Schematic diagram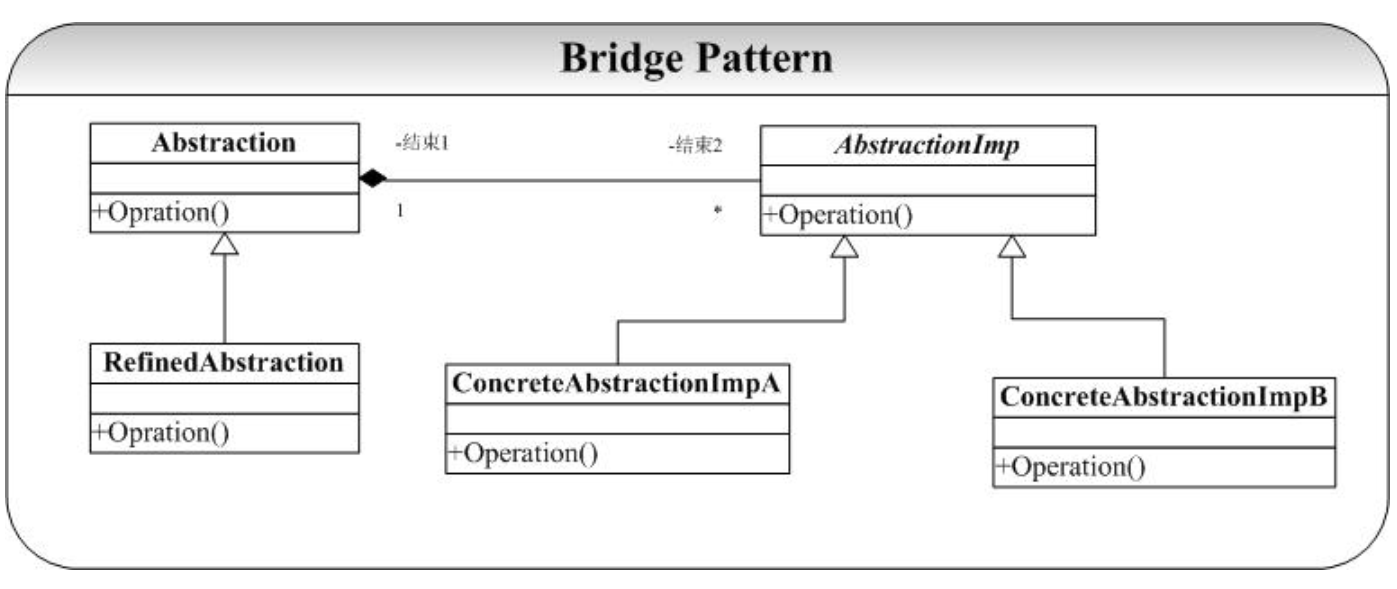
Bridge schema definition: divides abstraction and behavior into separate but dynamic combinations.
To summarize object-oriented, there are actually two sentences: one is Coupling, the other is Cohesion.
The goal of the object system is to maximize the Cohesion within the system modules and minimize the modules.
Coupling between blocks. However, this is also the most difficult part of the object-oriented design process, and you must know that
The development of OO system encountered such problems:
1) The customer gives you a need and uses a class to fulfill it (A);
2) Customer demand changes, there are two algorithms to implement the function, so the design changes, we pass an abstract base class,
Two specific classes are defined to implement two different algorithms (A1 and A2).
3) Customers tell us that for different operating systems, they abstract one level as an abstract base class
A0, which derives specific classes (A00 and A1, where A00 denotes the original class A) for each operating system, does not implement
Same as the customer requirements on the operating system, so we have a total of four classes.
4) Maybe the user's needs have changed, such as a new algorithm.....
5) We are stuck in a depression of changing demand, which has also led to the rapid expansion of human beings.
Bridge mode solves these kinds of problems.
In the structure diagram of Bridge mode, you can see that the system is divided into two relatively independent parts, with the abstract part on the left
On the right is the implementation part, which can be modified independently of each other: e.g. customer requirements in the above question
Change, when user needs need to derive a specific subclass from Abstraction, they don't need to inherit as above
Subclasses A1 and A2 need to be added when the method is implemented. In addition, due to the addition of algorithm in the above problem, only the right need to be changed.
Edge implementation (add a materialized subclass) without changing or adding a specific subclass to the right
Give an example:
For example, one cup of coffee has four subclass implementation classes: medium cup with milk, large cup with milk, medium cup without milk, and large cup without milk.
However, we have noticed that there are overlapping concepts in the four subclasses above, which can be considered from another perspective. These four classes are actually a combination of two roles: abstraction and behavior, which are abstracted as: medium and large cups; behavior: milk without milk (e.g. orange juice with apple juice).
Implementing four subclasses has a fixed binding relationship between abstraction and behavior, and if the behavior of adding grape juice is dynamically increased in the future, two additional classes must be added: medium and large. Obviously confusing and poorly scalable.
