Preface
We can publish a subscription to the channel in redis. Anyone who listens to the channel can receive the published content!
redis subscription listening configuration class
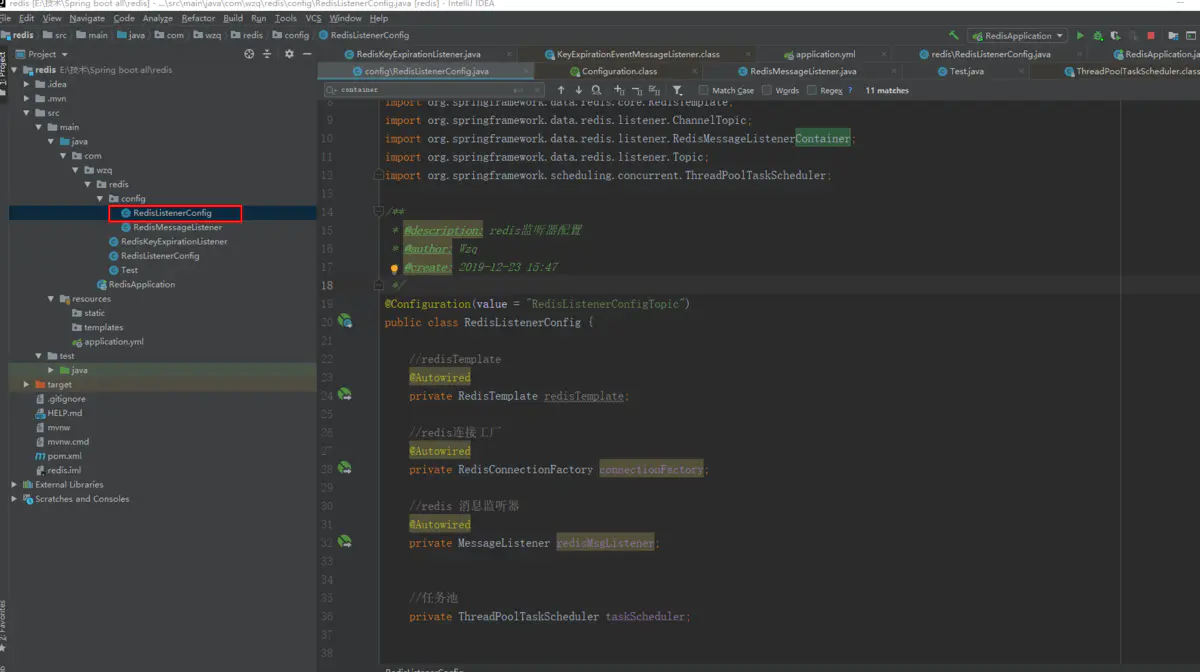
image.png
The code is as follows:
RedisListenerConfig.java
package com.wzq.redis.config; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.data.redis.connection.MessageListener; import org.springframework.data.redis.connection.RedisConnectionFactory; import org.springframework.data.redis.core.RedisTemplate; import org.springframework.data.redis.listener.ChannelTopic; import org.springframework.data.redis.listener.RedisMessageListenerContainer; import org.springframework.data.redis.listener.Topic; import org.springframework.scheduling.concurrent.ThreadPoolTaskScheduler; /** * @description: redis Monitor configuration * @author: Wzq * @create: 2019-12-23 15:47 */ @Configuration(value = "RedisListenerConfigTopic") public class RedisListenerConfig { //redisTemplate @Autowired private RedisTemplate redisTemplate; //redis connection factory @Autowired private RedisConnectionFactory connectionFactory; //redis message listener @Autowired private MessageListener redisMsgListener; //Task pool private ThreadPoolTaskScheduler taskScheduler; /** *@Description Create task pool and run thread to wait for redis message *@Param [] *@Return org.springframework.scheduling.concurrent.ThreadPoolTaskScheduler *@Author Wzq *@Date 2019/12/23 *@Time 15:51 */ @Bean public ThreadPoolTaskScheduler iniTaskScheduler(){ if(taskScheduler != null){ return taskScheduler; } taskScheduler = new