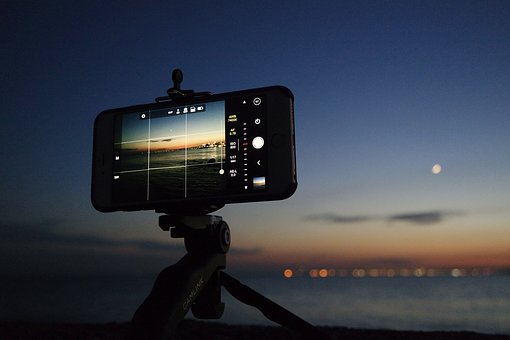
IO in Java
Classification of IO
The first is:
Input and output streams
The second is:
Byte stream and character stream
The third category:
Node Flow and Processing Flow
Node flow processes data, and processing flow is processed on the basis of node flow.
Focus in IO:
InputStream OutputStream FileInputStream FileOutputStream
InputStream
int read(byte[] b,int off,int len)
OutputStream
void write(byte[] b,int off,int len)
Talking about IO
//First: Input stream and output stream //The second type: byte stream character stream //Third: Node Flow Processing Flow //FileInputStream class Test{ public static void main(String args[]){ FileInputStream fis = null; try{ fis = new FileInputStream("e:/read.txt"); byte[] buffer = new byte[100]; fis.read(buffer,0,buffer.length); for(int i = 0;i<buffer.length;i++){ System.out.println(buffer[i]); } } catch(Exception e){ System.out.println(e); } } }
class Test{ public static void main(String args[]){ FileInputStream fis = null; FileOutputStream fos = null; try{ fis = new FileInputStream("e:/read.txt"); fos = new FileOutputStream("e:/write.txt"); byte[] buffer = new byte[100]; int temp = fis.read(buffer,0,buffer.length); fos.write(buffer,0,temp); } catch(Exception e){ System.out.println(e); } } }
class Test{ public static void main(String args[]){ FileInputStream fis = null; FileOutputStream fos = null; try{ fis = new FileInputStream("e:/read.txt"); fos = new FileOutputStream("e:/write.txt"); byte[] buffer = new byte[1024]; while(true){ int temp = fis.read(buffer,o,buffer.length); if(temp = -1){ break; } fos.write(buffer,0,temp); } }catch(Exception e){ System.out.println(e); }finally{ try{ fis.close(); fos.close(); }catch(Excepiton e){ System.out.println(e); } } } }
//Character stream public class TextChar public static void main(String args[]){ FileReader fr = null; FileWriter fw = null; try{ fr = new FileReader("e:/read.txt"); fw = new FileWriter("e:/write.txt"); char[] buffer = new char[100]; int temp = fr.read(buffer,0,buffer.length); fw.write(buffer,0,temp); } catch(Exception e){ System.out.println(e); }finally{ try{ fr.close(); fw.close(); } catch(Excepiton e){ System.out.println(e); } } }
//FileReader and Buffered Reader class Test{ public static void main(String args[]){ FileReader fileReader = null; BufferedReader bufferedReader = null; try{ fileReader = new FileReader("e:/read.txt"); bufferedReader = new BufferedReader(fileReader); String line = null; while(true){ line = bufferedReader.readLine(); if(line == null){ break; } System.out.println(line); } }catch(Exception e){ System.out.println(e); } finally{ try{ bufferedReader.close(); fileReader.close(); } catch(Exception e){ System.out.println(e); } } } }
public class Test{ public static void main(String[] args) throws Exception{ //Byte stream FileInputStream in = new FileInputStream("c:/read.txt"); FileOutStream out = new FileOutputStream("c:/write.txt"); byte[] buffer = new byte[1024]; int len; while( (len = in.read(buffer)) != -1){ out.write(buffer,0,len); } in.close(); out.close(); //Character stream BufferedReader bf = new BufferedReader(new FileReader("c:/read.txt"); BufferedWriter bw = new BufferedWriter(new FileWriter("c:/write.txt"); String str; while( (str=bf.readLine()) != null ){ bw.write(str); bw.newLine(); } bf.close(); bw.close(); } }
Byte stream: InputStream byte input stream, OutputStream byte output stream
Character stream: Reader character input stream, Writer character output stream
Data stream: Data Input Stream data input stream, Data Output Stream data output stream
File read and write
objective
1. Several Methods of Mastering Document Reading and Writing
Use of FileOutputStream and FileInputStream classes.
