Recently, I wanted to write something interesting, so I finished this simple, not very beautiful gadget.
First you have to make sure that your computer has node s, and then you can do things step by step.~~
1. Create a folder
2. Clear the current folder address bar and enter cmd.exe in the folder address bar
3. We need to download a little something and type it on the command line.
- npm install express returns and waits for a while
- npm install express-session returns and waits for a while
- npm install ejs return and wait for a while
- npm install socket.io will wait for a while to get it done!!!
4. After installation, there will be a node_modules folder under the folder you built. Next, we need to create two folders, one folder (public) to store static resources, and add jquery files, and one folder (views) to store static template ejs files.
5. Next we need to create an entry file (app.js) under the folder you created.
6.app.js must be written
var express=require('express'); var app=express(); //Load express web server var http=require('http').Server(app); //Load websocket server - > http://localhost:3000/socket.io/socket.io.js var io=require('socket.io')(http); //Listening Port 3000 http.listen(3000);
7. (1) Loading routing, processing routing, configuring ejs templates, and handling static resource managers
- app.get app.post
- app.set("view engine","ejs");
- app.use(express.static('./public'));
(2) Establish two files, index.ejs and chat.ejs. We need to introduce them under chat.ejs.
<script src="/socket.io/socket.io.js"></script>
<script src="/jquery-1.12.4.js"></script>
If you don't have it locally, you can play like this.~~
Can I quote online?
<script src="http://code.jquery.com/jquery-latest.js"></script>
8.app.get routing action ='check'
- Three Conditions for Judging Landing
1-cannot be empty
2-Unable to rename
3 - Register and jump the chat page