ThreadPoolTaskScheduler(); taskScheduler.setPoolSize(20); return taskScheduler; } /** *@Description Define Redis listening container *@Param [] *@Return org.springframework.data.redis.listener.RedisMessageListenerContainer *@Author Wzq *@Date 2019/12/23 *@Time 15:52 */ @Bean public RedisMessageListenerContainer initRedisContainer(){ RedisMessageListenerContainer container = new RedisMessageListenerContainer(); //redis connection factory container.setConnectionFactory(connectionFactory); //Set up run task pool container.setTaskExecutor(iniTaskScheduler()); //Define a listening channel named topic1 Topic topic = new ChannelTopic("topic1"); //Define the message of Redis that the listener listens to container.addMessageListener(redisMsgListener,topic); return container; } }
Monitor class
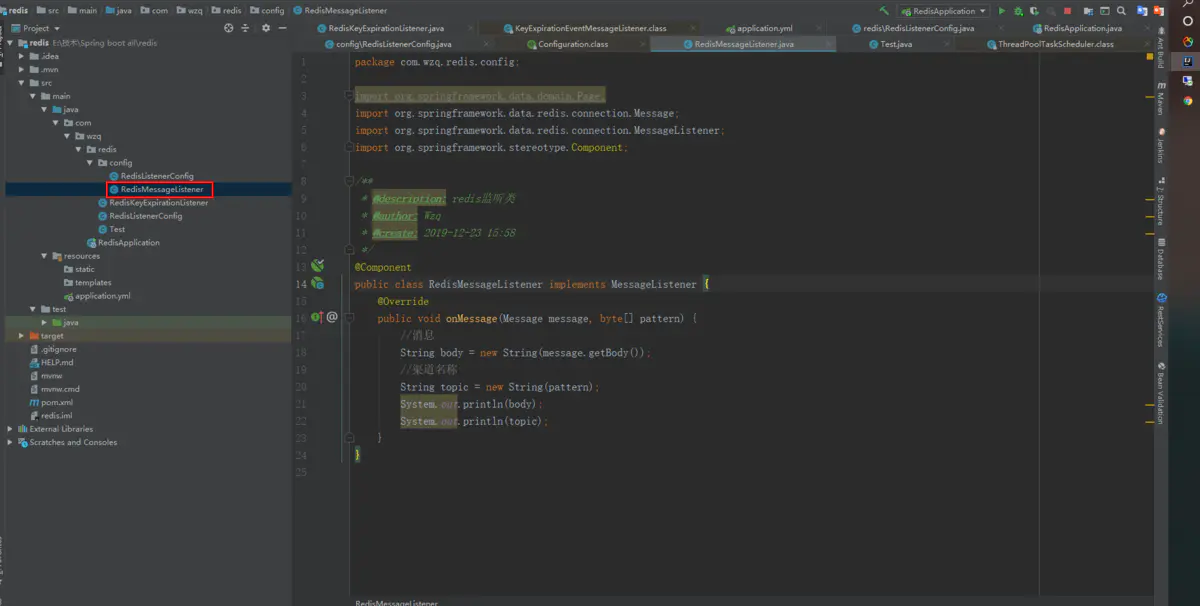
image.png
RedisMessageListener.java
package com.wzq.redis.config; import org.springframework.data.domain.Page; import org.springframework.data.redis.connection.Message; import org.springframework.data.redis.connection.MessageListener; import org.springframework.stereotype.Component; /** * @description: redis Monitor class * @author: Wzq * @create: 2019-12-23 15:58 */ @Component public class RedisMessageListener implements MessageListener { @Override public void onMessage(Message message, byte[] pattern) { //news String body = new String(message.getBody()); //Channel name String topic = new String(pattern); System.out.println(body); System.out.println(topic); } }
Publish and subscribe (there are two ways)
1. Use the redis command line to publish
Order:
PUBLISH topic1 hello!

