First question
unexpected check-in questions, traversal.
#FA Yi ans = 0 for i in range(1,2021): for j in str(i): if j == "2": ans += 1 print(ans) #Method II s=0 for i in range(1, 2021): s+=str(i).count('2') #The count function can only be used with strings, so you need to convert numbers to strings print(s)
Second question
look carefully, the problem is divided into two steps. The first step is to read the file, and the second step is to traverse according to the meaning of the problem. There are not many technologies to speak of, but we should pay attention to the boundary conditions, because if the boundary is sometimes obtained and sometimes not obtained, our code will find less than one or more numbers, which may eventually lead to errors (there are generally problems in this kind of problem, because its technology is not difficult, that is, whether you are careful or not).
int_file = open(r"./int.txt") #read file matrix = [] while True: data = int_file.readline() # Take out one row of data at a time, but it really wastes a lot of time... if data[-1] != '\n': # The last element of the last row of data is the number [0, 2] matrix.append(list(data)) break else: data = data[:-1] a = list(data) matrix.append(a) int_file.close() row = len(matrix) # that 's ok col = len(matrix[0]) # column count = 0 for i in range(row): for j in range(col): if matrix[i][j] == '2': # The retrieved data types are all strings, so the character '2' is used if j + 3 < col: if matrix[i][j] + matrix[i][j + 1] + matrix[i][j + 2] + matrix[i][j + 3] == "2020": count += 1 if i + 3 < row: if matrix[i][j] + matrix[i + 1][j] + matrix[i + 2][j] + matrix[i + 3][j] == "2020": count += 1 if i + 3 < row and j + 3 < col: if matrix[i][j] + matrix[i + 1][j + 1] + matrix[i + 2][j + 2] + matrix[i + 3][j + 3] == "2020": count += 1 print(count)
Question 3
obviously, this question examines our understanding of the built-in package datetime in Python. As long as you master datetime, this question can be written quickly (in fact, you don't need to be familiar with it, just understand a function). Of course, it's OK to manually write the code, but it's troublesome.
import datetime start = datetime.date(2000,1,1) end = datetime.date(2020,10,1) days = datetime.timedelta(days=1) # The timedelta of a day available for calculation represents the time difference between two times res = 0 while start <= end: if start.day == 1 or start.weekday() == 0: res += 2 else: res += 1 start += days print(res)
Question 4
a typical big flicker problem looks complex, but it can be done without code at all.
line 20, column 20. Obviously, on the diagonal, let's first observe the numbers on the diagonal, which are 1, 5, 13... Respectively. Of course, we can continue to write. The sequence is 1, 5, 13, 25, 41... When writing this step, the law comes out naturally. The difference between their adjacent numbers is 4, 8, 12, 16... That is, an equal difference sequence with a tolerance of 4. The recurrence relationship is
a
n
−
a
n
−
1
=
4
(
n
−
1
)
a_n-a_{n-1} = 4(n-1)
An − an − 1 = 4(n − 1) and
a
1
=
1
a_1=1
a1=1. Finally
a
n
=
2
n
(
n
−
1
)
+
1
a_n = 2n(n-1)+1
an=2n(n−1)+1. Then put
n
=
20
n=20
n=20 can be substituted.
of course, if you have to write code, it's not impossible. You just need to create one
2
×
20
−
1
=
39
2\times20-1=39
two × 20 − 1 = 39
39
×
39
39\times39
thirty-nine × 39 matrix simulation array can be generated. But this method is obviously not concise enough (that is, lazy and don't want to write).
Question 5
Obviously, this question examines our familiarity with bubble ranking. We all understand that the time complexity of bubble sorting is
O
(
n
2
)
O(n^2)
O(n2), but it is generally limited to this. But when it comes to the number of exchanges, we need to know that the number of exchanges in bubble sorting is
f
(
n
)
=
n
(
n
−
1
)
/
2
f(n)=n(n-1)/2
f(n)=n(n − 1) / 2 (in the case of complete reverse order), then it is obvious that the case where the question requires the shortest string must be in the case of complete reverse order. At the same time, it also requires the smallest dictionary order. It is obvious that it is arranged from A. how many letters do you need?
first of all, its
f
(
n
)
f(n)
f(n) must be greater than or equal to 100. We substitute it into the formula and find that the nearest to 100 is
n
=
15
n=15
n=15, corresponding
f
(
n
)
=
105
)
f(n)=105)
f(n)=105), that is, if my arrangement is
o
n
m
l
k
j
i
h
g
f
e
e
d
c
b
a
onmlkjihgfeedcba
Onmlkjihgfedcba, we need to exchange 105 times when using bubble sorting, but our requirement is 100 times. If this is only the case, we have a variety of exchange methods, and we can exchange any letter forward 5 times.
