1, Servlet
1.1 introduction to Servlet
- Servlet is a technology for sun company to develop dynamic web
- sun provides an interface called Servlet in these API s. If you want to develop a Servlet program, you only need to complete two small steps:
1. Write a class to implement the Servlet interface
2. Deploy the developed Java classes to the web server. - The Java program that implements the Servlet interface is called Servlet
1.2 introduction to servlet method
- The initialization method is executed only once when the servlet is created
void init(ServletConfig config)
- Provide a service method, which will be called every time the Servlet is accessed
void service(ServletRequest req,ServletResponse res)
- Destroy method, which is called when the Servlet is destroyed. Destroy the Servlet when memory is freed or the server is shut down
void destory()
- Get ServletConfig object
ServletConfig getServletConfig()
- Get Servlet information
String getServleInfo()
1.3 configuring servlets using XML
-
Servlet interface Sun has two default implementation classes: httpservlet and genericservlet
-
Configuration method of Java < URL pattern >
1. Full path configuration
Start with / for example: / hello / servlet01 hello
2. Directory matching
Start with / and end with / for example: / / aaa/*
3. Extension matching
Cannot start with /, start with *, for example: * action *. jsp
Steps:
- Write an ordinary class to inherit HtttpServlet
HelloServlet.java
package Servlet01; import java.io.IOException; import java.io.PrintWriter; import jakarta.servlet.ServletException; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse; public class HelloServlet extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { PrintWriter out=resp.getWriter(); out.print("hello servlet"); } @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { } }
- Write Servlet mapping
(why mapping is needed: we write Java programs, but we need to access them through the browser, and the browser needs to connect to the web server, so we need to register the Servlet we write in the web service and give it a path that the browser can access)
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:web="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd http://java.sun.com/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee" id="WebApp_ID" version="2.4"> <display-name>test01</display-name> <!-- servlet Label to Tomcat to configure servlet program --> <servlet> <!-- servlet-name Label to Servlet The program has an alias (usually a class name) --> <servlet-name>HelloServlet</servlet-name> <!-- servlet-class yes servlet The full class name of the program --> <servlet-class>Servlet01.HelloServlet</servlet-class> </servlet> <!-- servlet-mapping Label to servlet Program configuration access address --> <servlet-mapping> <!-- servlet-name The tag is used to tell the server which address I currently configure servlet Program use --> <servlet-name>HelloServlet</servlet-name> <!-- url-pattern Label configuration access address / The slash indicates when the server parses http://ip:port / Project path /hello Indicates that the address is http://ip:port / Project path / hello --> <url-pattern>/hello</url-pattern> </servlet-mapping> </web-app>
-
Access path
http://localhost:8080/test01/hello
-
Result diagram
1.4 configuring servlets with annotations
Method: @ WebServlet("/demo01")
ServlertDemo01.java
package Servlet02; import java.io.IOException; import java.io.PrintWriter; import jakarta.servlet.ServletException; import jakarta.servlet.annotation.WebServlet; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse; @WebServlet("/demo01") public class ServlertDemo01 extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { resp.setContentType("text/html;charset=UTF-8"); PrintWriter out=resp.getWriter(); out.print("hello Annotation configuration servlet"); 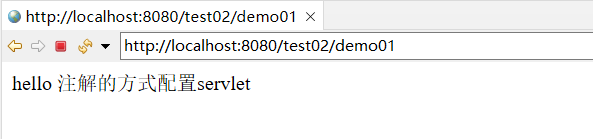 } @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { // TODO Auto-generated method stub super.doPost(req, resp); } }
Result diagram
1.5 Mapping problems
- A servlet can specify a mapping path
<servlet-mapping> <servlet-name>hello</servlet-name> <url-pattern>/hello</url-pattern> </servlet-mapping>
- A servlet can specify multiple mapping paths
<!--Servlet Request path for--> <servlet-mapping> <servlet-name>hello</servlet-name> <url-pattern>/hello</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>hello</servlet-name> <url-pattern>/hello1</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>hello</servlet-name> <url-pattern>/hello2</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>hello</servlet-name> <url-pattern>/hello3</url-pattern> </servlet-mapping>
- A servlet can specify multiple mapping paths
<!--Servlet Request path for--> <servlet-mapping> <servlet-name>hello</servlet-name> <url-pattern>/hello/*</url-pattern> </servlet-mapping>
- Default request path
<!--Servlet Request path for--> <servlet-mapping> <servlet-name>hello</servlet-name> <url-pattern>/*</url-pattern> </servlet-mapping>
- Specify some suffixes or prefixes, etc
<!--Servlet Request path for--> <servlet-mapping> <!--You can customize the suffix to implement request mapping Note:*Path that cannot be preceded by item mapping (slash) --> <servlet-name>hello</servlet-name> <url-pattern>*.do</url-pattern> </servlet-mapping>
- Priority issues
The inherent mapping path is specified with the highest priority. If it cannot be found, the default processing request is taken.