image.png
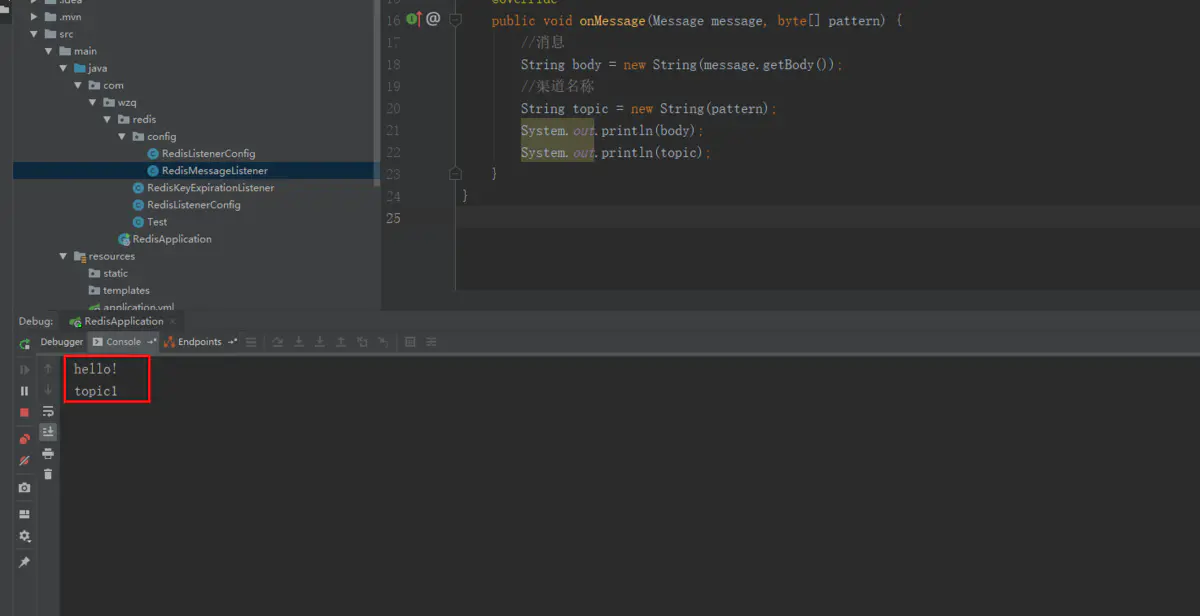
image.png
2. Publish using redisTemplate object
redisTemplate.convertAndSend("topic1","wzq Handsome!");
Detailed code TestController.java
package com.wzq.redis; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.redis.core.RedisTemplate; import org.springframework.data.redis.core.StringRedisTemplate; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; /** * @description: * @author: Wzq * @create: 2019-12-23 16:16 */ @RestController public class Test { @Autowired StringRedisTemplate redisTemplate; @GetMapping(value = "/test") public void test(){ redisTemplate.convertAndSend("topic1","wzq Handsome!"); } }
Visit:
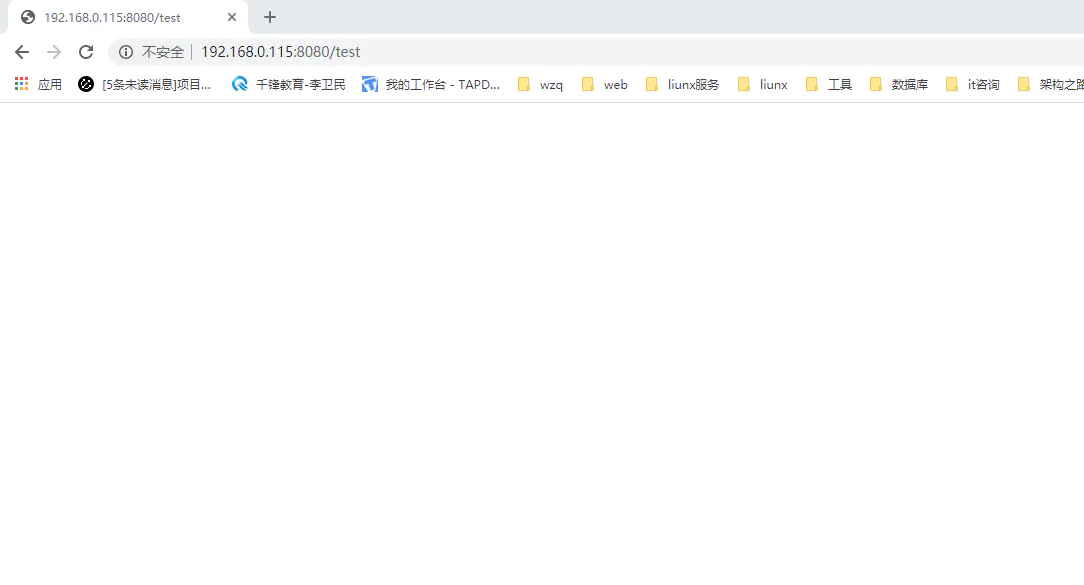
image.png
Received successfully!