at this time, the dictionary order will play a role. The so-called minimum dictionary order is the minimum alphabet order. Then we should make the letters in front of the comparative alphabet appear at the front as much as possible. However, since the subject needs to be in reverse order, we should make the initial letters as front as possible, 105 times, then
o
n
m
l
k
j
i
h
g
f
e
e
d
c
b
a
onmlkjihgfeedcba
The sixth letter of onmlkjihgfedcba is exchanged five times in the first place.
j
o
n
m
l
k
i
h
g
f
e
e
d
c
b
a
jonmlkihgfeedcba
Jonmlkihgfedcba is our answer.
Question 6
it's more like a sign in question than the first question.
n = eval(input()) num1, num2 = 0, 0 for i in range(n): score = eval(input()) if score >= 60: num1 += 1 if score >= 85: num2 += 1 precent_qualified = format(num1 / n * 100, '.0f') precent_excellent = format(num2 / n * 100, '.0f') print(str(precent_qualified) + "%") print(str(precent_excellent) + "%")
Question 7
since the word length is no more than 1000, this is also a check-in question, which is the most complex but traversal
26
×
1000
26\times1000
twenty-six × 1000 times, completely able to
1
s
1s
Complete the execution within 1s.
lis, lit = [], [0] * 26 s = input() for i in range(26): lis.append(chr(97+i)) for i in range(len(lis)): for k in s: if k == lis[i]: lit[i] += 1 for i in range(len(lit)): if lit[i] == max(lit): p = lis[i] print(p) print(max(lit))
Question 8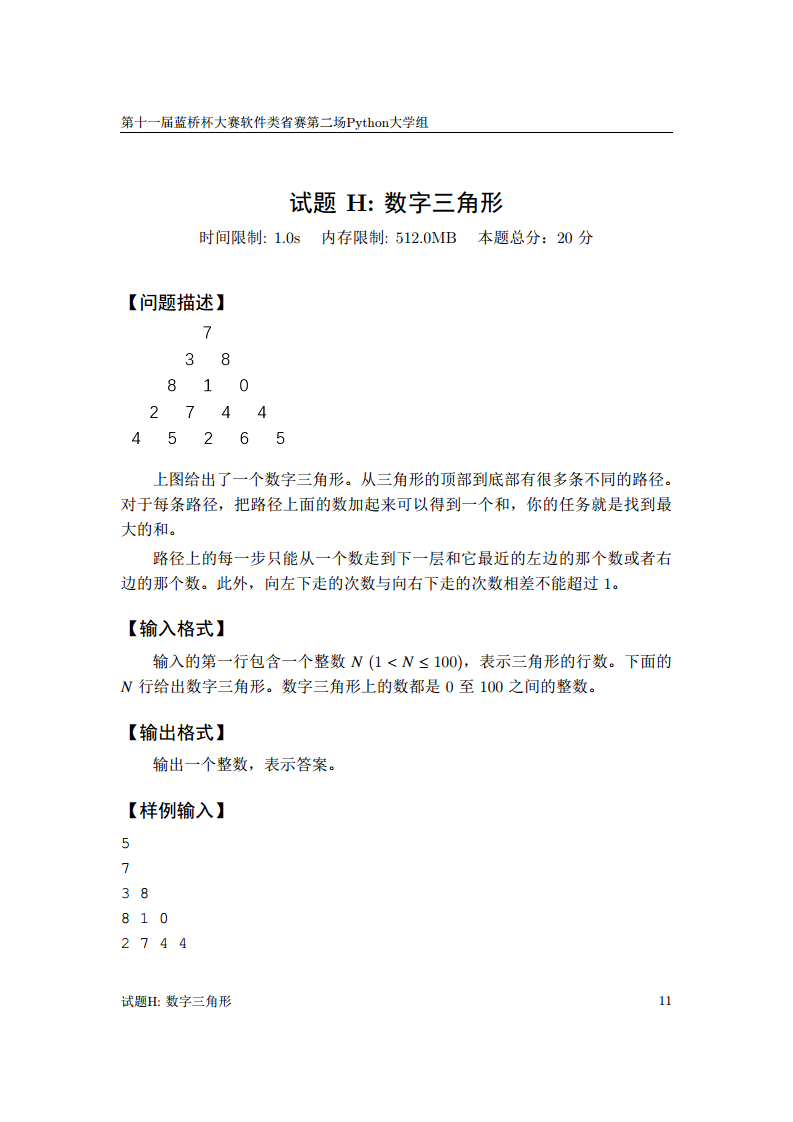
students who have studied algorithms probably know that digital triangle is a particularly classic introductory problem of dynamic programming. There are a lot of explanations on the Internet. Among them, I personally recommend the program design and algorithm taught by Guo Wei of Peking University in the University MOOC (if you are interested, you can go and see it yourself, but there is no link here). The problem of digital triangle is explained in detail.
