Spring boot + Vue implements RestFul API interaction
In the RestFul API, the front and back ends are separated, and the back end is not responsible for rendering the view. It is only responsible for returning the specified front end request and the back end Rest style API. After receiving the request from the front end, the back end will perform some corresponding operations according to the request method type and parameters. Then return the data in JSON format to the front end. The front end uses ajax technology to send http requests, and can use native APIs, such as xhr, fetch and promise APIs. $. ajax in Jquery, and axios, a commonly used third-party http library
1, Writing back-end API s with SpringBoot
1.1 write the simplest API service
The port I defined in application.yml is 3030, so subsequent requests will be http://localhost:3030 As the root path, I wrote the simplest API below, and then we started the project
@CrossOrigin @RestController public class StartController { @RequestMapping("/") public String Test() { return "hello"; } }
If you see this page, the project has been started successfully.
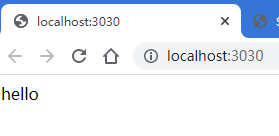
Next, let's explain the notes of this project
- @Cross origin projects that separate the front and back ends will encounter this problem. Use this annotation to solve cross domain requests
- @RestController uses this annotation, and the data we return will be automatically returned to the front end in JSON format
- @The RequestMapping("/") annotation indicates the path of the configuration request. We have not specified the request method, so any method can be accepted
1.2 common methods of receiving parameters
Other request methods are the same. Here we take the GET request as an example
// Without parameters, all data of parameters are generally obtained @GetMapping("/get") public String testGet() { return "I am GET request"; } // Carry request parameters to obtain data @GetMapping("/get/params") public String test (@RequestParam("name") String name) { return "name" + name; } // RestAPI to get the data in the request path. It is generally used to request a single piece of data @GetMapping("/get/{id}") public String testGet1(@PathVariable("id") Integer id) { return "id:" + id; } // Receiving entity parameters can be received as long as they correspond to the attributes of the entity one by one. Accept parameters as entity classes @GetMapping("/get/model") public Map<String,Object> testGet2(@ModelAttribute User user) { Map<String,Object> map = new HashMap<>(); map.put("user",user); map.put("code",200); map.put("msg","Request succeeded~"); return map; } // Accept entity classes in JSON format @GetMapping("/get/json/data") public Map<String,Object> testGet3(@RequestBody User user) { Map<String,Object> map = new HashMap<>(); map.put("user",user); map.put("code",200); map.put("msg","Request succeeded~"); return map; }
2, Using axios to complete ajax requests
2.1 basic configuration of Axios
Let's look at the instructions on the official website
Example method
The following are the available example methods. The specified configuration will be merged with the configuration of the instance. axios#request(config) axios#get(url[, config]) axios#delete(url[, config]) axios#head(url[, config]) axios#options(url[, config]) axios#post(url[, data[, config]]) axios#put(url[, data[, config]]) axios#patch(url[, data[, config]])
Instance configuration
{ url: '/user', methods: 'GET', data: { "username": "abc", "password": "123321" } ... }
Example:
// Direct request GET axios.get('/user') // The request parameters will be displayed in the url: / user? Username = Admin & password = 123456 axios.get("/user",{ params: { "username" : "admin", "password": "123456" } }) // The request parameters are configured in the way of configuration. The request parameters will be passed in the form of JSON string. If the content type is set as form in the header, you can use ordinary parameters to receive axios({ url: '/user', methods: 'post', data: { "username": "admin", "password": "123321" }, },{ headers: { "token": "123321" } })
2.2 axios instance
2.2.1 GET request
1, get request without parameters
The backend code receives a get request without parameters
// Without parameters @GetMapping("/get") public String testGet() { return "I am GET request"; }
Writing ajax from the front end
async function testGetAsync() { // GET request without parameters const {data: res} = await this.axios.get("http://localhost:3030/get") console.log(res) }
Operation results