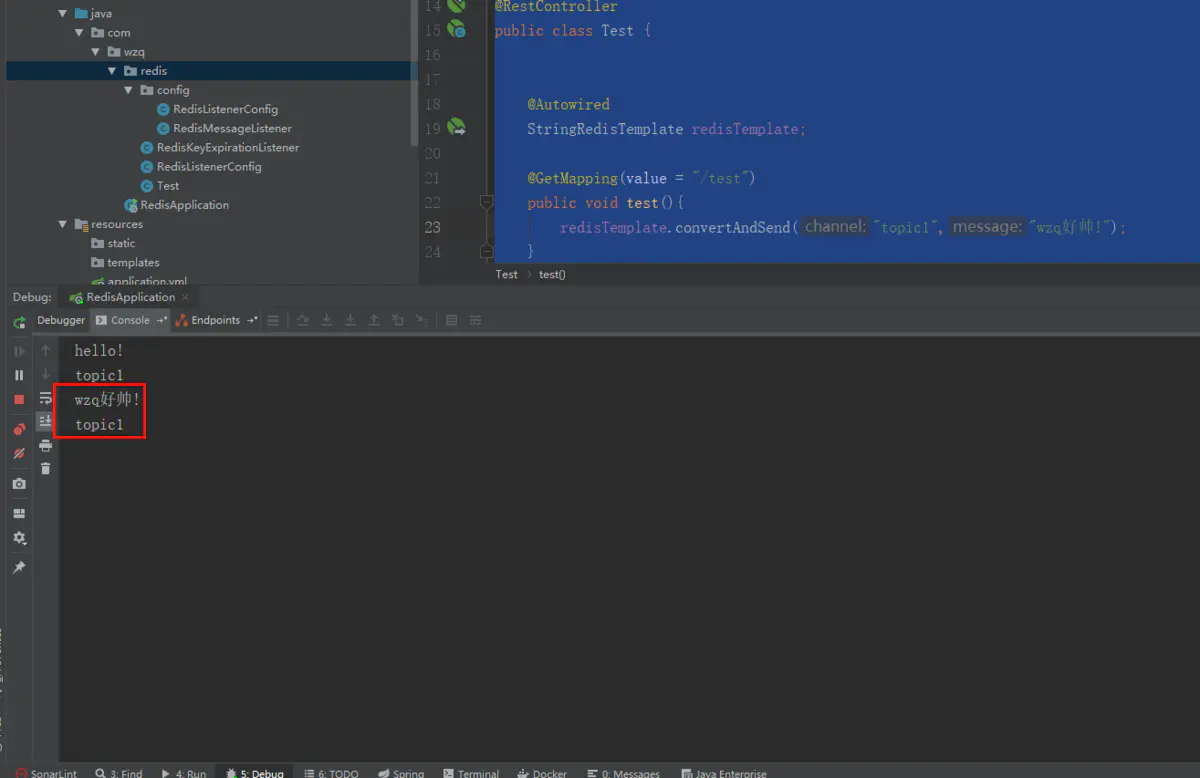
image.png
Personal wechat public, often update some practical dry goods:
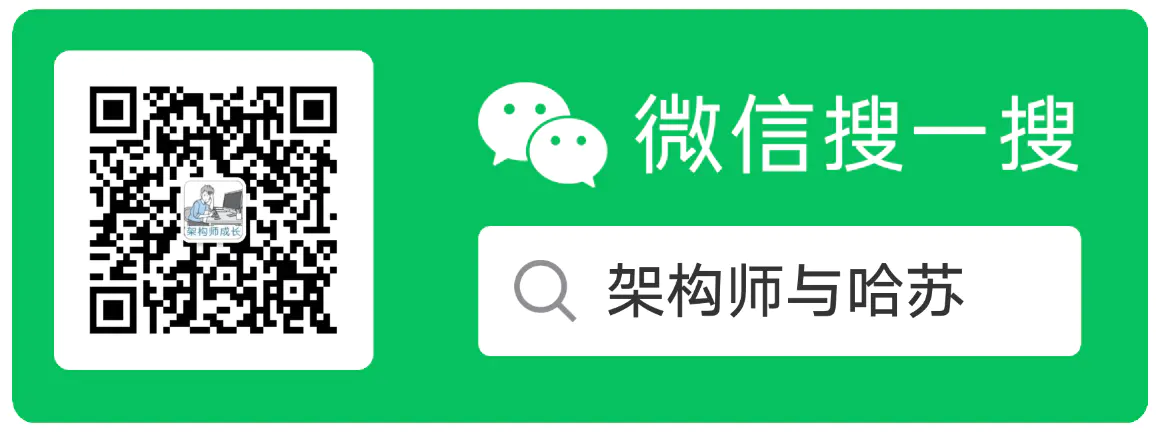