Catalog
- replace
- operator
1. Replacement
If the expression contains special characters, the Shell will be replaced. For example, using variables in double quotation marks is a kind of substitution, and escape characters are also a kind of substitution.
Examples:
#!/bin/bash a=10 echo -e "a is $a \n"
Output:
a is 10
Here - e denotes the substitution of escaped characters. If the - e option is not used, the output will be as it is: A is 10 n
You can use the - E option of the echo command to prohibit escape, and the default is not; you can use the - n option to prohibit insertion of newline characters.
Variable substitution
Variable substitution can change the value of a variable according to its state (whether it is empty, whether it is defined, etc.).
Variable substitutions available:
form | Explain |
---|---|
${var} | The original value of a variable |
${var:-word} | If the variable VaR is empty or unset, it returns word without changing the value of var. |
${var:=word | If the variable var is empty or unset, return word and set the value of var to word. |
${var:?message} | If the variable VaR is empty or unset, the message is sent to the standard error output, which can be used to detect whether the variable var can be assigned normally. If this replacement appears in the Shell script, the script will stop running. |
${var:+word} | If the variable VaR is defined, then word is returned without changing the value of var. |
Examples:
echo ${var:-"Variable undefined"} echo "1 - var The value of: ${var}" echo ${var:="var It's already worth it."} echo "2 - var The value of: ${var}" unset var echo ${var:+"var Variables are deleted"} echo "3 - var The value of: $var" var="Prefix" echo ${var:+"var Appointed Prefix"} echo "4 -var The value of: $var" unset var echo ${var:?"Print information"} echo "5 - var The value of: ${var}"
Output:
Variable undefined The value of 1 - var: var already has value Value of 2 - var: var already has value The value of 3 - var: var is assigned Prefix The value of 4-var: Prefix / test.sh: line 18: var: print information
2. Operator
Bash supports many operators, including arithmetic operators, relational operators, Boolean operators, string operators and file test operators.
Native bash does not support simple mathematical operations, but it can be implemented by other commands, such as awk and expr, which are most commonly used.
expr is an expression computing tool, which can be used to complete the evaluation of expressions.
Examples:
var=`expr 2 + 2` echo $var
Output result 4,
Two points to note:
It is wrong to have spaces between expressions and operators, such as 2 + 2, which must be written as 2 + 2, unlike most programming languages we are familiar with.
The complete expression is to be included. Note that this character is not the usual single quotation mark, under the Esc key.
Arithmetic operator
Let's start with an example of using arithmetic operators:
#!/bin/sh a=10 b=20 val=`expr $a + $b` echo "a + b : $val" val=`expr $a - $b` echo "a - b : $val" val=`expr $a \* $b` echo "a * b : $val" val=`expr $b / $a` echo "b / a : $val" val=`expr $b % $a` echo "b % a : $val" if [ $a == $b ] then echo "a is equal to b" fi if [ $a != $b ] then echo "a is not equal to b" fi
Output:
a + b : 30 a - b : -10 a * b : 200 b / a : 2 b % a : 0 a is not equal to b
In order to realize multiplication operation, a backslash must be added before the multiplication sign (*).
Relational operator
Relational operators only support numbers, not strings, unless the value of a string is a number.