2, get request with parameters
- The RequestParam annotation in SpringBoot is used to receive the data in the key value pair in the url Backend code:
// Carry request parameters @GetMapping("/get/params") public String test (@RequestParam("name") String name) { return "name" + name; }
Front end ajax code
// GET request with parameters const {data: res1} = await this.axios.get('http://localhost:3030/get/params',{ params: { name: 'coco' } }) console.log(res1)
The get request carries parameters. We can find the change of the request path, and the request parameters can be seen
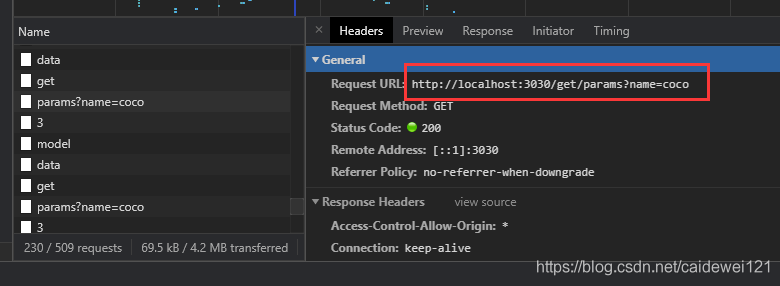
Response results
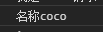
3, Request path with parameters
It should be added that this way of carrying parameters in the request path is in the standard Restful API format. Generally, it is used to obtain a single data in the get request or delete a record in the delete method Back end code
// RestAPI to get the data in the request path @GetMapping("/get/{id}") public String testGet1(@PathVariable("id") Integer id) { return "id:" + id; }
Front end ajax code
// The path carries parameters. I wrote the request path using the new syntax in ES6 const {data: res2} = await this.axios.get(`http://localhost:3030/get/3`) console.log(res2)
Response results

The above is where GET requests are used more
2.2.2 POST request
In RestFul API, it is generally used to submit FORM forms. The post request also carries more parameters than the get request. When I write projects, post requests are often used for login FORM submission, data addition, etc
For the convenience of testing, I wrote the following entity class. In order to avoid unnecessary trouble, I usually don't write constructors here
public class User { private String username; private Integer age; private String password; //getter, setter and toString methods are omitted }
1, Receive parameters as entity classes
- The ModelAttribute annotation in SpringBoot is used to receive objects (the data sent by the front end must correspond to the attributes of the entity one by one. If one does not correspond, the back end cannot inject the data sent by the front end into the entity)
Back end code
// Receiving entity parameters can be received as long as they correspond to the attributes of the entity one by one @PostMapping("/post/model") public Map<String,Object> testPost(@RequestBody User user) { System.out.println("Entity:"+user.toString()); Map<String,Object> map = new HashMap<>(); map.put("user",user); map.put("code",200); map.put("msg","Request succeeded~"); return map; }
Front end ajax code
// Receive entity class const {data: res3} = await this.axios.post('http://localhost:3030/post/model',{ age: 12, username: "admin", password: "123321" })
Supplementary notes
- When POST is used to submit data in axios, the data will be sent to the back end in application/json, which is different from the traditional form expression.
- So @ ModelAttribute cannot be used on the back end. If other methods are used to send post requests, we can set the value of content type in the header to application/x-www-form-urlencoded;charset=UTF-8
- Therefore, all data received in SpringBoot must be annotated with @ RequestBody, which means that the front-end data is received in JSON format
Operation results:
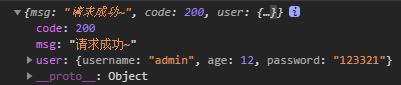
2.2.3 PUT request
PUT requests are often used to update data in Restful API. Using this, we can distinguish POST requests. The use method is almost the same as that of POST requests
Back end code
@PutMapping("/put/data") public Map<String,Object> testGet3(@RequestBody User user) { Map<String,Object> map = new HashMap<>(); map.put("user",user); map.put("code",200); map.put("msg","Got it put Request, entity class encapsulation succeeded~"); return map; }
Front end code
const {data: res4} = await this.axios.put('http://localhost:3030/put/data',{ age: 3, username: "coco", password: "adada" },{ headers: { "token": "123123131231dadawdw" } }) console.log(res4)
Operation effect

2.2.4 DELETE request
The DELETE request is used to DELETE the data in a record. Like the GET request, it is used to obtain the parameters in the url
Back end code
@DeleteMapping("/delete/{id}") public String testDelete(@PathVariable("id") Integer id) { return "delete: " + id; }
Front end code
const {data: result} = await this.axios.delete(`http://localhost:3030/delete/${5}`) console.log(result)
Operation effect

Well, that's the end of today's content~
If you have anything you want to know, you can put it forward