Solutions to Browser Compatibility Problems
Popularity: Browser compatibility problems are often caused by inconsistent definitions of some standards by individual browsers (yes, that particular browser). As the saying goes: Without IE, there will be no harm.
Tips: The content is self-summarized, there will inevitably be errors or bug s, you are welcome to correct and supplement, this post will continue to update.
Normalize.css
Different browsers have different default styles, which can be smoothed out with Normalize.css. Of course, you can also customize reset.css, which belongs to your business.
<link href="https://cdn.bootcss.com/normalize/7.0.0/normalize.min.css" rel="stylesheet">
Simple rough method
* { margin: 0; padding: 0; }
html5shiv.js
Solve the problem that the browsers below ie9 do not recognize the new tags of HTML 5.
<!--[if lt IE 9]>
<script type="text/javascript" src="https://cdn.bootcss.com/html5shiv/3.7.3/html5shiv.min.js"></script>
<![endif]-->
respond.js
Solve the problem that browsers below ie9 do not support CSS3 Media Query.
<script src="https://cdn.bootcss.com/respond.js/1.4.2/respond.min.js"></script>
picturefill.js
Solve the problem that browsers such as IE 9 10 11 do not support < picture > tags
<script src="https://cdn.bootcss.com/picturefill/3.0.3/picturefill.min.js"></script>
IE Conditional Notes
IE conditional annotations are only for IE browsers and are not valid for other browsers
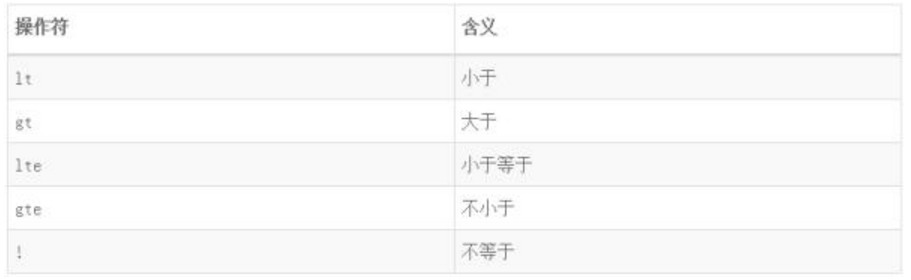
IE Attribute Filter (the more commonly used hack method)
For different IE browsers, different characters can be used to style specific versions of IE browsers.
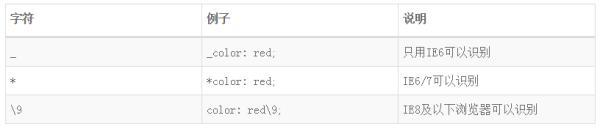
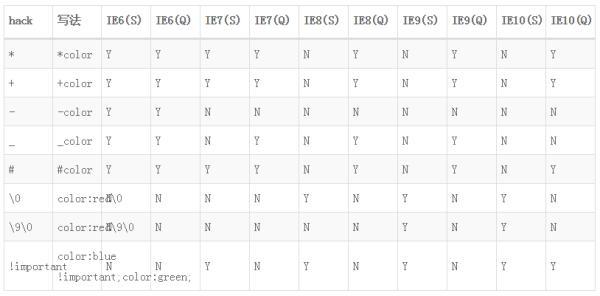
Browser CSS Compatible Prefix
-o-transform:rotate(7deg); // Opera -ms-transform:rotate(7deg); // IE -moz-transform:rotate(7deg); // Firefox -webkit-transform:rotate(7deg); // Chrome transform:rotate(7deg); // Unified Identification Statement
The order of several CSS states of a tag
Many new people write a label style, will wonder why the style of writing is ineffective, or click on hyperlinks, hover, active style is ineffective, in fact, the style of writing is overwritten.
The correct order of a tags should be: == love hate==
- link: Normal State
- Visited: After being visited
- hover: When the mouse is on the link
- active: When the link is pressed
Perfect solution to Placeholder
<input type="text" value="Name *" onFocus="this.value = '';" onBlur="if (this.value == '') {this.value = 'Name *';}">
Best Practices for Clearing Floating
.fl { float: left; }
.fr { float: right; }
.clearfix:after { display: block; clear: both; content: ""; visibility: hidden; height: 0; }
.clearfix { zoom: 1; }
BFC Solves Edge Overlapping Problem
When margin margin margin is set for adjacent elements, margin will take the maximum value and discard the small value. In order not to overlap the margins, we can add a parent element to the child element and set the parent element to BFC: overflow: hidden.
