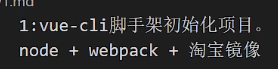
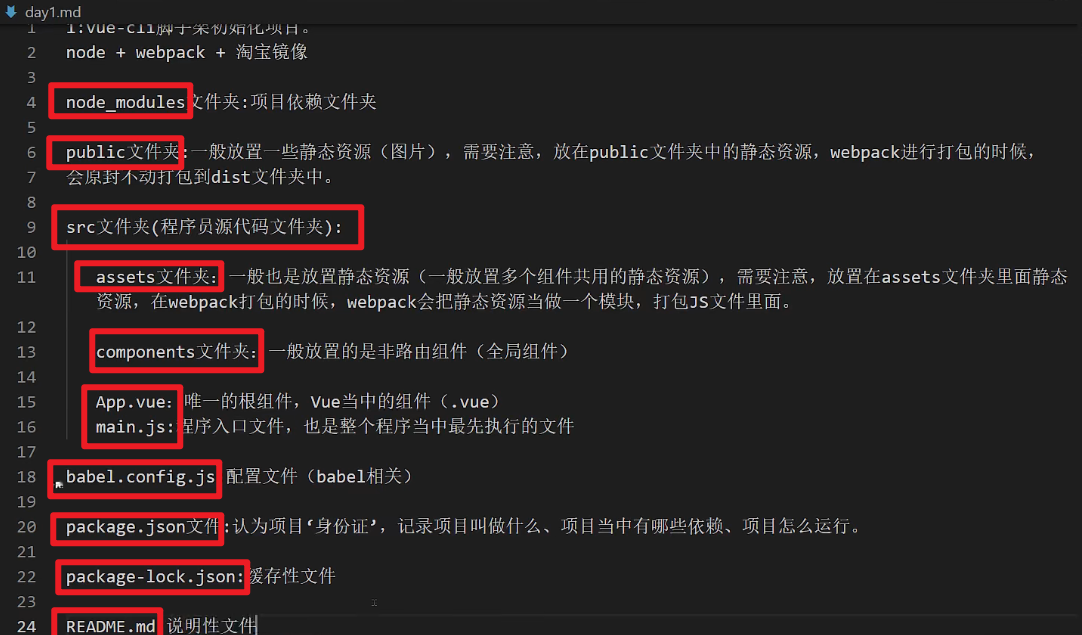
1 create project
vue create app
If the vue cli version is below 3, it needs to be upgraded. create is the usage of 3
npm uninstall -g vue-cli npm install -g @vue/cli npm install -g @vue/cli@4.5.13
Note: to use Vue cli v4 five point one three
Vue cli v4 5.13 I installed Vue cli V5 0.1, there is a problem when configuring to automatically open the browser - open, so use the version in the video
2 open the project and automatically open the browser
$ cd app $ npm run serve
In. Package JSON -- > scripts add - open
// "serve": "vue-cli-service serve", "serve": "vue-cli-service serve --open",//Browser opens automatically
3 use of scaffold
There are two ways to create a project:
vue init webpack Name of the project vue create entry name
Scaffold Directory: public + assets folder
node_modules:Place where the project depends public:Generally, some shared static resources are placed. When they are packaged and put online, public The resources in the folder are packed intact dist Inside the folder src: Programmer source folder -----assets Folder: often place some static resources (pictures), assets Resources in folder webpack Will be packaged into a module( js (inside the folder) -----components folder:Generally, non routing components (or components shared by the project) are placed App.vue Unique root component main.js Entry document [the document first executed by the program] babel.config.js:babel configuration file package.json: See project description, project dependency and project operation instructions README.md:Project description document
Configure the items downloaded from the scaffold slightly
3.1: browser opens automatically
stay package.json In the file "scripts": { "serve": "vue-cli-service serve --open", "build": "vue-cli-service build", "lint": "vue-cli-service lint" },
3.2 close the eslint verification tool
Create Vue config. JS file: need to be exposed
module.exports = { lintOnSave:false, }
3.3 setting of SRC folder alias
(jsconfig.json is configured for vscode. If there is a vehicle plug-in, this file will also be read. The path alias in the project is configured in webpack)
Because when the project is large, src (source code folder): there will be many directories in it, so it is inconvenient to find files. The advantage of setting the alias of src folder is that it will be easier to find files
Create jsconfig JSON file, placed in the root directory
{ "compilerOptions": { "baseUrl": "./", "paths": { "@/*": [ "src/*" ] } }, "exclude": [ "node_modules", "dist" ] }
If an error is reported, check JS in the setting
Lower left gear - > Settings - > JS / TS strict null checks???