9. We landed, to chat, we have to tell others what we call!!! So here we need to deal with session s and show them on the chat page.
10. So we have to start building websocket Communications
- client
(1)Load <script src="/socket.io/socket.io.js"></script> <script src="/jquery-1.12.4.js"></script> <script> var socket=io(); socke.emit('Event name','data'); Data can be objects (landers and content) </script>
- server
(2) io.on('connection',function(socket){ socket.on('Event name',function(data){ io.emit('New event name',data); }); });
- client
(3) socket.on('New event name',function(msg){ dom Operating handle msg Data rendering to us dom structure })
These are the basic ideas and steps.
Now that everything is ready, start coding.
app.js
var express=require('express'); var app=express(); var http=require('http').Server(app); var io=require('socket.io')(http); var session=require('express-session'); app.use(session({ secret: 'keyboard cat', resave: false, saveUninitialized: true, //cookie: { secure: true } })); //template engine app.set("view engine","ejs"); //Static Services app.use(express.static('./public')); var alluser=[]; //middleware //Display Home Page app.get('/',function(req,res,next){ res.render('index'); }); //Confirm login and check if this person has a username nickname that cannot be duplicated app.get('/check',function(req,res,next){ var yonghuming=req.query.yonghuming; if(!yonghuming){ res.send('User name must be filled in'); return; } if(alluser.indexOf(yonghuming) != -1){ res.send('User name renaming'); return; } alluser.push(yonghuming); console.log(alluser); req.session.yonghuming=yonghuming; res.redirect("/chat"); }); //chat room app.get('/chat',function(req,res,next){ //console.log(req.session.yonghuming); //console.log(123); if(!req.session.yonghuming){ res.redirect("/"); return; } res.render('chat',{ 'yonghuming':req.session.yonghuming }); }); io.on('connection',function(socket){ socket.on('liaotian',function(msg){ console.log(msg); //io.emit('liaotian',msg); //console.log(io); io.emit('liaotian',msg); }); }) //Listening port http.listen(3000); console.log('server start port 3000');
