1. Cookie operations related to WebDriver
- get_cookies(): get all cookies of the current domain name in the current session
- get_cookie(name): obtain the cookie value corresponding to the specified name of the current domain name in the current session
- delete_cookie(name): deletes the specified cookie
- delete_all_cookies(): delete all cookie s
- add_cookie(self, cookie_dict): add a cookie
1.1 when adding cookies, you need to add them one by one_ Dict example:
- driver.add_cookie({'name' : 'foo', 'value' : 'bar'})
- driver.add_cookie({'name' : 'foo', 'value' : 'bar', 'path' : '/'})
- driver.add_cookie({'name' : 'foo', 'value' : 'bar', 'path' : '/', 'secure':True})"
2. How to add cookie s to bypass login
Take Baidu as an example
- Let's log in to Baidu first, for example, and view the Application through F12. BDUSS is a cookie.
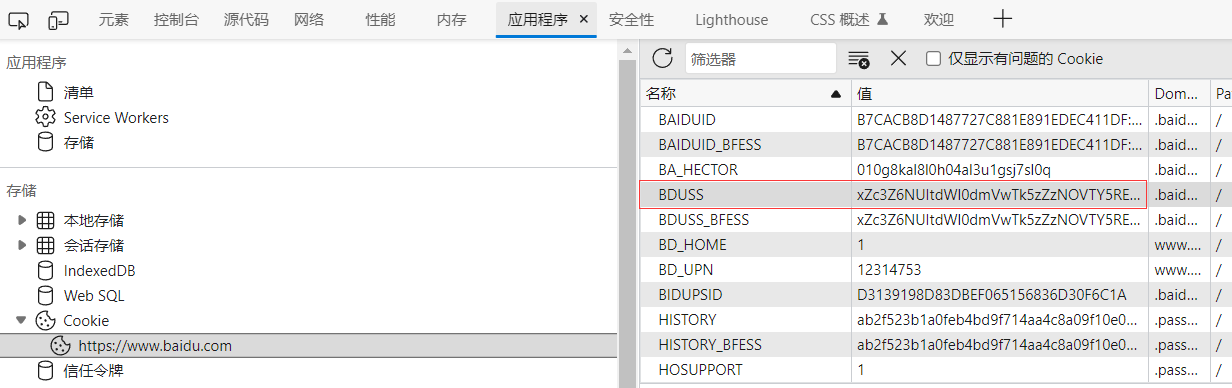
From the figure, you can find: name= "BDUSS",value = "3R-T21PamZseEJmLVlmQ1ZNS2ZRQ3VPeUJE"
Construct cookie dictionary
cookie = {'name' : 'BDUSS', 'value' : '3R-T21PamZseEJmLVlmQ1ZNS2ZRQ3VPeUJEVUtXUE**'}
Then call: driver.add_cookie(cookie_dict=cookie)
from selenium import webdriver import time driver = webdriver.Chrome() driver.maximize_window() time.sleep(1) cookie = {"name":"BDUSS", "value":"3R-T21PamZseEJmLVlmQ1ZNS2ZRQ3VPeUJEVUtXUEoxUkx2ODVTZ1REZmczOVZmSVFBQUFBJCQAAAAAAAAAAAEAAAA1p7iuyeTI1WluZwAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAOBSrl~gUq5fb"} driver.add_cookie(cookie_dict=cookie) driver.get("https://www.baidu.com/") time.sleep(10) driver.quit()
Traceback (most recent call last): File "I:/python/softwaredate/py_basics/1****/14 selenium cookie Bypass login.py", line 10, in <module> driver.add_cookie(cookie_dict=cookie) File "D:\software\python38\lib\site-packages\selenium\webdriver\remote\webdriver.py", line 894, in add_cookie self.execute(Command.ADD_COOKIE, {'cookie': cookie_dict}) File "D:\software\python38\lib\site-packages\selenium\webdriver\remote\webdriver.py", line 321, in execute self.error_handler.check_response(response) File "D:\software\python38\lib\site-packages\selenium\webdriver\remote\errorhandler.py", line 242, in check_response raise exception_class(message, screen, stacktrace) selenium.common.exceptions.InvalidCookieDomainException: Message: invalid cookie domain (Session info: chrome=85.0.4183.102)**
If an error is found, how to solve it:
Just add an address to open the web page above the cookie
from selenium import webdriver import time driver = webdriver.Chrome() driver.maximize_window() time.sleep(1) cookie = {"name":"BDUSS", "value":"3R-T21PamZseEJmLVlmQ1ZNS2ZRQ3VPeUJEVUtXUEoxUkx2ODVTZ1REZmczOVZmSVFBQUFBJCQAAAAAAAAAAAEAAAA1p7iuyeTI1WluZwAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAOBSrl~gUq5fb"} driver.get("https://www.baidu.com/") #Just add this line driver.add_cookie(cookie_dict=cookie) driver.get("https://www.baidu.com/") time.sleep(10) driver.quit()
The above method can bypass login, but pay attention to the following two points:
- The account generating the cookie cannot have an exit operation, otherwise the cookie will become invalid.