restart
4 project introduction
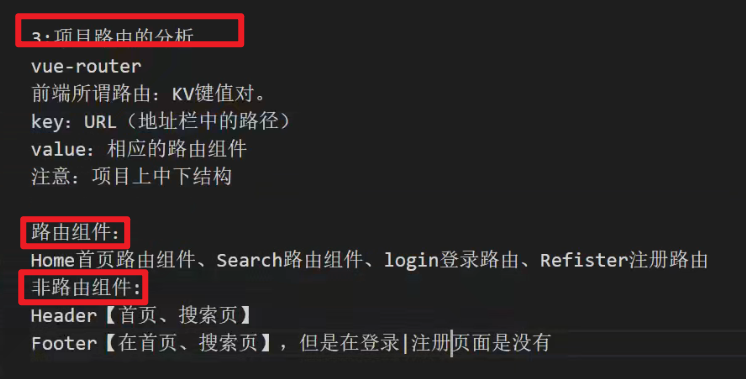
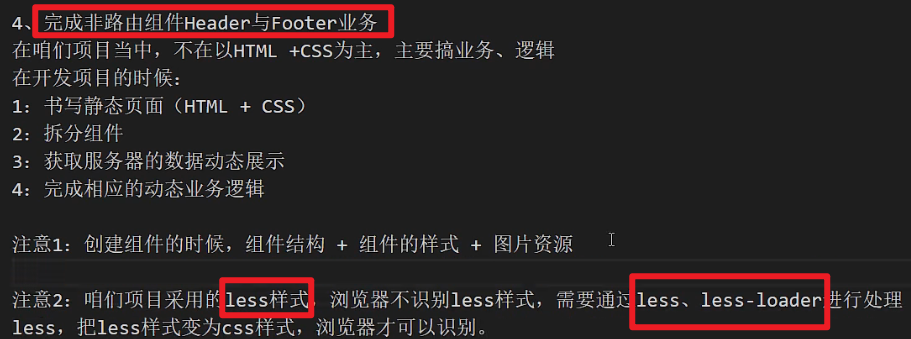
4.1 static page, style, clear default style, import
Copy the static resource page (HTML+CSS) into the project. The project uses less. You need to install less and less loader
cnpm install --save less less-loader@5
And add on style
<style lang="less" scoped></style>
reset.css files are generally placed under public and imported
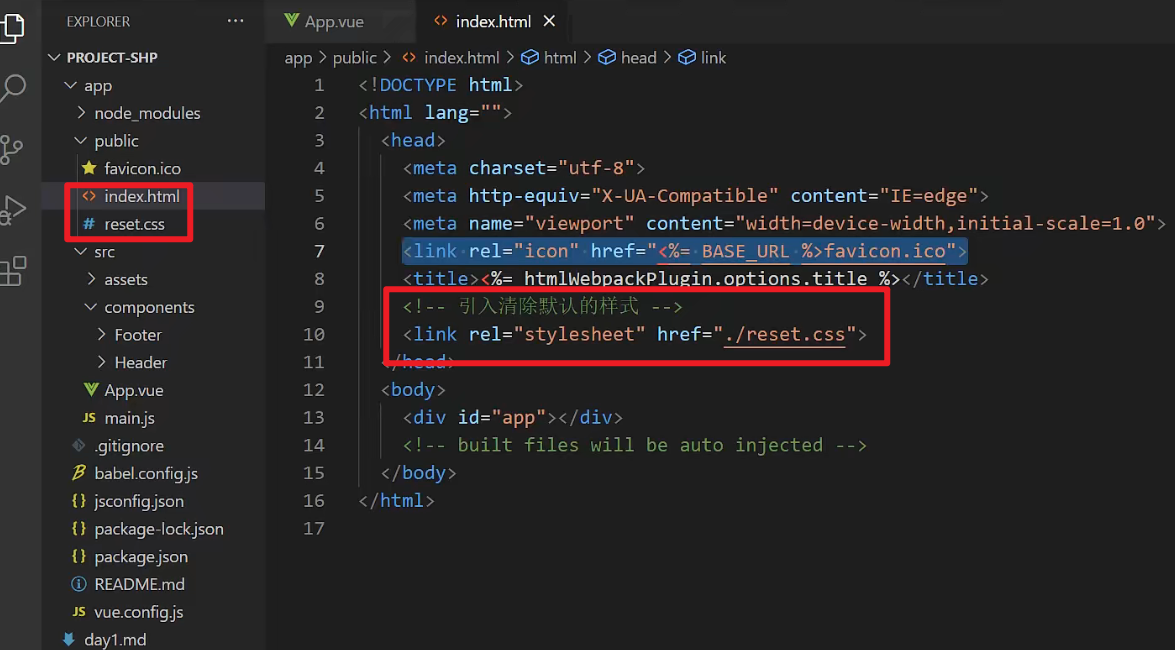
5. Construction of routing components
Installing Vue router
//Version 3.5.3 used in video cnpm install --save vue-router cnpm install --save vue-router@3.5.3 cnpm install --save vue-router@3
Write routing components in pages or assets
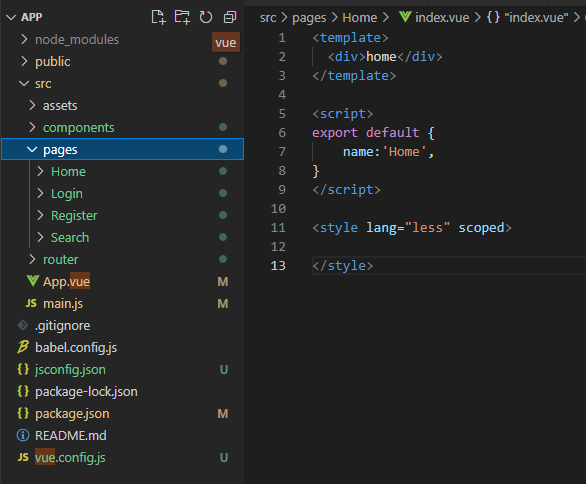
Configure routing in router
//Where to configure routing import Vue from "vue"; import VueRouter from 'vue-router'; //Using plug-ins Vue.use(VueRouter) //Introducing routing components import Home from '@/pages/Home' import Search from '@/pages/Search' import Login from '@/pages/Login' import Register from '@/pages/Register' //Configure routing export default new VueRouter({ //Configure routing routes:[ //redirect { path:'*', redirect:'/home', }, { path:'/home', component:Home, }, { path:'/search', component:Search, }, { path:'/login', component:Login, }, { path:'/register', component:Register, }, ] })
In the getting started file main JS register router
//Register the routing information. When the router is written here, the components have $route and $router belongs to
import Vue from 'vue' import App from './App.vue' // Vue.config.productionTip = false import router from '@/router' new Vue({ render: h => h(App), //Register routing: the following wording is kv, uniformly omit v, and cut router in lowercase //Register the routing information. When the router is written here, the components have $route and $router attributes router, }).$mount('#app')