Index. EJS (under views folder)
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> <style> div{ width:700px; height:30px; padding:40px; border:1px solid #000; margin:0 auto; } #yonghuming{ font-size:30px; } </style> </head> <body> <div> <form action="/check" method="get"> //Enter nicknames: <input type="text" id="yonghuming" name="yonghuming"> <input type="submit" value="Enter the chat room"> </form> </div> </body> </html>
chat.ejs
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"/> <title>Document</title> <style> .caozuo{ position:fixed; bottom:0; left:0; height:100px; background-color:#eee; width:100%; } .caozuo input{ font-size:30px; } .caozuo input[type=text]{ width:100%; } </style> </head> <body> <h1>Edison chat room<span id="yonghu"> Welcome:<%=yonghuming %></span></h1> <div> <ul class="liebiao"></ul> </div> <div class="caozuo"> <input type="text" id="neirong"/> <input type="button" id="fayan" value="speak"/> </div> <script src="/socket.io/socket.io.js"></script> <script src="/jquery-1.12.4.min.js"></script> <script> var socket=io(); $('#neirong').keydown(function(e){ if(e.keyCode==13){ //Return to send message socket.emit('liaotian',{ 'neirong':$('#neirong').val(), 'ren':$('#yonghu').html(), }); $(this).val(''); } }); /* $("#fayan").click(function(){ //Click the Speech button to send the message socket.emit('liaotian',{ 'neirong':$('#neirong').val(), 'ren':$('#yonghu').html(), }); });*/ socket.on('liaotian',function(msg){ $(".liebiao").prepend("<li><b>"+msg.ren+": </b>"+msg.neirong+"</li>"); }); </script> </body> </html>
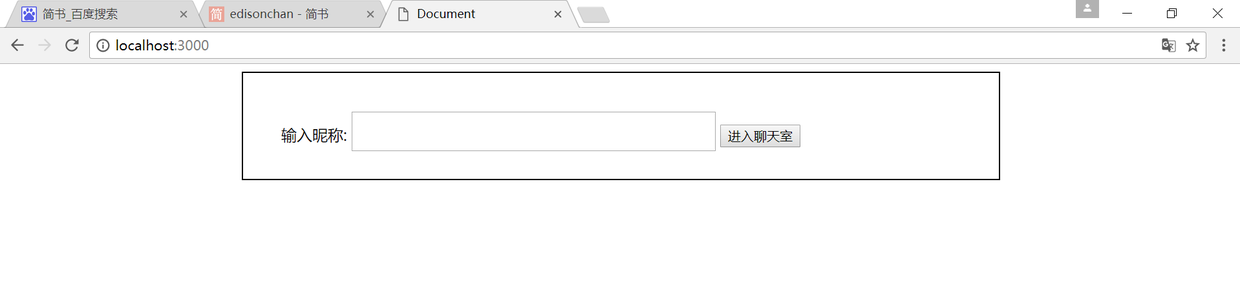
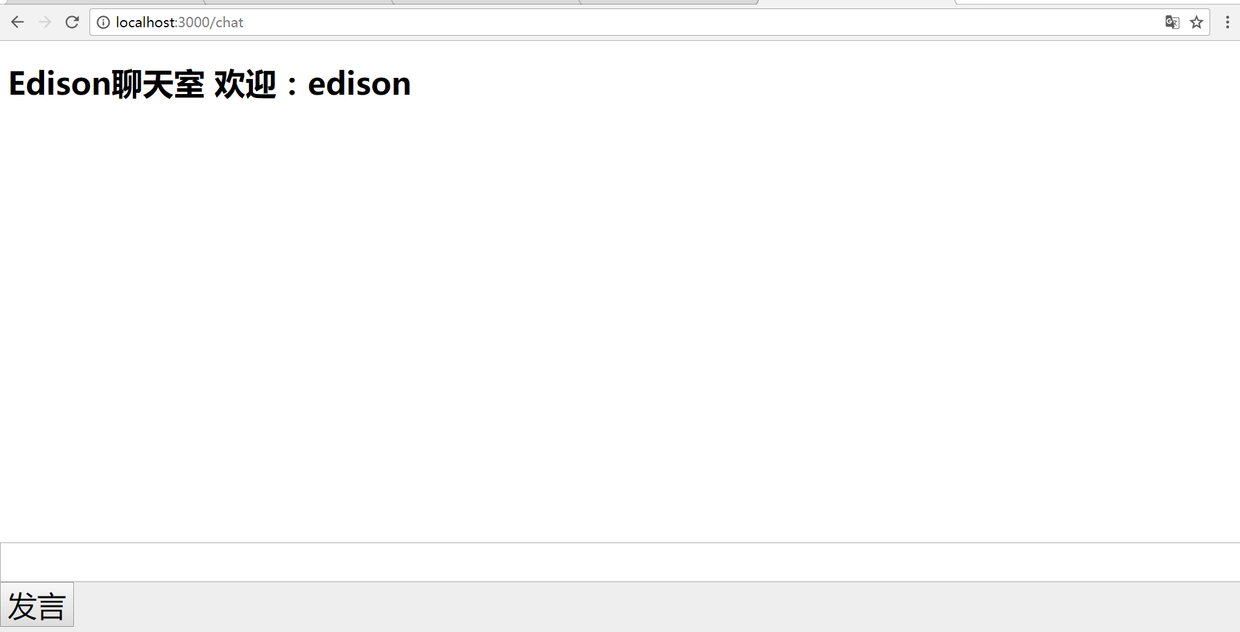
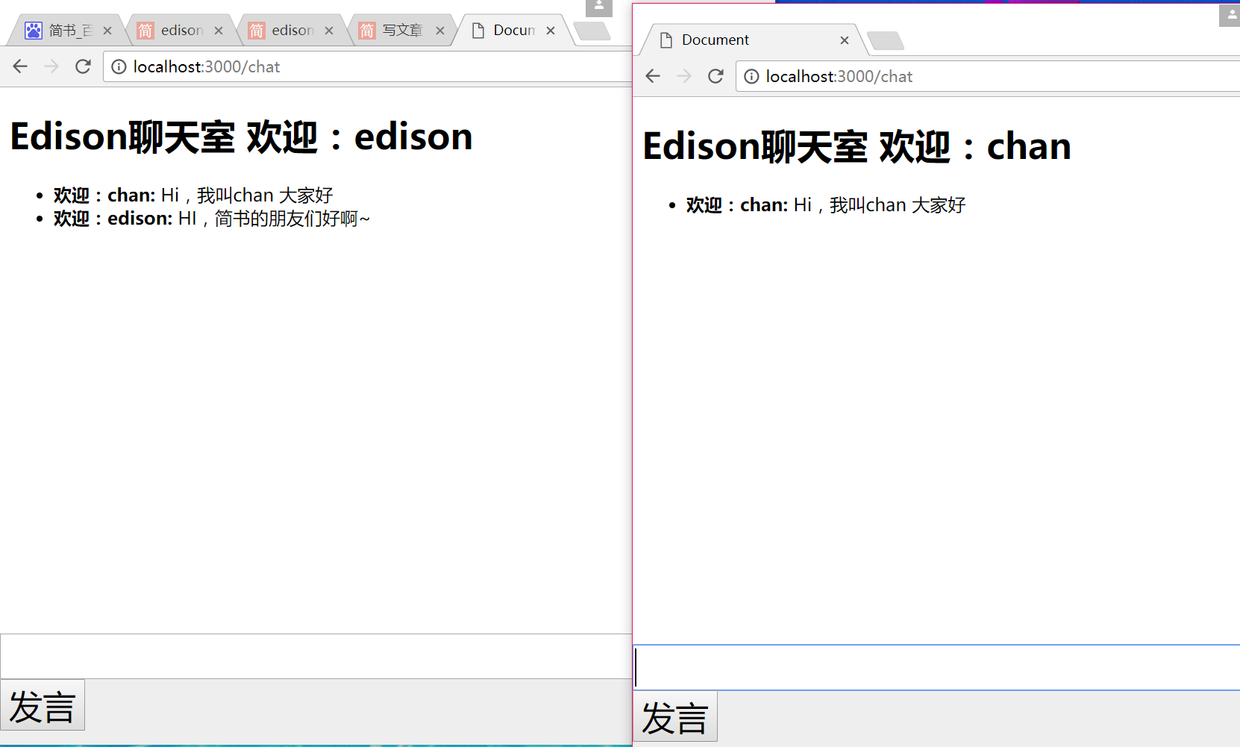