3 Conversion between basic data types
After reading the file, it is converted to uppercase and written to the target file, where src is the source file and des is the directory of the target file.
public class FileDemo { //Create a folder public static void createFolder(String path){ File folder=new File(path); if(folder.exists()){ System.out.println("The folder already exists!"); }else{ //Create when nonexistent folder.mkdir(); } } //Create a file public static void createFile(String path,String filename){ File file=new File(path,filename); //Document Judgement Is Existing if(file.exists()){ System.out.println("The file already exists!"); System.out.println(file.length()); }else{ try{ file.createNewFile(); }catch(IOException e){ System.out.println("File creation failed!"); } } } //Writing file public static void write(String path,String filename){ try{ String str="0123456789/nac"; String Upstr = str.toUpperCase();// byte b[]=Upstr.getBytes();// FileOutputStream fos=new FileOutputStream(new File(path,filename)); fos.write(b); fos.close(); }catch(FileNotFoundException e){ System.out.println("file does not exist"); }catch(IOException e){ System.out.println("Write file failed"); } } //read file public static void read(String path,String filename){ try{ int length=0; String str=""; byte buffer[]=new byte[10]; FileInputStream fis=new FileInputStream(new File(path,filename)); while((length=fis.read(buffer, 0, buffer.length)) !=-1){ str+=new String (buffer, 0, length); } System.out.println(str);// fis.close(); }catch(FileNotFoundException e){ System.out.println("file does not exist"); }catch(IOException e){ e.printStackTrace(); } } // public static void FileReaderCopy(String src,String des){ try{ FileReader fr=new FileReader(src); FileWriter fw=new FileWriter(des); char c[]=new char[1024]; int len=0; while((len=fr.read(c, 0, c.length)) != -1){ fw.write(c, 0, c.length); } fw.close(); fr.close(); } catch(FileNotFoundException e){ System.out.println("file does not exist"); }catch(IOException e){ System.out.println("Failure to read and write"); } } // public static void BufferedReaderCopy(String src,String des){ try{ BufferedReader br=new BufferedReader(new FileReader(src)); BufferedWriter bw=new BufferedWriter(new FileWriter(des)); String str=""; while((str=br.readLine()) != null){ String Upstr = str.toUpperCase();//Adding uppercase transformations bw.write(Upstr);// bw.newLine(); } bw.close(); br.close(); } catch(FileNotFoundException e){ System.out.println("File exists"); }catch(IOException e){ System.out.println("Failure to read and write"); } } //copy public static void copy(String src,String des){ try{ FileInputStream fis=new FileInputStream(src); FileOutputStream fos=new FileOutputStream(des); int c; while((c=fis.read()) != -1){ fos.write(c); } fos.close(); fis.close(); }catch(FileNotFoundException e){ System.out.println("file does not exist"); }catch(IOException e){ System.out.println("Failure to read and write"); } } //Copy file public static void copy1(String src,String des){ try{ FileInputStream fis=new FileInputStream(src); FileOutputStream fos=new FileOutputStream(des); int c; byte buff[]=new byte[1024]; while((c=fis.read(buff,0,buff.length)) != -1){ fos.write(buff,0,c); } fos.close(); fis.close(); }catch(FileNotFoundException e){ System.out.println("file does not exist"); }catch(IOException e){ System.out.println("Failure to read and write"); } } public static void main(String[] args) { // TODO Auto-generated method stub FileDemo.createFolder("c:/test"); FileDemo.createFile("c:/test", "1.txt"); FileDemo.write("c:/test", "1.txt"); FileDemo.read("c:/test", "1.txt"); FileDemo.read("c:/test", "FileDemo.java"); FileDemo.BufferedReaderCopy("c:/test/FileDemo.java", "c:/test/FileDemo2.java"); FileDemo.copy1("c:/test/1.mp3", "c:/test/2.mp3"); } }
read file
//read file public static void read(String path,String filename){ try{ int length = 0; String str = ""; byte buffer[]=new byte[10]; FileInputStream fis=new FileInputStream(new File(path,filename)); while((length=fis.read(buffer,0,buffer.length))!=-1){ str+=new String(buffer,0,length); } System.out.println(str); fis.close(); }catch(FileNotFoundException e){ System.out.println("file does not exist"); }catch(IOException e){ e.printStackTrace(); } }
File creation
public class FileDemo{ public static void createFolder(String path){ File folder = new File(path); if(folder.exists()){ System.out.println("file already exist!"); }else{ folder.mkdir(); } } public static void createFile(String path,String filename){ File file = new File(path,filename); if(file.exists()){ System.out.println("file already exist!"); System.out.println(file.length()); }else{ try{ file.createNewFile(); }catch(IOException e){ System.out.println("File creation failed"); } } } public static void main(String[] args){ FileDemo.createFolder("c:/test"); FileDemo.createFile("c:/test","1.txt"); } }
Writing file
public static void write(String path,String filename){ try{ String str = "234455"; byte b[] = str.getBytes(); FileOutputStream fos = new FileOutputStream(new File(path,filename)); fos.write(b); }catch(FileNotFoundException e){ System.out.println("file does not exist"); }catch(IOException e){ System.out.println("Write file failed"); } }
Reading and Writing of Documents
A key:
Main functions of file class: creating, reading, writing, deleting, etc.