1.6 ServletContext
When the web container starts, it will create a corresponding ServletContext object for each web application, which represents the current web application;
1.6.1 shared data
The data I saved in this servlet can be obtained in another servlet:
servlet to save data
package com.yan.study; import jakarta.servlet.ServletContext; import jakarta.servlet.ServletException; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse; import java.io.IOException; public class HelloServlet extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { //this.getInitParameter() initialization parameter //this. Getservletconfig() servlet configuration //this. Getservletcontext() servlet context ServletContext context=this.getServletContext(); String uname="Xiaoyan"; context.setAttribute("username",uname);//Save a data in the ServletContext with the name username and the value uname } }
servlet to get data (GetServlet)
package com.yan.study; import jakarta.servlet.ServletContext; import jakarta.servlet.ServletException; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse; import java.io.IOException; public class GetServlet extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { ServletContext context=this.getServletContext(); String username=(String) context.getAttribute("username"); resp.setContentType("text/html"); resp.setCharacterEncoding("utf-8"); resp.getWriter().print("name:"+username); } }
XML for configuring servlet s
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd" > <web-app> <display-name>Archetype Created Web Application</display-name> <servlet> <servlet-name>hello</servlet-name> <servlet-class>com.yan.study.HelloServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>hello</servlet-name> <url-pattern>/hello</url-pattern> </servlet-mapping> <servlet> <servlet-name>get</servlet-name> <servlet-class>com.yan.study.GetServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>get</servlet-name> <url-pattern>/get</url-pattern> </servlet-mapping> </web-app>
Test result diagram
1.6.2 obtaining initialization parameters
Configuration XML
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd" > <web-app> <display-name>Archetype Created Web Application</display-name> <!--Configure some web Application initialization parameters--> <context-param> <param-name>url</param-name> <param-value>jdbc:mysql://localhost:3306/mybatis</param-value> </context-param> <servlet> <servlet-name>gp</servlet-name> <servlet-class>study.ServletDemo03</servlet-class> </servlet> <servlet-mapping> <servlet-name>gp</servlet-name> <url-pattern>/gp</url-pattern> </servlet-mapping> </web-app>
ServletDemo03
package study; import jakarta.servlet.ServletContext; import jakarta.servlet.ServletException; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse; import java.io.IOException; public class ServletDemo03 extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { ServletContext context=this.getServletContext(); String url=context.getInitParameter("url"); resp.getWriter().print(url); } }
test result
1.6.3 request forwarding
- Write a ServletDemo04 and forward it to ServletDemo03
ServletDemo04
package study; import jakarta.servlet.RequestDispatcher; import jakarta.servlet.ServletContext; import jakarta.servlet.ServletException; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse; import java.io.IOException; public class ServletDemo04 extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { ServletContext context=this.getServletContext(); System.out.println("Entered ServletDemo04"); RequestDispatcher requestDispatcher=context.getRequestDispatcher("/gp");//Forwarded request path requestDispatcher.forward(req,resp);//Call forward to forward the request } }
Configuration xml
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd" > <web-app> <display-name>Archetype Created Web Application</display-name> <!--Configure some web Application initialization parameters--> <context-param> <param-name>url</param-name> <param-value>jdbc:mysql://localhost:3306/mybatis</param-value> </context-param> <servlet> <servlet-name>gp</servlet-name> <servlet-class>study.ServletDemo03</servlet-class> </servlet> <servlet-mapping> <servlet-name>gp</servlet-name> <url-pattern>/gp</url-pattern> </servlet-mapping> <servlet> <servlet-name>demo04</servlet-name> <servlet-class>study.ServletDemo04</servlet-class> </servlet> <servlet-mapping> <servlet-name>demo04</servlet-name> <url-pattern>/demo04</url-pattern> </servlet-mapping> </web-app>
test result
1.7 HttpServletResponse
The web server receives the http request from the client. For this request, create an HttpServletRequest object representing the request to respond to an HttpServletResponse;
- If you want to get the parameters requested by the client: find HttpServletRequest
- If you want to respond to the client with some information: find HttpServletResponse
Redirect [resp.sendRedirect();]
- Redirect to GetServlet when accessing RedirectServlet
RedirectServlet
package com.yan.study; import jakarta.servlet.ServletException; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse; import java.io.IOException; public class RedirectServlet extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { resp.sendRedirect("/servlet01_war/get"); } @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { } }
Configuration XML
<servlet> <servlet-name>red</servlet-name> <servlet-class>com.yan.study.RedirectServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>red</servlet-name> <url-pattern>/red</url-pattern> </servlet-mapping>
Result chart display
What is the difference between redirection and forwarding?