On app Add routing exit router view to Vue
Note: routing components appear in router view, while non routing components appear in header
<template> <div id="app"> <Header></Header> <!-- Route exit --> <router-view></router-view> <Footer></Footer> </div> </template> <script> // Non component routing is introduced here and component routing is introduced in router import Header from './components/Header' import Footer from './components/Footer' export default { name: 'App', components: { Header, Footer, } } </script> <style scoped> </style>
6 routing component, routing jump summary
//Declarative navigation <router-link to="/login">Sign in</router-link> <router-link class="register" to="/register">Free registration</router-link> <router-link class="logo" to="/home"> <img src="./images/logo.png" alt="" /> </router-link> //Programming navigation <button class="sui-btn btn-xlarge btn-danger" type="button" @click="goSearch"> search </button> goSearch(){ this.$router.push('/home')},
7 v-if and v-show
Routing meta information: when configuring routing, meta
<Footer v-show="$route.meta.show"></Footer>
If v-show does not work here, you can set a div on the footer to perform v-show, and then it can be displayed normally
At the bottom of the home page | search, there is a Footer component, while there is no Footer component for login and registration
Display | hide the Footer component, and select v-show|v-if
Routing meta information meta
Interview question: what is the difference between v-show and v-if?
v-show: controlled by style display
v-if: operate through the upper and lower trees of elements
Interview question: what are the optimization methods when developing a project?
1:v-show|v-if
2: Load on demand