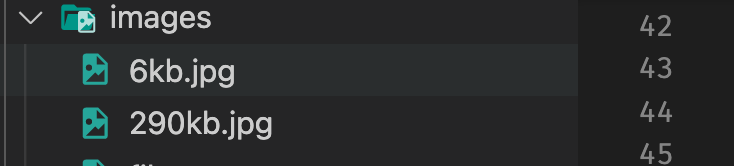
2. webpack config configuration
{ test:/\.jpg/, type:'asset' }
3. Packaging validation
dist only has file resources larger than 290kb, and those smaller than 8kb will be inlined. See the demo case for details.
summary
The introduction of resources in webpack5 kills a lot of loaders and changes them to type mode loaders, which is clearer. The examples in this article are detailed. For those not mentioned in the article, please check the demo.