Examples:
#!/bin/sh a=10 b=20 if [ $a -eq $b ] then echo "$a -eq $b : a,b Equal" else echo "$a -eq $b: a,b unequal" fi if [ $a -ne $b ] then echo "$a -ne $b: a,b unequal" else echo "$a -ne $b : a,b Equal" fi if [ $a -gt $b ] then echo "$a -gt $b: a greater than b" else echo "$a -gt $b: a Not more than b" fi if [ $a -lt $b ] then echo "$a -lt $b: a less than b" else echo "$a -lt $b: a Not less than b" fi if [ $a -ge $b ] then echo "$a -ge $b: a Greater than or equal to b" else echo "$a -ge $b: a less than b" fi if [ $a -le $b ] then echo "$a -le $b: a Less than or equal to b" else echo "$a -le $b: a greater than b" fi
Output:
10-eq 20: a, b 10-ne 20: a, b 10 -gt 20: a is not greater than b 10-lt 20: A is less than b 10-ge 20: A is less than b 10-le 20:a is less than or equal to b
operator | Explain | Give an example |
---|---|---|
-eq | Check if the two numbers are equal and return true equally. | [$a-eq $b] returns false. |
-ne | Check if the two numbers are equal and return true unequally. | [$a-ne $b] Returns true |
-gt | Check if the number on the left is greater than that on the right, and if so, return true. | [$a-gt $b] returns false. |
-lt | Check if the number on the left is less than the number on the right, and if so, return true. | [$a-lt $b] Returns true |
-ge | Check if the number on the left is large equal to the number on the right, and if so, return true. | [$a-ge $b] Returns false |
-le | Check if the number on the left is less than or equal to the number on the right, and if so, return true. | [$a-le $b] Returns true |
Boolean operator
Examples:
#!/bin/sh a=10 b=20 if [ $a != $b ] then echo "$a != $b : a is not equal to b" else echo "$a != $b: a is equal to b" fi if [ $a -lt 100 -a $b -gt 15 ] then echo "$a -lt 100 -a $b -gt 15 : returns true" else echo "$a -lt 100 -a $b -gt 15 : returns false" fi if [ $a -lt 100 -o $b -gt 100 ] then echo "$a -lt 100 -o $b -gt 100 : returns true" else echo "$a -lt 100 -o $b -gt 100 : returns false" fi
Output:
10 != 20 : a is not equal to b 10 -lt 100 -a 20 -gt 15 : returns true 10 -lt 100 -o 20 -gt 100 : returns true
operator | Explain | Give an example |
---|---|---|
! | If the expression is true, then false is returned, otherwise true is returned. | [! false] Returns true |
-o | Or an operation that returns true if an expression is true | [$a-lt 20-o $b-gt 100] Returns true |
-a | With operations, both expressions are true before returning true | [$a-gt $b] [$a-lt 20-a $b-gt 100] Returns false |
String operator
Examples:
#!/bin/sh a="hello world" b="Hello World" if [ "$a" = "$b" ] then echo "a,b Equal" else echo "a,b Inequality" fi if [ "$a" != "$b" ] then echo "a,b Inequality" else echo "a,b Equal" fi if [ -z "$a" ] then echo "Character creation a Length is 0." else echo "Character string a Length not 0" fi if [ -n "$a" ] then echo "Character string a Length not 0" else echo "Character string a Length is 0." fi if [ "$a" ] then echo "Character string a Not empty" else echo "Character string a Empty" fi
Output:
a, b are not equal a, b are not equal The length of string a is not zero The length of string a is not zero String a is not empty
Note: if [-z $a], it's best to write as follows: if [- Z'$a], the shell may warn: too many arguments
operator | Explain | Give an example |
---|---|---|
= | Check if two strings are equal and return true equally | [$a = $b] Returns false |
!= | Detecting whether two strings are equal and returning true unequally | [$a!= $b] Returns true |
-z | Detecting whether the length of the string is 0, returning true for 0 | [-z $a] returns false |
-n | Detecting whether the length of the string is 0, returning true if it is not 0 | [-n $a] Returns true |
str | Detect whether the string is empty and return true for none | [$a] Returns true |
File test operator
Examples:
#!/bin/sh file="/Users/chenzhichao/Desktop/1.png" if [ -e $file ] then echo "Document Existence" else echo "file does not exist" fi if [ -r $file ] then echo "Document readable" else echo "Document unreadable" fi if [ -w $file ] then echo "File Writable" else echo "Documents are not writable" fi if [ -x $file ] then echo "Document executable" else echo "Documents are not executable" fi if [ -d $file ] then echo "Files are directories" else echo "Files are not directories" fi
Output:
Document Existence Document readable File Writable Documents are not executable Files are not directories
File permissions can be modified by right-clicking on the file - > Introduction (Properties) - > Sharing and permissions.