The same thing: the page will jump
difference:
The url will not change when the request is forwarded
When redirecting, the url address will change
1.8 HttpServletRequest
HttpServletRequest represents the request of the client. The user accesses the server through the HTTP protocol. All the information in the HTTP request will be encapsulated in HttpServletRequest. All the information of the client will be obtained through the method of HttpServletRequest.
1.8.1 obtain parameters and request forwarding
- RequestDemo01 a servlet from form JSP, and redirect to success JSP
form.jsp
<%--<%@ page contentType="text/html;charset=UTF-8" language="java" %>--%> <%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false"%> <html> <head> <title>Title</title> </head> <body> <%--${pageContext.request.contextPath}Represents the current project--%> <form action="${pageContext.request.contextPath}/demo01" method="get"> user name:<input type="text" name="username"> password:<input type="password" name="password"> <input type="submit"> </form> </body> </html>
success.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Title</title> </head> <body> <h1>success</h1> </body> </html>
ResquestDemo01
package com.yan.study; import jakarta.servlet.ServletException; import jakarta.servlet.annotation.WebServlet; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse; import java.io.IOException; @WebServlet(urlPatterns = "/demo01") public class RequestDemo01 extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { String uname=req.getParameter("username"); String psw=req.getParameter("password"); System.out.println(uname+";"+psw); resp.sendRedirect("/test01_war/success.jsp"); } @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { super.doPost(req, resp); } }
Display of result graph (the first graph is the result of running form.js, and the second graph is the result of redirecting to success.jsp)
Figure 1:
Figure 2:
2, Cookie, Session
- Session: users open a browser, click many hyperlinks, access multiple web resources, and close the browser. This process can be called session.
Two techniques for saving sessions
- cookie
Client Technology (response, request) - session
Server technology. Using this technology, we can save the user's session information. We can put the information or data in the session.
2.1 Cookie
- Get cookie information from the request
- The server responds to the client cookie
Cookie[] cookies =req.getCookies();//Get cookie cookie.getName(); //Get the key of the cookie cookie.getValue(); //Get the value of the cookie Cookie cookie= new Cookie("name","value"); //Create a new cookie cookie.setMaxAge(24*60*60); //Set the validity period of the cookie resp.addCookie(cookie);//Respond to a cookie to the client
2.2 Session
What is a Session:
- The server will create a session object for each user (browser);
- A session monopolizes a browser. As long as the browser is not closed, the session exists;
- After the user logs in, the whole website can be accessed. (save user information)
The difference between Session and cookie:
- Cookie is to write the user's data to the user's browser, and the browser saves it (multiple can be saved)
- Session writes the user's data to the user's exclusive session and saves it in the server (saves important information and reduces the waste of server resources)
- The Session object is created by the server.
Using session
package com.yan.study; import jakarta.servlet.ServletException; import jakarta.servlet.annotation.WebServlet; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse; import jakarta.servlet.http.HttpSession; import java.io.IOException; @WebServlet(urlPatterns = "/sd") public class SessionDemo01 extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { //Solve the problem of garbled code resp.setContentType("text/html"); req.setCharacterEncoding("utf-8"); resp.setCharacterEncoding("utf-8"); //Get session HttpSession session= req.getSession(); //Save something in the session session.setAttribute("name",new Person("Xiaoyan",20)); //Gets the ID of the session String id=session.getId(); //Judge whether the Session is newly created if(session.isNew()){ resp.getWriter().write("session Created successfully, ID: "+id); }else { resp.getWriter().write("session Already exists on the server, ID: "+id); } } @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { super.doPost(req, resp); } }
Session auto Expiration: Web XML configuration
<!--set up session Default effective time--> <session-config> <!-- 15 Minutes later, session Automatic effect, in minutes--> <session-timeout>15</session-timeout> </session-config>