<div class="box" id="box">
<p>Lorem ipsum dolor sit.</p>
<div style="overflow: hidden;">
<p>Lorem ipsum dolor sit.</p>
</div>
<p>Lorem ipsum dolor sit.</p>
</div>
The Problem of IE6 Double Margin
Setting float in ie6 and margin at the same time will cause double margin problem
display: inline;
Solve the problem that browsers below IE9 cannot use opacity
opacity: 0.5; filter: alpha(opacity = 50); filter: progid:DXImageTransform.Microsoft.Alpha(style = 0, opacity = 50);
Solve the problem that IE6 does not support fixed absolute positioning and that elements positioned absolutely under IE6 will flicker when scrolling.
/* IE6 hack */
*html, *html body {
background-image: url(about:blank);
background-attachment: fixed;
}
*html #menu {
position: absolute;
top: expression(((e=document.documentElement.scrollTop) ? e : document.body.scrollTop) + 100 + 'px');
}
IE6 Background Scintillation
Question: Links and buttons are backed by CSS sprites, and background images will flicker under ie6. The reason is that IE6 does not cache the background image and reloads it every time the hover is triggered.
Solution: You can use JavaScript to set ie6 to cache these pictures:
document.execCommand("BackgroundImageCache", false, true);
Solve the problem of misalignment of lists and dates under IE6
The date < span > tag should be placed before the title < a > tag.
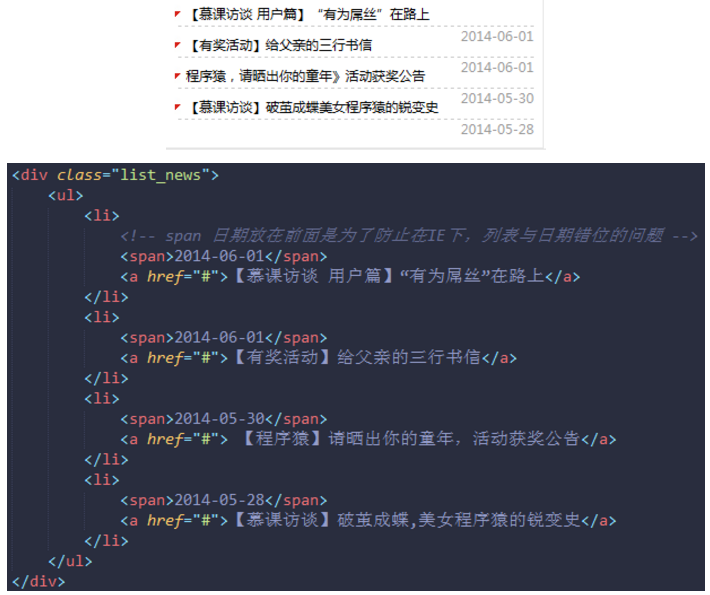
Solve the problem that IE6 does not support min-height attribute
min-height: 350px;
_height: 350px;
Let IE7 IE8 support CSS3 background-size attributes
Because background-size is a new attribute of CSS3, the low version of IE naturally does not support it, but foreigners have written an htc file called "background-size". background-size polyfill With this file, IE7 and IE8 can support background-size attributes. The principle is to create an img element to insert into the container and recalculate the width, height, left, top equivalents to simulate the effect of background-size.
html {
height: 100%;
}
body {
height: 100%;
margin: 0;
padding: 0;
background-image: url('img/37.png');
background-repeat: no-repeat;
background-size: cover;
-ms-behavior: url('css/backgroundsize.min.htc');
behavior: url('css/backgroundsize.min.htc');
}
Failure of IE6-7 line-height
Question: line-height doesn't work when img and text are put together in ie
Solution: All set to float
width:100%
width:100% is very convenient to use in ie. It searches the width value upward layer by layer, ignoring the influence of floating layer.
Search under Firefox until the end of the floating layer, so you can only add width:100% to all the floating layers in the middle, tired.
opera is a good idea. Follow ie
cursor:hand
Display hand cursor: hand, ie6/7/8, opera support, but safari, ff do not support
cursor: pointer;
The problem of automatic Line-Changing in td
Question: table width is fixed, td Line-Changing automatically
Solution: Set Table to table-layout: fixed, td to word-wrap: break-word
Let the layer be displayed on FLASH
If you want the content of the layer to be displayed on flash, you can set FLASH transparently.