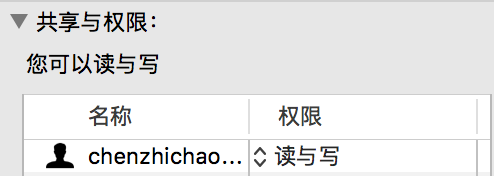
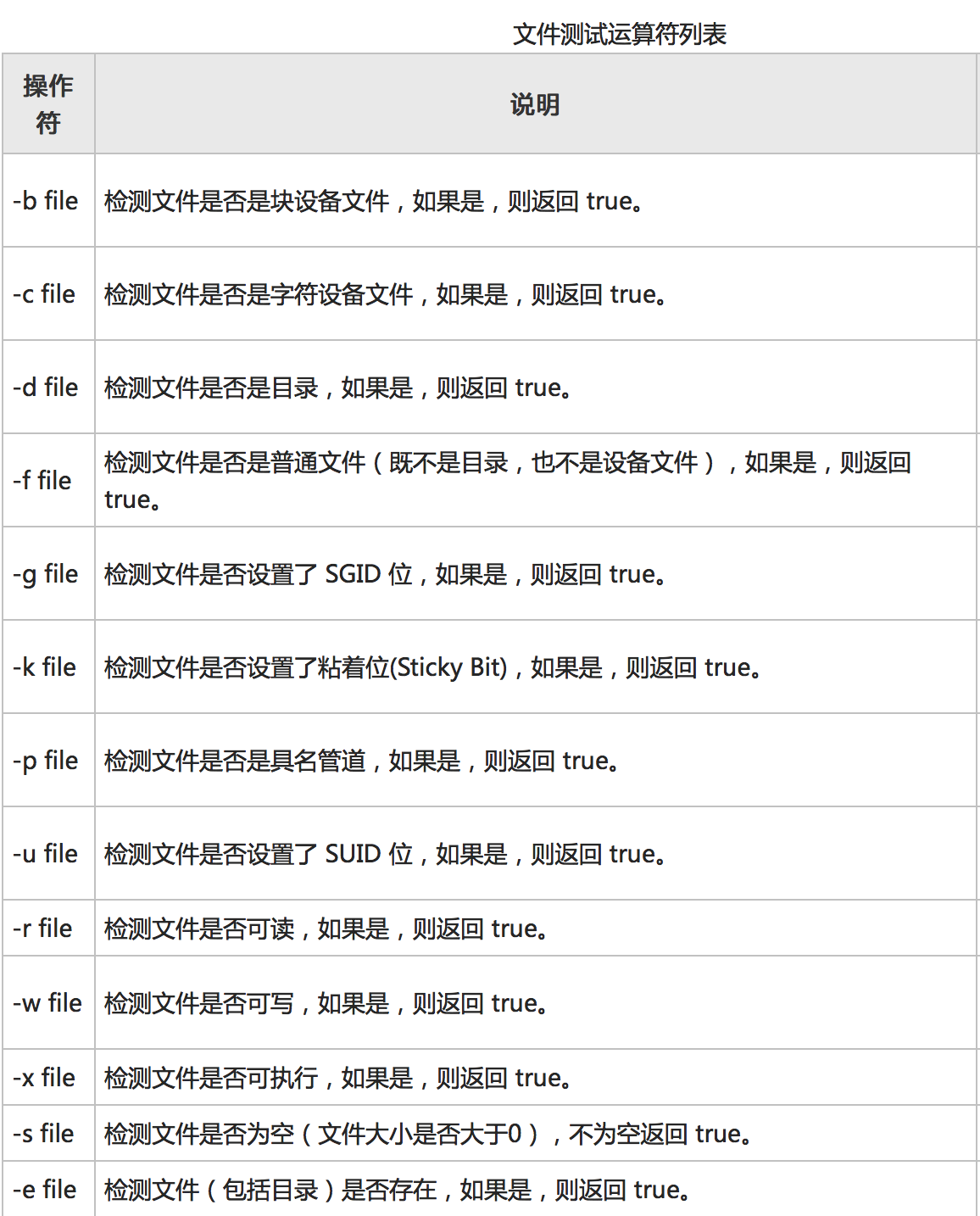