- Note the validity period of the cookie (expires / max age column). It may be necessary to update the cookie regularly
3. Interface login to obtain cookies and construct them into cookies_ Add dict to add_cookie
import requests from selenium import webdriver from time import sleep def get_cookie(username, password): login_url = 'http://****/newecshop/admin/privilege.php' data = dict(username=username,password=password, act='signin') res = requests.post(login_url, data=data, allow_redirects=False) esscp_id = res.cookies.get('ECSCP_ID') cookie = dict(name='ECSCP_ID',value=esscp_id) return cookie admin_url = 'http://****/newecshop/admin/index.php' driver = webdriver.Chrome() driver.get(admin_url) cookie = get_cookie(****, ****) driver.add_cookie(cookie) driver.get('http://***/newecshop/admin/order.php?act=list') sleep(3) driver.quit()
4. Launch Chrome browser to bypass login
Each time we open the browser for corresponding operations, the corresponding cache and cookie s will be saved to the default path of the browser. Let's check the personal data path first. Take chrome as an example, we enter it in the address bar chrome://version/
The profile path in the figure is what we need. We remove the following \ Default, and then add "– user data dir =" before the path to splice the path we want.
profile_directory = r'--user-data-dir=C:\Users\xxx\AppData\Local\Google\Chrome\User Data'
Next, when we start the browser, we start it with options. Note that before running the code, we need to close all running chrome programs, otherwise an error will be reported. All codes are as follows.
from selenium import webdriver import time import os,platform if platform.system() =="Windows": os.system("taskkill -im chrome* -f") # hold chrome Kill all the processes at the beginning else: os.system("killall -9 chrome*") # Get user directory my_dir = os.path.expanduser("~") profile_directory = r'--user-data-dir={}\AppData\Local\Google\Chrome\User Data'.format(my_dir) print(profile_directory) option = webdriver.ChromeOptions() option.add_argument(profile_directory) driver = webdriver.Chrome(chrome_options=option) driver.get("https://www.baidu.com/") time.sleep(2)
After selenium automation starts the browser, we will find that the bookmarks I saved before are completely above the browser, and the baidu account is also logged in.
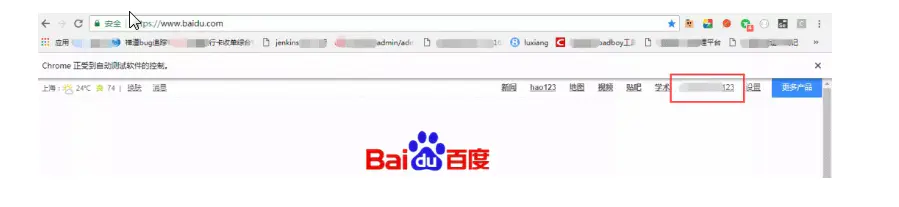
5. Website bypassing graphic verification code
The first figure in the article is the graphic verification code when the simple book logs in. After we log in to the simple book (the cookie has a certain time limit, which seems to be about 10 days and a half months), we change the link in the above code into the simple book, and then use the above method to realize the graphic verification code that bypasses the login page.
Original link:
Link: https://www.jianshu.com/p/d442a3cbef3a