let's take this problem as a digital triangle first. We said in the previous article that the most important part of dynamic programming is to find the recursive relationship and the starting conditions. That is, initialize the array and finally find the traversal order.
the starting condition is obvious, that is, the vertex number of the triangle. Initialize array in this problem, we can correct it in the input array, so use the original array. Then find the recursive formula. Dynamic programming makes us need to optimize every step as much as possible, so we can write the recursive formula
d
p
[
i
]
[
j
]
+
=
m
a
x
(
d
p
[
i
−
1
]
[
j
]
,
d
p
[
i
−
1
]
[
j
−
1
]
)
dp[i][j]+=max(dp[i-1][j],dp[i-1][j-1])
dp[i][j]+=max(dp[i − 1][j],dp[i − 1][j − 1]) (most of them are like this. I haven't found the counterexample, but an error will be reported when submitting it on the official website of the blue Bridge Cup. After reading other people's codes, it should be that the boundary conditions are not handled, that is
i
=
j
i=j
i=j, and
j
=
0
j=0
If j=0, there is no problem adding judgment).
after processing the general digital triangle, let's look at the limiting conditions. The difference between the left and right times cannot exceed 1. In fact, you can know by simply drawing. The result of this painting will eventually fall in the middle of the last line. If it is an odd line, it is the middle one. For an even line, you can find the two larger numbers in the middle
n = eval(input()) lst = [] for i in range(n): m = list(map(int,input().split())) lst.append(m) list_1 = [[0 for _ in range(n)] for _ in range(n)] list_1[0][0] = lst[0][0] for k in range(1,n): for t in range(k+1): if t == 0: list_1[k][t] = list_1[k-1][t] + lst[k][t] elif t == k: list_1[k][t] = list_1[k-1][t-1] + lst[k][t] else: list_1[k][t] = max(list_1[k-1][t-1],list_1[k-1][t]) + lst[k][t] if n % 2 == 0: print(max(list_1[n-1][n//2-1],list_1[n-1][n//2])) else: print(list_1[n-1][n//2])
Question 9
to be honest, I planned to do it as a mathematical problem at the beginning. However, I wrote a pile of derivation. I found that it was difficult for me to give formulas for each case, so I couldn't calculate, so I had to be honest and honest.
of course, the reality is that I didn't roll it out. The reason will be explained later. I looked elsewhere An article , let's see, the code is also the blogger's.
N = int(input()) #Enter N setpointline = set() sumvalue =1 for i in range(N): seta = set() #Traverse once each time linex,liney = map(int,input().split()) for x,y in setpointline: if(x==linex and y==liney): #If coincident sumvalue-=1 # seta=set() #Remove the elements of the original collection break elif(x==linex): #If the slopes are the same, there are no intersections continue else: nodex = (y-liney)/(linex-x) #intersection nodey = x*nodex + y #Substitute into any equation seta.add((nodex,nodey)) #Enter set sumvalue+=len(seta)+1 #That is to join if ((linex, liney) not in setpointline): setpointline.add((linex, liney)) # Is there a heavy edge print(sumvalue) #Number of output sub planes
Question 10
slightly
Write it at the back
I used to write articles at will. This year, on New Year's day, I wrote the problem solution of the 11th Blue Bridge Cup for my students. I realized that I still have many shortcomings, so I hope to stick to one blog post every week this year, but I'm lazy. Until the last few hours of the last day of this week, I only looked at the simple questions in front in a hurry. There was no time to look at the next two questions, so the code of question 9 didn't come out, and question 10 didn't even look.
so set up a small one here
f
l
a
g
flag
flag, I hope I can get rid of my procrastination in the new year. Don't put everything to the end. It's really bad.
then, writing the problem solution can really help us find many deficiencies. For example, for problem 8, I think I understand the digital triangle well and the dynamic programming is OK. However, when I re wrote the code today, I submitted it three times in the Blue Bridge Cup system, 10, 70 and 20 respectively. Finally, I got full marks after reading other people's code. This also reminds me, Blogging and problem solving help us understand our mastery of knowledge. Only when you write it can you know how your foundation is.
finally, I hope you can make progress together. In 2022, I hope you can supervise me every Monday (maybe algorithms, data structures and some problem solutions)