File Read-Write Operation
Class File
Objects of File class
It is used to obtain the information of the file itself, such as the directory where the file is located, the length of the file, the permission of reading and writing the file, etc. It does not involve the operation of reading and writing the file.
Constructor
File(String filename) File(String directoryPath,String filename) File(File f,String filename)
Get the properties of the file
String getName() boolean canRead() boolean canWrite() long length() boolean isFile()etc.
Directory operation
boolean mkdir(): Create a directory. String[] list(): Returns all files in the directory as strings. File[] listFiles(): Returns all files in the directory as File objects.
File operation
boolean createNewFile(): Create a new file. boolean delete(): Delete a file
The concept of flow
Java input and output functions are implemented by means of input and output stream classes.
The java.io package contains a large number of classes to complete the input and output streams.
Classification of streams in Java:
The direction of flow can be divided into two kinds: input flow and output flow.
The data type of stream can be divided into byte stream and character stream.
Input stream classes are subclasses of either the abstract class InputStream (byte input stream) or the abstract class Reader (character input stream).
Output stream classes are subclasses of either the abstract class OutputStream (byte output stream) or the abstract class Writer (character output stream).
Input stream
Input stream is used to read data. Users can read data from input stream, but cannot write data.
The input stream reads the data as follows:
(1) Open a stream.
For example: FileInputStream inputFile=new FileInputStream("data source");
(2) Reading information from information sources.
For example: inputFile.read();
(3) Close the flow.
For example: inputFile.close();
output stream
The output stream is used to write data. Only write, not read.
The process of writing data to the output stream is as follows:
(1) Open a stream.
For example: FileOutputStream outFile=new FileOutputStream("data source");
(2) Write the information to the destination.
For example: outFile.write(inputFile.read()):
(3) Close the flow. Such as:
For example: outFile.close();
IO
- Objectives of I/O operations
- Classification of IO
- Method of reading and writing files
Objectives of I/O operations
The goal is to read data from the data source and write it to the data destination.
Input from one place to output from a java program to another place.
File and Data Flow
Write and read out data files. The input/output operations in Java are in the form of data streams. The two forms of data streams are 16-bit characters or 8-bit bytes.
Operating objects of data streams:
Reading and Writing of Data Files Data transmission between threads Data transmission between networks
Read/write steps:
Import the input/output package, import the java.io. * package
Create file objects
FileReader/FileWriter class for reading and writing text files
Data InputStream (for file reading) and Data OutputStream (for file writing) class files read and write
FileInputStream (for file readout) and FileOutputStream objects (for file writing)
Close file
close function closes files
Properties of File Objects
create a file
boolean createNewFile();
create subdirectory
boolean mkdir(); boolean mkdirs();
Rename/move files
boolean renameTo(File dest);
Delete files
boolean delete();
Check whether the file exists
boolean exists();
Check whether it is a document or not
boolean isFile();
Check whether it is a folder or not
boolean isDirectory();
Query file length
long length();
For the rest of my life, you are the only one.
Brief Book Author: Dashu Xiaosheng
Post90s handsome young man, good development habits; ability to think independently; initiative and good communication
Brief Book Blog: https://www.jianshu.com/u/c785ece603d1
epilogue
- Next, I will continue to explain other knowledge in depth. I am interested in continuing to pay attention to it.
- A little gift or a little compliment