1,<param name=" wmode " value="transparent" />
2,<param name="wmode" value="opaque"/>
Keyboard Event keyCode Compatibility Writing
var inp = document.getElementById('inp')
var result = document.getElementById('result')
function getKeyCode(e) {
e = e ? e : (window.event ? window.event : "")
return e.keyCode ? e.keyCode : e.which
}
inp.onkeypress = function(e) {
result.innerHTML = getKeyCode(e)
}
Compatible Writing Method for Finding Window Size
// Visual area size of browser window (excluding toolbar and scrollbar contours)
// 1600 * 525
var client_w = document.documentElement.clientWidth || document.body.clientWidth;
var client_h = document.documentElement.clientHeight || document.body.clientHeight;
// Actual width and height of web content (including toolbar and scrollbar contours)
// 1600 * 8
var scroll_w = document.documentElement.scrollWidth || document.body.scrollWidth;
var scroll_h = document.documentElement.scrollHeight || document.body.scrollHeight;
// The actual width and height of the page content (excluding toolbars and scrollbar contours)
// 1600 * 8
var offset_w = document.documentElement.offsetWidth || document.body.offsetWidth;
var offset_h = document.documentElement.offsetHeight || document.body.offsetHeight;
// Rolling Height
var scroll_Top = document.documentElement.scrollTop||document.body.scrollTop;
Compatible Writing of DOM Event Handlers (Capability Detection)
var eventshiv = {
// event compatible
getEvent: function(event) {
return event ? event : window.event;
},
// <span class="hljs-build_in">type</span> compatibility
getType: <span class="hljs-keyword">function</span>(event) {
<span class="hljs-built_in">return</span> event.type;
},
// target compatible
getTarget: <span class="hljs-keyword">function</span>(event) {
<span class="hljs-built_in">return</span> event.target ? event.target : event.srcelem;
},
// Adding event handles
addHandler: <span class="hljs-keyword">function</span>(elem, <span class="hljs-built_in">type</span>, listener) {
<span class="hljs-keyword">if</span> (elem.addEventListener) {
elem.addEventListener(<span class="hljs-built_in">type</span>, listener, <span class="hljs-literal">false</span>);
} <span class="hljs-keyword">else</span> <span class="hljs-keyword">if</span> (elem.attachEvent) {
elem.attachEvent(<span class="hljs-string">'on'</span> + <span class="hljs-built_in">type</span>, listener);
} <span class="hljs-keyword">else</span> {
// Because. cannot be linked to <span class="hljs-string">'on'</span> strings, only []
elem[<span class="hljs-string">'on'</span> + <span class="hljs-built_in">type</span>] = listener;
}
},
// Remove event handles
removeHandler: <span class="hljs-keyword">function</span>(elem, <span class="hljs-built_in">type</span>, listener) {
<span class="hljs-keyword">if</span> (elem.removeEventListener) {
elem.removeEventListener(<span class="hljs-built_in">type</span>, listener, <span class="hljs-literal">false</span>);
} <span class="hljs-keyword">else</span> <span class="hljs-keyword">if</span> (elem.detachEvent) {
elem.detachEvent(<span class="hljs-string">'on'</span> + <span class="hljs-built_in">type</span>, listener);
} <span class="hljs-keyword">else</span> {
elem[<span class="hljs-string">'on'</span> + <span class="hljs-built_in">type</span>] = null;
}
},
// Adding event proxy
addAgent: <span class="hljs-keyword">function</span> (elem, <span class="hljs-built_in">type</span>, agent, listener) {
elem.addEventListener(<span class="hljs-built_in">type</span>, <span class="hljs-keyword">function</span> (e) {
<span class="hljs-keyword">if</span> (e.target.matches(agent)) {
listener.call(e.target, e); // this points to e.target
}
});
},
// Cancel default behavior
preventDefault: <span class="hljs-keyword">function</span>(event) {
<span class="hljs-keyword">if</span> (event.preventDefault) {
event.preventDefault();
} <span class="hljs-keyword">else</span> {
event.returnValue = <span class="hljs-literal">false</span>;
}
},
// Prevent Event Bubble
stopPropagation: <span class="hljs-keyword">function</span>(event) {
<span class="hljs-keyword">if</span> (event.stopPropagation) {
event.stopPropagation();
} <span class="hljs-keyword">else</span> {
event.cancelBubble = <span class="hljs-literal">true</span>;
}
}
};
Reprinted from - Nuggets - white nickname