So we use Bridge mode to separate abstraction from behavior.
How?
Take the coffee mentioned above for example.We originally intended to design only one interface (abstract class). With Bridge mode, we need to separate abstraction from behavior. Milking and not milk are behaviors. We Abstract them into a specialized behavior interface
package design.bridge; /** * Bridge Schema Definition: Separate abstraction from behavior, independent but dynamic * For example, one cup of coffee has four subclass implementation classes: medium cup with milk, large cup with milk, medium cup without milk, and large cup without milk. * However, we have noticed that there are conceptual overlaps in the four subclasses above, which can be considered from a different perspective. * These four categories are actually a combination of two roles: abstraction and behavior, which are abstracted as: medium and large cups; behavior is: milk without milk (e.g. orange juice with apple juice) */ public class BridgePattern { public static void main(String[] args) { // Instance Behavior CoffeeImp milk = new MilkCoffeeImp(); CoffeeImp sugar = new SugarCoffeeImp(); // For a large glass of milk Coffee coffee = new SuperSizeCoffee(milk); System.out.println("Large glass with milk begin"); // Pour coffee coffee.pourCoffee(); System.out.println("Large glass with milk end"); System.out.println("Medium cup with sugar begin"); //For a medium cup with sugar coffee = new MediumCoffee(sugar); // Pour coffee coffee.pourCoffee(); System.out.println("Medium cup with sugar end"); } } // Coffee Behavior Abstract Class abstract class CoffeeImp { public abstract void pourCoffeeImp(); } // Coffee abstract class abstract class Coffee { protected CoffeeImp coffeeImp; public Coffee(CoffeeImp coffeeImp) { this.coffeeImp = coffeeImp; } public abstract void pourCoffee(); } // Realize the specific situation of coffee cups, such as medium cups, large cups //Medium Cup class MediumCoffee extends Coffee { public MediumCoffee(CoffeeImp coffeeImp) { super(coffeeImp); } public void pourCoffee() { System.out.println("Medium Cup"); coffeeImp.pourCoffeeImp(); } } //Big Cup class SuperSizeCoffee extends Coffee { public SuperSizeCoffee(CoffeeImp coffeeImp) { super(coffeeImp); } public void pourCoffee() { System.out.println("Big Cup"); coffeeImp.pourCoffeeImp(); } } //Coffee behavior, milk class MilkCoffeeImp extends CoffeeImp { MilkCoffeeImp() { } public void pourCoffeeImp() { System.out.println("Add milk"); } } //Coffee behavior is achieved, sugar added class SugarCoffeeImp extends CoffeeImp { SugarCoffeeImp() { } public void pourCoffeeImp() { System.out.println("Adding sugar"); } }
Bridge is one of the more complex and difficult patterns in design patterns and is often used in OO development and design
One of the patterns. The benefits of decoupling abstraction and implementation thoroughly using a combination (delegation) approach are abstraction and Implementation
They can change independently and the coupling of the system is reduced very well.
GoF, when explaining the Bridge pattern, intends to state that the Bridge pattern "divides the abstract part into its implementation part"
It's simple, but complex, even Bruce Eckel in his blockbuster
In Thinking in Patterns, "Bridge mode is the Poorly described model described by GoF
"Style". I think this is also true. The reason is how to understand "Realization" in GoF's sentence: "Realization"
Especially when it comes to abstraction, our "default" understanding is that "implementation" is the implementation of a specific subclass of "abstraction". But what GoF means here is not the implementation of a virtual function (interface) in an abstract base class by a specific subclass of an abstract base class, but the combination of "implementation" and inheritance.Meaning refers to how to fulfill the user's needs, and also refers to the combination (delegation)By way of implementation, the implementation here does not refer to inheriting the base class or implementing the base class interface, but to fulfilling the user's needs through a combination of objects. By understanding this, you understand the Bridge pattern, and by understanding the Bridge pattern, your design will become more Elegant.
In fact, the fundamental difference between the Bridge mode and the solution using the problematic mode above is whether a functional requirement is achieved through inheritance or combination. So there is a principle in object-oriented analysis and design: Favor Composition Over Inheritance. That's why.
Example of JDBC
There are friends on the network who have some disagreements about whether or not JDBC uses Bridge mode. It is still clear that JDBC does use Bridge mode.
JDBC is actually just a set of specifications defined by Sun. Most of the real content under java.sql is interfaces. Other database vendors can provide their own JDBC implementations to support their own databases. As an application developer, you can completely rely on Sun's JDBC interfaces to complete all application development without caring which database is the data.Library vendors simply need to provide their own implementation of the database, without considering what kind of application their own database will be used for. This is also the separation of abstraction (database application) and implementation (vendor's JDBC implementation).
When it comes to code, consider that when we write a database application in JDBC, there is only one line of code associated with the specific database.
What this line of code does is it creates an instance of itself (com.mysql.jdbc.Driver) and registers it with java.sql.DriverManager.
DriverManager.getConnection() is then called to find the appropriate entry from the registered driver and return.